package zqw;
import javax.imageio.ImageIO;
import javax.swing.*;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.UUID;
public class Vido extends JFrame {
int mouseAtX = 0;
int mouseAtY = 0;
Thread jt;
private static File imgs = new File("");
int fps = 5;
private static boolean flag = false;
private static Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss");
public void display(){
this.setBounds(0,0,140,75);
this.setUndecorated(true);
this.setLayout(null);
JButton luz = new JButton("录制");
luz.setBounds(5,5,60,30);
luz.setBackground(Color.LIGHT_GRAY);
JButton zt = new JButton("暂停");
zt.setBounds(75,5,60,30);
zt.setEnabled(false);
zt.setBackground(Color.lightGray);
JButton dk = new JButton("打开");
dk.setBounds(5,40,60,30);
dk.setBackground(Color.LIGHT_GRAY);
JButton gb = new JButton("关闭");
gb.setBounds(75,40,60,30);
gb.setBackground(Color.LIGHT_GRAY);
jt = new Thread(()->{
BufferedImage image = null;
while (true){
try {
image = new Robot().createScreenCapture(new Rectangle(0, 0, screenSize.width, screenSize.height));
if(flag){
if (!Vido.imgs.exists()) {
Vido.imgs.mkdir();
}
ImageIO.write(image, "JPEG", new File(Vido.imgs, new Date().getTime() + ".jpg"));
Thread.sleep(200);
}
} catch (AWTException ex) {
ex.printStackTrace();
} catch (InterruptedException ex) {
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
}
});
jt.start();
luz.addActionListener((e)->{
if(luz.getText().equals("录制")){
Vido.imgs = new File(UUID.randomUUID().toString().replace("-",""));
flag = true;
luz.setText("终止");
zt.setEnabled(true);
zt.setBackground(Color.LIGHT_GRAY);
}else{
flag = false;
luz.setText("录制");
zt.setText("暂停");
zt.setEnabled(false);
zt.setBackground(Color.lightGray);
File imgs1 = new File(Vido.imgs.getPath());
File[] imgs = imgs1.listFiles();
new Thread(()->{
PicToAviUtil.convertPicToAvi(imgs, sdf.format(new Date())+".avi", fps);
deleteAll(imgs1);
try {
Runtime.getRuntime().exec("explorer.exe /c," + new File("").getCanonicalPath());
} catch (IOException ex) {
ex.printStackTrace();
}
}).start();
}
});
zt.addActionListener((e)->{
if(!luz.getText().equals("录制")){
if(zt.getText().equals("暂停")){
zt.setText("继续");
flag = false;
}else{
zt.setText("暂停");
flag = true;
}
}
});
dk.addActionListener((e)->{
try {
Runtime.getRuntime().exec("explorer.exe /c," + new File("").getCanonicalPath());
} catch (IOException ex) {
ex.printStackTrace();
}
});
gb.addActionListener((e)->{
flag = false;
deleteAll(imgs);
System.exit(0);
});
addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e){
mouseAtX = e.getPoint().x;
mouseAtY = e.getPoint().y;
}
});
addMouseMotionListener(new MouseMotionAdapter(){
public void mouseDragged(MouseEvent e){
setLocation((e.getXOnScreen()-mouseAtX),(e.getYOnScreen()-mouseAtY));//设置拖拽后,窗口的位置
}
});
this.add(luz);
this.add(zt);
this.add(gb);
this.add(dk);
this.setVisible(true);
}
private static boolean deleteAll(File f){
if(f.exists()){
if(f.isDirectory()){
File[] files = f.listFiles();
for (int i = 0; i < files.length; i++) {
boolean b = deleteAll(files[i]);
if(!b){
return b;
}
}
}
f.delete();
}else{
return false;
}
f.delete();
return true;
}
public static void main(String[] args) {
new Vido().display();
}
}

张青伟
- 粉丝: 0
- 资源: 3
最新资源
- Matlab版本2023b的Embedded Coder Support Package for ARM Cortex-M Processors支持包免费分享,1.8G压缩包分成3个(2/3)
- ghostscript-10.0.0
- 医疗保障信息平台定点医药机构接口规范
- Python编程基础入门到高级开发技巧指南
- 手机充电头外观尺寸检测机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- JSP EIMS系统-OA子系统的设计与开发(源代码+LW).zip
- (JSP)JTBC_CMS_2.0.0.8.zip
- linux java jdk8
- Windows系统上Tomcat的安装与配置详解
- Linux-Shell基础命令语言
- 服装图像数据集,衣服图像数据,包含服装属性
- Matlab版本2023b的Embedded Coder Support Package for ARM Cortex-M Processors支持包免费分享,1.8G压缩包分成3个(3/3)
- glove11111wwee.pdf
- ECharts象形柱图-圣诞愿望清单和山峰高度-4.zip
- ECharts象形柱图-人体含水量-2.zip
- ECharts象形柱图-驯鹿的速度-6.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


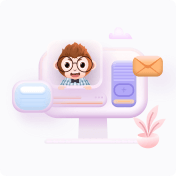