# -*- coding: utf-8 -*-
# PLEASE DO NOT EDIT THIS FILE, IT IS GENERATED AND WILL BE OVERWRITTEN:
# https://github.com/ccxt/ccxt/blob/master/CONTRIBUTING.md#how-to-contribute-code
from ccxt.async_support.base.exchange import Exchange
import json
from ccxt.base.errors import ExchangeError
from ccxt.base.errors import AuthenticationError
from ccxt.base.errors import PermissionDenied
from ccxt.base.errors import AccountSuspended
from ccxt.base.errors import ArgumentsRequired
from ccxt.base.errors import BadRequest
from ccxt.base.errors import BadSymbol
from ccxt.base.errors import InsufficientFunds
from ccxt.base.errors import InvalidOrder
from ccxt.base.errors import OrderNotFound
from ccxt.base.errors import OrderImmediatelyFillable
from ccxt.base.errors import NotSupported
from ccxt.base.errors import DDoSProtection
from ccxt.base.errors import RateLimitExceeded
from ccxt.base.errors import ExchangeNotAvailable
from ccxt.base.errors import OnMaintenance
from ccxt.base.errors import InvalidNonce
from ccxt.base.decimal_to_precision import TRUNCATE
from ccxt.base.precise import Precise
class binance(Exchange):
def describe(self):
return self.deep_extend(super(binance, self).describe(), {
'id': 'binance',
'name': 'Binance',
'countries': ['JP', 'MT'], # Japan, Malta
'rateLimit': 50,
'certified': True,
'pro': True,
# new metainfo interface
'has': {
'cancelAllOrders': True,
'cancelOrder': True,
'CORS': None,
'createOrder': True,
'fetchBalance': True,
'fetchBidsAsks': True,
'fetchClosedOrders': 'emulated',
'fetchCurrencies': True,
