"""
================================================================================
Music theory Python package, chords module
Copyright (C) 2008, Bart Spaans
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
================================================================================
The chords module is a huge module that builds on the \
[refMingusCoreIntervals intervals] module. It can be used to generate and \
recognise a plethora of chords. The following overview groups some of the functions you are most likely to use together.
== Generate Diatonic Chords ==
* Triads
* triad
* triads
* Sevenths
* seventh
* sevenths
== Generate Absolute Chords ==
* Triads
* minor_triad
* major_triad
* diminished_triad
* Sixths
* minor_sixth
* major_sixth
* Sevenths
* minor_seventh
* major_seventh
* dominant_seventh
* minor_major_seventh
* minor_seventh_flat_five
* diminished_seventh
* Ninths
* minor_ninth
* major_ninth
* dominant_ninth
* Elevenths
* minor_eleventh
* eleventh
* Thirteenths
* minor_thirteenth
* major_thirteenth
* dominant_thirteenth
* Augmented chords
* augmented_triad
* augmented_major_seventh
* augmented_minor_seventh
* Suspended chords
* suspended_second_triad
* suspended_fourth_triad
* suspended_seventh
* suspended_fourth_ninth
* suspended_ninth
* Altered chords
* dominant_flat_ninth
* dominant_sharp_ninth
* dominant_flat_five
* sixth_ninth
* hendrix_chord
== Get Chords by Function ==
* Function
* tonic and tonic7
* supertonic and supertonic7
* mediant and mediant7
* subdominant and subdominant7
* dominant and dominant7
* submediant and submediant7
* Aliases
* I, II, III, IV, V, VI
* ii, iii, vi, vii
* I7, II7, III7, IV7, V7, VI7
* ii7, iii7, vi7
== Useful Functions ==
* determine - Can recognize all the chords that can be generated with from_shorthand (a lot) and their inversions.
* from_shorthand - Generates chords from shorthand (eg. 'Cmin7')
================================================================================
"""
import intervals
import notes
import diatonic
from mt_exceptions import NoteFormatError
# A cache for composed triads
_triads_cache = {}
# A cache for composed sevenths
_sevenths_cache = {}
# A dictionairy that can be used to present
# lookup chord abbreviations. This dictionairy is also
# used in determine_seventh()
chord_shorthand_meaning = {
# Triads
"m" : " minor triad",
"M" : " major triad",
"" : " major triad",
"dim" : " diminished triad",
# Augmented chords
"aug" : " augmented triad",
"+" : " augmented triad",
"7#5" : " augmented minor seventh",
"M7+5" : " augmented minor seventh",
"M7+" : " augmented major seventh",
"m7+" : " augmented minor seventh",
"7+" : " augmented major seventh",
# Suspended chords
"sus47" :" suspended seventh",
"sus4" :" suspended fourth triad",
"sus2" :" suspended second triad",
"sus" :" suspended fourth triad",
"11" :" eleventh",
"sus4b9" :" suspended fourth ninth",
"susb9" :" suspended fourth ninth",
# Sevenths
"m7" :" minor seventh",
"M7" :" major seventh",
"dom7" :" dominant seventh",
"7" :" dominant seventh",
"m7b5" :" half diminished seventh",
"dim7" :" diminished seventh",
"m/M7" :" minor/major seventh",
"mM7" :" minor/major seventh",
# Sixths
"m6" : " minor sixth",
"M6" : " major sixth",
"6" : " major sixth",
"6/7" : " dominant sixth",
"67": " dominant sixth",
"6/9" :" sixth ninth",
"69" : " sixth ninth",
# Ninths
"9" : " dominant ninth",
"7b9" : " dominant flat ninth",
"7#9" :" dominant sharp ninth",
"M9" :" major ninth",
"m9" :" minor ninth",
# Elevenths
"7#11" :" lydian dominant seventh",
"m11" :" minor eleventh",
# Thirteenths
"M13" :" major thirteenth",
"m13" :" minor thirteenth",
"13" :" dominant thirteenth",
# Altered Chords
"7b5" :" dominant flat five",
# Special
"hendrix" :" hendrix chord",
"7b12" :" hendrix chord",
"5" :" perfect fifth",
}
#===================================================================
# Triads
#===================================================================
# diatonic
def triad(note, key):
"""Returns the triad on note in key as a list.
Example:
