## Python Instructions for MLAEDT-3D
Compute the Euclidean Distance Transform of a 1d, 2d, or 3d labeled image containing multiple labels in a single pass with support for anisotropic dimensions.
### Python Installation
*Requires a C++ compiler*
The installation process depends on `edt.cpp` for the Python bindings derived from `edt.pyx`. `edt.hpp` contains the algorithm implementation.
```bash
pip install numpy
pip install edt
```
### Recompiling `edt.pyx`
*Requires Cython and a C++ compiler*
```bash
cd python
cython -3 --cplus edt.pyx # generates edt.cpp
python setup.py develop # compiles edt.cpp and edt.hpp
# together into a shared binary e.g. edt.cpython-36m-x86_64-linux-gnu.so
```
### Python Usage
Consult `help(edt)` after importing. The edt module contains: `edt` and `edtsq` which compute the euclidean and squared euclidean distance respectively. Both functions select dimension based on the shape of the numpy array fed to them. 1D, 2D, and 3D volumes are supported. 1D processing is extremely fast. Numpy boolean arrays are handled specially for faster processing.
If for some reason you'd like to use a specific 'D' function, `edt1d`, `edt1dsq`, `edt2d`, `edt2dsq`, `edt3d`, and `edt3dsq` are available.
The three optional parameters are `anisotropy`, `black_border`, and `order`. Anisotropy is used to correct for distortions in voxel space, e.g. if X and Y were acquired with a microscope, but the Z axis was cut more corsely.
`black_border` allows you to specify that the edges of the image should be considered in computing pixel distances (it's also slightly faster).
`order` allows the programmer to determine how the underlying array should be interpreted. `'C'` (C-order, XYZ, row-major) and `'F'` (Fortran-order, ZYX, column major) are supported. `'C'` order is the default.
`parallel` controls the number of threads. Set it <= 0 to automatically determine your CPU count.
```python
import edt
import numpy as np
# e.g. 6nm x 6nm x 30nm for the S1 dataset by Kasthuri et al., 2014
labels = np.ones(shape=(512, 512, 512), dtype=np.uint32, order='F')
dt = edt.edt(labels, anisotropy=(6, 6, 30), black_border=True, order='F', parallel=1)
```
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:edt-2.0.0.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
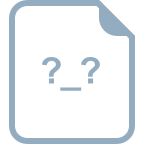
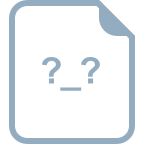
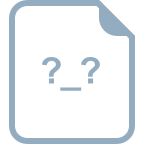
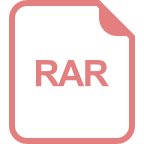
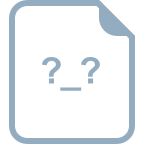
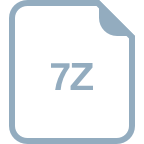
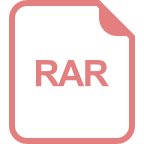
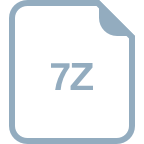
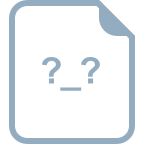
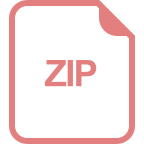
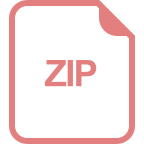
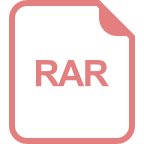
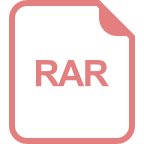
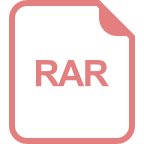
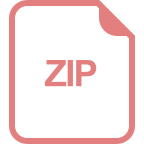
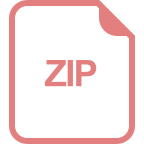
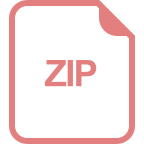
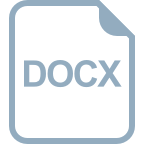
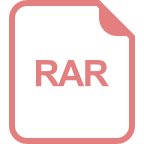
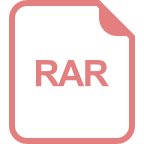
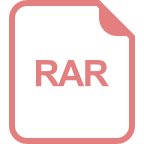
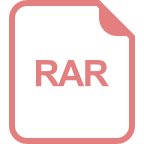
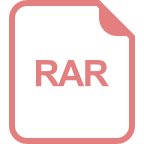
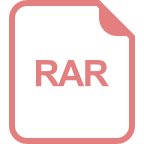
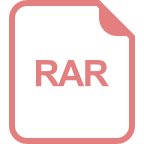
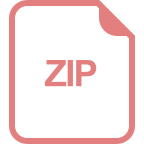
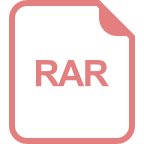
收起资源包目录


