# bimdata-api-client
BIMData API documentation
This Python package is automatically generated by the [OpenAPI Generator](https://openapi-generator.tech) project:
- API version: v1
- Package version: 1.0.0
- Build package: org.openapitools.codegen.languages.PythonClientCodegen
## Requirements.
Python 2.7 and 3.4+
## Installation & Usage
### pip install
If the python package is hosted on Github, you can install directly from Github
```sh
pip install git+https://github.com/GIT_USER_ID/GIT_REPO_ID.git
```
(you may need to run `pip` with root permission: `sudo pip install git+https://github.com/GIT_USER_ID/GIT_REPO_ID.git`)
Then import the package:
```python
import bimdata_api_client
```
### Setuptools
Install via [Setuptools](http://pypi.python.org/pypi/setuptools).
```sh
python setup.py install --user
```
(or `sudo python setup.py install` to install the package for all users)
Then import the package:
```python
import bimdata_api_client
```
## Getting Started
Please follow the [installation procedure](#installation--usage) and then run the following:
```python
from __future__ import print_function
import time
import bimdata_api_client
from bimdata_api_client.rest import ApiException
from pprint import pprint
# Configure API key authorization: Bearer
configuration = bimdata_api_client.Configuration()
configuration.api_key['access_token'] = 'YOUR_API_KEY'
# Uncomment below to setup prefix (e.g. Bearer) for API key, if needed
# configuration.api_key_prefix['access_token'] = 'Bearer'
# create an instance of the API class
api_instance = bimdata_api_client.CheckplanApi(bimdata_api_client.ApiClient(configuration))
cloud_pk = 'cloud_pk_example' # str |
ifc_pk = 'ifc_pk_example' # str |
project_pk = 'project_pk_example' # str |
