<?php
/**
* Zend Framework
*
* LICENSE
*
* This source file is subject to the new BSD license that is bundled
* with this package in the file LICENSE.txt.
* It is also available through the world-wide-web at this URL:
* http://framework.zend.com/license/new-bsd
* If you did not receive a copy of the license and are unable to
* obtain it through the world-wide-web, please send an email
* to license@zend.com so we can send you a copy immediately.
*
* @category Zend
* @package Zend_Date
* @copyright Copyright (c) 2005-2009 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
* @version $Id: Date.php 2504 2011-12-28 07:35:29Z liu21st $
*/
/**
* Include needed Date classes
*/
require_once 'Zend/Date/DateObject.php';
require_once 'Zend/Locale.php';
require_once 'Zend/Locale/Format.php';
require_once 'Zend/Locale/Math.php';
/**
* @category Zend
* @package Zend_Date
* @copyright Copyright (c) 2005-2009 Zend Technologies USA Inc. (http://www.zend.com)
* @license http://framework.zend.com/license/new-bsd New BSD License
*/
class Zend_Date extends Zend_Date_DateObject
{
private $_locale = null;
// Fractional second variables
private $_fractional = 0;
private $_precision = 3;
private static $_options = array(
'format_type' => 'iso', // format for date strings 'iso' or 'php'
'fix_dst' => true, // fix dst on summer/winter time change
'extend_month' => false, // false - addMonth like SQL, true like excel
'cache' => null, // cache to set
'timesync' => null // timesync server to set
);
// Class wide Date Constants
const DAY = 'dd';
const DAY_SHORT = 'd';
const DAY_SUFFIX = 'SS';
const DAY_OF_YEAR = 'D';
const WEEKDAY = 'EEEE';
const WEEKDAY_SHORT = 'EEE';
const WEEKDAY_NARROW = 'E';
const WEEKDAY_NAME = 'EE';
const WEEKDAY_8601 = 'eee';
const WEEKDAY_DIGIT = 'e';
const WEEK = 'ww';
const MONTH = 'MM';
const MONTH_SHORT = 'M';
const MONTH_DAYS = 'ddd';
const MONTH_NAME = 'MMMM';
const MONTH_NAME_SHORT = 'MMM';
const MONTH_NAME_NARROW = 'MMMMM';
const YEAR = 'y';
const YEAR_SHORT = 'yy';
const YEAR_8601 = 'Y';
const YEAR_SHORT_8601 = 'YY';
const LEAPYEAR = 'l';
const MERIDIEM = 'a';
const SWATCH = 'B';
const HOUR = 'HH';
const HOUR_SHORT = 'H';
const HOUR_AM = 'hh';
const HOUR_SHORT_AM = 'h';
const MINUTE = 'mm';
const MINUTE_SHORT = 'm';
const SECOND = 'ss';
const SECOND_SHORT = 's';
const MILLISECOND = 'S';
const TIMEZONE_NAME = 'zzzz';
const DAYLIGHT = 'I';
const GMT_DIFF = 'Z';
const GMT_DIFF_SEP = 'ZZZZ';
const TIMEZONE = 'z';
const TIMEZONE_SECS = 'X';
const ISO_8601 = 'c';
const RFC_2822 = 'r';
const TIMESTAMP = 'U';
const ERA = 'G';
const ERA_NAME = 'GGGG';
const ERA_NARROW = 'GGGGG';
const DATES = 'F';
const DATE_FULL = 'FFFFF';
const DATE_LONG = 'FFFF';
const DATE_MEDIUM = 'FFF';
const DATE_SHORT = 'FF';
const TIMES = 'WW';
const TIME_FULL = 'TTTTT';
const TIME_LONG = 'TTTT';
const TIME_MEDIUM = 'TTT';
const TIME_SHORT = 'TT';
const DATETIME = 'K';
const DATETIME_FULL = 'KKKKK';
const DATETIME_LONG = 'KKKK';
const DATETIME_MEDIUM = 'KKK';
const DATETIME_SHORT = 'KK';
const ATOM = 'OOO';
const COOKIE = 'CCC';
const RFC_822 = 'R';
const RFC_850 = 'RR';
const RFC_1036 = 'RRR';
const RFC_1123 = 'RRRR';
const RFC_3339 = 'RRRRR';
const RSS = 'SSS';
const W3C = 'WWW';
/**
* Generates the standard date object, could be a unix timestamp, localized date,
* string, integer, array and so on. Also parts of dates or time are supported
* Always set the default timezone: http://php.net/date_default_timezone_set
* For example, in your bootstrap: date_default_timezone_set('America/Los_Angeles');
* For detailed instructions please look in the docu.
