""" Python library for interacting with the Sunlight Labs API.
The Sunlight Labs API (http://services.sunlightlabs.com/api/) provides basic
legislator data, conversion between ids, and zipcode-district lookups.
"""
__author__ = "James Turk (jturk@sunlightfoundation.com)"
__version__ = "0.6.0"
__copyright__ = "Copyright (c) 2009 Sunlight Labs"
__license__ = "BSD"
import sys
import warnings
if sys.version_info[0] == 3:
from urllib.parse import urlencode
from urllib.request import urlopen
from urllib.error import HTTPError
else:
from urllib import urlencode
from urllib2 import urlopen
from urllib2 import HTTPError
try:
import json
except ImportError:
import simplejson as json
class SunlightApiError(Exception):
""" Exception for Sunlight API errors """
# results #
class SunlightApiObject(object):
def __init__(self, d):
self.__dict__ = d
def __repr__(self):
return '%s(%r)' % (self.__class__.__name__, self.__dict__)
class Legislator(SunlightApiObject):
def __str__(self):
if self.nickname:
fname = self.nickname
else:
fname = self.firstname
return '%s. %s %s (%s-%s)' % (self.title, fname, self.lastname,
self.party, self.state)
class LegislatorSearchResult(SunlightApiObject):
def __init__(self, d):
self.legislator = Legislator(d['legislator'])
self.score = d['score']
def __str__(self):
return '%s %s' % (self.score, self.legislator)
class Committee(SunlightApiObject):
def __init__(self, d):
self.__dict__ = d
self.subcommittees = [Committee(sc['committee']) for sc in getattr(self, 'subcommittees', [])]
self.members = [Legislator(m['legislator']) for m in getattr(self, 'members', [])]
def __str__(self):
return '%s' % (self.name)
class District(SunlightApiObject):
def __str__(self):
return '%s-%s' % (self.state, self.number)
# namespaces #
class sunlight(object):
apikey = None
@staticmethod
def _apicall(func, params):
if sunlight.apikey is None:
raise SunlightApiError('Missing sunlight apikey')
url = 'http://services.sunlightlabs.com/api/%s.json?apikey=%s&%s' % \
(func, sunlight.apikey, urlencode(params))
try:
response = urlopen(url).read().decode()
return json.loads(response)['response']
except HTTPError, e:
raise SunlightApiError(e.read())
except (ValueError, KeyError), e:
raise SunlightApiError('Invalid Response')
class legislators(object):
@staticmethod
def get(**kwargs):
result = sunlight._apicall('legislators.get', kwargs)['legislator']
return Legislator(result)
@staticmethod
def getList(**kwargs):
results = sunlight._apicall('legislators.getList', kwargs)
return [Legislator(l['legislator']) for l in results['legislators']]
@staticmethod
def search(name, threshold=0.9, all_legislators=False):
params = {'name':name, 'threshold': threshold}
if all_legislators:
params['all_legislators'] = 1
results = sunlight._apicall('legislators.search', params)['results']
return [LegislatorSearchResult(r['result']) for r in results]
@staticmethod
def allForZip(zipcode):
results = sunlight._apicall('legislators.allForZip', {'zip':zipcode})
return [Legislator(l['legislator']) for l in results['legislators']]
@staticmethod
def allForLatLong(latitude, longitude):
params = {'latitude':latitude, 'longitude':longitude}
results = sunlight._apicall('legislators.allForLatLong', params)
return [Legislator(l['legislator']) for l in results['legislators']]
class committees(object):
@staticmethod
def get(committee_id):
results = sunlight._apicall('committees.get', {'id':committee_id})
return Committee(results['committee'])
@staticmethod
def getList(chamber):
results = sunlight._apicall('committees.getList', {'chamber':chamber})
return [Committee(c['committee']) for c in results['committees']]
@staticmethod
def allForLegislator(bioguide_id):
results = sunlight._apicall('committees.allForLegislator',
{'bioguide_id': bioguide_id})
return [Committee(c['committee']) for c in results['committees']]
class districts(object):
@staticmethod
def getDistrictsFromZip(zipcode):
results = sunlight._apicall('districts.getDistrictsFromZip', {'zip':zipcode})
return [District(r['district']) for r in results['districts']]
@staticmethod
def getZipsFromDistrict(state, district):
params = {'state':state, 'district':district}
results = sunlight._apicall('districts.getZipsFromDistrict', params)
return results['zips']
@staticmethod
def getDistrictFromLatLong(latitude, longitude):
params = {'latitude':latitude, 'longitude':longitude}
result = sunlight._apicall('districts.getDistrictFromLatLong', params)
return District(result['districts'][0]['district'])

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- 锂电池BMS的Matlab仿真模型
- 基于PID控制器的电动车充放电系统的simulink建模与仿真 对电动汽车蓄电池充放电控制策略的基本原理进行了介绍,包括 PI...
- 低电压故障穿越控制,基于模式平滑切的同步发电机低电压穿越控制方法(文章完全复现) 关键词:VSG,低电压穿越,模式平滑切
- 毕业设计:基于springboot+深度学习的人脸识别会议签到系统.zip
- java+web的源代码
- matlab语言与控制系统仿真
- 图书管理系统.zip,个人学习整理,仅供参考
- 图书 管理 系统,个人学习整理,仅供参考
- 基于Qt 5.13.0的图书管理系统.zip
- Jpeg Encoder ip jpeg编码器: 支持YCbCr422输入,422格式输出 每路数据为8bit 支持可编程量化表 纯verilog代码,方便移植到任何FPGA平台 使用vcs进行仿真
- 酒店管理系统,数据库开发
- 计算机联锁相关内容可指导
- python flask 调用百度api翻译项目(源码)
- Comsol太阳能吸收器,吸收太阳光谱与吸收效率
- STM32单片机开发的空气净化器项目,包括程序源码加原理图加pcb工程 主控采用stm32f103rct6可实现温湿度检测,ch2o检测,pwm风扇控制 程序注释详细,非常适合开发人员
- Django-eCommerce-website:具有许多高级自定义功能的 Django 电子商务网站、RDS Postgres...
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


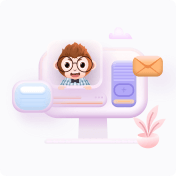