package Home;
import com.toedter.calendar.JDateChooser;
import java.awt.Color;
import java.awt.HeadlessException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Timer;
import java.util.TimerTask;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import net.proteanit.sql.DbUtils;
public class main extends javax.swing.JFrame {
PreparedStatement pst = null;
ResultSet rs = null;
Connection conn = dbConfig.getConn();
public main() {
initComponents();
defaults();
time();
accountsTabls();
}
private void time() {
Calendar cal = new GregorianCalendar();
int day = cal.get(Calendar.DAY_OF_MONTH);
int Month = cal.get(Calendar.MONTH);
int year = cal.get(Calendar.YEAR);
datemi.setText("logged in at: " + day + "/" + Month + "/" + (year));
datemi.setForeground(Color.RED);
int min = cal.get(Calendar.MINUTE);
int sec = cal.get(Calendar.SECOND);
int hour = cal.get(Calendar.HOUR_OF_DAY);
timeMi.setText("time: " + hour + ":" + min + ":" + sec + " sec");
timeMi.setForeground(Color.RED);
}
private void clear() {
txtArea.setText("");
txtContact.setText("");
txtDateIsseud.setDate(new Date());
txtDuedate.setDate(new Date());
txtFname.setText("");
txtId.setText("");
txtInterest.setText("");
txtLname.setText("");
txtTotalRetUrn.setText("");
txtSpecificAmount.setText("");
ComboAmountLend.setSelectedIndex(0);
ComboCoraterol.setSelectedIndex(0);
ComboDuration.setSelectedIndex(0);
ComboState.setSelectedIndex(0);
ComboServedby.setSelectedIndex(0);
txtInterest.setText("");
txtArea1.setText("");
txtContact1.setText("");
txtDateIsseud.setDate(new Date());
txtDuedate1.setDate(new Date());