*
* @param string|integer|Zend_Date|array $date OPTIONAL Date value or value of date part to set
* ,depending on $part. If null the actual time is set
* @param string $part OPTIONAL Defines the input format of $date
* @param string|Zend_Locale $locale OPTIONAL Locale for parsing input
* @return Zend_Date
* @throws Zend_Date_Exception
*/
public function __construct($date = null, $part = null, $locale = null)
{
if (is_object($date) and !($date instanceof Zend_TimeSync_Protocol) and
!($date instanceof Zend_Date)) {
if ($locale instanceof Zend_Locale) {
$locale = $date;
$date = null;
$part = null;
} else {
$date = (string) $date;
}
}
if (($date !== null) and !is_array($date) and !($date instanceof Zend_TimeSync_Protocol) and
!($date instanceof Zend_Date) and !defined($date) and Zend_Locale::isLocale($date, true, false)) {
$locale = $date;
$date = null;
$part = null;
} else if (($part !== null) and !defined($part) and Zend_Locale::isLocale($part, true, false)) {
$locale = $part;
$part = null;
}
$this->setLocale($locale);
if (is_string($date) && ($part === null) && (strlen($date) <= 5)) {
$part = $date;
$date = null;
}
if ($date === null) {
if ($part === null) {
$date = time();
} else if ($part !== self::TIMESTAMP) {
$date = self::now($locale);
$date = $date->get($part);
}
}
if ($date instanceof Zend_TimeSync_Protocol) {
$date = $date->getInfo();
