package ticketbooking.util.database;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.*;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.JFrame;
import net.sf.jasperreports.engine.JasperCompileManager;
import net.sf.jasperreports.engine.JasperFillManager;
import net.sf.jasperreports.engine.JasperPrint;
import net.sf.jasperreports.engine.JasperReport;
import net.sf.jasperreports.view.JRViewer;
import org.sqlite.SQLiteConfig;
import ticketbooking.alert.AlertMaker;
import ticketbooking.models.*;
public class DatabaseHandler {
private static final String Jdbc_driver="org.sqlite.JDBC";
private static final String Connection_string="jdbc:sqlite:Aurora.db";
public static final String users_table="CREATE TABLE if not exists users(" +
"username VARCHAR(20) PRIMARY KEY NOT NULL,"+
"fullname varchar(40) NOT NULL,"+
"email varchar(30) NOT NULL,"+
"password varchar(60) NOT NULL,"+
"phone INT(10) NOT NULL)";
public static final String admin_table="CREATE TABLE if not exists admin(" +
"username VARCHAR(20) PRIMARY KEY NOT NULL,"+
"password VARCHAR(60) NOT NULL)";
public static final String movie_table="CREATE TABLE if not exists movie("+
"id INT(5) PRIMARY KEY NOT NULL,"+
"name VARCHAR UNIQUE NOT NULL,"+
"director varchar NOT NULL,"+
"cast varchar NOT NULL,"+
"details varchar NOT NULL,"+
"rating DECIMAL NOT NULL,"+
"poster BLOB NOT NULL)";
public static final String theatre_table="CREATE TABLE if not exists theatre("+
"id INT(5) PRIMARY KEY NOT NULL,"+
"name VARCHAR NOT NULL,"+
"address VARCHAR NOT NULL,"+
"hall INT(1) NOT NULL,"+
"platinum DECIMAL NOT NULL,"+
"gold DECIMAL NOT NULL,"+
"silver DECIMAL NOT NULL,"+
"UNIQUE(name,hall))";
public static final String show_table="CREATE TABLE if not exists show(" +
