/*
* Created by JFormDesigner on Thu May 14 18:04:12 CST 2020
*/
package FlowerStore.Forms;
import java.beans.*;
import javax.imageio.ImageIO;
import javax.swing.table.*;
import FlowerStore.Entity.Customer;
import FlowerStore.Entity.Flower;
import FlowerStore.Entity.Orders;
import FlowerStore.Entity.ShopList;
import FlowerStore.Factory.FactoryDAO;
import FlowerStore.Factory.FactoryService;
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.regex.Pattern;
import javax.swing.*;
import javax.swing.UIManager;
/**
* @author Ruvik
*/
public class Customer_Home extends JFrame {
List<Flower> list=new ArrayList<>();//存储所有花的列表
DefaultTableModel tableModel=new DefaultTableModel();//查询表格的数据源
private String name;//顾客的名字
private Customer customer=new Customer();
private int CustomerID;//顾客的ID
private String head[]=new String[] {"名字", "价格","数量", "颜色", "有货商店"};//花的表头
private String ListHead[]=new String[] {"名字", "单价","数量", "总价"};//购物车的表头
private String OrderList[]=new String[] {"名字", "单价","数量", "总价","日期"};//订单的表头
// JFormDesigner - Variables declaration - DO NOT MODIFY //GEN-BEGIN:variables
private JTabbedPane tabbedPane1;
private JPanel Check;
private JScrollPane scrollPane1;
private JTable FlowerList;
private JLabel ChooseTitle;
private JLabel Namelabel;
private JLabel Pricelabel;
private JTextField price1;
private JTextField price2;
private JLabel label6;
private JLabel numlabel;
private JTextField num1;
private JTextField num2;
private JLabel label8;
private JLabel label18;
private JLabel label19;
private JComboBox comboBox_name;
private JComboBox comboBox_color;
private JComboBox comboBox_shop;
private JButton buttonchoose;
private JPanel Buy;
private JLabel picturelabel;
private JLabel label9;
private JLabel label10;
private JLabel label11;
private JLabel namelabel;
private JLabel colorlabel;
private JLabel Numlabel;
private JLabel label15;
private JTextField buy_numtext;
private JLabel label16;
private JLabel pricelabel;
private JButton AddToList;
private JButton buyNowButton;
private JComboBox Buy_comboBox;
private JPanel shopping_cart;
private JButton checkout;
private JScrollPane scrollPane2;
private JTable shoplist;
private JLabel tips;
private JPanel Orders;
private JScrollPane scrollPane3;
private JTable listorder;
private JLabel ordertips;
private JPanel Me;
private JLabel Title;
private JLabel username;
private JLabel sex;
private JLabel phone;
private JLabel sign;
private JTextField usernametext;
private JTextField sextext;
private JTextField phonetext;
private JTextField signtext;
private JLabel PasswordTitle;
private JLabel originalP;
private JLabel newP;
private JButton SaveInformation;
private JLabel UserId_Title;
private JLabel UserID;
private JPasswordField original_password;
private JPasswordField new_password;
private JButton SavePass;
private JLabel label2;
private JPopupMenu popupMenu1;
private JMenuItem menuItem_delete;
// JFormDesigner - End of variables declaration //GEN-END:variables
public Customer_Home(){
initComponents();
}
//判断字符串是否为纯数字
public static boolean isInteger(String str) {
Pattern pattern = Pattern.compile("^[-\\+]?[\\d]*$");
return pattern.matcher(str).matches();
}
//region 加载数据
private void thisWindowOpened(WindowEvent e) {
// TODO add your code here
InitData();//个人信息及查询页面加载
InitShopList();//购物车预加载
InitOrdersJPanel();//订单预加载
}
//加载查询界面
private void InitData(){
//region 个人信息获取及自动填充
name=login.name;
customer=FactoryDAO.getICustomer().getCustomerbyName(name);
CustomerID=customer.getCustomer_id();
UserID.setText(Integer.toString(customer.getCustomer_id()));
usernametext.setText(customer.getCustomer_name());
