# libimagequant—Image Quantization Library
Small, portable C library for high-quality conversion of RGBA images to 8-bit indexed-color (palette) images.
It's powering [pngquant2](https://pngquant.org).
## License
Libimagequant is dual-licensed:
* For Free/Libre Open Source Software it's available under GPL v3 or later with additional [copyright notices](https://raw.github.com/ImageOptim/libimagequant/master/COPYRIGHT) for older parts of the code.
* For use in closed-source software, AppStore distribution, and other non-GPL uses, you can [obtain a commercial license](https://supso.org/projects/pngquant). Feel free to ask [email protected] for details and custom licensing terms if you need them.
## Download
The [library](https://pngquant.org/lib) is currently a part of the [pngquant2 project](https://pngquant.org). [Repository](https://github.com/ImageOptim/libimagequant).
## Compiling and Linking
The library can be linked with ANSI C, C++, [C#](https://github.com/ImageOptim/libimagequant/blob/master/libimagequant.cs), [Rust](https://github.com/pornel/libimagequant-rust), [Java](https://github.com/ImageOptim/libimagequant/tree/master/org/pngquant) and [Golang](https://github.com/larrabee/go-imagequant) programs. It has no external dependencies.
To build on Unix-like systems run:
make static
it will create `libimagequant.a` which you can link with your program.
gcc yourprogram.c /path/to/libimagequant.a
On BSD, use `gmake` (GNU make) rather than the native `make`.
Alternatively you can compile the library with your program simply by including all `.c` files (and define `NDEBUG` to get a fast version):
gcc -std=c99 -O3 -DNDEBUG libimagequant/*.c yourprogram.c
### Building for use in Rust programs
In [Rust](https://www.rust-lang.org/) you can use Cargo to build the library. Add [`imagequant`](https://crates.io/crates/imagequant) to dependencies of the Rust program. You can also use `cargo build` in [`imagequant-sys`](https://crates.io/crates/imagequant-sys) to build `libimagequant.a` for any C-compatible language.
### Building for Java JNI
To build Java JNI interface, ensure `JAVA_HOME` is set to your JDK directory, and run:
# export JAVA_HOME=$(locate include/jni.h) # you may need to set JAVA_HOME first
make java
It will create `libimagequant.jnilib` and classes in `org/pngquant/`.
On Windows run `make java-dll` and it'll create `libimagequant.dll` instead.
### Compiling on Windows/Visual Studio
The library can be compiled with any C compiler that has at least basic support for C99 (GCC, clang, ICC, C++ Builder, even Tiny C Compiler), but Visual Studio 2012 and older are not up to date with the 1999 C standard. Use Visual Studio **2015** and the [MSVC-compatible branch of the library](https://github.com/ImageOptim/libimagequant/tree/msvc).
To build on Windows, install CMake and use it to generate a makefile/project for your build system.
Build instructions
mkdir build
cd build
cmake ..
cmake --build .
To generate a 64-bit Visual Studio project instead:
mkdir build
cd build
cmake -G "Visual Studio 15 2017 Win64" ..
cmake --build .
### Building as shared library
To build on Unix-like systems run:
./configure --prefix=/usr
make libimagequant.so
To install on Unix-like systems run:
make install
## Overview
The basic flow is:
1. Create attributes object and configure the library.
2. Create image object from RGBA pixels or data source.
3. Perform quantization (generate palette).
4. Store remapped image and final palette.
5. Free memory.
Please note that libimagequant only handles raw uncompressed arrays of pixels in memory and is completely independent of any file format.
<p>
/* See example.c for the full code! */
#include "libimagequant.h"
liq_attr *attr = liq_attr_create();
liq_image *image = liq_image_create_rgba(attr, example_bitmap_rgba, width, height, 0);
liq_result *res;
liq_image_quantize(image, attr, &res);
liq_write_remapped_image(res, image, example_bitmap_8bpp, example_bitmap_size);