$date = $this->_getTime($date['offset']);
$part = null;
} else if (parent::$_defaultOffset != 0) {
$date = $this->_getTime(parent::$_defaultOffset);
}
// set the timezone and offset for $this
$zone = @date_default_timezone_get();
$this->setTimezone($zone);
// try to get timezone from date-string
if (!is_int($date)) {
$zone = $this->getTimezoneFromString($date);
$this->setTimezone($zone);
}
// set datepart
if (($part !== null && $part !== self::TIMESTAMP) or (!is_numeric($date))) {
// switch off dst handling for value setting
$this->setUnixTimestamp($this->getGmtOffset());
$this->set($date, $part, $this->_locale);
// DST fix
if (is_array($date) === true) {
if (!isset($date['hour'])) {
$date['hour'] = 0;
}
$hour = $this->toString('H');
$hour = $date['hour'] - $hour;
switch ($hour) {
case 1 :
case -23 :
$this->addTimestamp(3600);
没有合适的资源?快使用搜索试试~ 我知道了~
(仅供学习)黄金农场游戏源码开源源码统H5农场复利源码带商城仓库商店模块农场复利理财系统
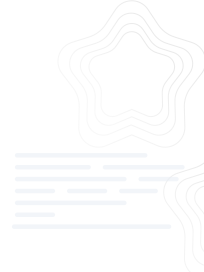
共2000个文件
png:856个
php:563个
html:253个


温馨提示
(仅供学习)开源源码统H5农场复利源码带商城仓库商店模块农场复利理财系统 淘金农场源码游戏攻略,包括果实、土地、道具、神像、宠物、工坊、训练场等介绍 黄金家园游戏将创造一个新的游戏体制,主要是以农场土地种植为背景的模拟经营游戏。玩家扮演一个农场的经营者完成从购买种子到耕种、浇水、施肥、除草、收获果实,再到出售给市场的整个过程。游戏模拟了作物的成长过程,所以玩家在经营农场的同时,可以感受“作物养成”带来的乐趣。游戏中,玩家可以可对房屋、土地等进行升级,获得更加强大、高效的农场
资源推荐
资源详情
资源评论
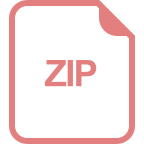
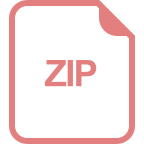
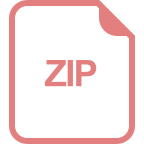