"movieid INT(5)," +
"theatreid INT(5)," +
"date DATE," +
"time TIME," +
"moviename VARCHAR," +
"theatrename VARCHAR," +
"hall INT(1)," +
"platinumseat INT," +
"goldseat INT," +
"silverseat INT," +
"FOREIGN KEY (movieid)" +
"REFERENCES movie (id)," +
"FOREIGN KEY (theatreid)" +
"REFERENCES theatre (id)," +
"PRIMARY KEY (theatreid,date,time))";
public static final String order_table="CREATE TABLE if not exists [order] "
+ "(orderid INTEGER PRIMARY KEY AUTOINCREMENT,username VARCHAR (20) REFERENCES users (username)"
+ ",movieid INT(5),theatreid INT(5),date DATE,time TIME,seats INT,category VARCHAR,total INT"
+ ",ordertime DATETIME,FOREIGN KEY (theatreid,date,time)REFERENCES show (theatreid,date,time));";
static Connection con=null;
static Statement stmt=null;
//This function connects the App with the database
public static void connect()
{
try {
Class.forName(Jdbc_driver);
SQLiteConfig config = new SQLiteConfig();
config.enforceForeignKeys(true);
con=DriverManager.getConnection(Connection_string,config.toProperties());
stmt=con.createStatement();
}
catch (ClassNotFoundException | SQLException ex)
{
AlertMaker.showNotification("Database Error","Connection not Established",AlertMaker.image_link);
}
}
public static void disconnect()
{
if(con!=null)
try {
stmt.close();
con.close();
} catch (SQLException ex) {
AlertMaker.showNotification("Database Error","Could not disconnect!",AlertMaker.image_cross);
}
}
//Modules to create tables for database ------------------------------------
public static void create_table(String create_table)
{
try {
stmt.executeUpdate(create_table);
} catch ( SQLException e ) {
AlertMaker.showErrorMessage(e);
}
}
public static void create_users_table()
{