const liq_palette *pal = liq_get_palette(res);
// Save the image and the palette now.
for(int i=0; i < pal->count; i++) {
example_copy_palette_entry(pal->entries[i]);
}
// You'll need a PNG library to write to a file.
example_write_image(example_bitmap_8bpp);
liq_result_destroy(res);
liq_image_destroy(image);
liq_attr_destroy(attr);
Functions returning `liq_error` return `LIQ_OK` (`0`) on success and non-zero on error.
It's safe to pass `NULL` to any function accepting `liq_attr`, `liq_image`, `liq_result` (in that case the error code `LIQ_INVALID_POINTER` will be returned). These objects can be reused multiple times.
There are 3 ways to create image object for quantization:
* `liq_image_create_rgba()` for simple, contiguous RGBA pixel arrays (width×height×4 bytes large bitmap).
* `liq_image_create_rgba_rows()` for non-contiguous RGBA pixel arrays (that have padding between rows or reverse order, e.g. BMP).
* `liq_image_create_custom()` for RGB, ABGR, YUV and all other formats that can be converted on-the-fly to RGBA (you have to supply the conversion function).
Note that "image" here means raw uncompressed pixels. If you have a compressed image file, such as PNG, you must use another library (e.g. libpng or lodepng) to decode it first.
You'll find full example code in "example.c" file in the library source directory.
## Functions
----
liq_attr* liq_attr_create(void);
Returns object that will hold initial settings (attributes) for the library. The object should be freed using `liq_attr_destroy()` after it's no longer needed.
Returns `NULL` in the unlikely case that the library cannot run on the current machine (e.g. the library has been compiled for SSE-capable x86 CPU and run on VIA C3 CPU).
----
liq_error liq_set_max_colors(liq_attr* attr, int colors);
Specifies maximum number of colors to use. The default is 256. Instead of setting a fixed limit it's better to use `liq_set_quality()`.
The first argument is attributes object from `liq_attr_create()`.
Returns `LIQ_VALUE_OUT_OF_RANGE` if number of colors is outside the range 2-256.
----
int liq_get_max_colors(liq_attr* attr);
Returns the value set by `liq_set_max_colors()`.
----
liq_error liq_set_quality(liq_attr* attr, int minimum, int maximum);
Quality is in range `0` (worst) to `100` (best) and values are analoguous to JPEG quality (i.e. `80` is usually good enough).
Quantization will attempt to use the lowest number of colors needed to achieve `maximum` quality. `maximum` value of `100` is the default and means conversion as good as possible.
If it's not possible to convert the image with at least `minimum` quality (i.e. 256 colors is not enough to meet the minimum quality), then `liq_image_quantize()` will fail. The default minimum is `0` (proceeds regardless of quality).
Quality measures how well the generated palette fits image given to `liq_image_quantize()`. If a different image is remapped with `liq_write_remapped_image()` then actual quality may be different.
Regardless of the quality settings the number of colors won't exceed the maximum (see `liq_set_max_colors()`).
The first argument is attributes object from `liq_attr_create()`.
Returns `LIQ_VALUE_OUT_OF_RANGE` if target is lower than minimum or any of them is outside the 0-100 range.
Returns `LIQ_INVALID_POINTER` if `attr` appears to be invalid.
liq_attr *attr = liq_attr_create();
liq_set_quality(attr, 50, 80); // use quality 80 if possible. Give up if quality drops below 50.
----
int liq_get_min_quality(liq_attr* attr);
Returns the lower bound set by `liq_set_quality()`.
----
int liq_get_max_quality(liq_attr* attr);
Returns the upper bound set by `liq_set_quality()`.
----
liq_image *liq_image_create_rgba(liq_attr *attr, void* pixels, int width, int height, double gamma);
Creates an object
没有合适的资源?快使用搜索试试~ 我知道了~
PyPI 官网下载 | libimagequant2-2.14.2.tar.gz
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
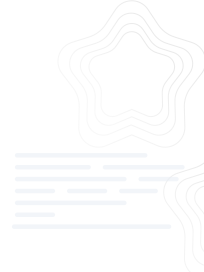
共57个文件
c:9个
h:7个
md:5个

