#include "cmainwindow.h"
#define STRETCH_RECT_WIDTH 8
#define STRETCH_RECT_HEIGHT 8
#define MIN_WIDTH 400
#define MIN_HEIGHT 300
CMainWindow::CMainWindow(QWidget *parent)
: QWidget(parent)
, m_bMousePressed(false)
, m_nMouseStyle(NO_SELECT)
, m_pTitleWidget(NULL)
{
this->resize(MIN_WIDTH, MIN_HEIGHT);
initMainWindow();
initWidget();
assembleWidget();
updateWindowStretchRect();
setSupportStretch(true);
}
CMainWindow::~CMainWindow()
{
}
void CMainWindow::initMainWindow()
{
setWindowFlags(Qt::FramelessWindowHint); // 设置窗口标志
setMinimumSize(MIN_WIDTH, MIN_HEIGHT); // 设置最小尺寸
setSizePolicy(QSizePolicy::Preferred, QSizePolicy::Preferred); // 设置尺寸属性
setMouseTracking(true); // 界面拉伸需要这个属性
}
void CMainWindow::initWidget()
{
m_pTitleWidget = new QWidget(this);
}
void CMainWindow::assembleWidget()
{
m_pTitleWidget->setGeometry(0, 0, width(), height());
m_pTitleWidget->setStyleSheet("background: rgb(249, 126, 129);");
}
void CMainWindow::mousePressEvent(QMouseEvent *event)
{
if( event->button() == Qt::LeftButton)
{
m_bMousePressed = true;
m_moveStartPoint = event->globalPos() - this->pos();
//当前窗口位置大小
windowRect = this->geometry();
}
event->ignore(); //表示继续向下传递事件,其他的控件还可以去获取
}
void CMainWindow::mouseReleaseEvent(QMouseEvent *event)
{
m_bMousePressed = false;
calculateCurrentStretchRect();
event->ignore();
}
void CMainWindow::mouseMoveEvent(QMouseEvent *event)
{
if(this->isMaximized())
{
return;
}
// stretchState = getCurrentStretchState(event->pos());
QPoint curPoint = event->pos();
//获取当前鼠标样式
if (!m_bMousePressed)
{
m_nMouseStyle = setCursorStyle(curPoint);
}
//拉伸窗口
if (m_bMousePressed && stretchState != NO_SELECT)
{
m_endPoint = this->mapToGlobal(event->pos());
updateWindowSize();
}
//移动窗口
if (m_bMousePressed && stretchState == NO_SELECT)
{
this->move(event->globalPos() - m_moveStartPoint);
}
updateWindowStretchRect();
event->ignore();
}
void CMainWindow::calculateCurrentStretchRect()
{
int width_X = width() - STRETCH_RECT_WIDTH * 2;
int height_Y = height() - STRETCH_RECT_HEIGHT * 2;
m_topBorderRect = QRect(STRETCH_RECT_WIDTH, 0, width_X, STRETCH_RECT_HEIGHT);
m_bottomBorderRect = QRect(STRETCH_RECT_WIDTH, height() - STRETCH_RECT_HEIGHT,
width_X, STRETCH_RECT_HEIGHT);
m_leftBorderRect = QRect(0, STRETCH_RECT_HEIGHT, STRETCH_RECT_WIDTH, height_Y);
m_rightBorderRect = QRect(width() - STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT,
STRETCH_RECT_WIDTH, height_Y);
m_leftTopRect = QRect(0, 0, STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
m_leftBottomRect = QRect(0, height() - STRETCH_RECT_HEIGHT,
STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
m_rightTopRect = QRect(width() - STRETCH_RECT_WIDTH, 0,
STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
m_rightBottomRect = QRect(width() - STRETCH_RECT_WIDTH, height() - STRETCH_RECT_HEIGHT,
STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
}
void CMainWindow::updateWindowStretchRect()
{
calculateCurrentStretchRect();
}
CMainWindow::WindowStretchRectState CMainWindow::getCurrentStretchState(QPoint curPoint)
{
WindowStretchRectState stretchState;
if (m_leftTopRect.contains(curPoint))
stretchState = LEFT_TOP_RECT;
else if (m_leftBottomRect.contains(curPoint))
stretchState = LEFT_BOTTOM_RECT;
else if (m_rightTopRect.contains(curPoint))
stretchState = RIGHT_TOP_RECT;
else if (m_rightBottomRect.contains(curPoint))
stretchState = RIGHT_BOTTOM_RECT;
else if (m_leftBorderRect.contains(curPoint))
stretchState = LEFT_BORDER;
else if (m_rightBorderRect.contains(curPoint))
stretchState = RIGHT_BORDER;
else if (m_topBorderRect.contains(curPoint))
stretchState = TOP_BORDER;
else if (m_bottomBorderRect.contains(curPoint))
stretchState = BOTTOM_BORDER;
else
stretchState = NO_SELECT;
return stretchState;
}
void CMainWindow::updateWindowSize()
{
switch(stretchState)
{
case LEFT_BORDER:
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setX(m_endPoint.x());
windowRect.setTopLeft(topLeftPoint);
}
break;
case RIGHT_BORDER:
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setX(m_endPoint.x());
windowRect.setBottomRight(bottomRightPoint);
}
break;
case TOP_BORDER:
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setY(m_endPoint.y());
windowRect.setTopLeft(topLeftPoint);
}
break;
case BOTTOM_BORDER:
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setY(m_endPoint.y());
windowRect.setBottomRight(bottomRightPoint);
}
break;
case LEFT_TOP_RECT:
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setX(m_endPoint.x());
topLeftPoint.setY(m_endPoint.y());
windowRect.setTopLeft(topLeftPoint);
}
break;
case LEFT_BOTTOM_RECT:
{
QPoint bottomLeftPoint = windowRect.bottomLeft();
bottomLeftPoint.setX(m_endPoint.x());
bottomLeftPoint.setY(m_endPoint.y());
windowRect.setBottomLeft(bottomLeftPoint);
}
break;
case RIGHT_TOP_RECT:
{
QPoint topRightPoint = windowRect.topRight();
topRightPoint.setX(m_endPoint.x());
topRightPoint.setY(m_endPoint.y());
windowRect.setTopRight(topRightPoint);
}
break;
case RIGHT_BOTTOM_RECT:
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setX(m_endPoint.x());
bottomRightPoint.setY(m_endPoint.y());
windowRect.setBottomRight(bottomRightPoint);
}
break;
default:
break;
}
if (windowRect.width() < MIN_WIDTH)
{
windowRect.setLeft(this->geometry().left());
windowRect.setWidth(MIN_WIDTH);
}
if (windowRect.height() < MIN_HEIGHT)
{
windowRect.setTop(this->geometry().top());
windowRect.setHeight(MIN_HEIGHT);
}
this->setGeometry(windowRect);
m_pTitleWidget->setGeometry(0, 0, width(), 36);
}
void CMainWindow::setSupportStretch(bool isSupportStretch)
{
this->setMouseTracking(isSupportStretch);
//对子控件设置
QList<QWidget*> widgetList = this->findChildren<QWidget*>();
foreach (QWidget* widget, widgetList)
{
widget->setMouseTracking(isSupportStretch);
}
if (m_pTitleWidget != NULL)
{
m_pTitleWidget->setMouseTracking(isSupportStretch);
}
}
int CMainWindow::setCursorStyle(const QPoint& curPoint)
{
stretchState = getCurrentStretchState(curPoint);
switch(stretchState)
{
case LEFT_TOP_RECT:
case RIGHT_BOTTOM_RECT:
{
setCursor(Qt::SizeFDiagCursor);
}
break;
case RIGHT_TOP_RECT:
case LEFT_BOTTOM_RECT:
{
setCursor(Qt::SizeBDiagCursor);
}
break;
case LEFT_BORDER:
case RIGHT_BORDER:
{
setCursor(Qt::SizeHorCursor);
}
break;
case TOP_BORDER:
case BOTTOM_BORDER:
{
setCursor(Qt::SizeVerCursor);
}
break;
default:
{
setCursor(Qt::ArrowCursor);
}
没有合适的资源?快使用搜索试试~ 我知道了~
Qt 自定义窗口边框拉伸
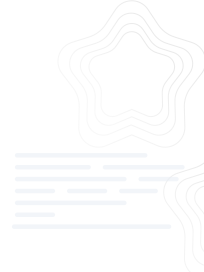
共4个文件
cpp:2个
pro:1个
h:1个