{{{
>>> triad("E", "C")
["E", "G", "B"]
>>> triad("E", "B")
["E", "G#", "B"]
}}}"""
return [note, intervals.third(note, key), intervals.fifth(note, key)]
def triads(key):
"""Returns all the triads in key. Implemented using a cache."""
if _triads_cache.has_key(key):
return _triads_cache[key]
res = map(lambda x: triad(x, key), diatonic.get_notes(key))
_triads_cache[key] = res
return res
# absolute
def major_triad(note):
"""Builds a major triad on note.
Example:
{{{
>>> major_triad("C")
["C", "E", "G"]
}}}"""
return [note, intervals.major_third(note), intervals.perfect_fifth(note)]
def minor_triad(note):
"""Builds a minor triad on note.
Example:
{{{
>>> minor_triad("C")
["C", "Eb", "G"]
}}}"""
return [note, intervals.minor_third(note), intervals.perfect_fifth(note)]
def diminished_triad(note):
"""Builds a diminished triad on note.
Example:
{{{
>>> diminished_triad("C")
["C", "Eb", "Gb"]
}}}"""
return [note, intervals.minor_third(note), intervals.minor_fifth(note)]
def augmented_triad(note):
"""Builds an augmented triad on note.
Example:
{{{
>>> augmented_triad("C")
["C", "E", "G#"]
}}}"""
return [note, intervals.major_third(note),\
notes.augment(intervals.major_fifth(note))]
#===================================================================
# Sevenths
#===================================================================
# diatonic
def seventh(note, key):
"""Returns the seventh chord on note in key.
Example:
{{{
>>> seventh("C", "C")
["C", "E", "G", "B"]
}}}"""
return triad(note, key) + [intervals.seventh(note, key)]
def sevenths(key):
"""Returns all the sevenths chords in key in a list"""
if _sevenths_cache.has_key(key):
return _sevenths_cache[key]
res = map(lambda x: seventh(x, key), diatonic.get_notes(key))
_sevenths_cache[key] = res
return res
#absolute
def major_seventh(note):
"""Builds a major seventh on note.
Example:
{{{
>>> major_seventh("C")
["C", "E", "G", "B"]
}}}"""
return major_triad(note) + [intervals.major_seventh(note)]
def minor_seventh(note):
"""Builds a minor seventh on note.
Example:
{{{
>>> minor_seventh("C")
["C", "Eb", "G", "Bb"]
}}}"""
return minor_triad(note) + [intervals.minor_seventh(note)]
def dominant_seventh(note):
"""Builds a dominant seventh on note.
Example:
{{{
>>> dominant_seventh("C")
["C", "E", "G", "Bb"]
}}}"""
return major_triad(note) + [intervals.minor_seventh(note)]
def half_diminished_seventh(note):
"""Builds a half diminished seventh (=minor seventh flat five) \
chord on note.
Example:
{{{
>>> half_diminished_seventh("C")
["C", "Eb", "Gb", "Bb"]
}}}"""
return diminished_triad(note) + [intervals.minor_seventh(note)]
def minor_seventh_flat_five(note):
"""See half_diminished_seventh(note) for docs"""
return half_diminished_seventh(note)
def diminished_seventh(note):
"""Builds a diminished seventh chord on note.
Example:
{{{
>>> diminished_seventh("C")
["C", "Eb", "Gb", "Bbb"]
}}}"""
return diminished_triad(note) + [notes.diminish(\
intervals.minor_seventh(note))]
def
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:mingus-0.4.0.3.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
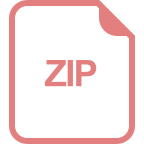
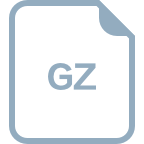
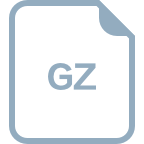
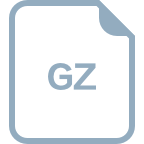
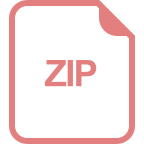
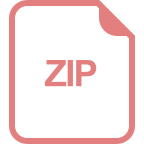
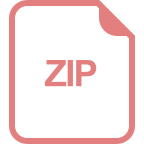
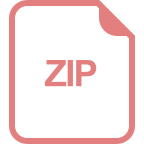
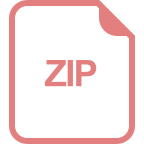
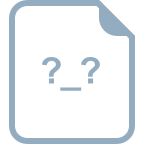
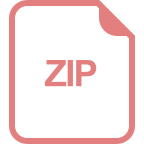
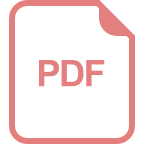
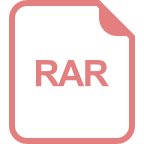
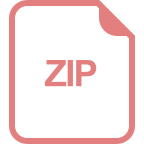
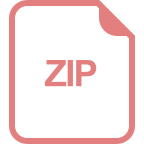
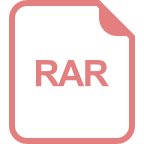
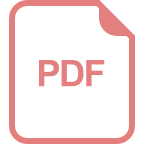
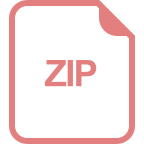
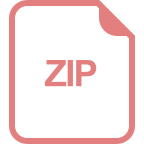
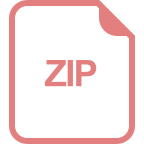
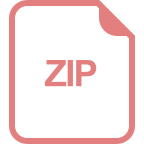
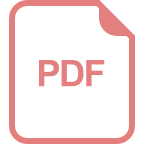
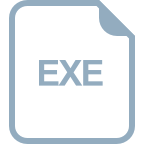
收起资源包目录