'fetchDepositAddress': True,
'fetchDeposits': True,
'fetchFundingFees': True,
'fetchFundingHistory': True,
'fetchFundingRate': True,
'fetchFundingRateHistory': True,
'fetchFundingRates': True,
'fetchIndexOHLCV': True,
'fetchIsolatedPositions': True,
'fetchMarkets': True,
'fetchMarkOHLCV': True,
'fetchMyTrades': True,
'fetchOHLCV': True,
'fetchOpenOrders': True,
'fetchOrder': True,
'fetchOrderBook': True,
'fetchOrders': True,
'fetchPositions': True,
'fetchPremiumIndexOHLCV': False,
'fetchStatus': True,
'fetchTicker': True,
'fetchTickers': True,
'fetchTime': True,
'fetchTrades': True,
'fetchTradingFee': True,
'fetchTradingFees': True,
'fetchTransactions': None,
'fetchTransfers': True,
'fetchWithdrawals': True,
'setLeverage': True,
'setMarginMode': True,
'transfer': True,
'withdraw': True,
},
'timeframes': {
'1m': '1m',
'3m': '3m',
'5m': '5m',
'15m': '15m',
'30m': '30m',
'1h': '1h',
'2h': '2h',
'4h': '4h',
'6h': '6h',
'8h': '8h',
'12h': '12h',
'1d': '1d',
'3d': '3d',
'1w': '1w',
'1M': '1M',
},
'urls': {
'logo': 'https://user-images.githubusercontent.com/1294454/29604020-d5483cdc-87ee-11e7-94c7-d1a8d9169293.jpg',
'test': {
'dapiPublic': 'https://testnet.binancefuture.com/dapi/v1',
'dapiPrivate': 'https://testnet.binancefuture.com/dapi/v1',
'fapiPublic': 'https://testnet.binancefuture.com/fapi/v1',
'fapiPrivate': 'https://testnet.binancefuture.com/fapi/v1',
'fapiPrivateV2': 'https://testnet.binancefuture.com/fapi/v2',
'public': 'https://testnet.binance.vision/api/v3',
'private': 'https://testnet.binance.vision/api/v3',