资源来自pypi官网。 资源全名:libimagequant2-2.14.2.tar.gz
资源推荐
资源详情
资源评论
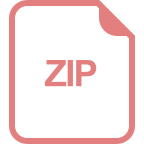
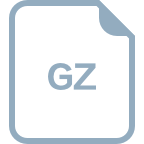
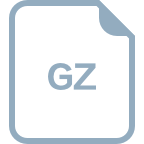
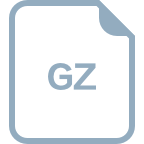
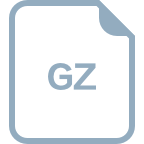
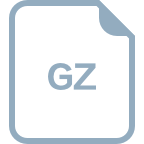
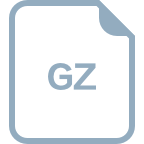
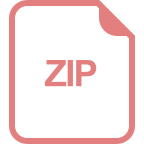
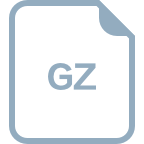
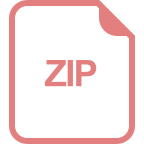
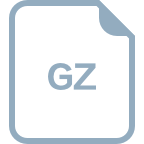
收起资源包目录


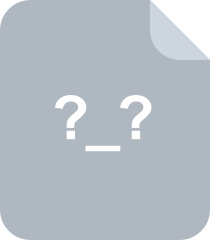
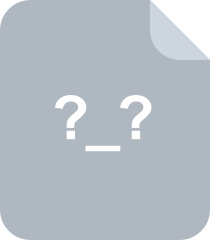

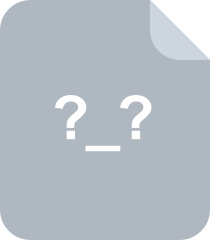

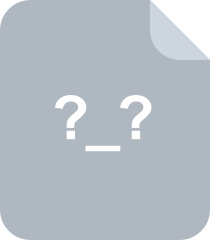
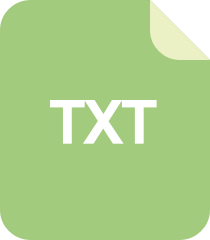
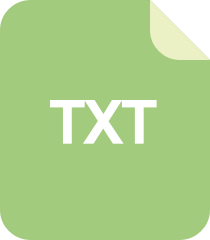
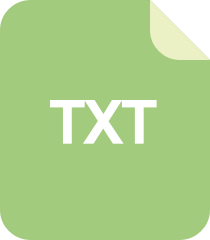
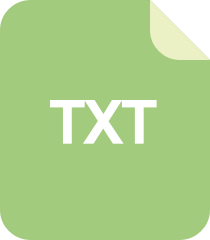
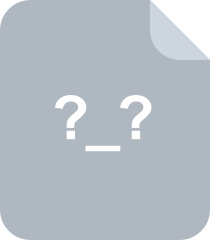
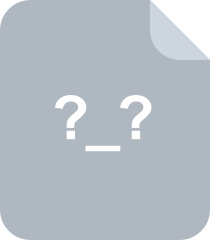
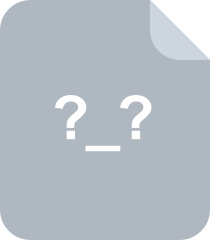

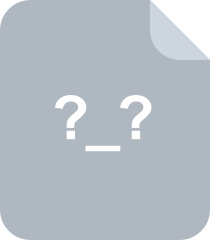
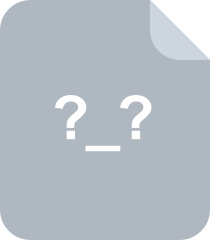
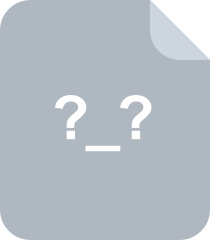
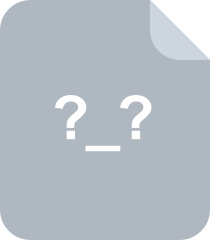
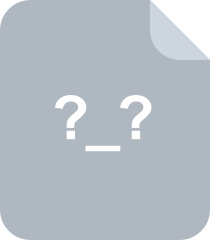
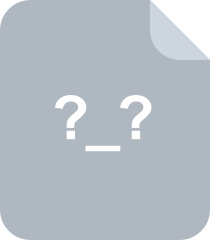


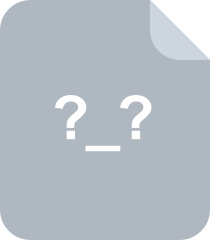
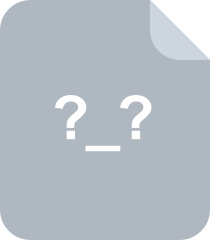
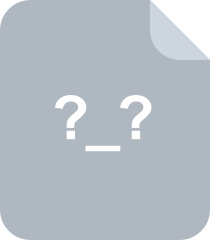