ifc_checker = bimdata_api_client.IfcChecker() # IfcChecker |
try:
api_response = api_instance.create_checker(cloud_pk, ifc_pk, project_pk, ifc_checker)
pprint(api_response)
except ApiException as e:
print("Exception when calling CheckplanApi->create_checker: %s\n" % e)
```
## Documentation for API Endpoints
All URIs are relative to *https://api-staging.bimdata.io/*
Class | Method | HTTP request | Description
------------ | ------------- | ------------- | -------------
*CheckplanApi* | [**create_checker**](docs/CheckplanApi.md#create_checker) | **POST** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker |
*CheckplanApi* | [**create_checker_result**](docs/CheckplanApi.md#create_checker_result) | **POST** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result |
*CheckplanApi* | [**create_checkplan**](docs/CheckplanApi.md#create_checkplan) | **POST** /cloud/{cloud_pk}/project/{project_pk}/checkplan |
*CheckplanApi* | [**create_rule**](docs/CheckplanApi.md#create_rule) | **POST** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule |
*CheckplanApi* | [**create_rule_component**](docs/CheckplanApi.md#create_rule_component) | **POST** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{rule_pk}/rulecomponent |
*CheckplanApi* | [**create_ruleset**](docs/CheckplanApi.md#create_ruleset) | **POST** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset |
*CheckplanApi* | [**delete_checker**](docs/CheckplanApi.md#delete_checker) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{id} |
*CheckplanApi* | [**delete_checker_result**](docs/CheckplanApi.md#delete_checker_result) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result/{id} |
*CheckplanApi* | [**delete_checkplan**](docs/CheckplanApi.md#delete_checkplan) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{id} |