'v3': 'https://testnet.binance.vision/api/v3',
'v1': 'https://testnet.binance.vision/api/v1',
},
'api': {
'wapi': 'https://api.binance.com/wapi/v3',
'sapi': 'https://api.binance.com/sapi/v1',
'dapiPublic': 'https://dapi.binance.com/dapi/v1',
'dapiPrivate': 'https://dapi.binance.com/dapi/v1',
'dapiPrivateV2': 'https://dapi.binance.com/dapi/v2',
'dapiData': 'https://dapi.binance.com/futures/data',
'fapiPublic': 'https://fapi.binance.com/fapi/v1',
'fapiPrivate': 'https://fapi.binance.com/fapi/v1',
'fapiData': 'https://fapi.binance.com/futures/data',
'fapiPrivateV2': 'https://fapi.binance.com/fapi/v2',
'public': 'https://api.binance.com/api/v3',
'private': 'https://api.binance.com/api/v3',
'v3': 'https://api.binance.com/api/v3',
'v1': 'https://api.binance.com/api/v1',
},
'www': 'https://www.binance.com',
'referral': {
'url': 'https://www.binance.com/en/register?ref=BLEJC98C',
'discount': 0.2,
},
'doc': [
'https://binance-docs.github.io/apidocs/spot/en',
],
'api_management': 'https://www.binance.com/en/usercenter/settings/api-management',
'fees': 'https://www.binance.com/en/fee/schedule',
},
'depth': 1,
'api': {
# the API structure below will need 3-layer apidefs
'sapi': {
'get': {
'accountSnapshot': 1,
'system/status': 1,
# these endpoints require self.apiKey
'margin/asset': 1,
'margin/pair': 1,
'margin/allAssets': 1,
'margin/allPairs': 1,
'margin/priceIndex': 1,
# these endpoints require self.apiKey + self.secret
'asset/assetDividend': 1,
'asset/dribblet': 1,
'asset/transfer': 1,
'asset/assetDetail': 1,
'asset/tradeFee': 1,