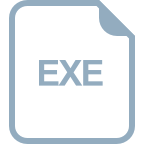
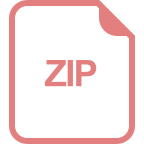
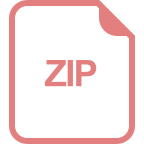
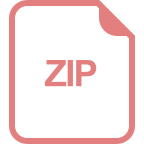
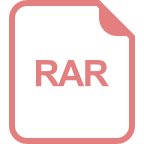
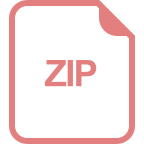
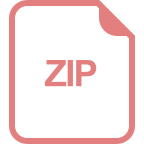
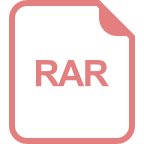
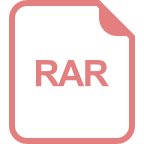
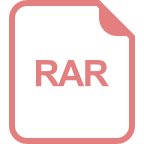
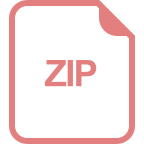
收起资源包目录

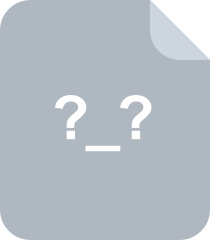
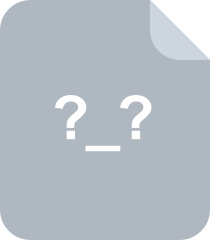
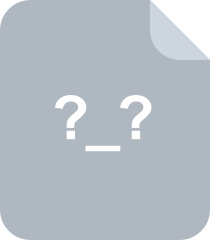
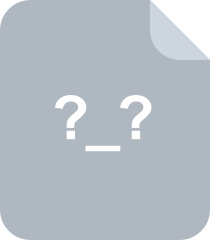
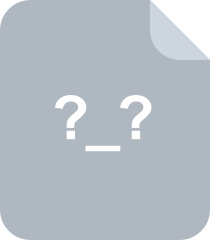
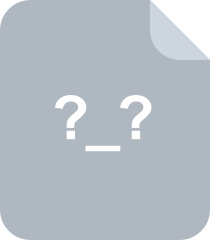
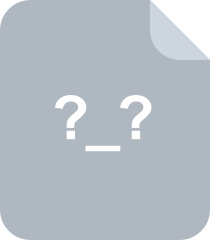
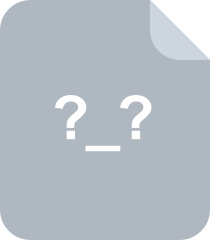
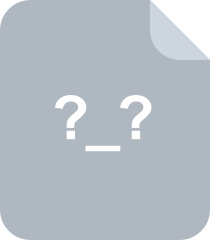
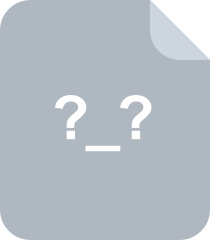
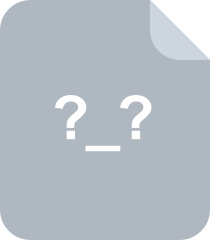
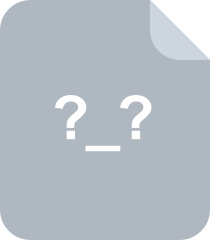
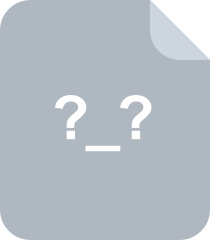
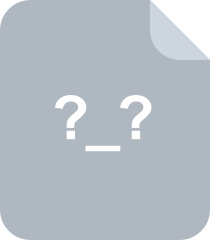
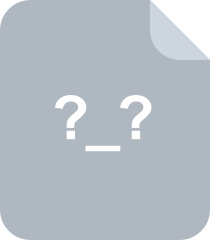
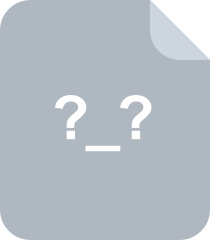
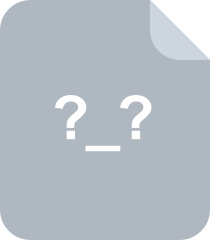
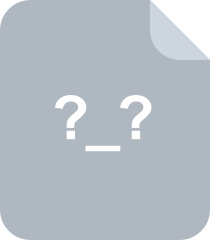
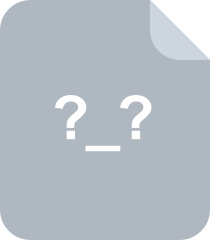
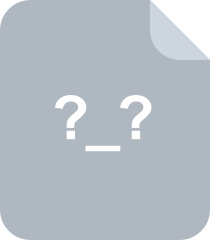
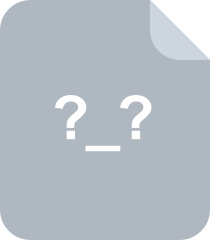
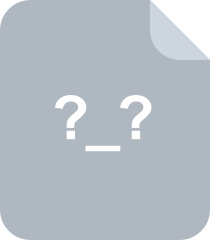
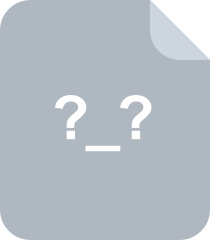