*CheckplanApi* | [**delete_rule**](docs/CheckplanApi.md#delete_rule) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{id} |
*CheckplanApi* | [**delete_rule_component**](docs/CheckplanApi.md#delete_rule_component) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{rule_pk}/rulecomponent/{id} |
*CheckplanApi* | [**delete_ruleset**](docs/CheckplanApi.md#delete_ruleset) | **DELETE** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{id} |
*CheckplanApi* | [**full_update_checker**](docs/CheckplanApi.md#full_update_checker) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{id} |
*CheckplanApi* | [**full_update_checker_result**](docs/CheckplanApi.md#full_update_checker_result) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result/{id} |
*CheckplanApi* | [**full_update_checkplan**](docs/CheckplanApi.md#full_update_checkplan) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{id} |
*CheckplanApi* | [**full_update_rule**](docs/CheckplanApi.md#full_update_rule) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{id} |
*CheckplanApi* | [**full_update_rule_component**](docs/CheckplanApi.md#full_update_rule_component) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{rule_pk}/rulecomponent/{id} |
*CheckplanApi* | [**full_update_ruleset**](docs/CheckplanApi.md#full_update_ruleset) | **PUT** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{id} |
*CheckplanApi* | [**get_checker**](docs/CheckplanApi.md#get_checker) | **GET** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{id} |
*CheckplanApi* | [**get_checker_result**](docs/CheckplanApi.md#get_checker_result) | **GET** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result/{id} |
