#MIT License
#Copyright (c) 2017 Tim Wentzlau
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this software and associated documentation files (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
import time
from datetime import datetime
from kervi.values.kervi_value import KerviValue
from kervi.core.utility.component import KerviComponent
from kervi.config import Configuration
class NumberValue(KerviValue):
"""
Value that holds a float value.
If this value is an input it is shown as a slider on dashboards.
If is an output it is possible to specify different kinds of gauges.
"""
def __init__(self, name, **kwargs):
KerviValue.__init__(self, name, "number-value", **kwargs)
#self.spine = Spine()
self._min_value = -100
self._max_value = 100
self._unit = ""
self._type = None
self._display_unit = ""
self._default_value = 0.0
self._value = 0
self._delta = None
self._ui_parameters["type"] = ""
self._ui_parameters["value_icon"] = None
self._ui_parameters["min_integer_digits"] = 1
self._ui_parameters["min_fraction_digits"] = 1
self._ui_parameters["max_fraction_digits"] = 1
self._ui_parameters["show_sparkline"] = False
self._ui_parameters["pad_auto_center"] = False
self._ui_parameters["chart_buttons"] = True
self._ui_parameters["chart_grid"] = True
self._ui_parameters["chart_interval"] = "5min"
self._ui_parameters["chart_fill"] = True
self._ui_parameters["chart_point"] = 0
if Configuration:
unit_system = Configuration.display.unit_systems.default
chart_time_format = Configuration.display.unit_systems.systems[unit_system].datetime.chart
self._ui_parameters["chart_time_format"] = chart_time_format
else:
self._ui_parameters["chart_time_format"] = {
"millisecond": "h:mm:ss.SSS",
"second": "h:mm:ss",
"minute": "h:mm",
"hour": "h",
"day": "MMM D",
"week": "ll",
"month": "MMM YYYY",
"quarter":"[Q]Q - YYYY",
"year": "YYYY",
}
self._ui_parameters["tick"] = 1.0
self._ui_parameters["display_unit"] = True
self._last_reading = None
@property
def delta(self):
"""
Enter how much a the value should change before it triggers changes events and updates links.
:type: ``float``
"""
return self._delta
@delta.setter
def delta(self, value):
self._delta = value
@property
def max(self):
"""
Maximum value.
:type: ``float``
"""
return self._max_value
@max.setter
def max(self, value):
self._max_value = value
@property
def min(self):
"""
Minimum value.
:type: ``float``
"""
return self._min_value
@min.setter
def min(self, value):
self._min_value = value
@property
def unit(self):
"""
Metric Unit of value.
:type: ``str``
"""
return self._unit
@unit.setter
def unit(self, value):
self._unit = value
@property
def type(self):
"""
Value type.
:type: ``str``
"""
return self._type
@type.setter
def type(self, value):
self._type = value
@property
def display_unit(self):
"""
Display unit of value.
:type: ``str``
"""
if self._display_unit:
return self._display_unit
else:
config = Configuration.display.unit_systems
default_system = config.default
units = config.systems[default_system]
display_unit = units.get(self._type, self._unit)
return display_unit
@display_unit.setter
def display_unit(self, value):
self._display_unit = value
def _get_ui_parameters(self, ui_parameters):
ui_parameters["display_unit"] = self.display_unit
return ui_parameters
def _get_info(self, **kwargs):
return {
"isInput":self.is_input,
"unit":self._unit,
"value":self.value,
"maxValue":self._max_value,
"minValue":self._min_value,
"command":self.command,
"ranges":self._event_ranges,
"sparkline":self._sparkline,
}
def __delta_exceeded(self, value):
if self._delta is None:
return True
elif value is None:
return False
elif self._value is None:
return True
elif value >= self._value + self.delta:
return True
elif value <= self._value - self.delta:
return True
else:
return False
def _set_value(self, new_value, allow_persist=True):
if self.__delta_exceeded(new_value):
self.spine.log.debug(
"value change on input:{0} value:{1}",
self.component_id,
new_value
)
old_value = self._value
self._value = new_value
self.value_changed(new_value, old_value)
for observer in self._observers:
if isinstance(observer, tuple):
item, transformation = observer
if transformation:
item.kervi_value_changed(self, transformation(new_value))
else:
item.kervi_value_changed(self, new_value)
else:
observer.kervi_value_changed(self, new_value)
self._check_value_events(new_value, old_value)
if self._persist_value and allow_persist:
self.settings.store_value("value", self.value)
timestamp = datetime.utcnow().strftime("%Y-%m-%d %H:%M:%S")
if len(self._sparkline) == 0:
self._sparkline += [{"timestamp":timestamp, "value":new_value}]
elif len(self._sparkline) >= 10:
self._sparkline.pop(0)
self._sparkline += [{"timestamp":timestamp, "value":new_value}]
self._last_reading = time.clock()
val = {"value_id":self.component_id, "value":new_value, "timestamp":datetime.utcnow().strftime("%Y-%m-%d %H:%M:%S")}
self.spine.trigger_event(
"valueChanged",
self.component_id,
{"id":self.component_id, "value":new_value, "timestamp":datetime.utcnow().strftime("%Y-%m-%d %H:%M:%S")},
self._log_values,
groups=self.user_groups
)

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- C语言内存管理终极指南:malloc、free原理与防泄漏技巧.pdf
- C语言内存管理终极指南:malloc、free原理与避坑实战.pdf
- C语言嵌入式开发入门:LED控制与传感器数据采集实战.pdf
- C语言如何读取CSV文件?用fscanf实现数据清洗的5个关键步骤.pdf
- C语言内存泄漏自救手册:3种工具+5个案例快速排查.pdf
- C语言实战:手把手教你用STM32开发智能风扇项目.pdf
- C语言数据结构入门:链表实现与内存优化全流程.pdf
- C语言实现贪吃蛇:从控制台到图形界面的进阶之路.pdf
- C语言数组越界与指针运算:99%初学者踩过的坑.pdf
- Audio Developer v1.1.0
- C语言数据类型陷阱:从隐式转换到精度丢失的避坑手册.pdf
- C语言数据类型全解析:从int到结构体,彻底搞懂变量存储原理.pdf
- C语言算法入门:手把手教你用三大结构实现经典排序.pdf
- C语言算法入门:50道经典例题带你玩转逻辑思维.pdf
- C语言算法思维训练:排序、查找与链表的7天突破计划.pdf
- C语言网络编程:从Socket基础到简易聊天室开发.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


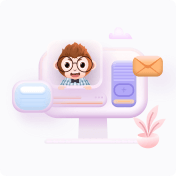