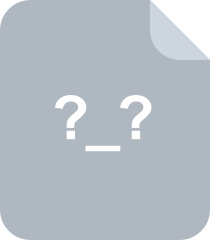
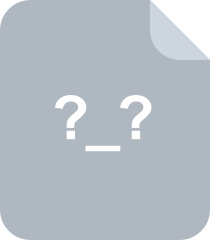
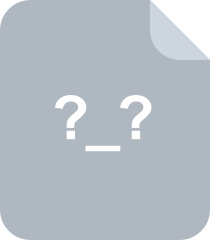
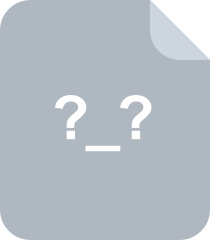
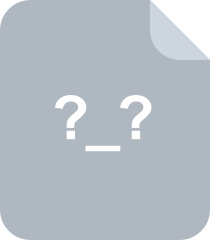
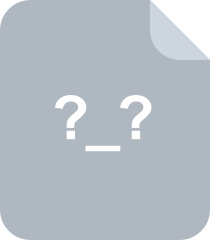
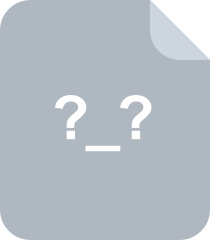
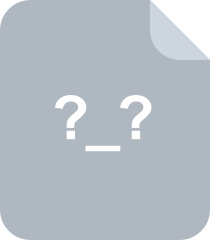
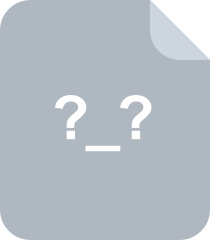
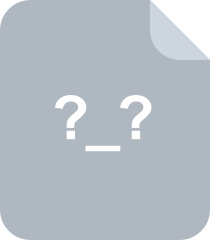
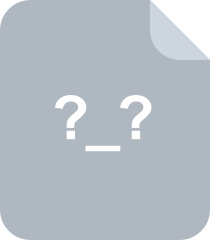
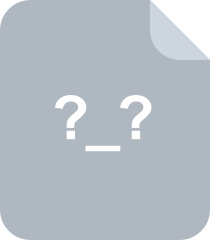
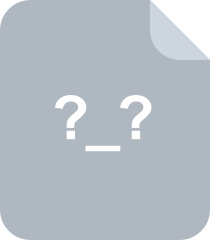
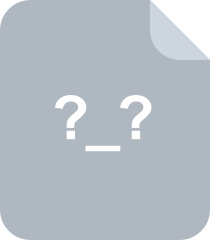
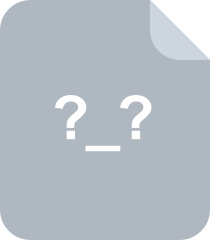
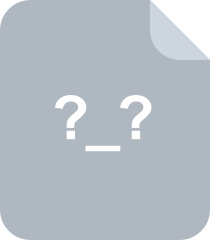
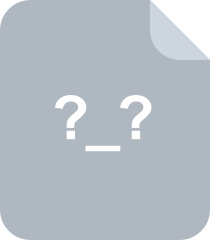
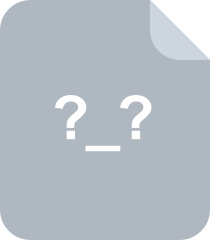
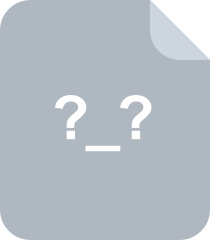
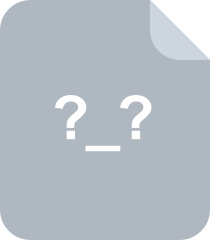
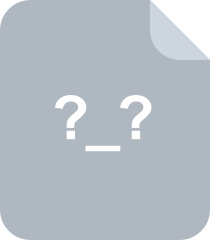
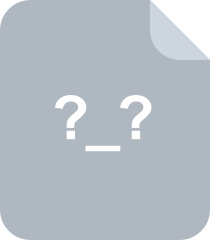
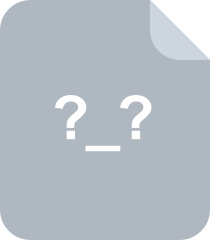
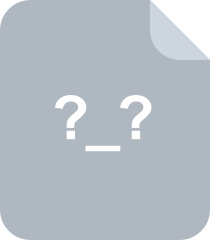
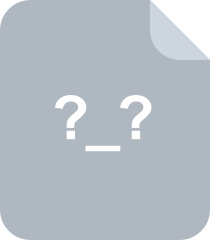
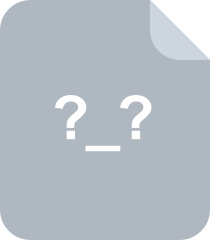
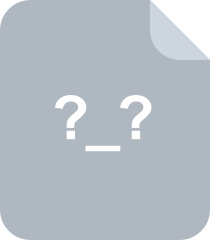
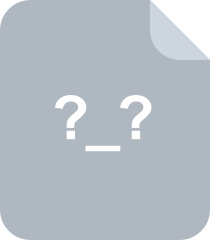
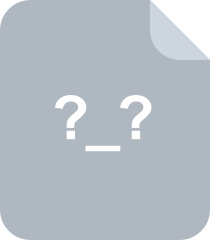
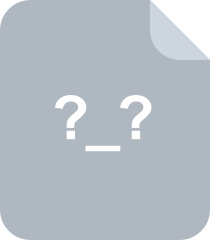
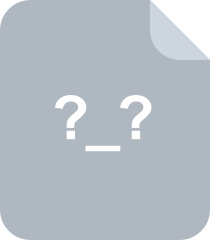
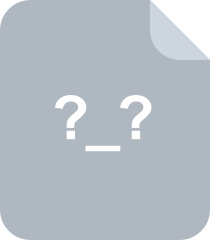
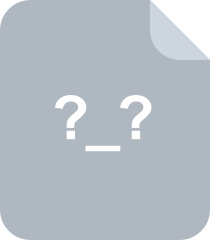