*CheckplanApi* | [**get_checker_results**](docs/CheckplanApi.md#get_checker_results) | **GET** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result |
*CheckplanApi* | [**get_checkers**](docs/CheckplanApi.md#get_checkers) | **GET** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker |
*CheckplanApi* | [**get_checkplan**](docs/CheckplanApi.md#get_checkplan) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{id} |
*CheckplanApi* | [**get_checkplans**](docs/CheckplanApi.md#get_checkplans) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan |
*CheckplanApi* | [**get_rule**](docs/CheckplanApi.md#get_rule) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{id} |
*CheckplanApi* | [**get_rule_component**](docs/CheckplanApi.md#get_rule_component) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{rule_pk}/rulecomponent/{id} |
*CheckplanApi* | [**get_rule_components**](docs/CheckplanApi.md#get_rule_components) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule/{rule_pk}/rulecomponent |
*CheckplanApi* | [**get_rules**](docs/CheckplanApi.md#get_rules) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{ruleset_pk}/rule |
*CheckplanApi* | [**get_ruleset**](docs/CheckplanApi.md#get_ruleset) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset/{id} |
*CheckplanApi* | [**get_rulesets**](docs/CheckplanApi.md#get_rulesets) | **GET** /cloud/{cloud_pk}/project/{project_pk}/checkplan/{check_plan_pk}/ruleset |
*CheckplanApi* | [**launch_new_check**](docs/CheckplanApi.md#launch_new_check) | **POST** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{id}/launch-check |
*CheckplanApi* | [**update_checker**](docs/CheckplanApi.md#update_checker) | **PATCH** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{id} |