'asset/get-funding-asset': 1,
'margin/loan': 1,
'margin/repay': 1,
'margin/account': 1,
'margin/transfer': 1,
'margin/interestHistory': 1,
'margin/forceLiquidationRec': 1,
'margin/order': 1,
'margin/openOrders': 1,
'margin/allOrders': 1,
'margin/myTrades': 1,
'margin/maxBorrowable': 5,
'margin/maxTransferable': 5,
'margin/isolated/transfer': 1,
'margin/isolated/account': 1,
'margin/isolated/pair': 1,
'margin/isolated/allPairs': 1,
'margin/interestRateHistory': 1,
'margin/orderList': 2,
'margin/allOrderList': 10,
'margin/openOrderList': 3,
'fiat/orders': 1,
'fiat/payments': 1,
'futures/tran
没有合适的资源?快使用搜索试试~ 我知道了~
PyPI 官网下载 | ccxt-1.57.57.tar.gz
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 98 浏览量
2022-01-09
19:05:53
上传
评论
收藏 1.69MB GZ 举报
温馨提示
资源来自pypi官网。 资源全名:ccxt-1.57.57.tar.gz
资源推荐
资源详情
资源评论
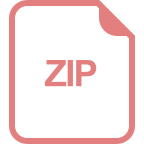
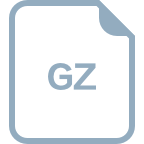
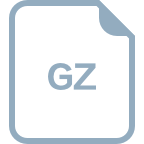
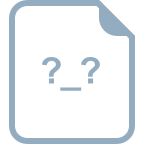
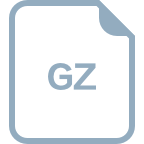
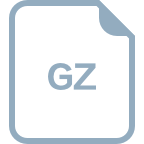
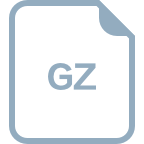
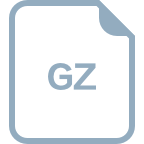
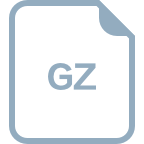
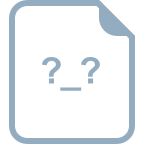
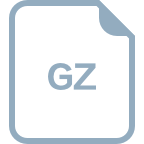
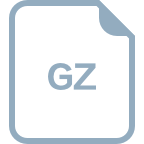
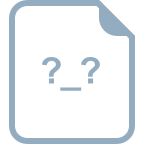
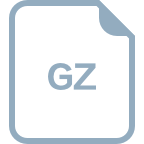
收起资源包目录

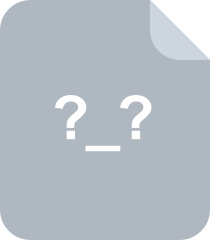
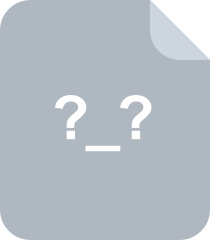
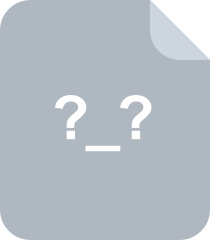
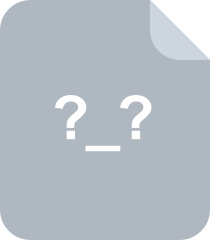