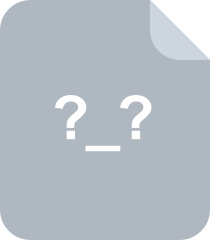
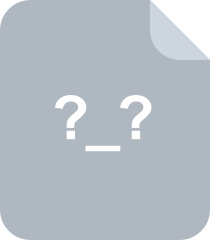
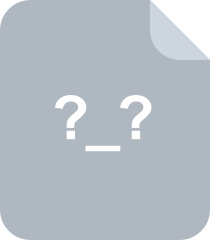
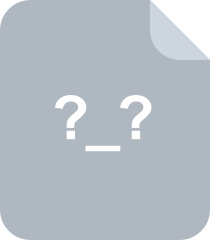

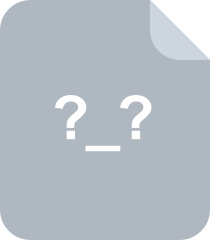
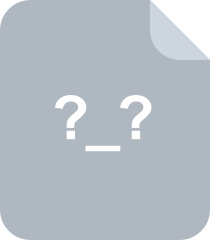
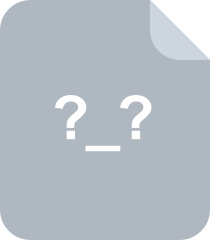
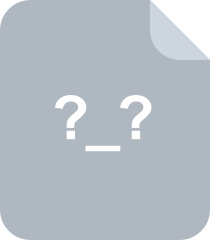
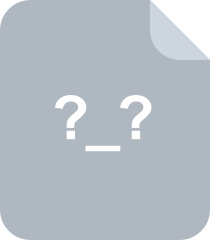
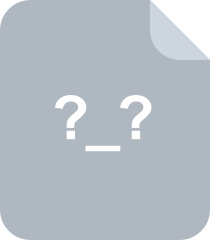
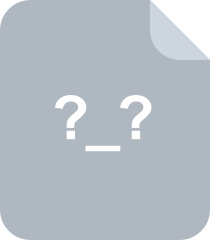
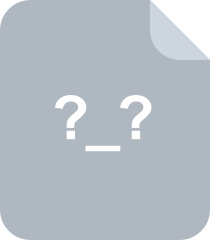
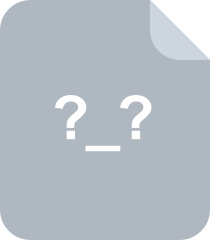
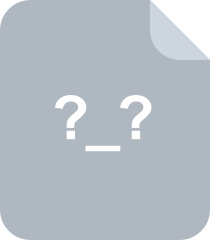


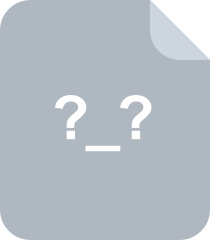
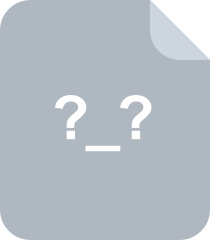
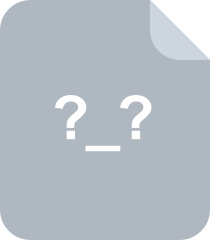
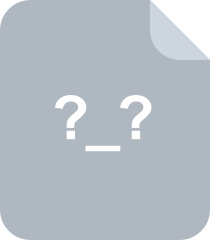
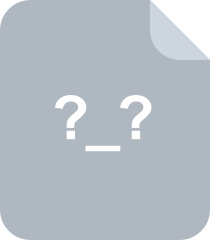
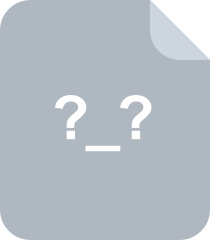