create_table(users_table);
}
public static void create_admin_table()
{
create_table(admin_table);
}
public static void create_movie_table()
{
create_table(movie_table);
}
public static void create_theatre_table()
{
create_table(theatre_table);
}
public static void create_show_table()
{
create_table(show_table);
}
public static void create_order_table()
{
create_table(order_table);
}
public static void create_all_tables()
{
connect();
System.out.println("connected");
create_users_table();
create_admin_table();
create_movie_table();
create_theatre_table();
create_show_table();
create_order_table();
insert_root_admin();
disconnect();
}
//--------------------------------------------------------------------------
//Modules to insert record in a table---------------------------------------
public static void insert_root_admin()
{
String sql="insert into admin values('root123','cbfdac6008f9cab4083784cbd1874f76618d2a97')";
try{
stmt.executeUpdate(sql);
}
catch(SQLException ex)
{
System.out.println("Root admin already present.");
}
}
public static int insert_record(String sql)
{
int val=0;
try {
connect();
val=stmt.executeUpdate(sql);
} catch ( SQLException e ) {
System.out.println(e.getMessage());
}
return val;
}
public static boolean insert_show(String sql)
{
try{
stmt.executeUpdate(sql);
return true;
}
catch(SQLException ex)
{
System.out.println(ex.getMessage());
return false;
}
}
public static int insert_user(User user)
{
String sql = "INSERT INTO users VALUES('"+
user.getUsername()+"','"+
user.getFullname()+"','"+
user.getEmail()+"','"+
user.getPassword()+"','"+
user.getContact()+"')";
return insert_record(sql);
}
public static int insert_admin(Admin admin)
{
String sql = "INSERT INTO admin VALUES('"+