txtFname1.setText("");
txtId1.setText("");
txtInterest1.setText("");
txtLname1.setText("");
txtTotalRetUrn1.setText("");
txtSpecificAmount1.setText("");
ComboAmountLend1.setSelectedIndex(0);
ComboCoraterol1.setSelectedIndex(0);
ComboDuration1.setSelectedIndex(0);
ComboState1.setSelectedIndex(0);
ComboServedby1.setSelectedIndex(0);
txtInterest1.setText("");
}
private void defaults() {
ComboAmountLend.setSelectedIndex(6);
}
public void pst(){
try {
pst.setString(1, txtId.getText());
pst.setString(2, txtFname.getText());
pst.setString(3, txtLname.getText());
pst.setString(4, txtContact.getText());
pst.setString(5, ComboCoraterol.getSelectedItem().toString());
pst.setString(6, ComboState.getSelectedItem().toString());
pst.setString(7, txtArea.getText());
pst.setString(8, ComboAmountLend.getSelectedItem().toString());
pst.setString(9, ComboDuration.getSelectedItem().toString());
pst.setString(10, txtInterest.getText());
pst.setString(11, ((JTextField) txtDateIsseud.getDateEditor().getUiComponent()).getText());
pst.setString(12, ((JTextField) txtDuedate.getDateEditor().getUiComponent()).getText());
pst.setString(13, ComboServedby.getSelectedItem().toString());
pst.setString(14, txtSpecificAmount.getText());
pst.setString(15, txtTotalRetUrn.getText());
} catch (SQLException e) {
JOptionPane.showMessageDialog(null, e);
}
}
public void clearAcc(){
try {
pst.setString(1, txtId1.getText());
pst.setString(2, txtFname1.getText());
pst.setString(3, txtLname1.getText());
pst.setString(4, txtContact1.getText());
pst.setString(5, ComboCoraterol1.getSelectedItem().toString());