*CheckplanApi* | [**update_checker_result**](docs/CheckplanApi.md#update_checker_result) | **PATCH** /cloud/{cloud_pk}/project/{project_pk}/ifc/{ifc_pk}/checker/{checker_pk}/result/{id} |
*Che
没有合适的资源?快使用搜索试试~ 我知道了~
PyPI 官网下载 | bimdata-api-client-1.2.0.tar.gz
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 148 浏览量
2022-01-09
12:31:05
上传
评论
收藏 67KB GZ 举报
温馨提示
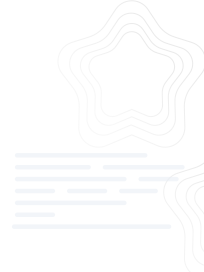
共94个文件
py:86个
txt:4个
pkg-info:2个

资源来自pypi官网。 资源全名:bimdata-api-client-1.2.0.tar.gz
资源推荐
资源详情
资源评论
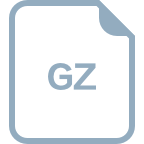
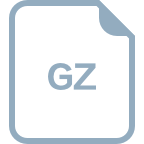
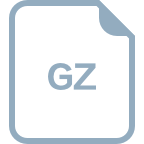
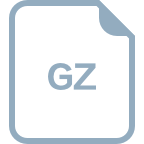
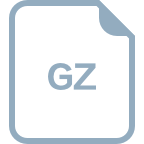
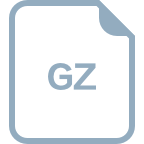
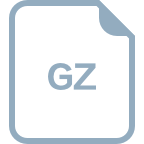
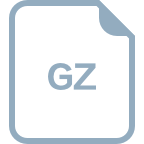
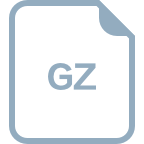
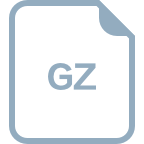
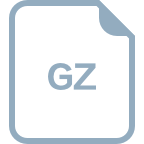
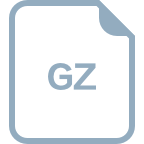
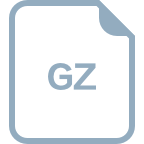
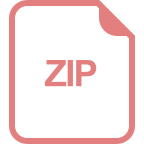
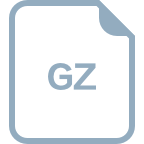
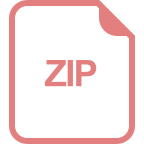
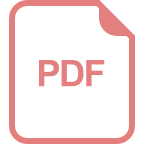
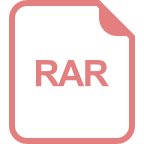
收起资源包目录


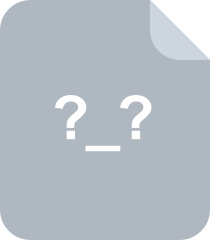


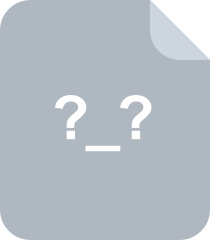
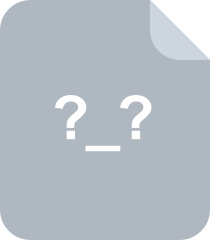
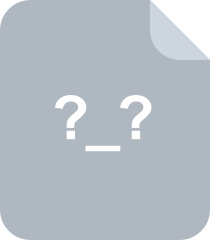
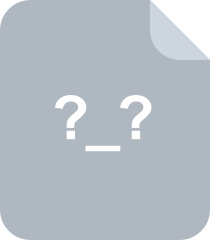
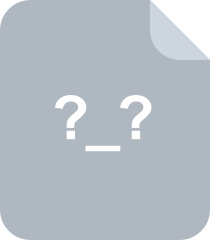
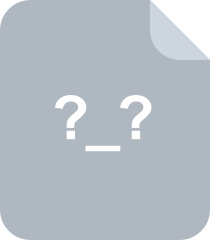
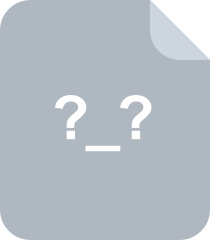
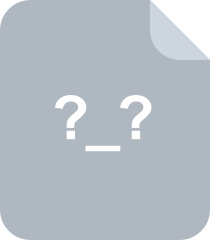
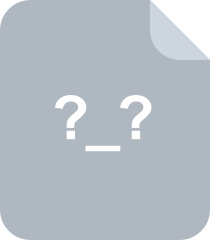
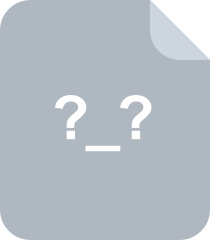
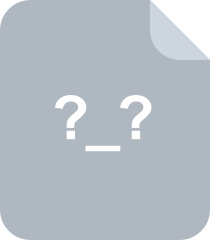
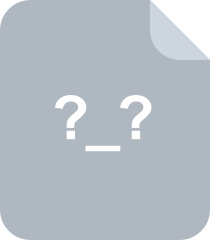
