### MD5加密算法 #### 简介 MD5(Message-Digest Algorithm 5)是一种广泛使用的密码散列函数,可以产生一个128位(16字节)的散列值,通常用来确保数据的完整性和进行简单的密码加密。在Java中,MD5可以通过`java.security.MessageDigest`类来实现。 #### Java实现 以下是一段Java代码示例,展示了如何使用Java实现MD5加密: ```java package com; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; public class MD5 { private final static String[] strDigits = {"0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"}; public MD5() { } private static String byteToArrayString(byte bByte) { int iRet = bByte; if (iRet < 0) { iRet += 256; } int iD1 = iRet / 16; int iD2 = iRet % 16; return strDigits[iD1] + strDigits[iD2]; } private static String byteToNum(byte bByte) { int iRet = bByte; if (iRet < 0) { iRet += 256; } return String.valueOf(iRet); } private static String byteToString(byte[] bByte) { StringBuffer sBuffer = new StringBuffer(); for (int i = 0; i < bByte.length; i++) { sBuffer.append(byteToArrayString(bByte[i])); } return sBuffer.toString(); } public static String getMD5Code(String strObj) { String resultString = null; try { resultString = new String(strObj); MessageDigest md = MessageDigest.getInstance("MD5"); // 将字符串转换为字节数组,并计算其散列值 resultString = byteToString(md.digest(strObj.getBytes())); } catch (NoSuchAlgorithmException ex) { ex.printStackTrace(); } return resultString; } public static void main(String[] args) { MD5 getMD5 = new MD5(); System.out.println(getMD5.getMD5Code("000001")); } } ``` #### 解析与扩展 1. **初始化MD5对象**: - `MessageDigest.getInstance("MD5")`:此方法用于创建一个MD5消息摘要对象,它是通过传递字符串“MD5”作为参数实现的。这一步是使用MD5算法的前提。 2. **字符串到字节数组的转换**: - `strObj.getBytes()`:将输入的字符串转换为字节数组,这是为了适应`MessageDigest`类中的`digest()`方法,该方法接受字节数组作为输入。 3. **计算散列值**: - `md.digest(strObj.getBytes())`:调用`digest()`方法计算输入字符串的散列值。返回的是一个字节数组。 4. **字节数组转十六进制字符串**: - `byteToString(md.digest(strObj.getBytes()))`:这个步骤将上述得到的字节数组转换成一个十六进制形式的字符串表示。这是通过遍历数组并将每个字节转换成两个十六进制字符的方式完成的。 5. **异常处理**: - `catch (NoSuchAlgorithmException ex)`:这里捕获了一个可能发生的异常,即无法找到指定算法的情况。这通常发生在JVM不支持所请求的算法时。 6. **输出结果**: - 在`main`方法中,通过调用`getMD5Code`方法并传入一个测试字符串,最后打印出计算得到的MD5散列值。 #### 使用场景与限制 - **应用场景**:MD5常被用于数据校验、密码存储等场合。例如,在用户登录系统中,可以将用户的明文密码通过MD5加密后存储于数据库中,登录时只需对用户输入的密码进行同样的加密操作并与数据库中的记录比较即可。 - **安全性问题**:尽管MD5算法在早期非常流行,但随着时间的发展,人们已经找到了一些有效的攻击方法来破解MD5哈希值,因此它不再被认为是安全的密码散列算法。对于安全性要求较高的应用,建议使用更现代的散列函数,如SHA-256等。 通过以上分析,我们可以看出这段Java代码提供了一个简单的MD5加密实现方案,适用于一些基本的数据加密需求。然而,在实际应用中,还需要考虑更多的安全因素,以确保系统的整体安全性。
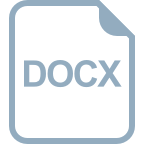
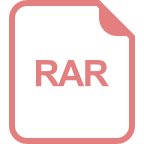
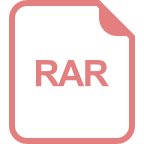
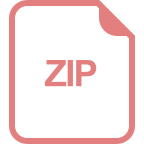
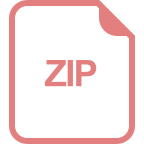
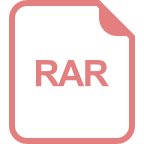
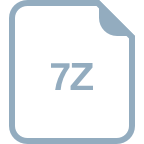
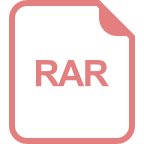
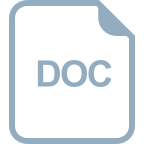
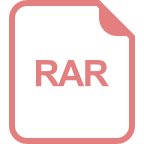
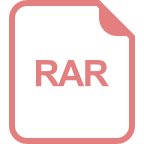
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
/*
* MD5 算法
*/
public class MD5 {
// 全局数组
private final static String[] strDigits = { "0", "1", "2", "3", "4", "5",
"6", "7", "8", "9", "a", "b", "c", "d", "e", "f" };
public MD5() {
}
// 返回形式为数字跟字符串
private static String byteToArrayString(byte bByte) {
int iRet = bByte;
// System.out.println("iRet="+iRet);
if (iRet < 0) {
iRet += 256;
}
int iD1 = iRet / 16;
int iD2 = iRet % 16;
return strDigits[iD1] + strDigits[iD2];
}
// 返回形式只为数字
private static String byteToNum(byte bByte) {
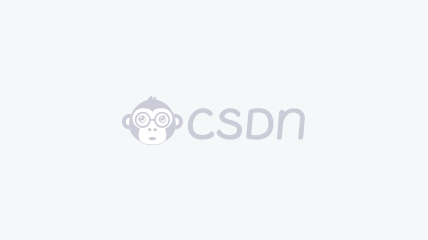

- 粉丝: 5
- 资源: 16
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

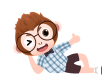
最新资源

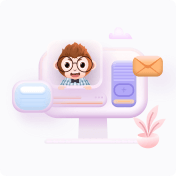