pst.setString(6, ComboState1.getSelectedItem().toString());
pst.setString(7, txtArea1.getText());
pst.setString(8, ComboAmountLend1.getSelectedItem().toString());
pst.setString(9, ComboDuration1.getSelectedItem().toString());
pst.setString(10, txtInterest1.getText());
pst.setString(11, txtIssueTime.getText());
pst.setString(12, ((JTextField) txtDuedate1.getDateEditor().getUiComponent()).getText());
pst.setString(13, ComboServedby1.getSelectedItem().toString());
pst.setString(14, txtSpecificAmount1.getText());
pst.setString(15, txtTotalRetUrn1.getText());
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
}
private void accountsTabls(){
//============================ setup account tables=========================
try {
String sql="select * from withdrawals";
String sql1="select * from deposits";
String sql2="select * from overall";
//========================withdrwal==================
pst=conn.prepareStatement(sql);
rs=pst.executeQuery();
tblWithdraw.setModel(DbUtils.resultSetToTableModel(rs));
int i=0;
for (int j = 0; j < tblWithdraw.getRowCount(); j++) {
i+=Integer.parseInt(tblWithdraw.getValueAt(j, 13).toString());
}
lblwithdraw.setText(""+ i);
//========================================= deposit========================
pst=conn.prepareStatement(sql1);
rs=pst.executeQuery();
tblDeposit.setModel(DbUtils.resultSetToTableModel(rs));
try {
int k=0;
for (int j = 0; j < tblDeposit.getRowCount(); j++) {
k+=Integer.parseInt(tblDeposit.getValueAt(j, 14).toString());
}
lbldeposit.setText(""+ k);
} catch (Exception e) {