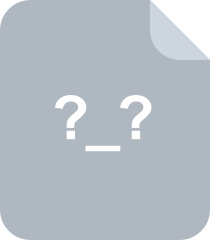
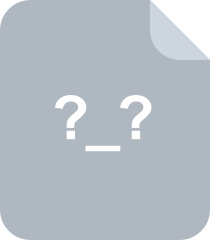
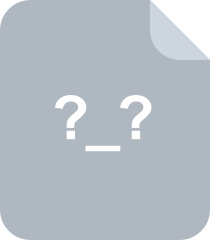
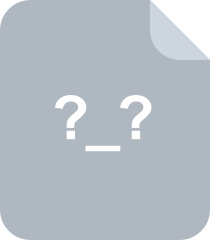
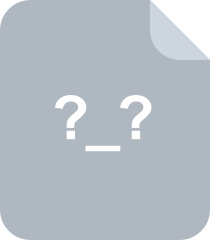
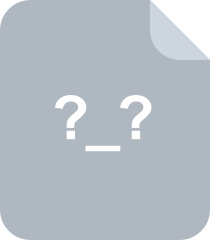
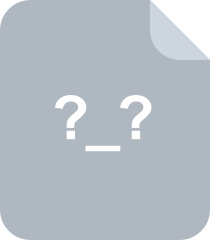
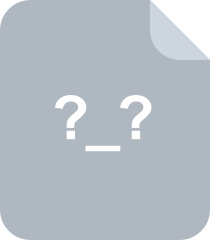
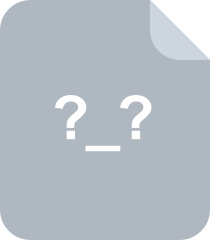
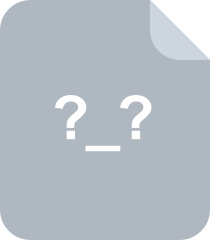
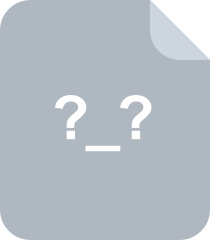
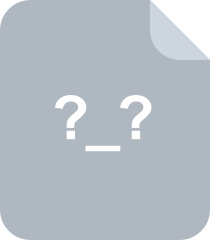
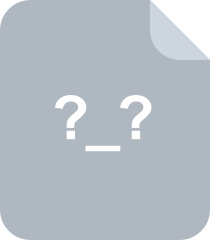
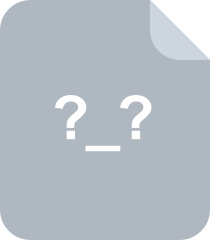
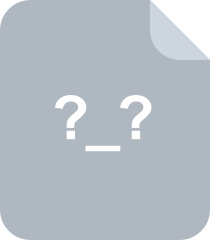
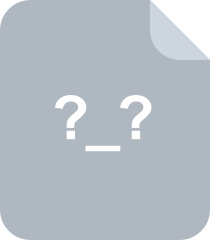
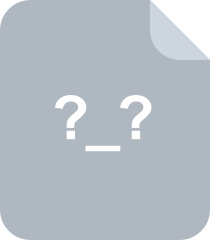
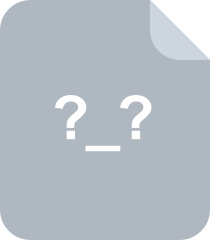
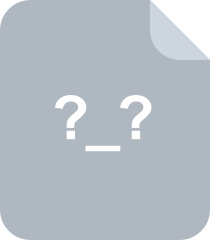
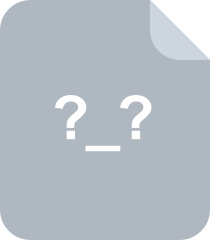
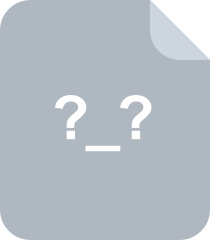
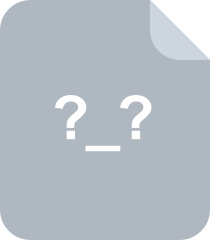
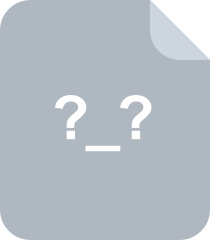
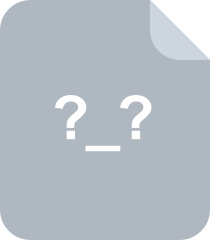

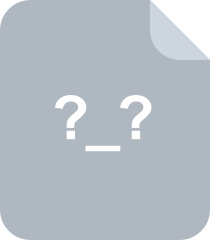
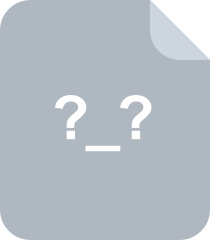
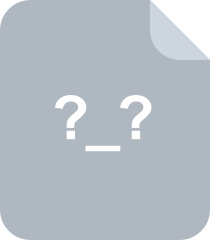
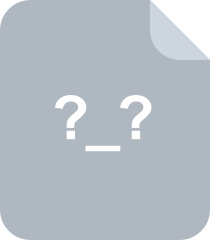
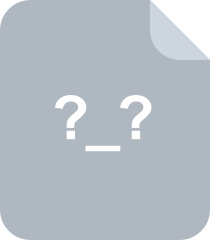
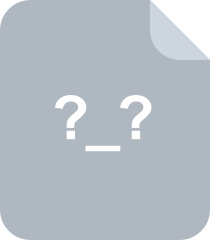
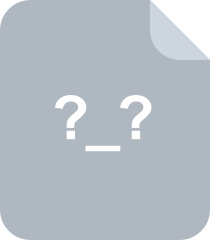
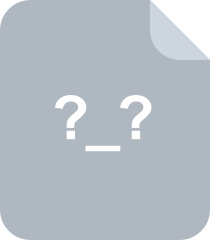
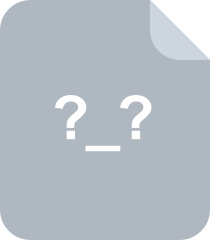

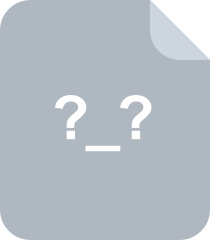
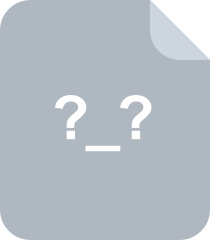
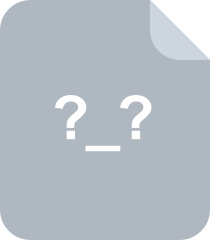
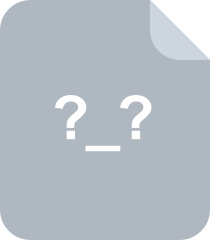
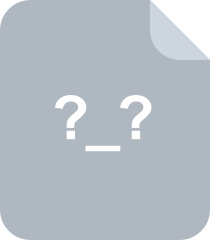
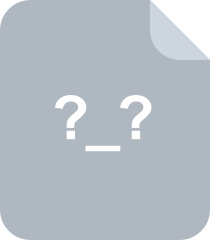
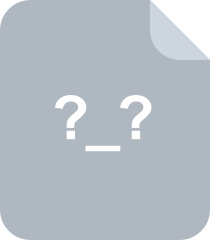
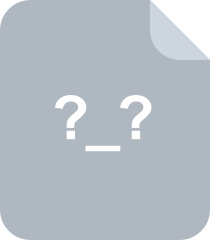
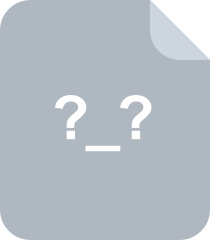
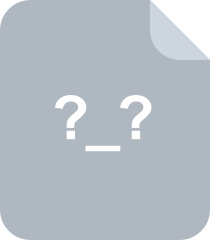