admin.getUsername()+"','"+
admin.getPassword()+"')";
return insert_record(sql);
}
public static boolean order_transaction(String sql1,String sql2)
{
stmt=null;
con=null;
//disconnect();
connect();
try {
con.setAutoCommit(false);
stmt.executeUpdate(sql1);
stmt.executeUpdate(sql2);
con.commit();
con.setAutoCommit(true);
return true;
} catch (SQLException ex) {
System.out.println(ex.getMessage());
try {
con.rollback();
con.setAutoCommit(true);
} catch (SQLException ex1) {
Logger.getLogger(DatabaseHandler.class.getName()).log(Level.SEVERE, null, ex1);
}
return false;
}
}
public static int insert_movie(String sql,File file)
{
int val=0;
try {
connect();
PreparedStatement ps=con.prepareStatement(sql);
FileInputStream input=new FileInputStream(file);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buf = new byte[1024];
for (int readNum; (readNum = input.read(buf)) != -1;){
bo
没有合适的资源?快使用搜索试试~ 我知道了~
Java电影管理系统源码项目.rar
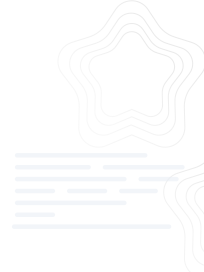
共231个文件
java:50个
fxml:46个
png:42个

0 下载量 118 浏览量
2023-09-05
10:00:09
上传
评论
收藏 22.13MB RAR 举报
温馨提示
Java电影管理系统源码项目 电影管理系统是一个java项目。在此项目中,您可以在两个不同的模块中使用它,即一个作为用户,另一个作为系统管理员。作为用户,您只能预订电影放映并查看其详细信息。而作为管理员,您可以管理整个剧院。您可以添加和删除电影节目。此外,您还可以添加不同的剧院。
资源推荐
资源详情
资源评论
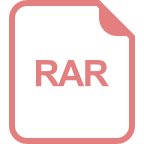
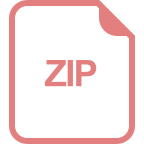
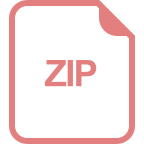
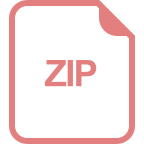
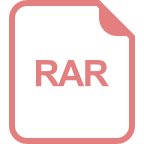
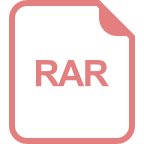
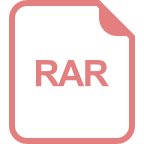
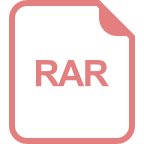
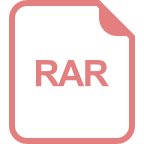
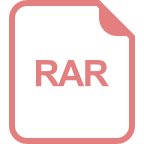
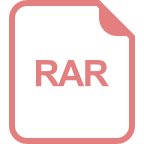
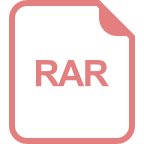