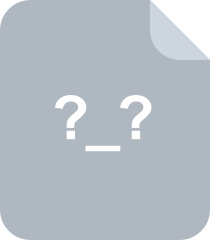


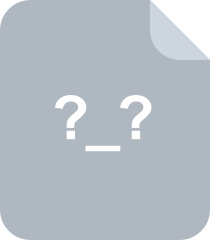

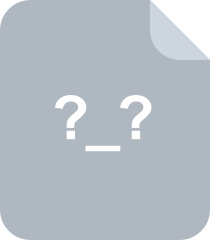
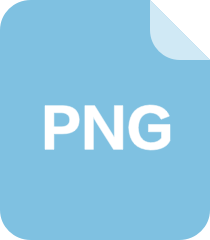

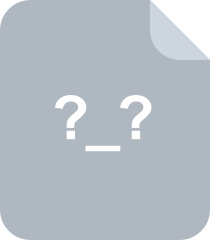

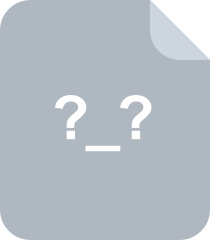
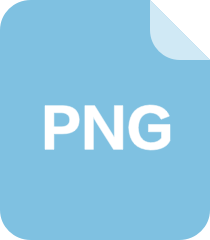

