# blessed
A curses-like library with a high level terminal interface API for node.js.
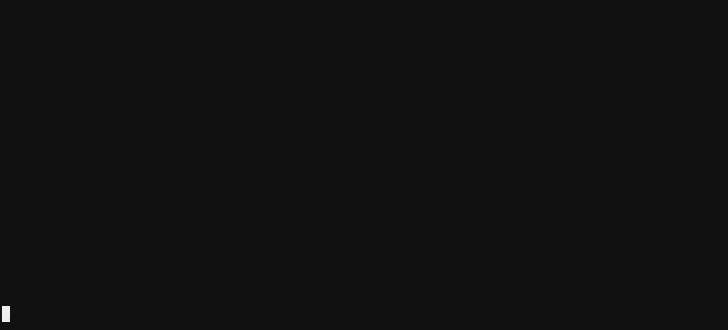
Blessed is over 16,000 lines of code and terminal goodness. It's completely
implemented in javascript, and its goal consists of two things:
1. Reimplement ncurses entirely by parsing and compiling terminfo and termcap,
and exposing a `Program` object which can output escape sequences compatible
with _any_ terminal.
2. Implement a widget API which is heavily optimized for terminals.
The blessed renderer makes use of CSR (change-scroll-region), and BCE
(back-color-erase). It draws the screen using the painter's algorithm and is
sped up with smart cursor movements and a screen damage buffer. This means
rendering of your application will be extremely efficient: blessed only draws
the changes (damage) to the screen.
Blessed is arguably as accurate as ncurses, but even more optimized in some
ways. The widget library gives you an API which is reminiscent of the DOM.
Anyone is able to make an awesome terminal application with blessed. There are
terminal widget libraries for other platforms (primarily [python][urwid] and
[perl][curses-ui]), but blessed is possibly the most DOM-like (dare I say the
most user-friendly?).
Blessed has been used to implement other popular libraries and programs.
Examples include: the [slap text editor][slap] and [blessed-contrib][contrib].
The blessed API itself has gone on to inspire [termui][termui] for Go.
## Install
``` bash
$ npm install blessed
```
## Example
This will render a box with line borders containing the text `'Hello world!'`,
perfectly centered horizontally and vertically.
__NOTE__: It is recommend you use either `smartCSR` or `fastCSR` as a
`blessed.screen` option. This will enable CSR when scrolling text in elements
or when manipulating lines.
``` js
var blessed = require('blessed');
// Create a screen object.
var screen = blessed.screen({
smartCSR: true
});
screen.title = 'my window title';
// Create a box perfectly centered horizontally and vertically.
var box = blessed.box({
top: 'center',
left: 'center',
width: '50%',
height: '50%',
content: 'Hello {bold}world{/bold}!',
tags: true,
border: {
type: 'line'
},
style: {
fg: 'white',
bg: 'magenta',
border: {
fg: '#f0f0f0'
},
hover: {
bg: 'green'
}
}
});
// Append our box to the screen.
screen.append(box);
// Add a png icon to the box
var icon = blessed.image({
parent: box,
top: 0,
left: 0,
type: 'overlay',
width: 'shrink',
height: 'shrink',
file: __dirname + '/my-program-icon.png',
search: false
});
// If our box is clicked, change the content.
box.on('click', function(data) {
box.setContent('{center}Some different {red-fg}content{/red-fg}.{/center}');
screen.render();
});
// If box is focused, handle `enter`/`return` and give us some more content.
box.key('enter', function(ch, key) {
box.setContent('{right}Even different {black-fg}content{/black-fg}.{/right}\n');
box.setLine(1, 'bar');
box.insertLine(1, 'foo');
screen.render();
});
// Quit on Escape, q, or Control-C.
screen.key(['escape', 'q', 'C-c'], function(ch, key) {
return process.exit(0);
});
// Focus our element.
box.focus();
// Render the screen.