JOptionPane.showMessageDialog(null, e);
}
//======================================= members============================
pst=conn.prepareStatement(sql2);
rs=pst.executeQuery();
tblmembersinfo.setModel(DbUtils.resultSetToTableModel(rs));
int i2=0;
for (int j = 0; j < tblmembersinfo.getRowCount(); j++) {
i2+=Integer.parseInt(tblmembersinfo.getValueAt(j, 1).toString());
}
lblmambers.setText(""+ i2);
} catch (SQLException ex) {
JOptionPane.showMessageDialog(null, ex);
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jLabel58 = new javax.swing.JLabel();
jPanel1 = new javax.swing.JPanel();
jPanel2 = new javax.swing.JPanel();
jLabel5 = new javax.swing.JLabel();
pnl_layout = new javax.swing.JPanel();
home = new javax.swing.JPanel();
Action = new javax.swing.JPanel();
btnGeneralInfo = new javax.swing.JButton();
jLabel32 = new javax.swing.JLabel();
jLabel34 = new javax.swin
没有合适的资源?快使用搜索试试~ 我知道了~
Java 财务管理系统源码
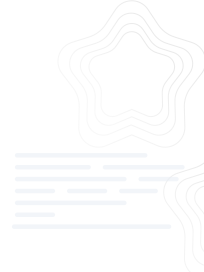
共141个文件
class:52个
png:50个
gif:8个

2 下载量 97 浏览量
2023-09-06
23:12:14
上传
评论 1
收藏 1.43MB RAR 举报
温馨提示
Java 财务管理系统源码 财务管理系统是一个维护银行部门官方记录的java项目。整个系统都在Netbeans IDE 中。该系统有一个SQL Lite数据库作为后端支持。该系统支持该系统所需的所有功能。 关于系统 财务管理系统易于理解和操作。由于这是银行系统一小部分的示例,因此它具有该系统的一些基本特征。这里主要部分是由该系统的管理员控制的。在这里他可以创建一些可以操作系统的用户。管理员还可以删除个人帐户。不仅如此,管理员还可以终止提款方法。管理员可以查看财务公司的统计数据。他们还可以查看个人账户及其简要摘要。这样,它可以帮助他们保持记录最新且更高效。 从用户的角度来看,用户只需在该系统中开设一个账户即可。作为用户,他/她可以存入他们想要存入的金额。他们还可以提取资金。
资源推荐
资源详情
资源评论
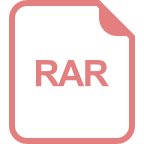
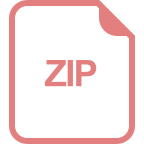
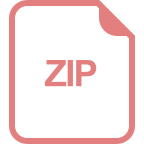
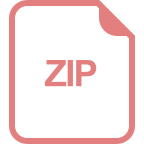