温馨提示
代码可直接使用,简单易懂,主要是将窗口分为9部分,四个角、四条边和窗口显示区,鼠标在不同区域时候获取不同鼠标图标,鼠标按下记录初始点坐标,按下时移动鼠标计算窗口应改变的大小,根据鼠标所在区域改变窗口大小。仅参考思路,就不传优化后的代码了,分高了评论下,我去改低,下载人多了分会自动涨
资源推荐
资源详情
资源评论
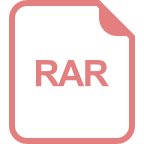
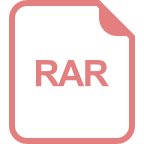
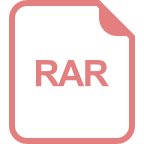
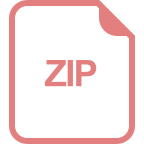
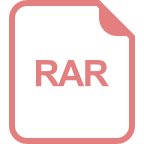
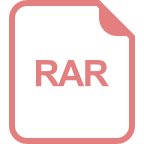
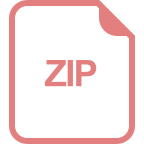
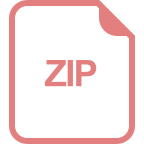
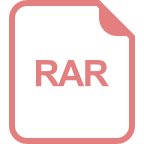
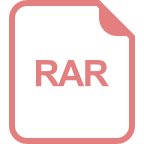
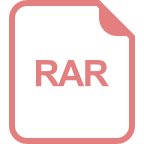
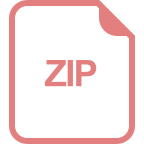
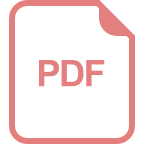
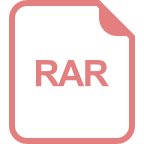
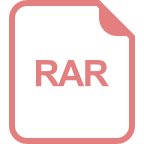
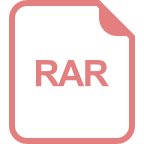
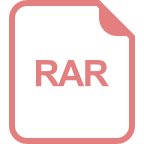
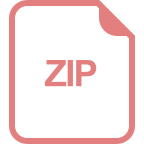
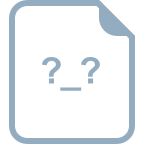
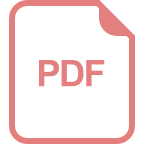
收起资源包目录