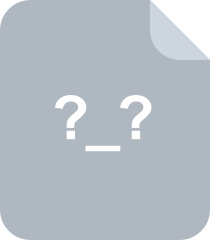
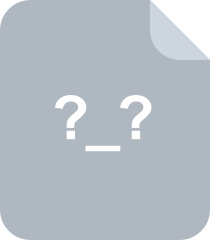
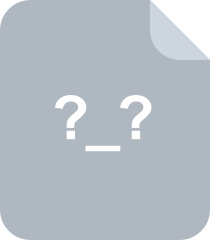
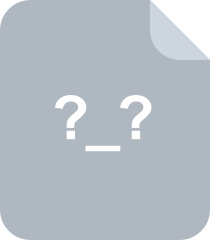
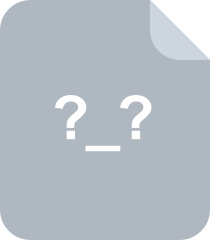
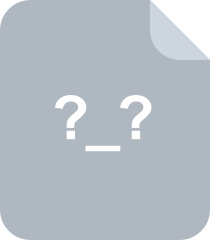
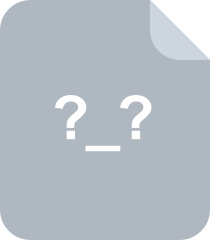
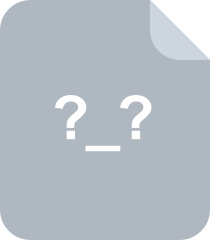
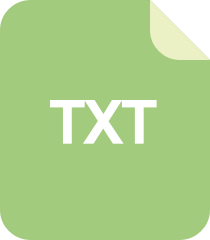
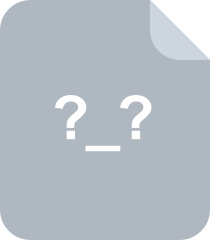
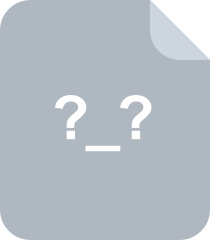
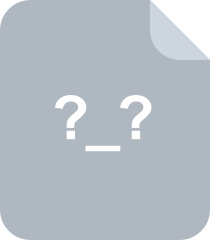
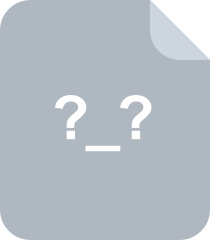
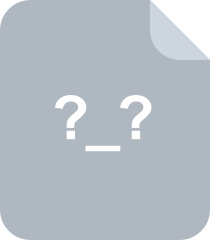

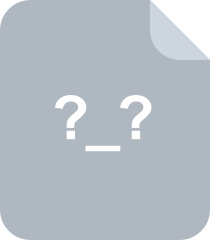
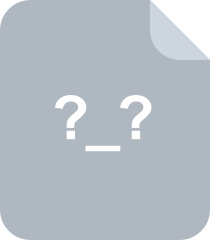
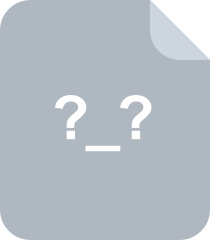
共 57 条
- 1
资源评论
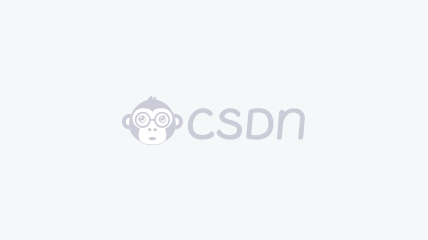
- baidu_198505232023-10-17感谢资源主分享的资源解决了我当下的问题,非常有用的资源。

挣扎的蓝藻
- 粉丝: 13w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

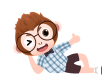
最新资源
- 基于python实现的高考志愿填报参考系统源码+sql数据库(高分毕业设计).zip
- 基于python实现的高考志愿填报参考系统源码+数据库(毕业设计).zip
- 人工智能大赛无人车挑战杯车道线检测python源码.zip
- 基于风控模型的银行客户信用风险评估系统源码+项目说明+数据集(使用jupter notebook).zip
- python基于可变卷积改进的U-Net网络实现对胰腺细胞的精准切割源码(高分项目).zip
- python基于可变卷积改进的U-Net网络实现对胰腺细胞的精准切割源码(高分项目).zip
- 基于javascript实现的蚁群算法(JS代码)
- 基于python实现的随机森林(python代码)
- python读取excel到数据库中,简单的数据库管理脚本
- 7777端口抓包数据集
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


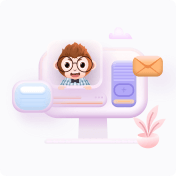
安全验证
文档复制为VIP权益,开通VIP直接复制