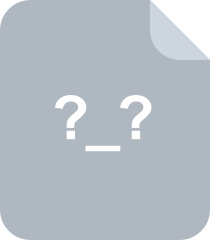
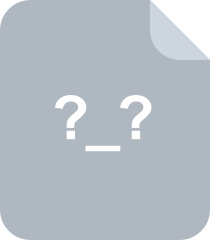
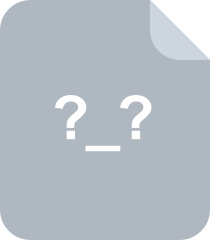
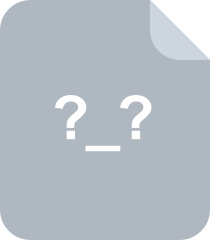
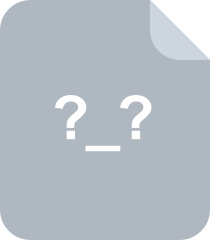
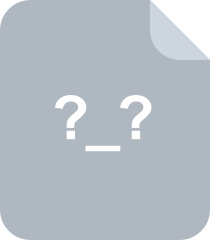
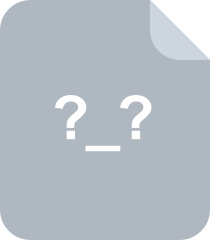
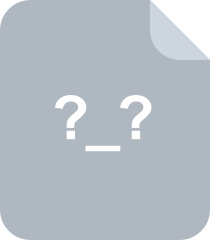
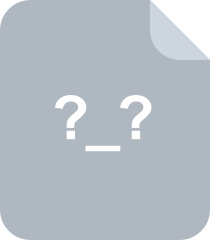
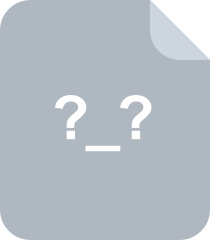
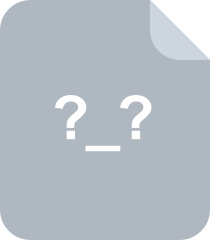
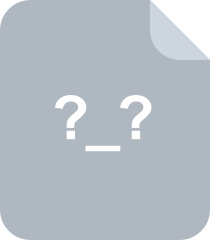
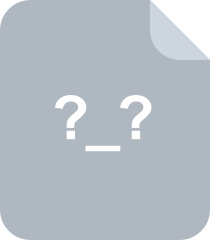
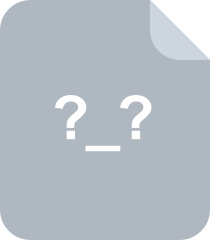
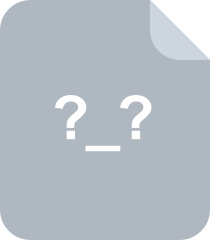
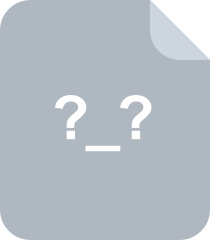
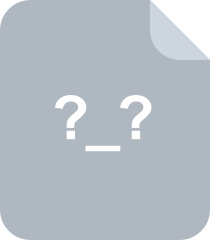
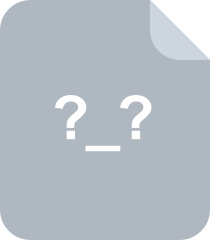
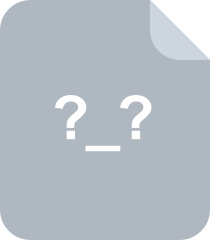
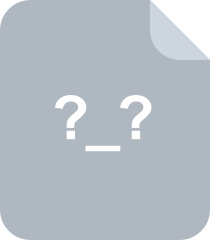
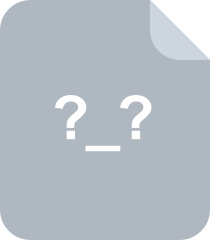
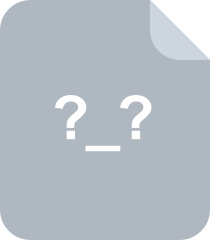
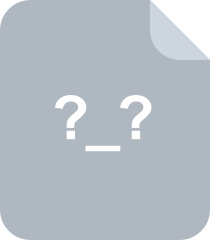
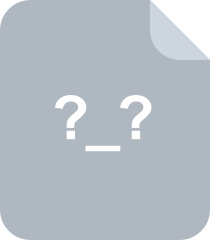
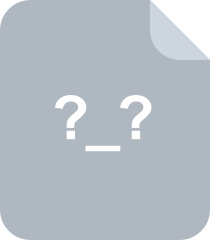
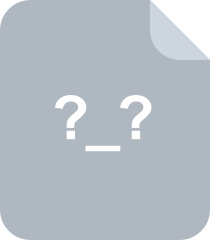
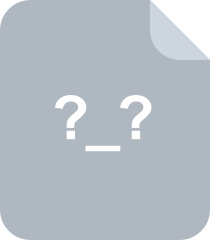
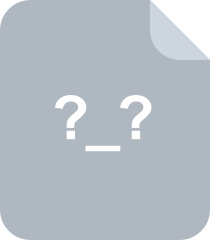
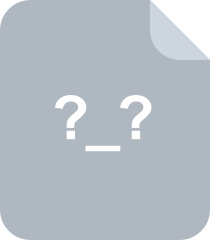
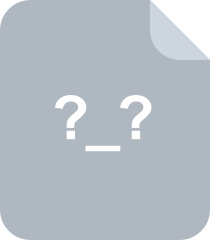
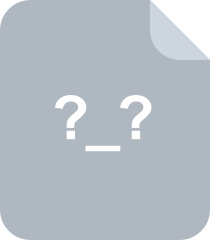
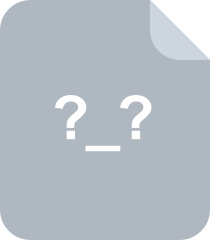
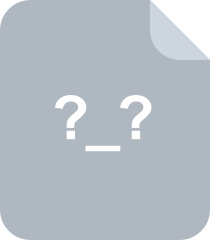
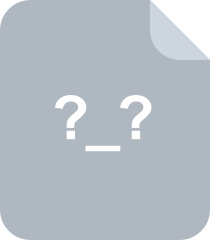
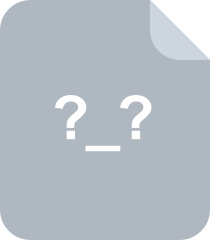
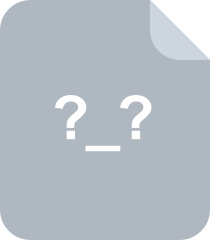
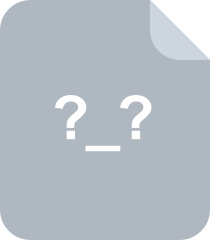