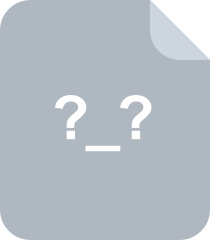
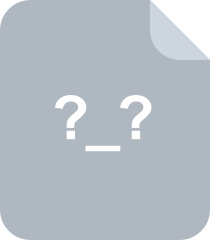
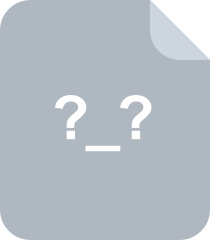
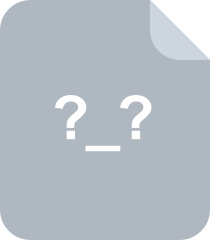
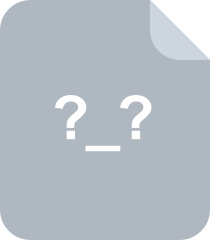
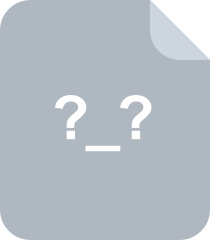
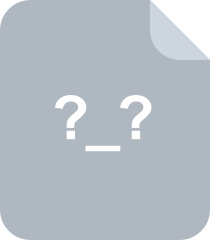

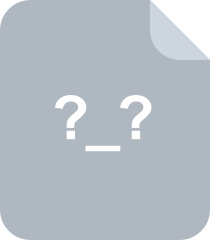
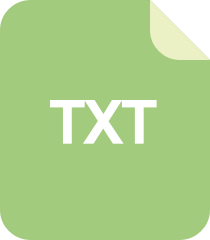
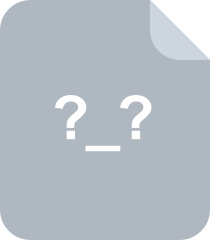
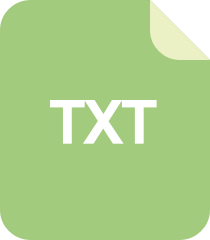
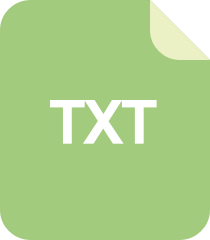
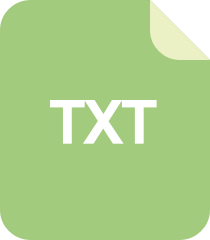
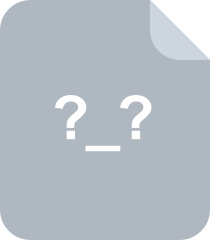
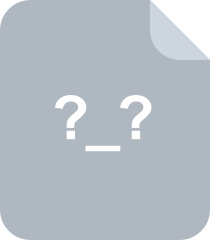
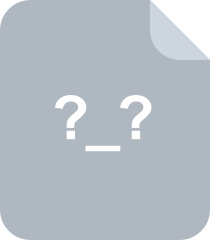
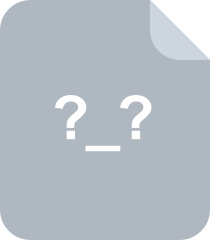
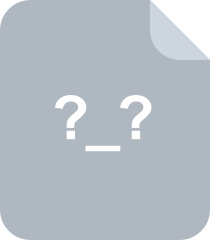
共 18 条
- 1
资源评论
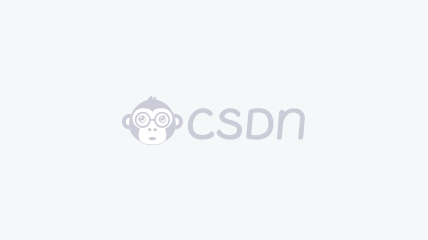

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

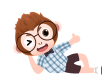
最新资源
- OpenCV开发资源.txt
- YOLO v3 的 PyTorch 实现,包括训练和测试,并可适用于用户定义的数据集.zip
- 安卓开发学习资源.txt
- yolo v3 物体检测系统的 Go 实现.zip
- YOLO v1 pytorch 实现.zip
- python爱心代码高级.txt
- Yolo for Android 和 iOS - 用 Kotlin 和 Swift 编写的实时移动深度学习对象检测.zip
- Yolnp 是一个基于 YOLO 检测车牌的项目.zip
- Unity Barracuda 上的 Tiny YOLOv2.zip
- Ultralytics YOLO iOS App 源代码可用于在你自己的 iOS 应用中运行 YOLOv8.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


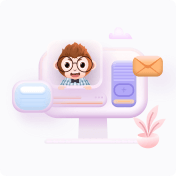
安全验证
文档复制为VIP权益,开通VIP直接复制