screen.render();
```
## Documentation
### Widgets
- [Base Nodes](#base-nodes)
- [Node](#node-from-eventemitter) (abstract)
- [Screen](#screen-from-node)
- [Element](#element-from-node) (abstract)
- [Boxes](#boxes)
- [Box](#box-from-element)
- [Text](#text-from-element)
- [Line](#line-from-box)
- [ScrollableBox](#scrollablebox-from-box) (deprecated)
- [ScrollableText](#scrollabletext-from-scrollablebox) (deprecated)
- [BigText](#bigtext-from-box)
- [Lists](#lists)
- [List](#list-from-box)
- [FileManager](#filemanager-from-list)
- [ListTable](#listtable-from-list)
- [Listbar](#listbar-from-box)
- [Forms](#forms)
- [Form](#form-from-box)
- [Input](#input-from-box) (abstract)
- [Textarea](#textarea-from-input)
- [Textbox](#textbox-from-textarea)
- [Button](#button-from-input)
- [Checkbox](#checkbox-from-input)
- [RadioSet](#radioset-from-box)
- [RadioButton](#radiobutton-from-checkbox)
- [Prompts](#prompts)
- [Prompt](#prompt-from-box)
- [Question](#question-from-box)
- [Message](#message-from-box)
- [Loading](#loading-from-box)
- [Data Display](#data-display)
- [ProgressBar](#progressbar-from-input)
- [Log](#log-from-scrollabletext)
- [Table](#table-from-box)
- [Special Elements](#special-elements)
- [Terminal](#terminal-from-box)
- [Image](#image-from-box)
- [ANSIImage](#ansiimage-from-box)
- [OverlayImage](#overlayimage-from-box)
- [Video](#video-from-box)
- [Layout](#layout-from-element)
### Other
- [Helpers](#helpers)
### Mechanics
- [Content & Tags](#content--tags)
- [Colors](#colors)
- [Attributes](#attributes)
- [Alignment](#alignment)
- [Escaping](#escaping)
- [SGR Sequences](#sgr-sequences)
- [Style](#style)
- [Colors](#colors-1)
- [Attributes](#attributes-1)
- [Transparency](#transparency)
- [Shadow](#shadow)
- [Effects](#effects)
- [Events](#events)
- [Event Bubbling](#event-bubbling)
- [Poisitioning](#positioning)
- [Rendering](#rendering)
- [Artificial Cursors](#artificial-cursors)
- [Multiple Screens](#multiple-screens)
- [Server Side Usage](#server-side-usage)
### Notes
- [Windows Compatibility](#windows-compatibility)
- [Low-level Usage](#low-level-usage)
- [Testing](#testing)
- [Examples](#examples)
- [FAQ](#faq)
## Widgets
Blessed comes with a number of high-level widgets so you can avoid all the
nasty low-level terminal stuff.
### Base Nodes
#### Node (from EventEmitter)
The base node which everything inherits from.
##### Options:
- __screen__ - The screen to be associated with.
- __parent__ - The desired parent.
- __children__ - An arrray of children.
##### Properties:
- Inherits all from EventEmitter.
- __type__ - Type of the node (e.g. `box`).
- __options__ - Original options object.
- __parent__ - Parent node.
- __screen__ - Parent screen.
- __children__ - Array of node's children.
- __data, _, $__ - An object for any miscellanous user data.
- __index__ - Render index (document order index) of the last render call.
##### Events:
- Inherits all from EventEmitter.
- __adopt__ - Received when node is added to a parent.
- __remove__ - Received when node is removed from it's current parent.
- __reparent__ - Received when node gains a new parent.
- __attach__ - Received when node is attached to the screen directly or
somewhere in its ancestry.
- __detach__ - Received when node is detached from the screen directly or
somewhere in its ancestry.
##### Methods:
- Inherits all from EventEmitter.
- __prepend(node)__ - Prepend a node to this node's children.
- __append(node)__ - Append a node to this node's children.
- __remove(node)__ - Remove child node from node.
- __insert(node, i)__ - Insert a node to this node's children at index `i`.
- __insertBefore(node, refNode)__ - Insert a node to this node's children
before the reference node.
- __insertAfter(node, refNode)__ - Insert a node from node after the reference
node.
- __detach()__ - Remove node from its parent.
- __emitDescendants(type, args..., [iterator])__ - Emit event for element, and
recursively emit same event for all descendants.
- __get(name, [default])__ - Get user property with a potential default value.
- __set(name, value)__ - Set user property to value.
#### Screen (from Node)
The screen on which every other node renders.
##### Options:
- __program__ - The blessed `Program` to be associated with. Will be
automatically instantiated if none is provided.
- __smartCSR__ - Attempt to perform CSR optimization on all possible elements
(not just full-width ones, elements with uniform cells to their sides).
This is known to cause flickering with elements that are not full-width,