#### This project is forked from https://github.com/polkascan/py-substrate-interface
# Python Polymath Substrate Interface
[](https://pypi.org/project/polymath-substrate-interface/)
[](https://pypi.org/project/polymath-substrate-interface/)
Python Polymath Substrate Interface Library
## Description
This library specializes in interfacing with a Polymesh node, providing additional convenience methods to deal with
SCALE encoding/decoding (the default output and input format of the Substrate JSONRPC), metadata parsing, type registry
management and versioning of types.
## Documentation
https://polkascan.github.io/py-substrate-interface/
## Installation
```bash
pip install polymath-substrate-interface
```
### Initialization
The following examples show how to initialize for Polymesh chain:
#### Substrate Node Template
Compatible with https://github.com/substrate-developer-hub/substrate-node-template
```python
substrate = SubstrateInterface(
url="http://127.0.0.1:9933",
address_type=42,
type_registry_preset='substrate-node-template'
)
```
If custom types are introduced in the Substrate chain, the following example will add compatibility by creating a custom type
registry JSON file and including this during initialization:
```json
{
"runtime_id": 2,
"types": {
"MyCustomInt": "u32",
"MyStruct": {
"type": "struct",
"type_mapping": [
["account", "AccountId"],
["message", "Vec<u8>"]
]
}
},
"versioning": [
]
}
```
```python
custom_type_registry = load_type_registry_file("my-custom-types.json")
substrate = SubstrateInterface(
url="http://127.0.0.1:9933",
address_type=42,
type_registry_preset='substrate-node-template',
type_registry=custom_type_registry
)
```
## Keeping type registry presets up to date
When on-chain runtime upgrades occur, types used in call- or storage functions can be added or modified. Therefor it is
important to keep the type registry presets up to date. At the moment the type registry for Polymesh is being actively maintained for this library and an check and update procedure can be triggered with:
```python
substrate.update_type_registry_presets()
```
## Examples
### Get extrinsics for a certain block
```python
# Set block_hash to None for chaintip
block_hash = "0x588930468212316d8a75ede0bec0bc949451c164e2cea07ccfc425f497b077b7"
# Retrieve extrinsics in block
result = substrate.get_runtime_block(block_hash=block_hash)
for extrinsic in result['block']['extrinsics']:
if 'account_id' in extrinsic:
signed_by_address = ss58_encode(address=extrinsic['account_id'], address_type=2)
else:
signed_by_address = None
print('\nModule: {}\nCall: {}\nSigned by: {}'.format(
extrinsic['call_module'],
extrinsic['call_function'],
signed_by_address
))
# Loop through params
for param in extrinsic['params']:
if param['type'] == 'Address':
param['value'] = ss58_encode(address=param['value'], address_type=2)
if param['type'] == 'Compact<Balance>':
param['value'] = '{} DOT'.format(param['value'] / 10**12)
print("Param '{}': {}".format(param['name'], param['value']))
```
### Make a storage call
The modules and storage functions are provided in the metadata (see `substrate.get_metadata_storage_functions()`),
parameters will be automatically converted to SCALE-bytes (also including decoding of SS58 addresses).
```python
balance_info = substrate.get_runtime_state(
module='System',
storage_function='Account',
params=['5E9oDs9PjpsBbxXxRE9uMaZZhnBAV38n2ouLB28oecBDdeQo']
).get('result')
if balance_info:
print("\n\nCurrent free balance: {} KSM".format(
balance_info.get('data').get('free', 0) / 10**12
))
```
Or get a historic balance at a certain block hash:
```python
balance_info = substrate.get_runtime_state(
module='System',
storage_function='Account',
params=['5E9oDs9PjpsBbxXxRE9uMaZZhnBAV38n2ouLB28oecBDdeQo'],
block_hash=block_hash
).get('result')
if balance_info:
print("\n\nFree balance @ {}: {} KSM".format(
block_hash,
balance_info.get('data').get('free', 0) / 10**12
))
```
Or get all the key pairs of a map:
```python
# Get all the stash and controller bondings.
all_bonded_stash_ctrls = substrate.iterate_map(
module='Staking',
storage_function='Bonded',
block_hash=block_hash
)
```
### Create and send signed extrinsics
The following code snippet illustrates how to create a call, wrap it in an signed extrinsic and send it to the network:
```python
from substrateinterface import SubstrateInterface, SubstrateRequestException, Keypair
substrate = SubstrateInterface(
url="ws://127.0.0.1:9944",
address_type=42,
type_registry_preset='kusama'
)
keypair = Keypair.create_from_mnemonic('episode together nose spoon dose oil faculty zoo ankle evoke admit walnut')
call = substrate.compose_call(
call_module='Balances',
call_function='transfer',
call_params={
'dest': '5E9oDs9PjpsBbxXxRE9uMaZZhnBAV38n2ouLB28oecBDdeQo',
'value': 1 * 10**12
}
)
extrinsic = substrate.create_signed_extrinsic(call=call, keypair=keypair)
try:
result = substrate.submit_extrinsic(extrinsic, wait_for_inclusion=True)
print("Extrinsic '{}' sent and included in block '{}'".format(result['extrinsic_hash'], result['block_hash']))
except SubstrateRequestException as e:
print("Failed to send: {}".format(e))
```
### Create mortal extrinsics
By default `immortal` extrinsics are created, which means they have an indefinite lifetime for being included in a
block. However it is recommended to use specify an expiry window, so you know after a certain amount of time if the
extrinsic is not included in a block, it will be invalidated.
```python
extrinsic = substrate.create_signed_extrinsic(call=call, keypair=keypair, era={'period': 64})
```
The `period` specifies the number of blocks the extrinsic is valid counted from current head.
### Keypair creation and signing
```python
mnemonic = Keypair.generate_mnemonic()
keypair = Keypair.create_from_mnemonic(mnemonic)
signature = keypair.sign("Test123")
if keypair.verify("Test123", signature):
print('Verified')
```
By default a keypair is using SR25519 cryptography, alternatively ED25519 can be explictly specified:
```python
keypair = Keypair.create_from_mnemonic(mnemonic, crypto_type=KeypairType.ED25519)
```
### Creating keypairs with soft and hard key derivation paths
```python
mnemonic = Keypair.generate_mnemonic()
keypair = Keypair.create_from_uri(mnemonic + '//hard/soft')
```
By omitting the mnemonic the default development mnemonic is used:
```python
keypair = Keypair.create_from_uri('//Alice')
```
### Getting estimate of network fees for extrinsic in advance
```python
keypair = Keypair(ss58_address="EaG2CRhJWPb7qmdcJvy3LiWdh26Jreu9Dx6R1rXxPmYXoDk")
call = self.kusama_substrate.compose_call(
call_module='Balances',
call_function='transfer',
call_params={
'dest': 'EaG2CRhJWPb7qmdcJvy3LiWdh26Jreu9Dx6R1rXxPmYXoDk',
'value': 2 * 10 ** 3
}
)
payment_info = self.kusama_substrate.get_payment_info(call=call, keypair=keypair)
```
### Offline signing of extrinsics
This example generates a signature payload which can be signed on another (offline) machine and later on sent to the
network with the generated signature.
Generate signature payload on online machine:
```python
substrate = SubstrateInterface(
url="http://127.0.0.1:9933",
address_type=42,
type_registry_preset='substrate-node-template',
)
call = substrate.compose_call(
call_module='Balances',
call_function='transfer',
call_params={
'dest': '5GrwvaEF5zXb26Fz9rcQpDWS57CtERHpNehXC

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- 基于Python+yolo水表识别 框架html + css + jquery + python +idea + django + MySQL + yolo
- 圣诞节倒计时工具制作-代码.zip
- multisim声控流水灯仿真电路设计 功能: 制作一个声控的LED流水灯电路,20只灯珠依次点亮,当 音量高时流水灯切快,当音调低时流水灯切慢 1.制作拾音整形电路; 2.制作LED驱动电路; 3
- Petrel三维地质建模与裂缝建模 内容包括1数据导入,2构造建模,3岩相建模,4属性建模,裂缝建模,总共十七节精讲 可以边看边操作的项目数据 对应的是2020年的新版精讲视频 包括视频
- 机械设计大件物品分拣生产线设计sw17可编辑非常好的设计图纸100%好用.zip
- 基于Servlet+Jsp+Mysql实现的学生管理系统(两个版本idea eclipse)
- 毕业设计-流程或者教程.zip
- 基于S7-200 PLC和MCGS的电机转速闭环速度控制系统 带解释的梯形图程序,接线图原理图图纸,io分配,组态画面
- 如何快速使用阿里云OSS-PHP-SDK完成常见文件操作,如创建存储空间(Bucket)、上传文件(Object)、下载文件等
- Net-SNMP V5.7.0 Windows安装包
- java飞机大战小游戏代码+答辩ppt
- 企业人事管理系统,使用C#和Sql Server开发,包含详细的使用说明文档
- WinHex单文件版x32x64
- 机械设计常用电伴热带温度控制器sw19可编辑非常好的设计图纸100%好用.zip
- 群晖 DSM DS 218 PLAY VERSION文件
- 永磁同步电机控制资料,内容详细,包括参考lunwen,公式推导,模型搭建过程,电机控制书籍等等,CSDN沉沙
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


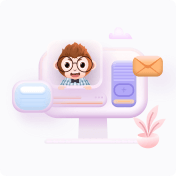