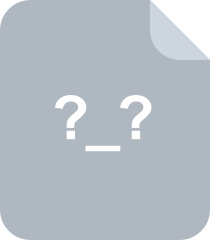
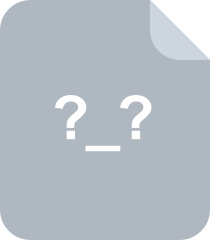
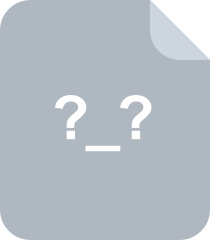
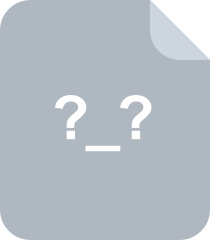
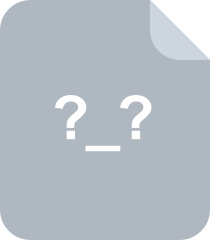
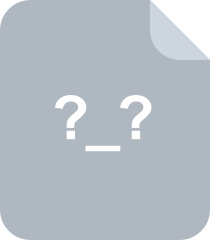
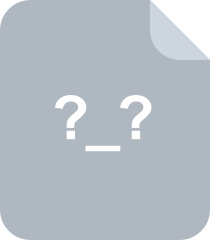
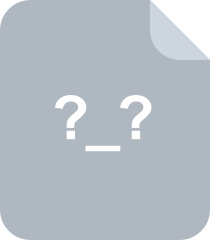
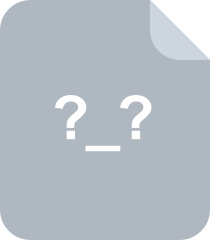
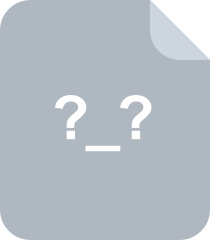
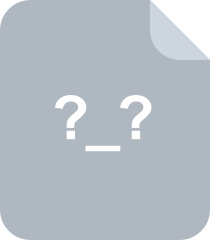
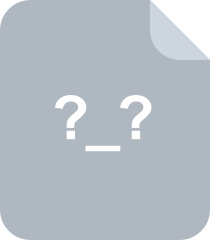
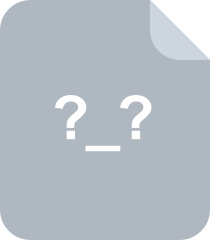
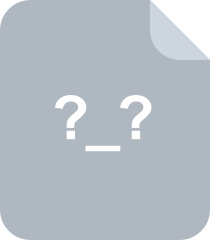
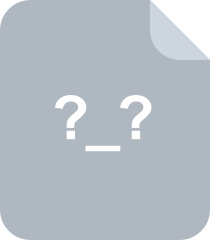
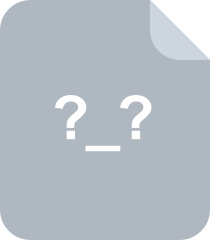
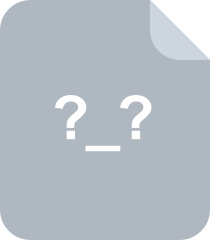
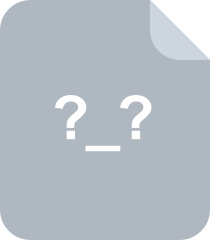
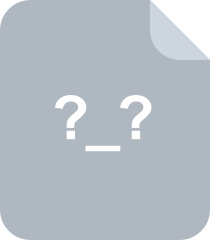
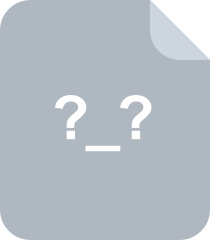
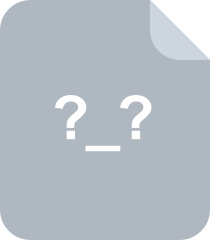
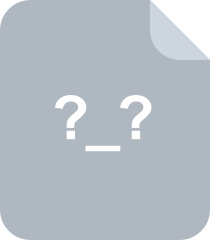
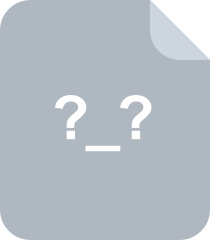
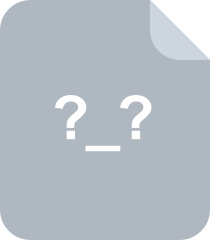
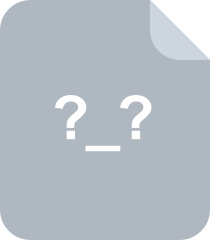
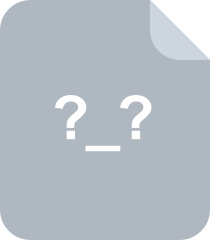
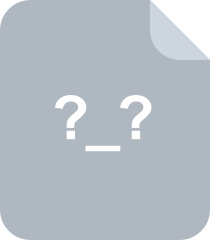
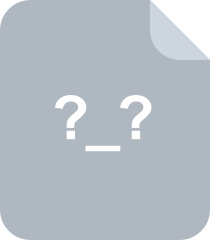
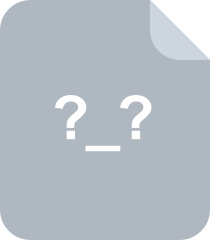
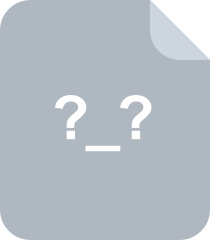

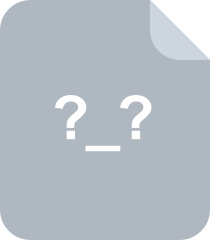
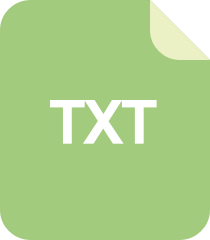
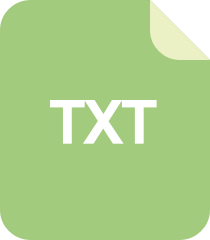
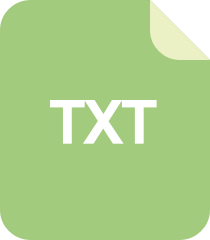
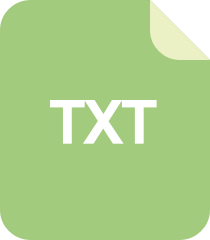
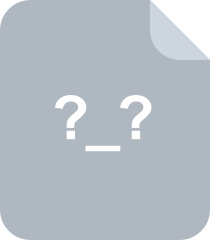
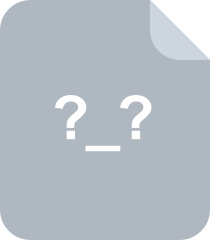
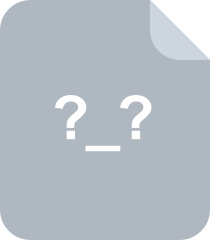
共 94 条
- 1
资源评论
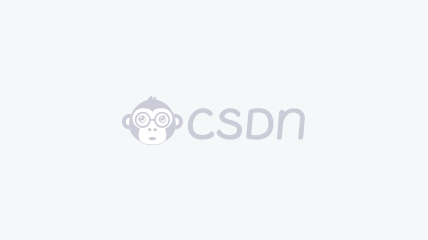

挣扎的蓝藻
- 粉丝: 13w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

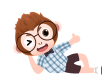
安全验证
文档复制为VIP权益,开通VIP直接复制
