# Django Easy Validator
this piece of software is inspired by the validation system of [laravel](https://laravel.com/docs/5.5/validation),
you can use this software to validate your POST/GET data easily and softly.







## Requirementse
- Python 3.4
- Python 3.5
- Python 3.6
## Installation
install using pip :
`pip install django-easy-validator`
install using source code:
`python setup.py install`
## Usage
1. create your own validator with Validator class.
```python
from validator import Validator
class LoginValidator(Validator):
username = 'required|email'
password = 'required'
message = {
'username': {
'required': _('username is required'),
'email': _('username is not an email address')
},
'password': {
'required': _('password is required')
}
}
```
2. spawn your validation class then call validate method to get it out.
```python
validator = LoginValidator({'username': 'michael', 'password': '12345678'})
status = validator.validate()
if status:
print("login succeed")
else:
errors = validator.get_message()
print(errors)
```
## Supported Rules
- [required](#required)
- [accepted](#accepted)
- [date](#data)
- [date_before](#date_before)
- [date_after](#date_after)
- [date_range](#date_range)
- [datetime](#datetime)
- [datetime_before](#datetime_before)
- [datetime_after](#datetime_after)
- [active_url](#active_url)
- [numberic](#numberic)
- [digits](#digits)
- [regex](#regex)
- [email](#email)
--------------------------------------------------------------
### required
```python
class LoginValidator(Validator):
username = 'required'
```
the field with required rule cant be null or empty.
### accepted
```python
class LoginValidator(Validator):
username = 'required'
remember_me = 'accepted'
```
the field with accepted must be one of the following strings, and the
string case is insensitive.
```python
['yes', 'no', 'true', 'false', '0', '1']
```
### date
```python
class RegisterValidator(Validator):
birthday = 'required|date:%Y-%m-%d'
```
the field with date must be a python date format string, the parameter
should be a format suit the datetime.datetime.strptime function.
### date_before
```python
class SubmitValidator(Validator):
due = 'required|date_before:2017-12-31'
```
the field with date_before must be a date format string suit xxxx-mm-dd.
### date_after
```python
class SubmitValidator(Validator):
date = 'required|date_after:2017-11-12'
```
the field with date_after must be a date format string suit xxxx-mm-dd.
### date_range
```python
class SubmitValidator(Validator):
date_range = 'required|date_range:2017-01-01,2017-12-31'
```
the field with date_range must be 2 date format strings and it should suit xxxx-mm-dd.
### datetime
```python
class RegisterValidator(Validator):
login_time = 'required|datetime:%Y-%m-%d %H:%M:%S'
```
the field with datetime and the parameter must be a datetime string suit the datetime.datetime.strptime function.
### datetime_before
```python
class LoginValidator(Validator):
time = 'required|datetime_before:2017-12-12 13:14:00'
```
the field with datetime_before must be a datetime string can strap to a datetime object and so as the parameter.
### datetime_after
```python
class LoginValidator(Validator):
time = 'required|datetime_after:2017-12-12 13:14:00'
```
the field with datetime_after must be a datetime string can strap to a datetime object and so as the parameter.
### active_url
```python
class ActiveURLValidator(Validator):
url = 'active_url'
```
the field with active_url must be a active url you could connect to and get reply.
### numberic
```python
class RegisterValidator(Validator):
id_number = 'required|numberic'
```
the field with numberic must be a number, it should be a integer not a float or something.
### digits
```python
class RegisterValidator(Validator):
product_series = 'required|digits'
```
the field with digits must only contains digits token.
### regex
```python
class RegisterValidator(Validator):
id_number = 'required|regex:^0[0-9]{5,10}$'
```
the field with regex must be a string, the parameter could be any regex pattern string, this
rule will match the field value with the paramter pattern out.
### email
```python
class RegisterValidator(Validator):
username = 'required|email'
```
the field with email must be a valid email address string.
## Advanced Topic
advanced topic
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:django-easy-validator-1.0.4.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
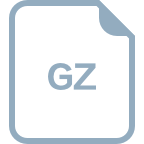
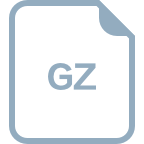
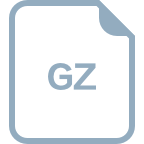
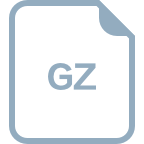
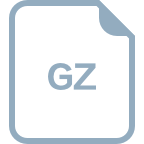
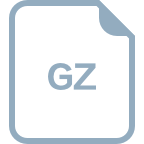
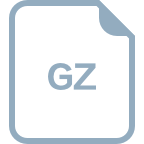
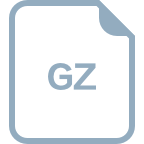
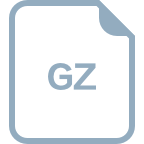
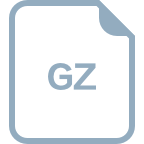
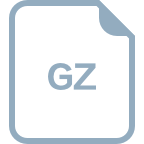
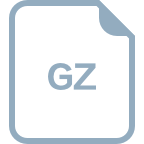
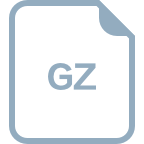
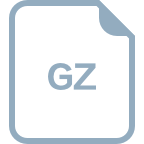
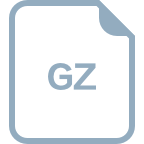
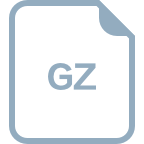
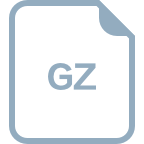
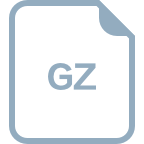
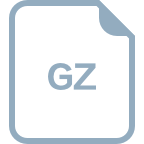
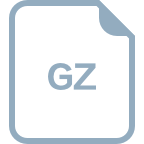
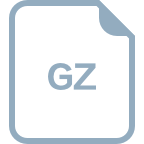
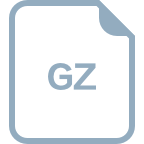
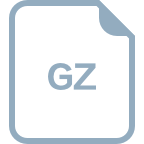
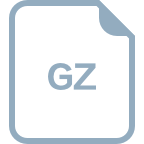
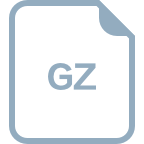
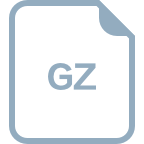
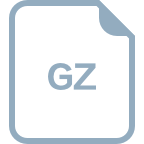
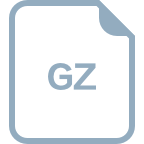
收起资源包目录