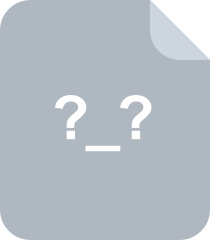
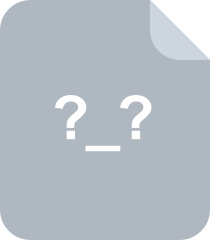
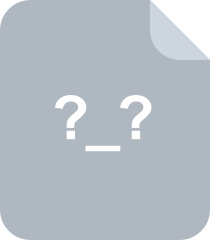
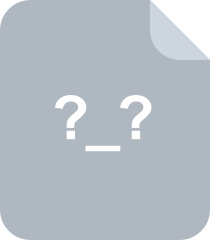
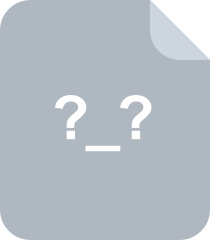
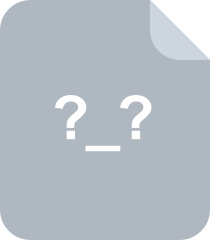
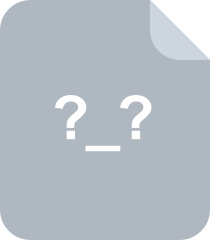
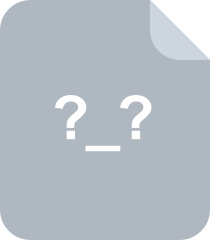
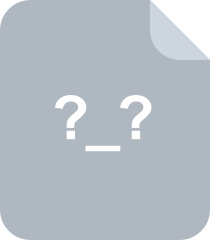
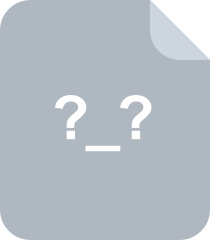
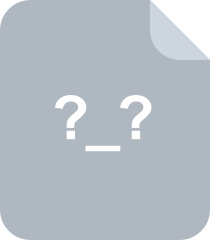
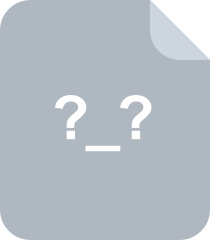
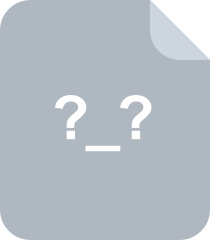
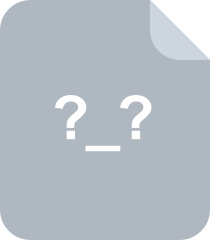
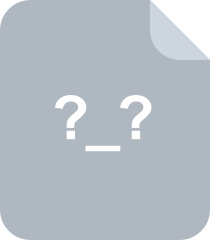
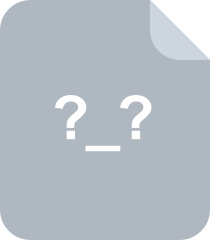
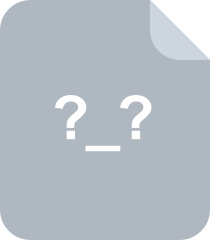
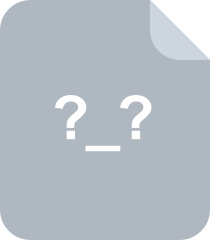
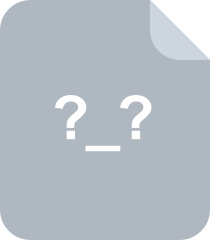
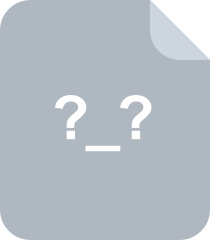
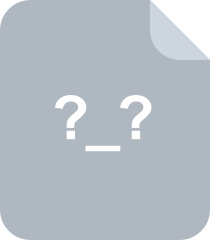
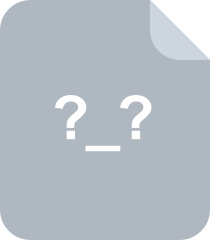


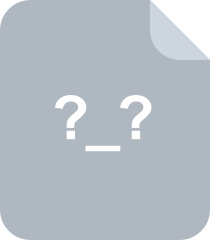
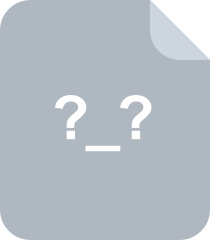
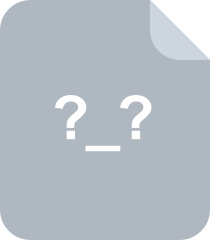
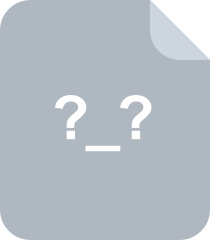
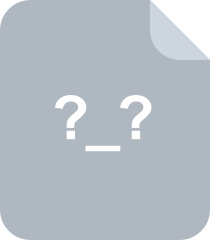
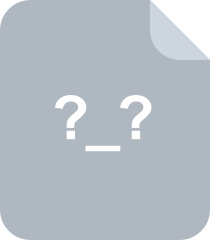
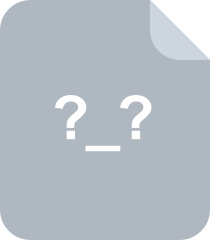
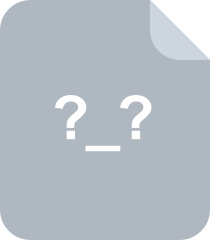
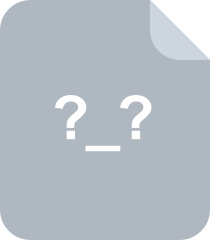
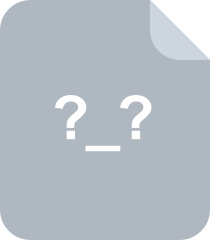