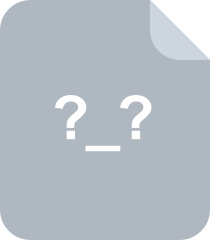
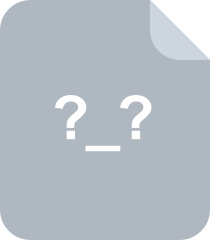
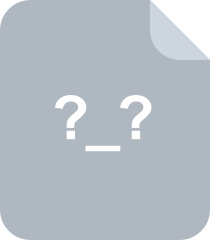
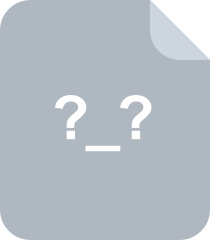
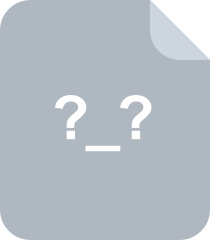
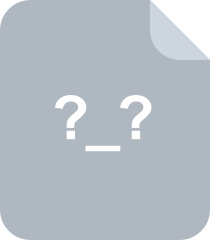
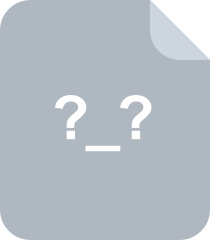
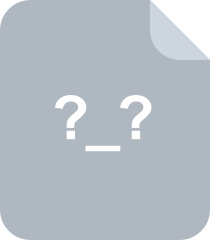
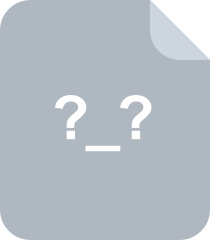
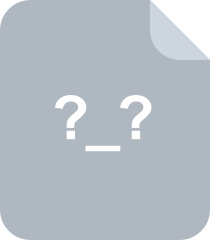
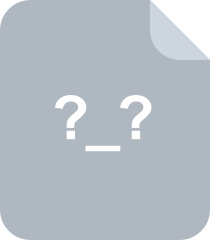
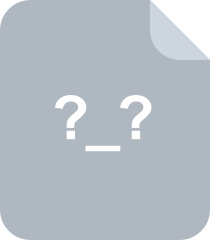
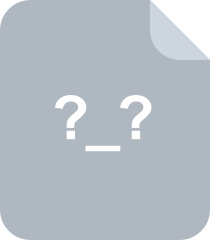
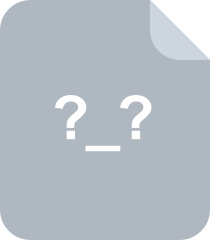
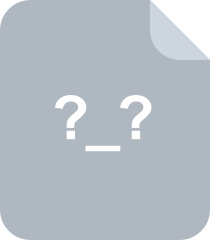
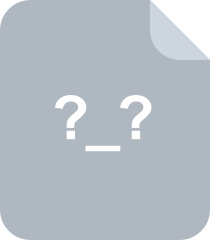
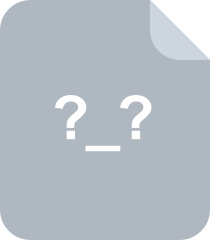
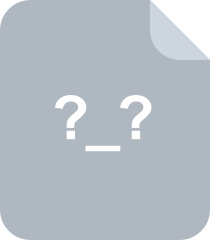
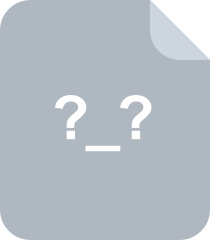
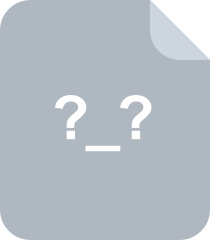
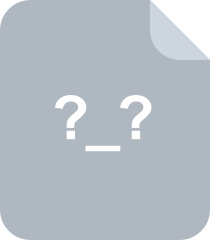
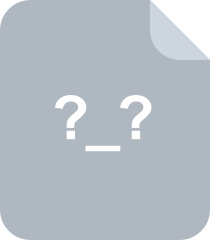
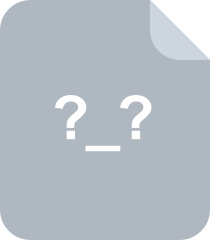
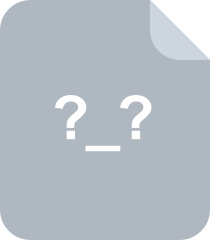
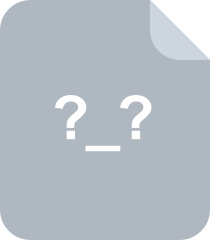
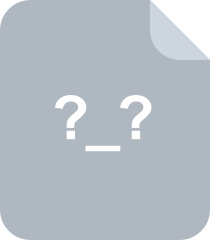
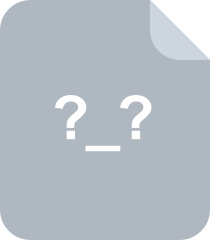
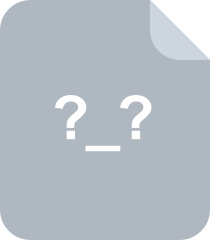
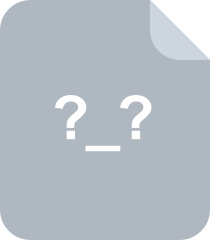
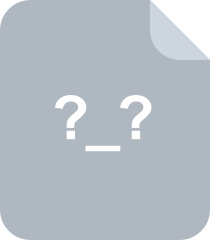
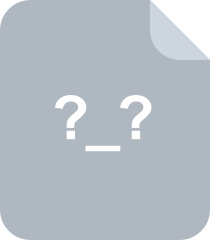
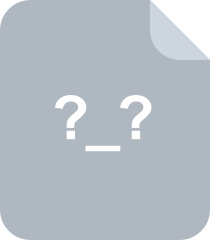
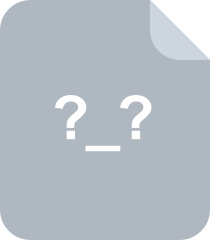
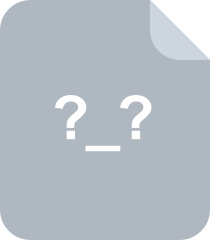
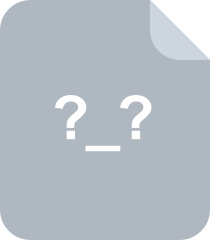
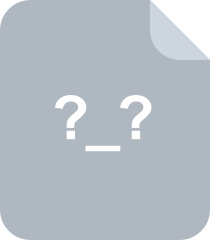
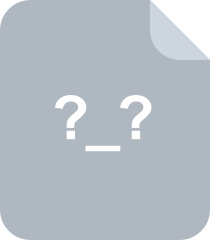
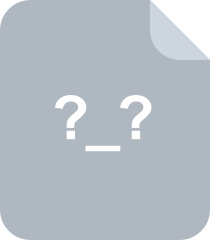