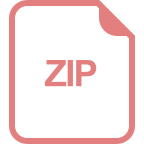
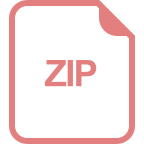
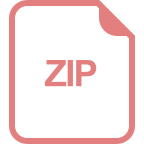
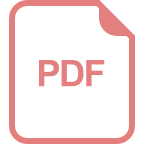
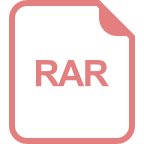
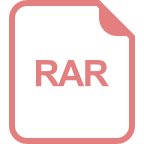
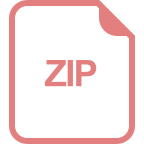
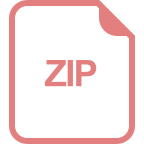
收起资源包目录

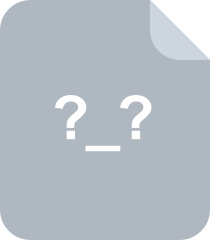
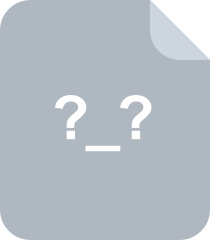
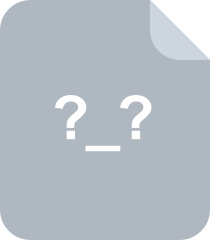
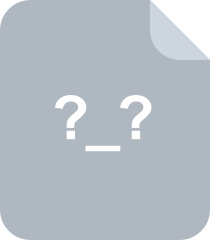
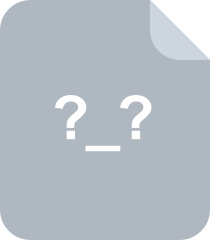
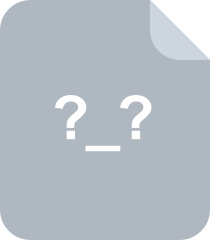
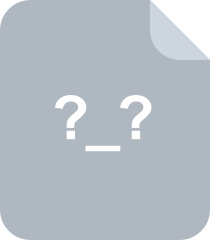
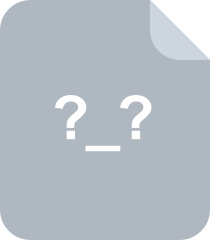
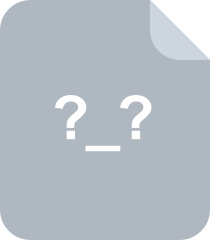
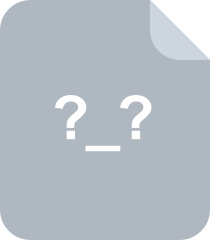
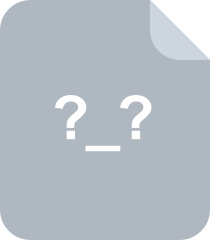
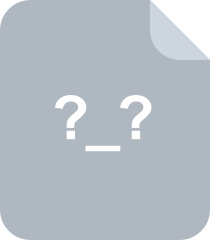
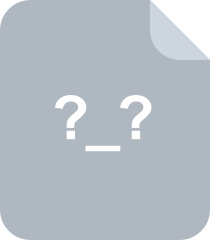
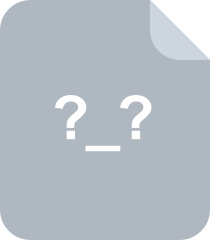
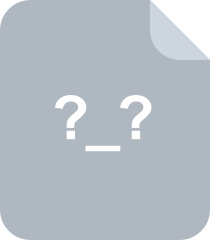
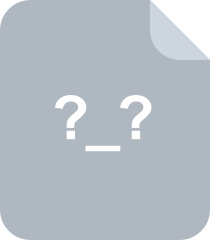
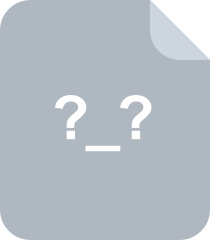
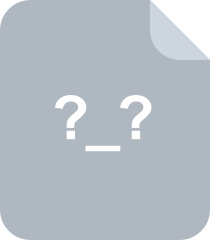
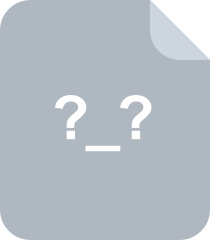
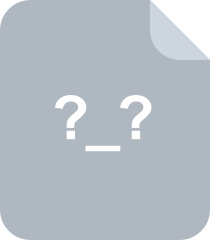
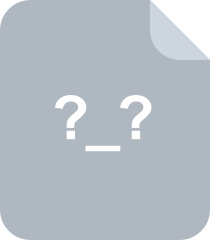
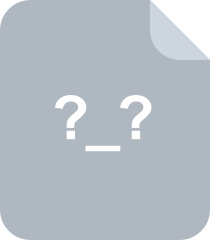
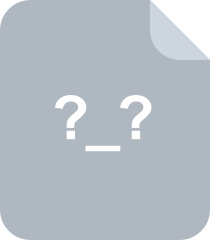
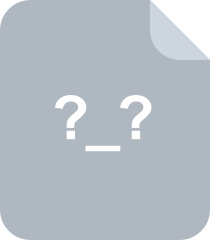
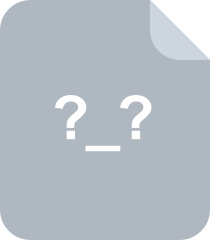
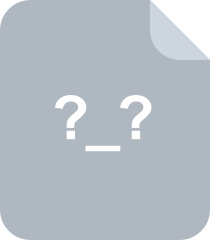
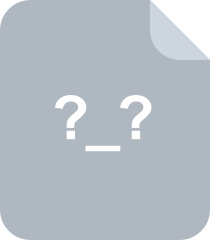
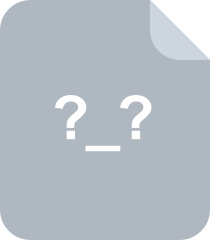
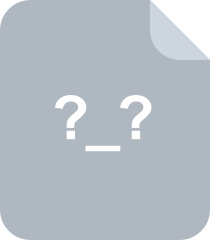
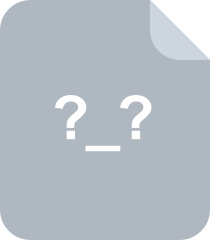
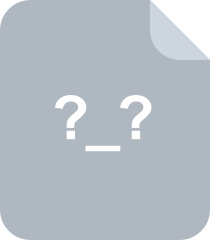