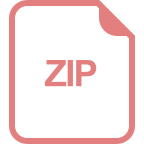
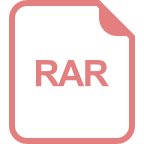
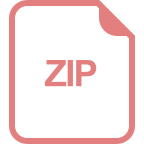
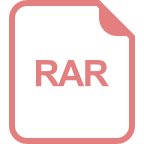
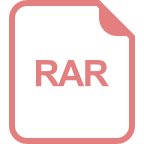
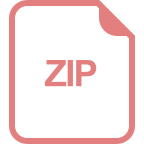
收起资源包目录

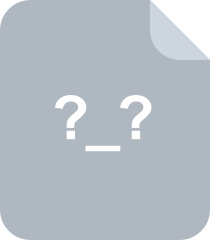
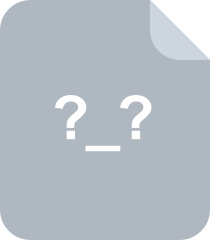
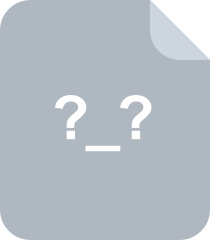
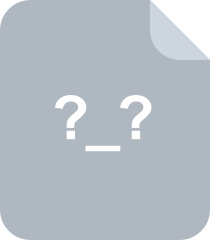
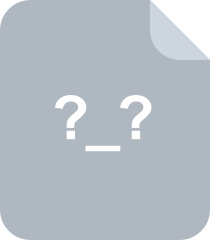
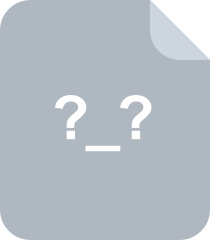
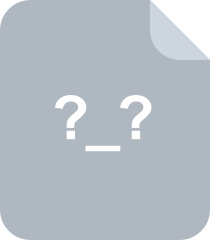
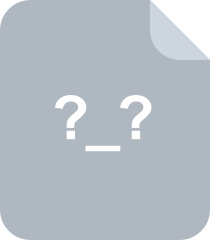
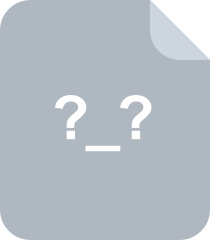
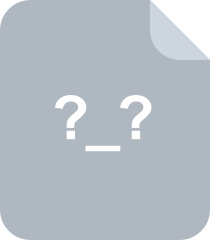
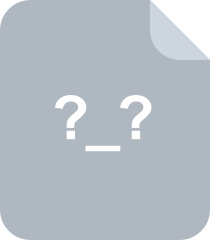
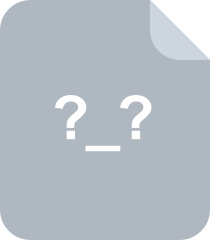
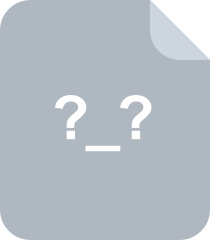
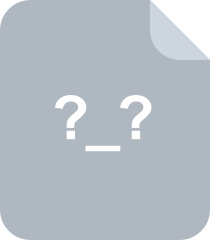
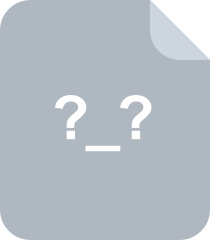
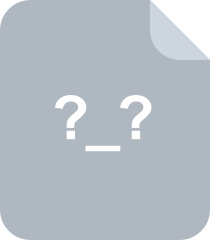
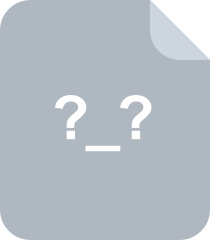
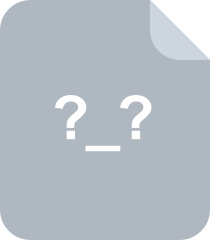
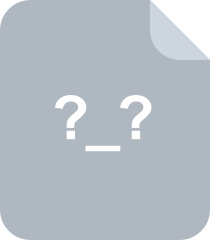
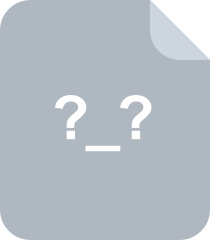
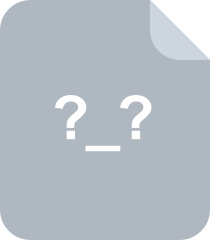
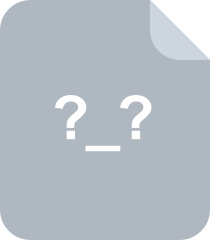
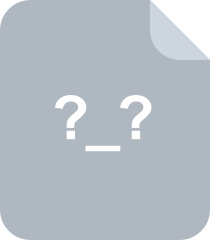
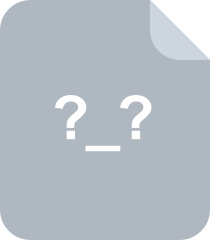
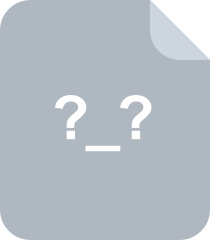
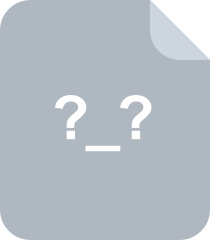
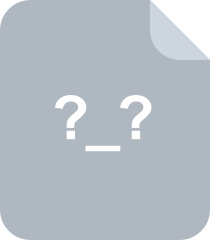
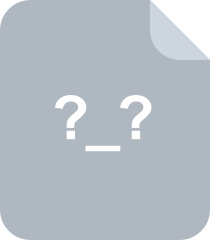
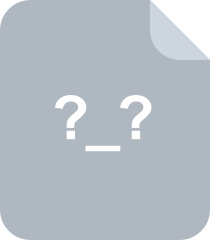
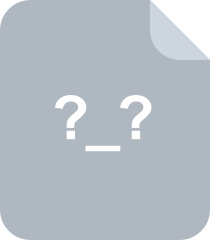
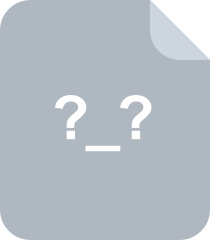
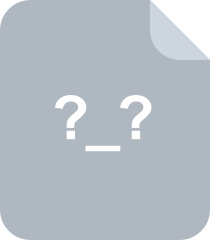
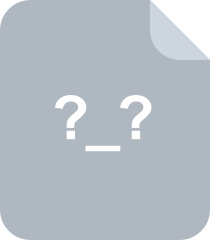
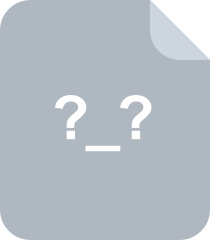
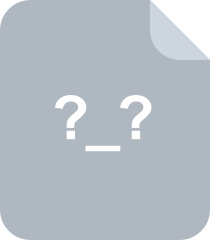
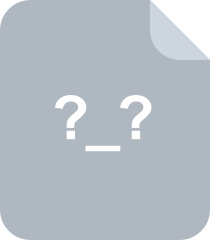
