# automl_research
Code repository for AutoML research to support Foreshadow project
---
## Feature Type Inference (Intent Resolution)
When analyzing raw data set feature columns in `Foreshadow`, the type (intent) of the each feature column has to be known a priori to select the appropriate feature transformation downstream.
The goal of this research project is to build an intent resolver that can separate numerical and categorical raw feature columns. More classes can be added in the future.
### Installation
This library was developed on Python 3.6.8 and uses the same package dependencies as `Foreshadow` as of Oct. 17, 2019.
To install additional package dependencies for research-based functionalities, run the following:
```
pip install -r research_requirements.txt
```
### Usage
The functionality of this library is exposed through the `IntentResolver` class API as shown below. The class outputs a prediction of "Numerical", "Categorical" or "Neither" for each raw feature column. Predictions with confidences lower than the `threshold` parameter (default = 0.7) in the `.predict` method are set to "Neither".
```
import pandas as pd
from lib import IntentResolver
# Initialise object
raw = pd.read_csv('path_to_dataset.csv', encoding='latin', low_memory=False)
resolver = IntentResolver(raw)
# Predict intent
# Outputs a pd.Series of predicted intents
resolver.predict()
# OR: Predict intent with confidences at a lower threshold (i.e. less rigorous prediction)
# Outputs a pd.DataFrame of predicted intent and confidences
resolver.predict(threshold=0.6, return_conf=True)
```
### Data Sources
- [Original Meta Data Set (OMDS)](https://github.com/pvn25/ML-Data-Prep-Zoo/tree/master/ML%20Schema%20Inference/Data)
- [360 Raw Data Sets (RDSs)](https://drive.google.com/file/d/1HGmDRBSZg-Olym2envycHPkb3uwVWHJX/view) (Sourced from the [GitHub README.md](https://github.com/pvn25/ML-Data-Prep-Zoo/tree/master/ML%20Schema%20Inference))
### References
1. V. Shah, P. Kumar, K. Yang, and A. Kumar, “Towards semi-automatic mlfeature type inference."
2. N. Hynes, D. Sculley, and M. Terry, “The data linter: Lightweight, auto-mated sanity checking for ml data sets,” in NIPS MLSys Workshop, 2017.
没有合适的资源?快使用搜索试试~ 我知道了~
PyPI 官网下载 | foreshadow-0.3.dev2.tar.gz
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 82 浏览量
2022-01-11
20:31:32
上传
评论
收藏 990KB GZ 举报
温馨提示
资源来自pypi官网。 资源全名:foreshadow-0.3.dev2.tar.gz
资源推荐
资源详情
资源评论
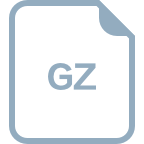
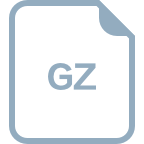
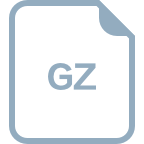
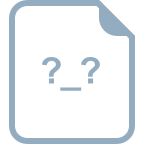
收起资源包目录

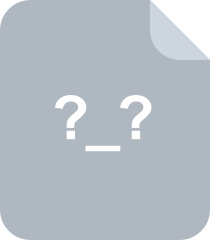
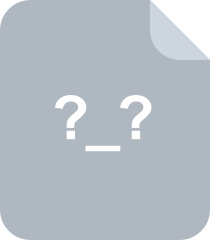
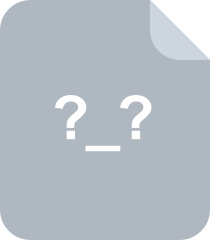
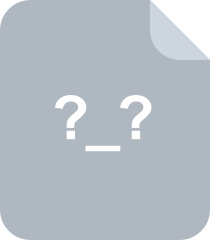
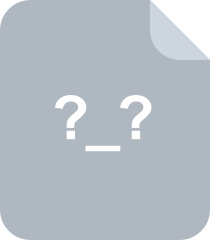