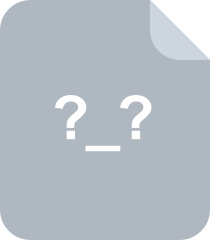
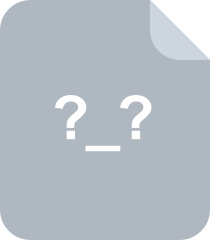
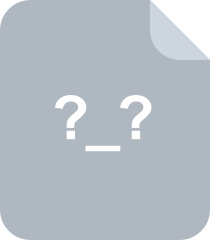
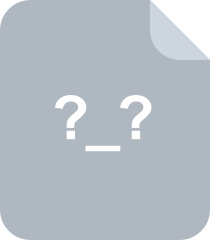
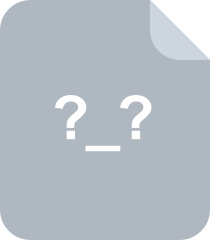
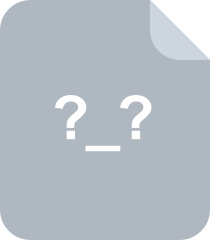
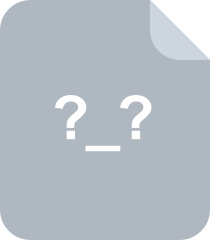
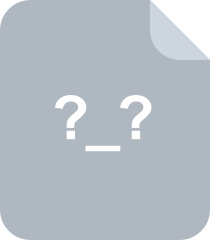
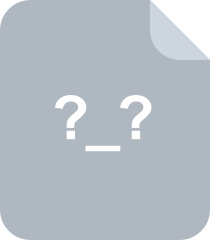
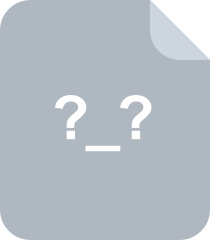
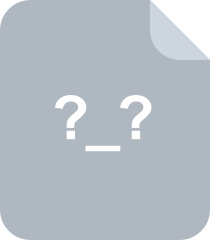
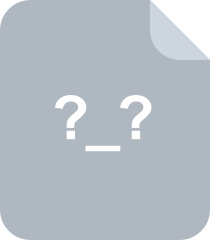
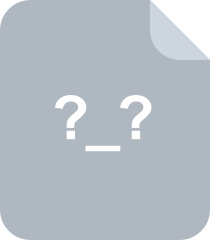
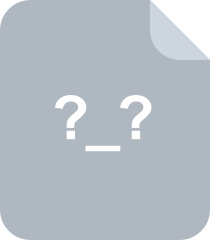
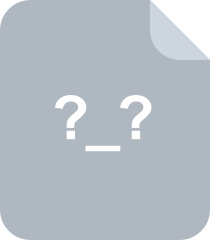
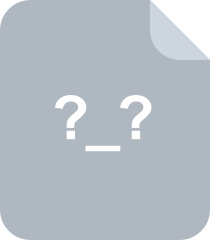
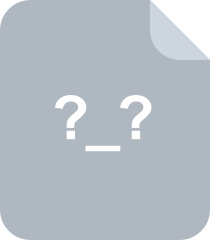
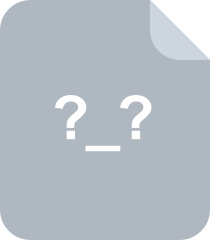
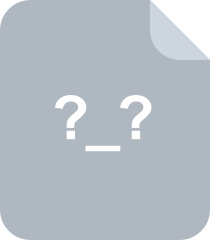
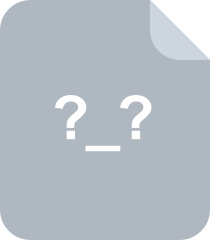
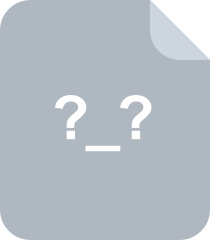
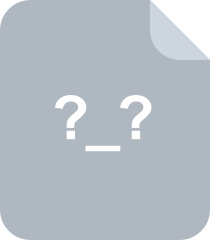
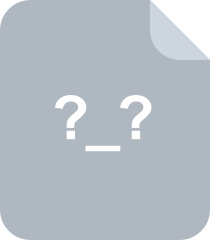
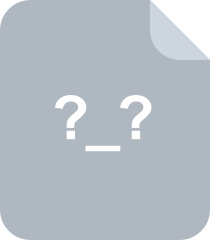
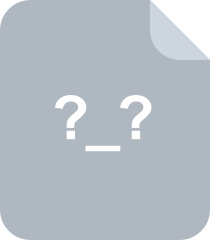
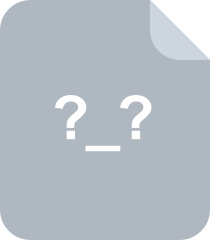
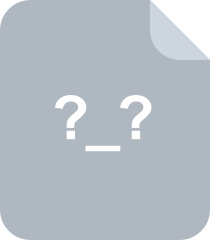
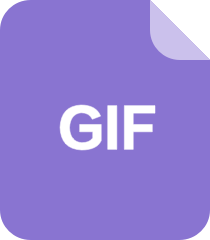
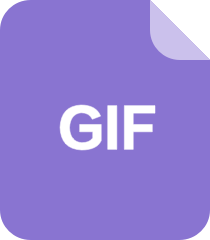
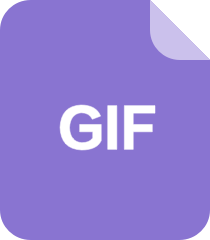
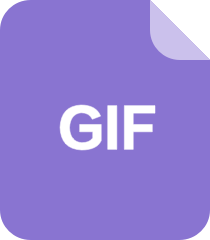
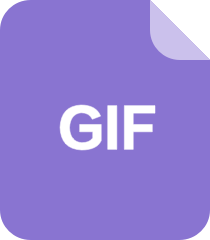
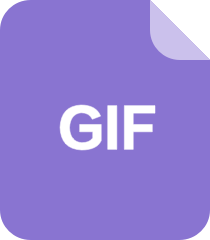
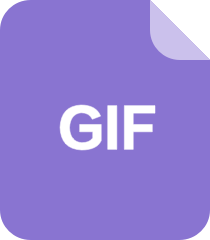
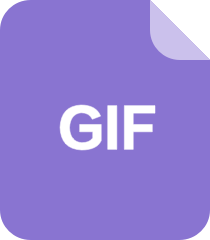
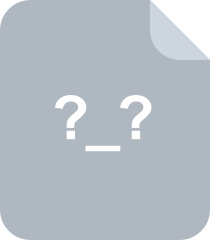
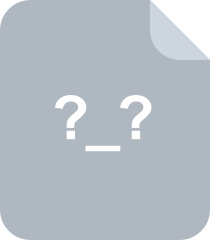
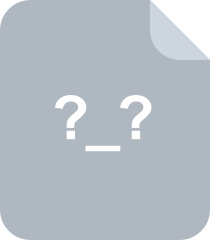