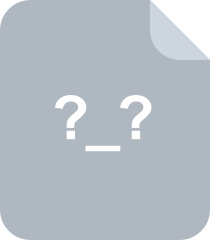
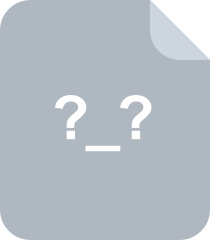
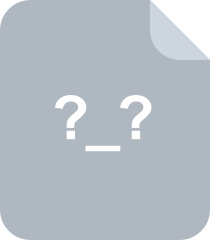

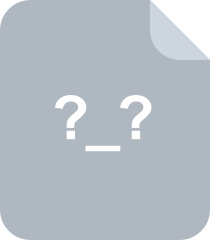
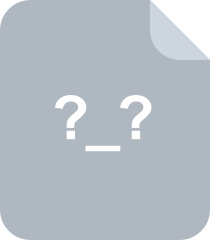
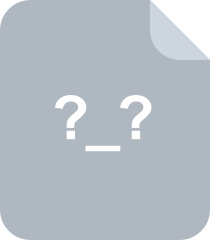
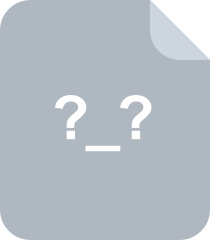
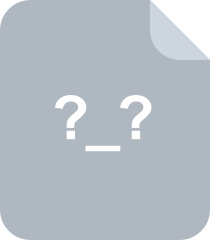
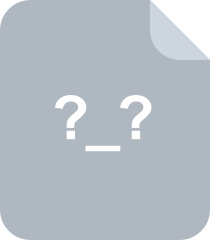
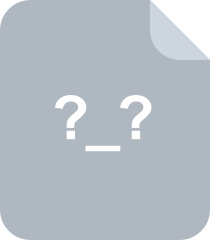
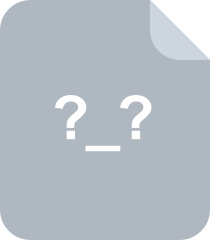
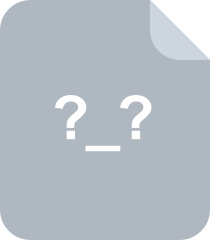

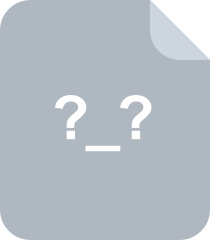
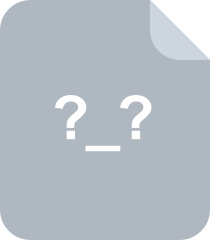
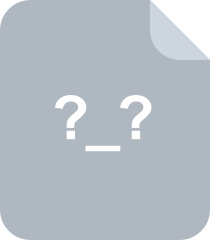
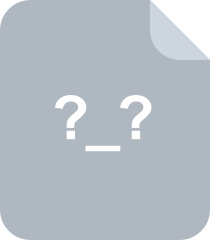
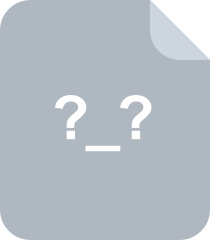
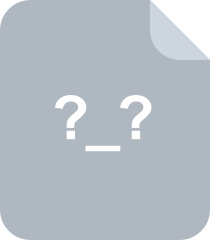
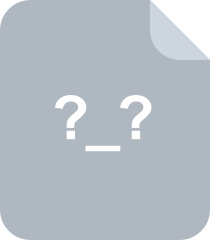
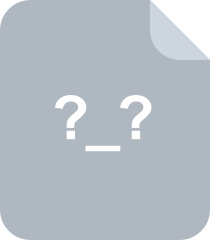
共 59 条
- 1
资源评论
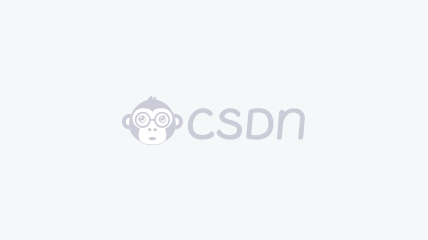

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

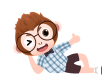
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


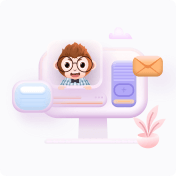
安全验证
文档复制为VIP权益,开通VIP直接复制