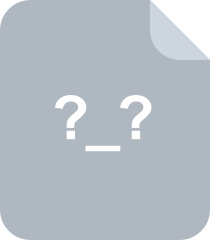
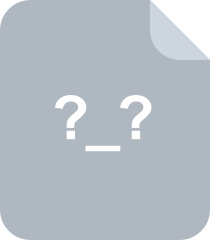
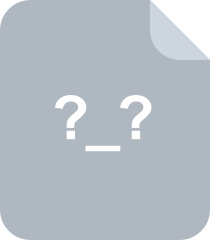
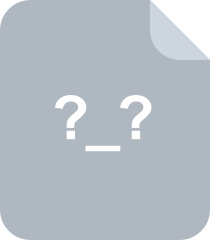
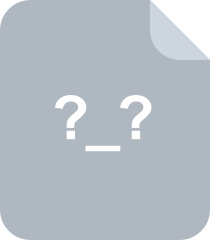
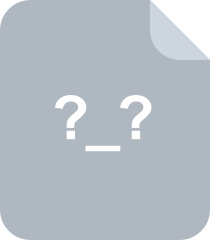
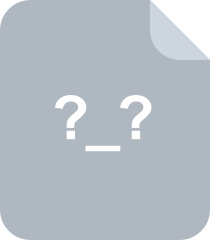
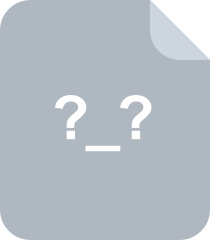
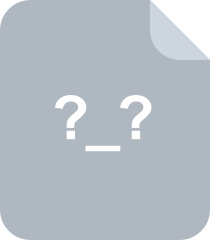
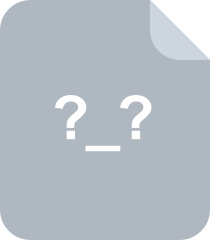
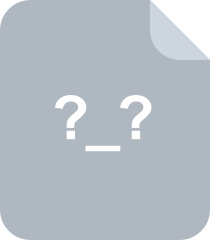
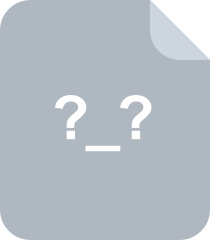
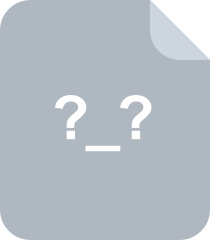
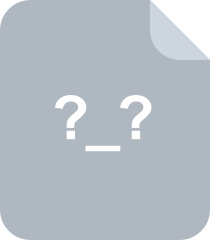
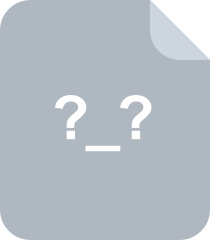
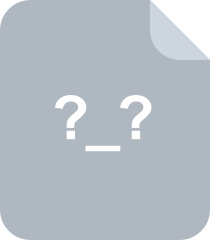
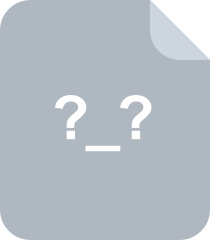
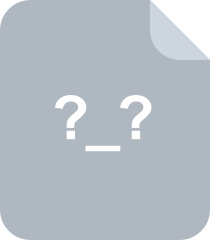
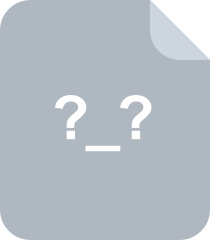
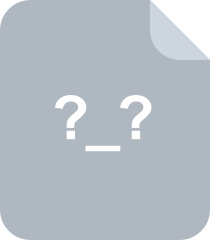
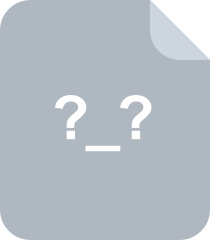
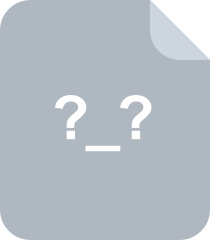
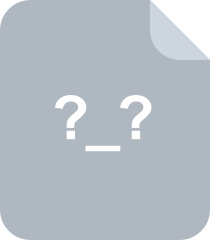
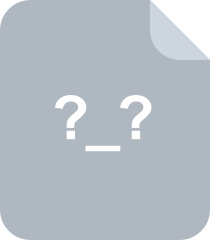
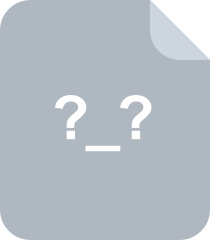
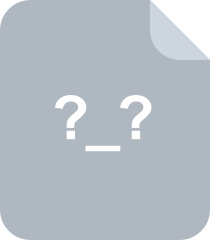
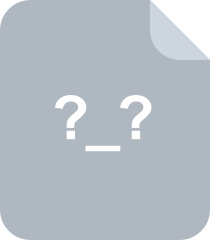
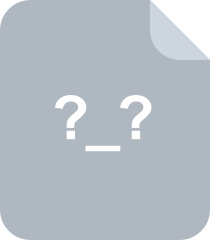
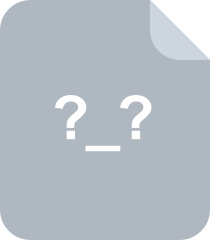
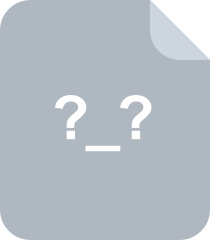
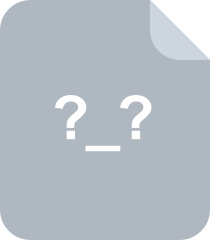
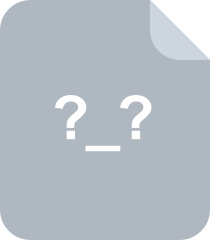
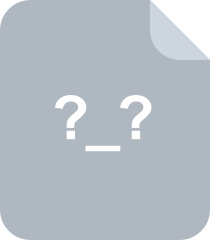
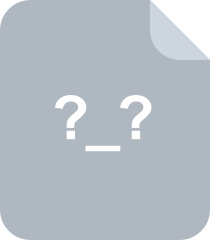