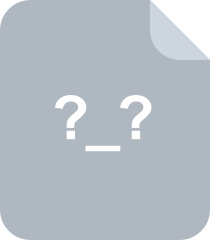
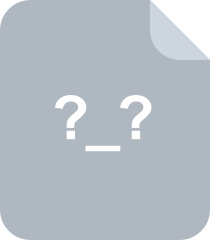
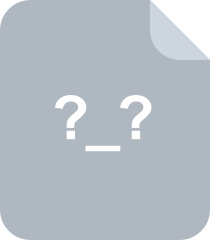
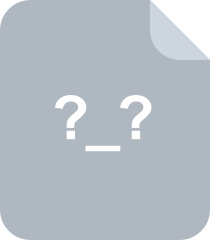
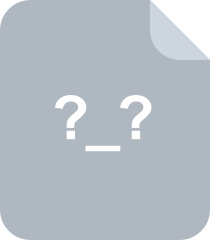
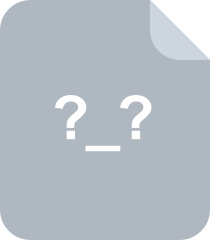
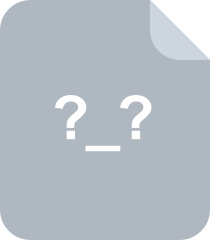
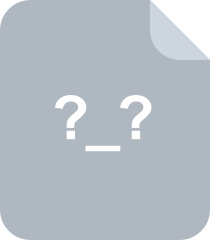
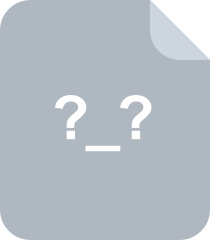
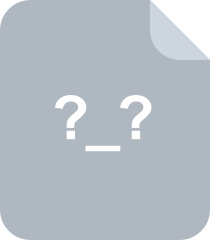
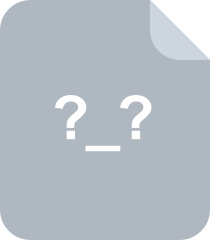
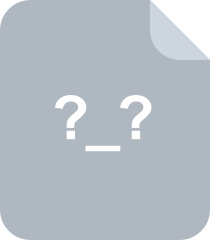
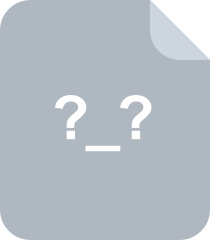
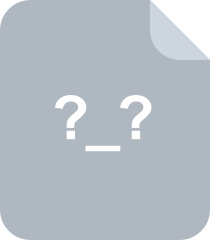
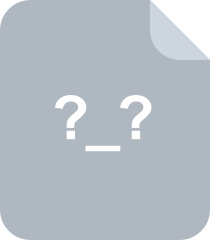
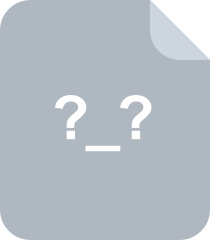
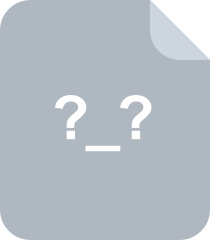
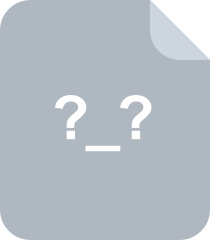
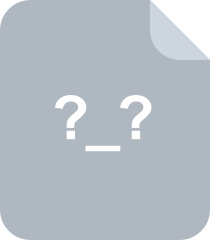
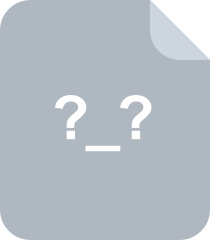
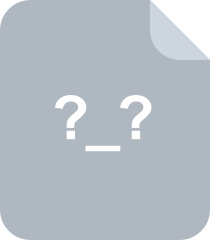
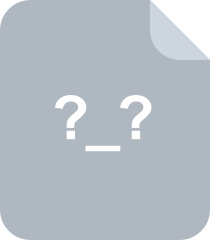
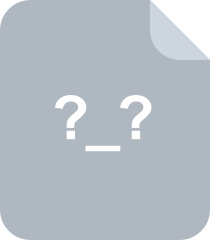
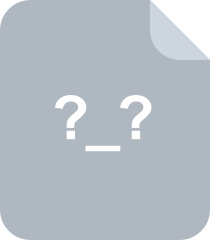
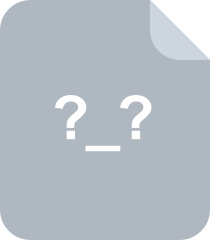
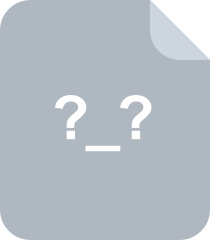
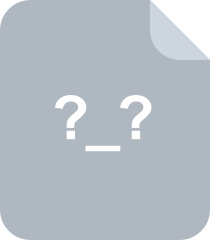
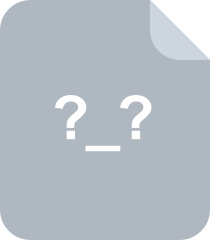
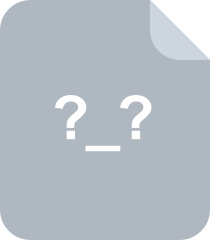
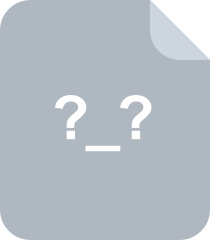
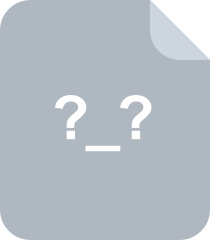
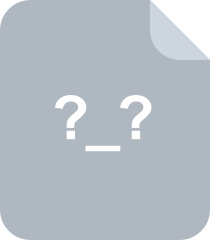
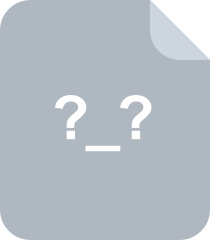
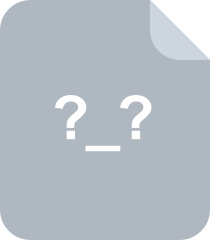
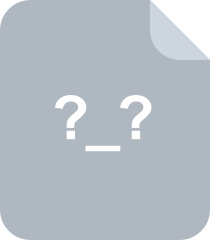
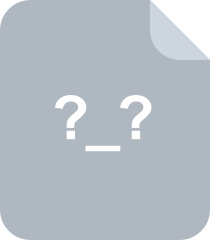
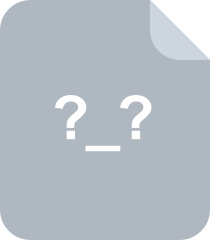
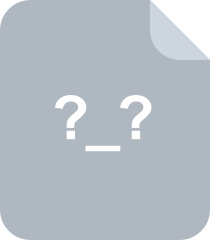
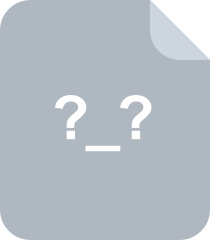
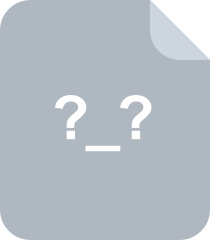
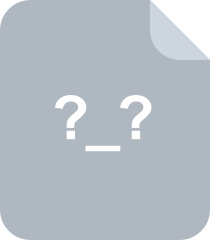
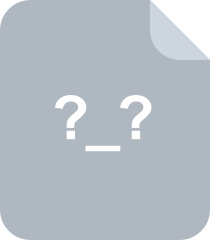