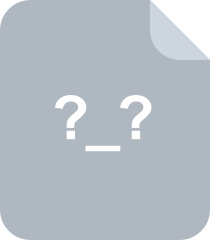
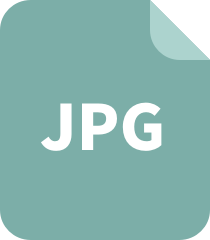
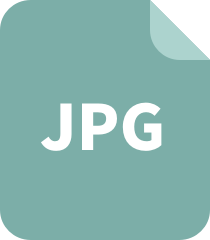
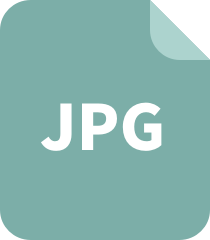
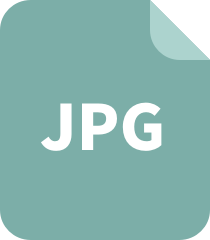
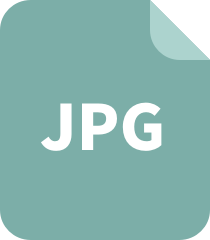
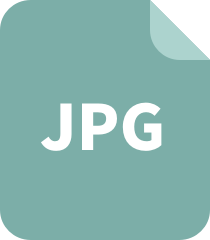
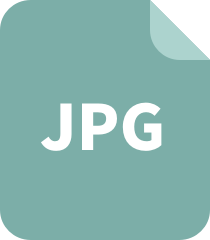
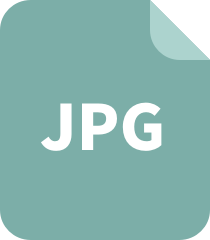
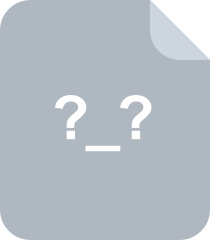
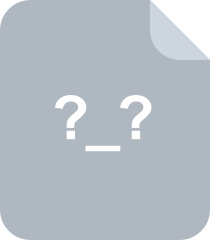
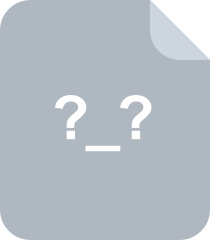
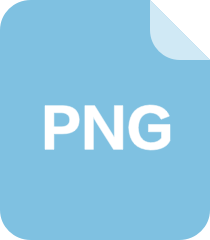
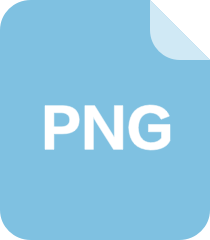
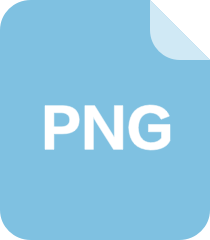
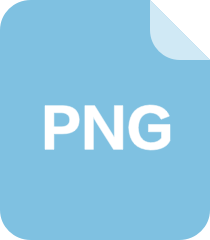
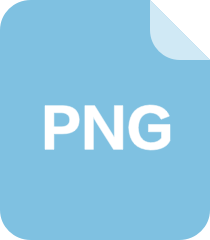
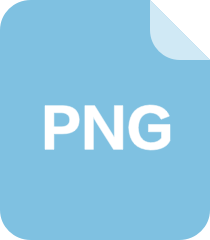
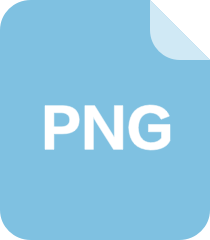
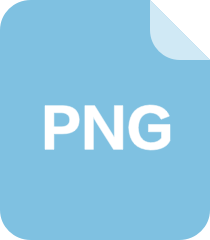
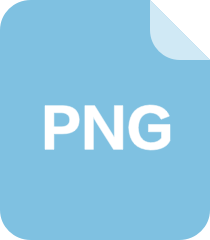
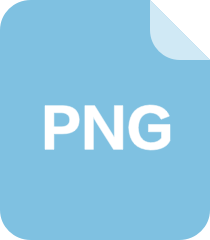
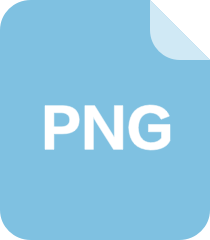
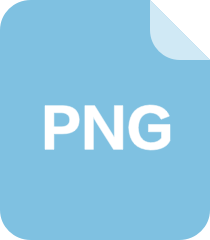
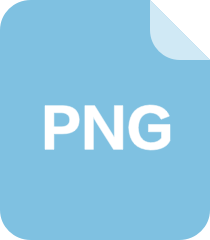
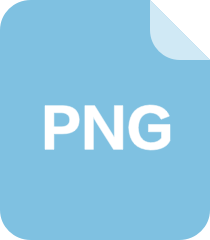
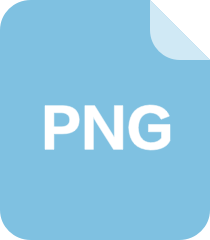
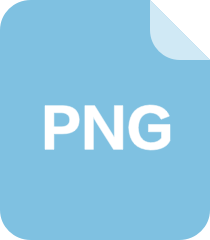
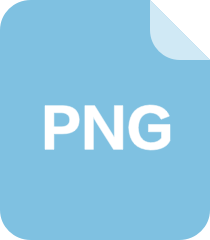
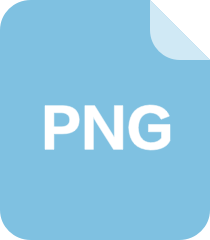
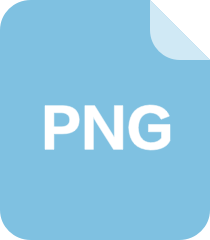
共 141 条
- 1
- 2
资源评论
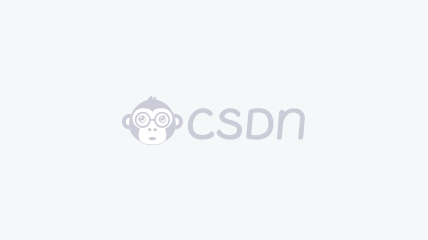

小云同志你好
- 粉丝: 1065
- 资源: 1061
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

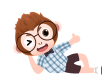
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


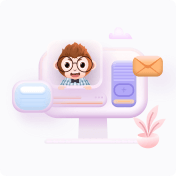
安全验证
文档复制为VIP权益,开通VIP直接复制