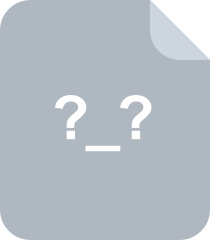
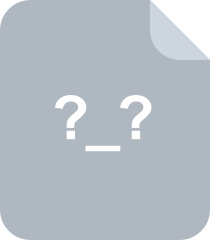
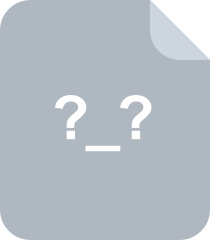
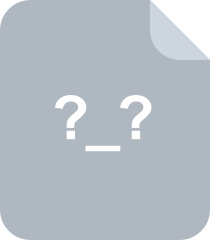
共 4 条
- 1
资源评论
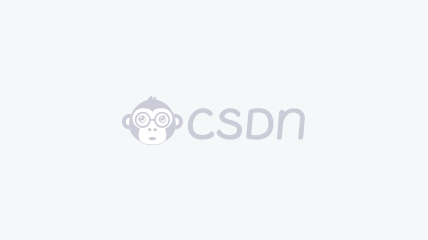
- 长沙红胖子Qt(长沙创微智科)2019-08-24闪烁这么明显也发上来?拖动右边和上边的时候兜兜转转又一年2019-10-25重要是思路,代码都是可以优化的,大学时候写的代码质量比较差,工作后都放笔记里面了

兜兜转转又一年
- 粉丝: 16
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

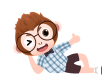
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


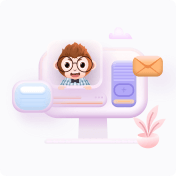
安全验证
文档复制为VIP权益,开通VIP直接复制