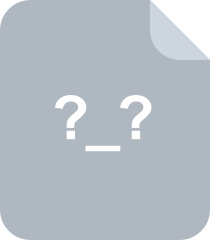
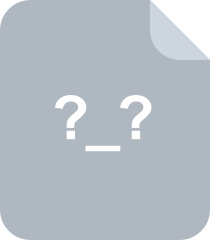
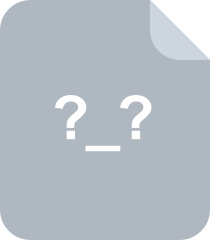
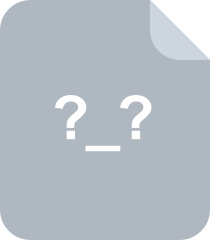
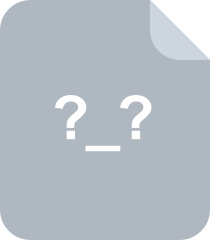
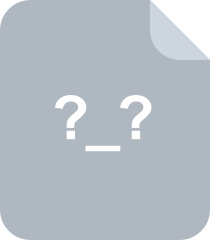
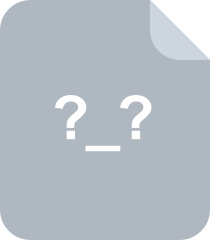
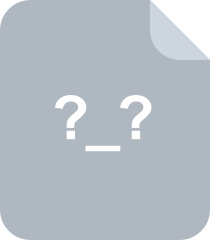
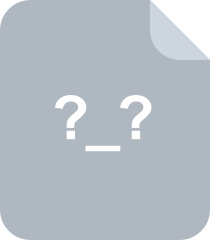
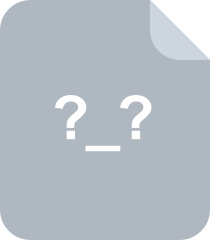
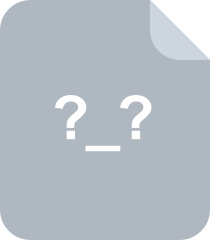
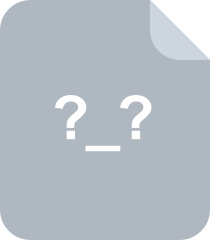
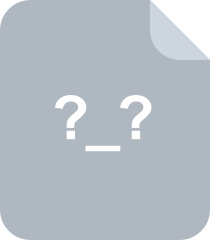
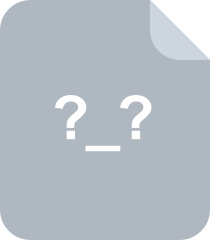
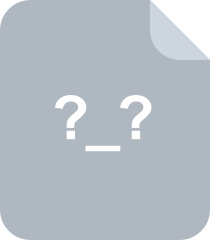
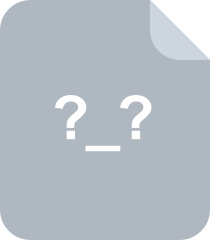
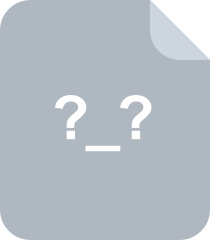
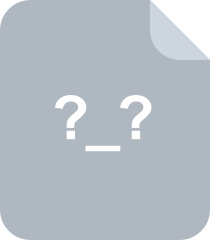
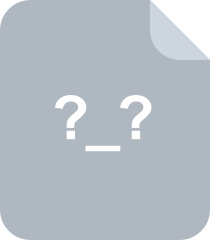
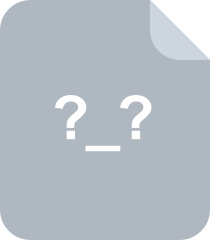
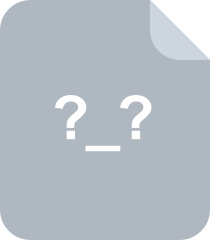
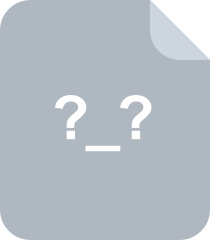
共 231 条
- 1
- 2
- 3
资源评论
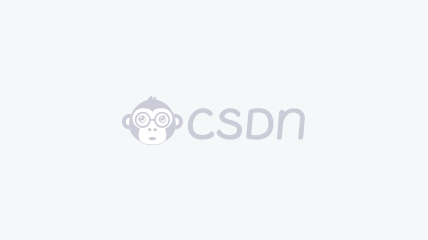

小云同志你好
- 粉丝: 1065
- 资源: 1061
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

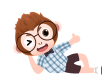
最新资源
- 2023年海南省海口市中考物理真题及答案.doc
- 2023年海南省高考生物试卷及答案.doc
- 2023年海南省中考物理真题及答案.doc
- 2023年海南省三亚市中考物理真题及答案.doc
- 2023年黑龙江哈尔滨中考英语真题及答案.doc
- 2023年湖北恩施州中考语文真题及答案.doc
- 2023年黑龙江哈尔滨中考语文真题及答案.doc
- Lazarus RSA 生成公私钥及加密解密代码,可直接用于工程中.7z
- CM0304bt442和NB433阵型.rar
- 2023年湖北黄冈中考数学真题及答案.doc
- 蓝桥杯赛事备考攻略与资源整合
- 2023年湖北十堰中考地理真题及答案.doc
- 2023年湖北随州中考物理真题及答案.doc
- Direct 3D 中基于动作的游戏引擎.zip
- Apache OpenOffice 开源办公软件合计4.1.14 Windows x86.exe
- Direct3D 11 (FX11) 的效果是一个管理运行时,用于一起创作 HLSL 着色器、渲染状态和运行时变量 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


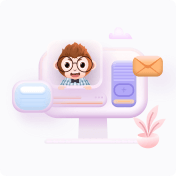
安全验证
文档复制为VIP权益,开通VIP直接复制