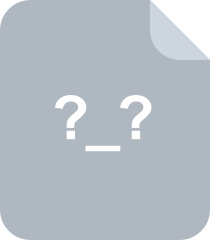
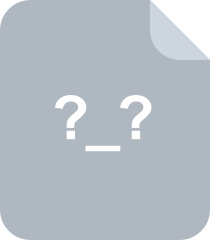

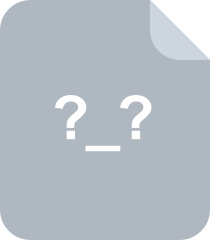
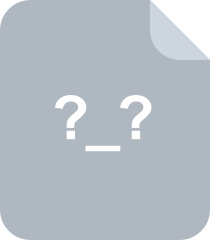
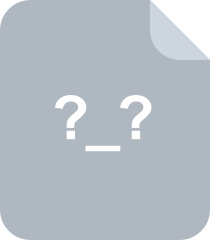
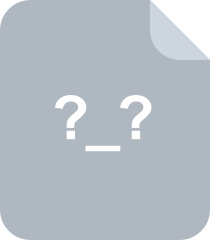
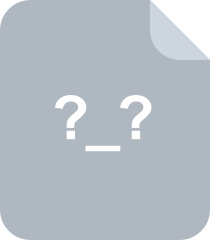

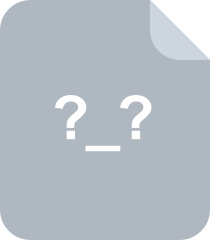
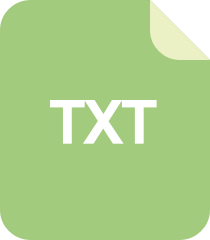
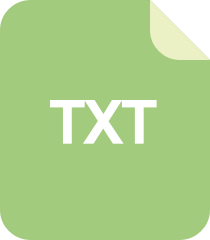
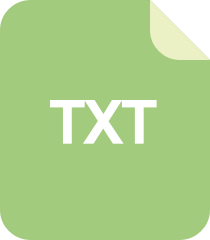
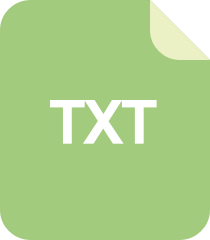
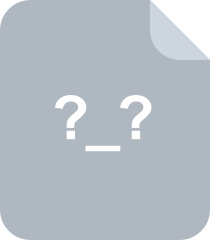

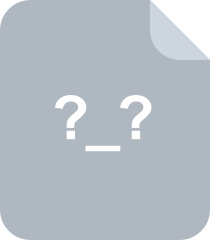
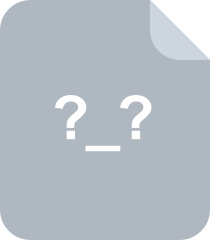
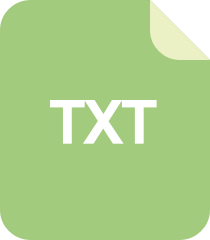
共 16 条
- 1
资源评论
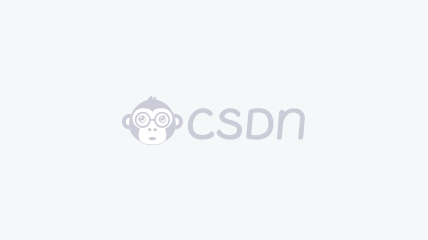

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

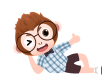
最新资源
- HAL库驱动TCS3200颜色识别模块-STM32F103ZET6
- boost电路参数详细计算.xls
- HTML+CSS+JavaScript实现带飘雪花效果的圣诞树
- 实习实训大作业-基于python的电商产品评论数据情感分析源码+说明(高分项目)
- HTML与CSS创建圣诞树及动态雪花效果
- 数据结构与算法:Python递归实现计算二叉树的深度
- 前端开发中的平安夜贺卡HTML代码示例
- C# WPF一个测弹力,显示曲线的工具 .zip
- 本地磁盘学习使用仅供参考
- 本地磁盘学习使用仅供参考
- 基于Kaggle数据集的泰坦尼克号幸存者预测机器学习实践
- 本地磁盘学习使用仅供参考
- 视频游戏人物检测35-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord数据集合集.rar
- 本地磁盘学习使用仅供参考
- 本地磁盘学习使用仅供参考
- HTML、CSS与JavaScript实现圣诞节雪花飘落效果
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


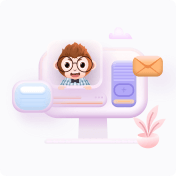
安全验证
文档复制为VIP权益,开通VIP直接复制