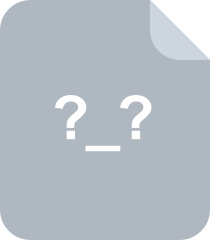
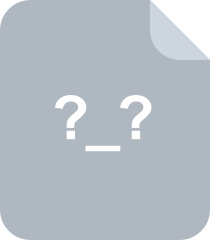
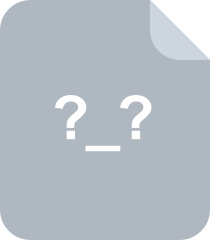
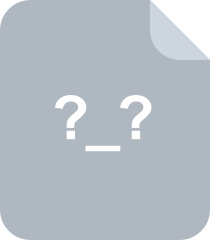
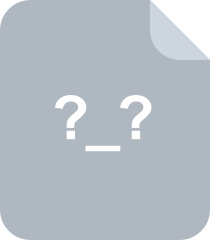
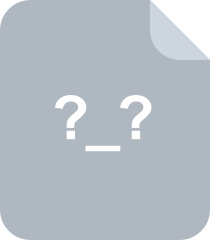
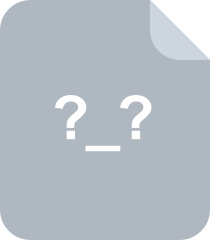
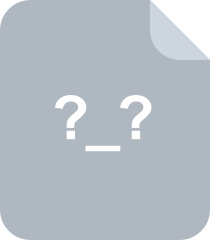
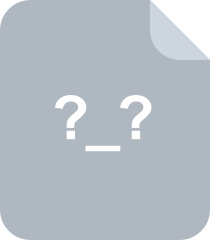
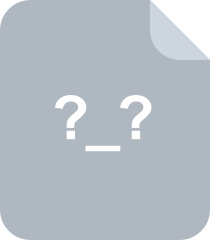
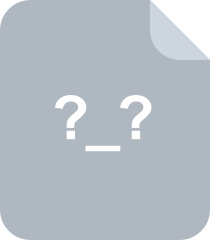
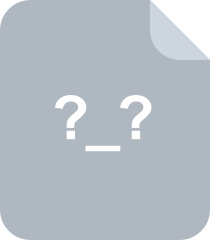
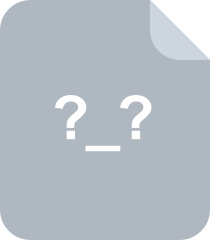
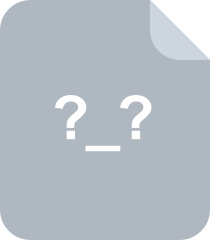
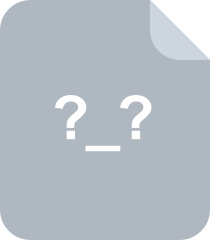
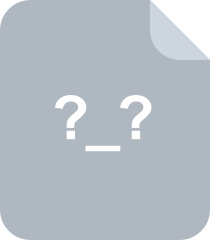
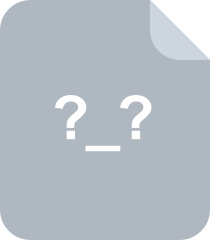
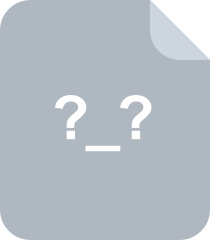
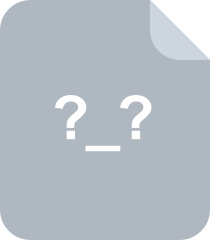
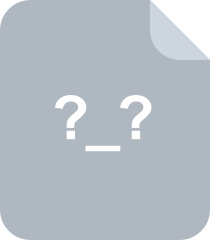
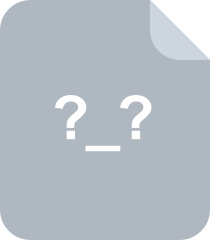
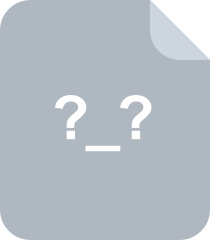
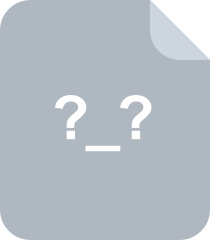
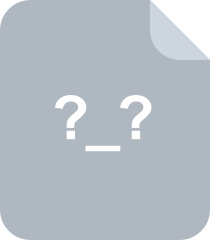
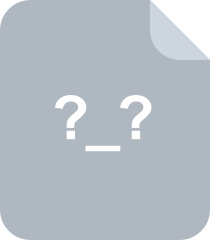
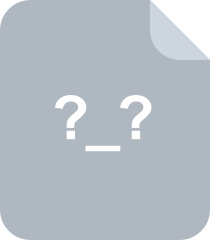
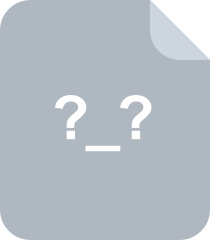
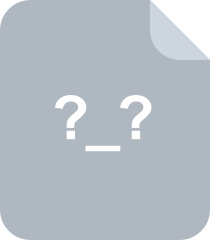
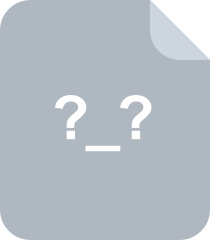
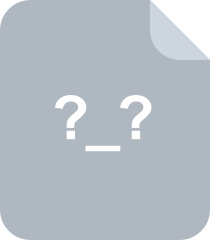
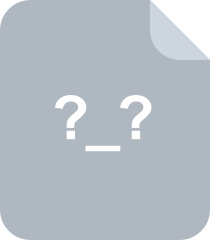
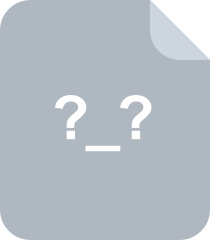
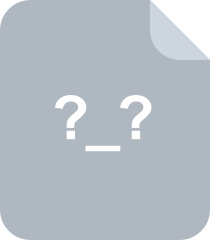
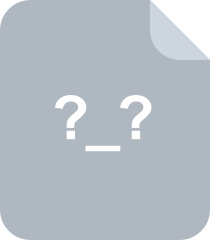