sextext.setText(customer.getCustomer_sex());
phonetext.setText(customer.getCustomer_phone());
signtext.setText(customer.getCustomer_sign());
//endregion
//region 获取全部花的信息
tableModel=new DefaultTableModel(FactoryService.getiCustomerService().CheckAllFlowers(head),head){
//使不可编辑
public boolean isCellEditable(int row, int column)
{
return false;
}
};
FlowerList.setModel(tableModel);//填充Jtable
FlowerList.getSelectionModel().setSelectionMode(ListSelectionModel.SINGLE_SELECTION);//
//清空数据重新加载
comboBox_name.removeAllItems();
comboBox_color.removeAllItems();
comboBox_shop.removeAllItems();
//第一个为空值,默认不选以及可以实现多次选择
comboBox_name.addItem(null);
comboBox_color.addItem(null);
comboBox_shop.addItem(null);
//自动填充下拉菜单
list=FactoryDAO.getIFlowers().CheckAllFlowers();
//填充名字(名字不会出现重复)
for(Flower v:list){
comboBox_name.addItem(v.getFlower_name());
Buy_comboBox.addItem(v.getFlower_name());
}
//填充颜色
List<String> ColorList=FactoryDAO.getIFlowers().checkAllColors();
for(String v:ColorList){
comboBox_color.addItem(v);
}
//填充商店
List<Integer> ShopID=FactoryDAO.getIFlowers().checkAllShops();
for(Integer v:ShopID){
comboBox_shop.addItem(FactoryDAO.getIStore().CheckStoreByID(v).getStore_name());
}
//endregion
}
//加载购物车
private void InitShopList() {
DefaultTableModel tableModelShop=new DefaultTableModel();//表格的数据源
tableModelShop = new DefaultTableModel(FactoryService.getiCustomerService().CheckMyList(ListHead, CustomerID), ListHead) {
//使不可编辑
public boolean isCellEditable(int row, int column) {
return false;
}
};
if (FactoryService.getiCustomerService().CheckMyList(ListHead, CustomerID).length!=0) {
shoplist.setModel(tableModelShop);//填充Jtable
shoplist.getSelectionModel().setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
shoplist.setVisible(true);
checkout.setText("结算");
tips.setText("右键购物车内容可以删除哦~");
} else {
shoplist.setVisible(false);
tips.setVisible(true);
tips.setText("购物车竟然是空的哦~ 再忙,也要记得买点什么犒劳自己~");
String s="买买买!";
checkout.setText(s);
}
}
//加载账单
private void InitOrdersJPanel(){
DefaultTableModel tableModelOrders=new DefaultTableModel();//表格的数据源
tableModelOrders = new DefaultTableModel(FactoryService.getiCustomerService().CheckMyOrder(OrderList,CustomerID), OrderList) {
//使不可编辑
public boolean isCellEditable(int row, int column) {
return false;
}
};
if(FactoryService.getiCustomerService().CheckMyOrder(OrderList,CustomerID).length!=0){
listorder.setModel(tableModelOrders);//填充Jtable
listorder.getSelectionModel().setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
order

王二空间
- 粉丝: 7485
- 资源: 2099
最新资源
- 基于springboot+vue的在线拍卖系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的学生网上请假系统设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的新冠病毒密接者跟踪系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的新闻稿件管理系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的学生选课系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的疫苗发布和接种预约系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的在线商城系统设计与开发-代码(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的疫情打卡健康评测系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的智能物流管理系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的知识管理系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的在线文档管理系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的中小型医院网站(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的“衣依”服装销售平台的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于springboot的房屋租赁系统(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的车辆管理系统设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于springboot+vue的it技术交流和分享平台的设计与实现(Java毕业设计,附源码,部署教程).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


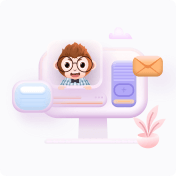