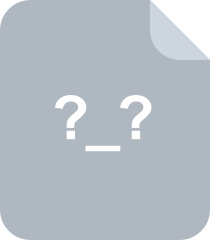
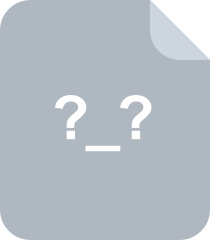
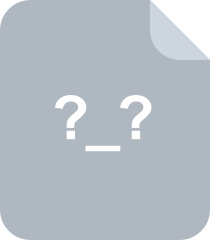
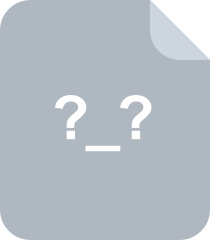
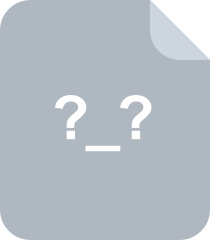
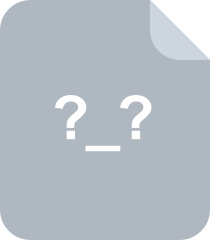
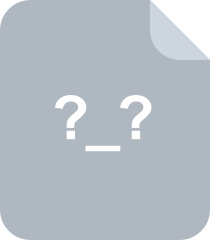
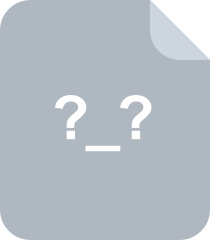
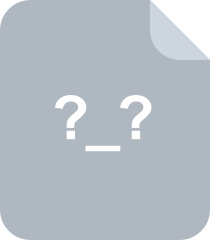
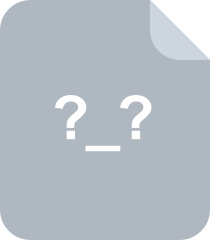
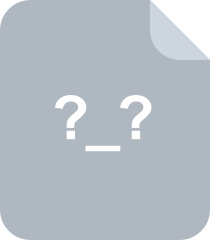
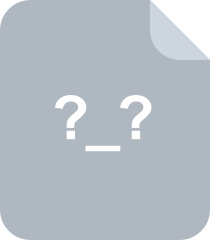
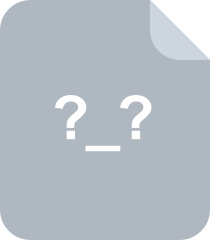
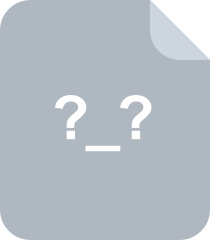
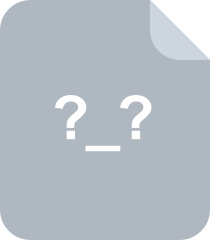
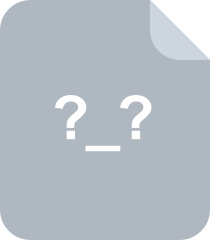
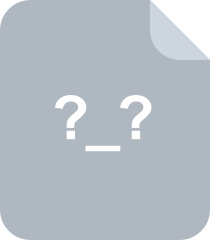
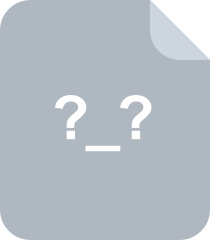
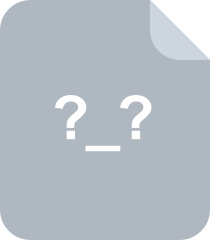
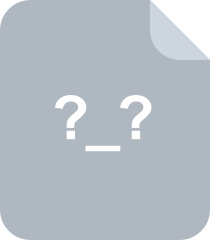
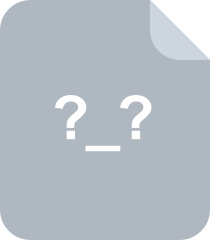
共 273 条
- 1
- 2
- 3
资源评论
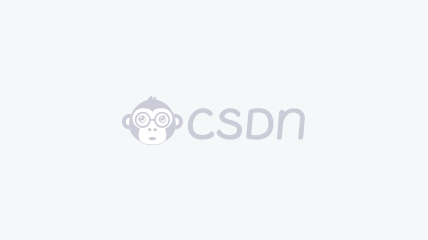

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

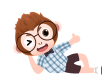
最新资源
- MATLAB界面设计报告.pdf
- 基于PHP实现的学生宿舍管理系统+项目源码+文档说明
- 微信小程序制作方案及流程-微信程序方案.pdf
- 【java毕业设计】家用电器销售网站源码(ssm+jsp+mysql+说明文档+LW).zip
- 【java毕业设计】固定资产管理系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 如何降低电源的待机功耗
- Java基础面试题梳理及其关键知识点解析
- 【java毕业设计】个性化影片推荐系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 课堂作业-基于PHP实现功能简单的学生管理系统+项目源码+文档说明
- 【java毕业设计】个人交友网站源码(ssm+jsp+mysql+说明文档+LW).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


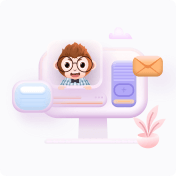
安全验证
文档复制为VIP权益,开通VIP直接复制