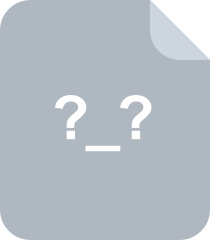
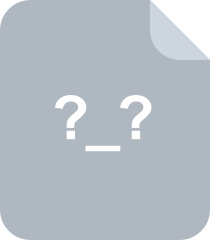
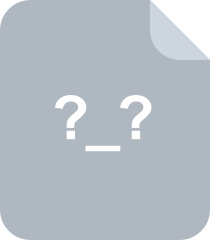
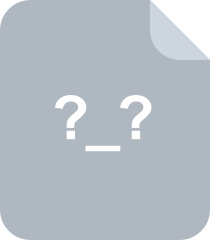
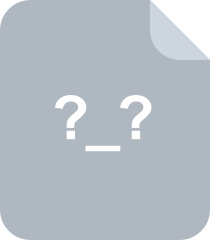
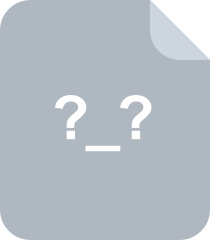
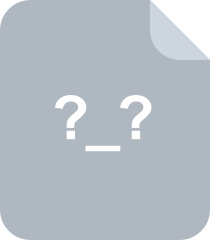
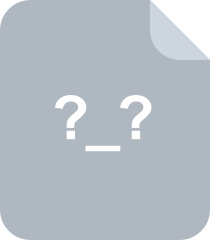
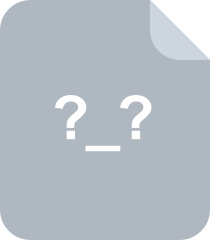
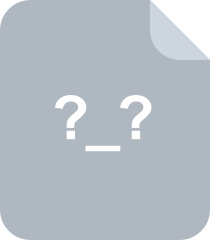
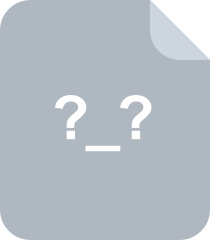
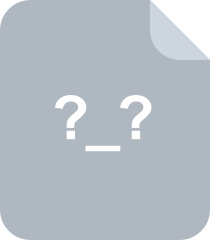
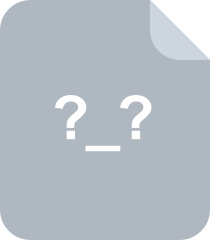
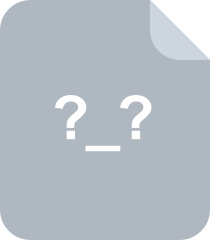
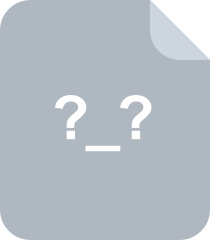
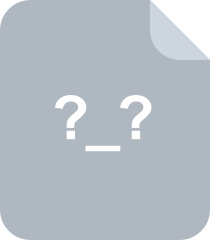
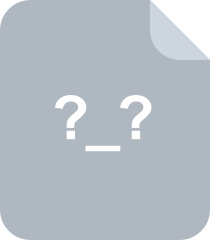
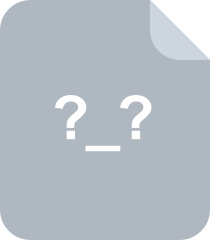
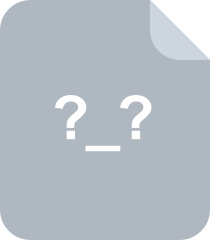
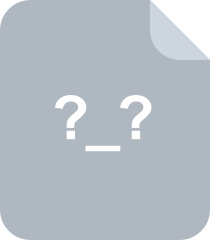
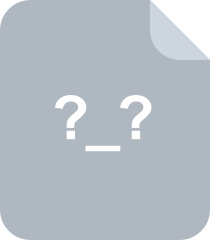
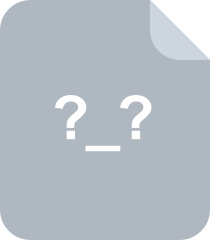
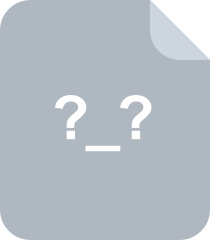
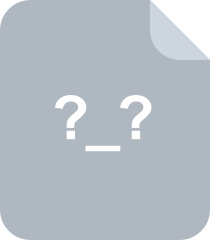
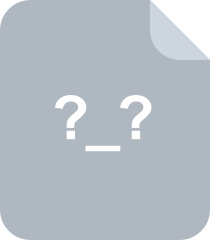
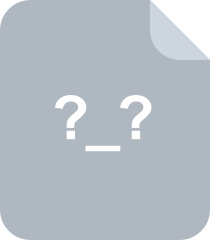
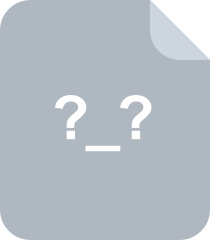
共 192 条
- 1
- 2
资源评论
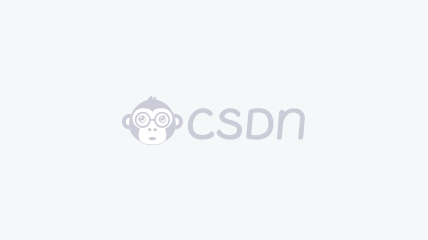

挣扎的蓝藻
- 粉丝: 13w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

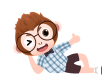
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


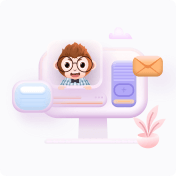
安全验证
文档复制为VIP权益,开通VIP直接复制