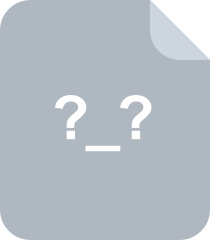
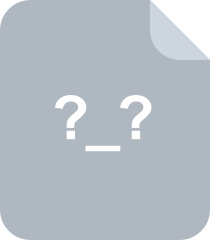
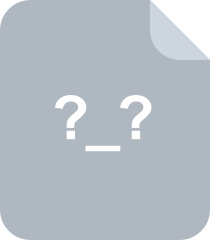
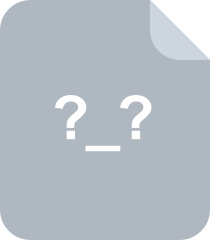
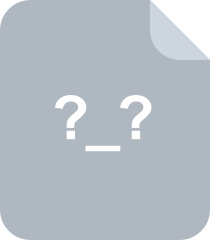
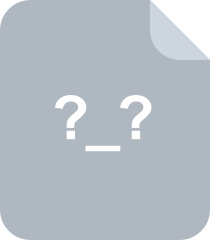
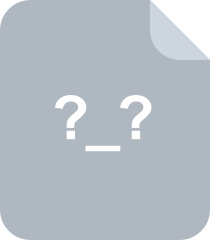
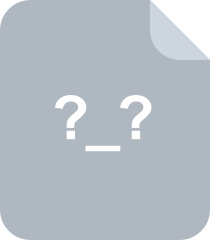
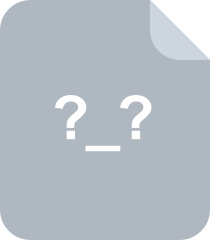
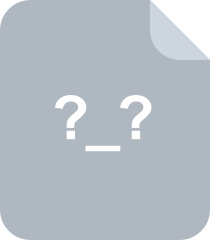
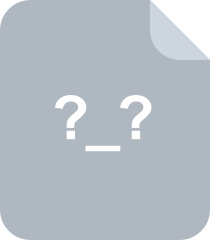
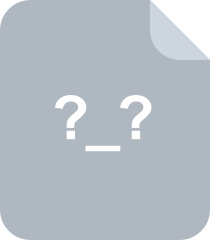
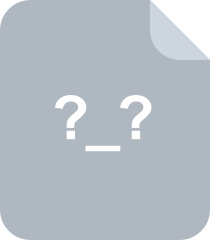
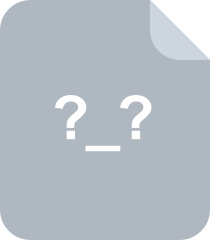
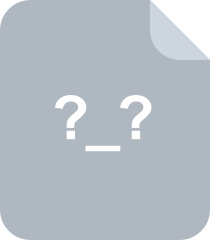
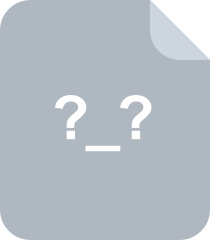
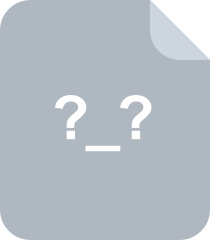
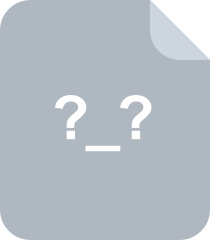
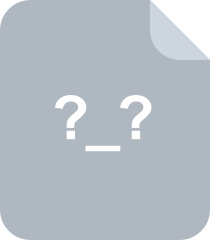
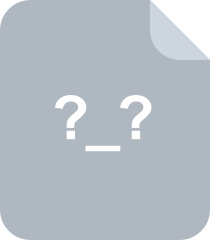
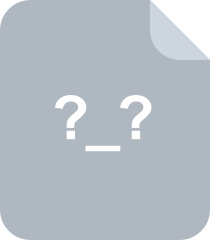
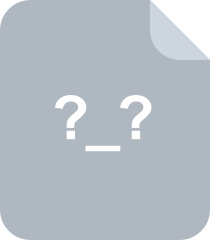
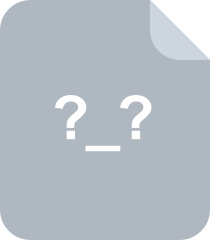
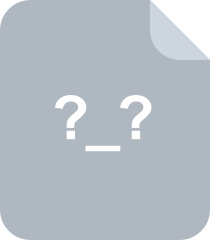
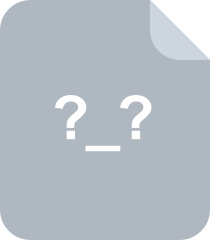
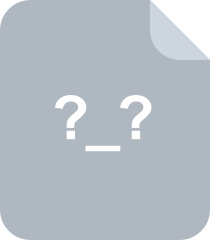
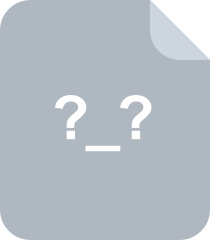
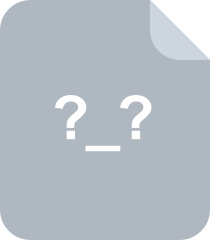
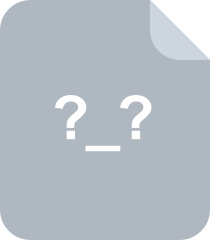
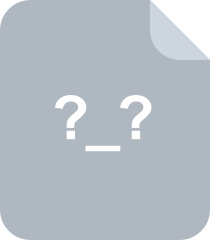
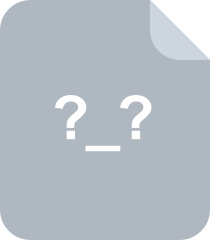
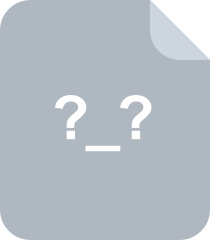
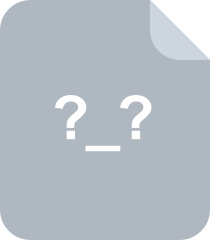
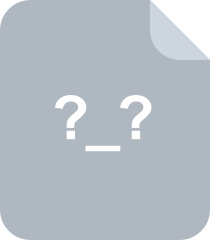
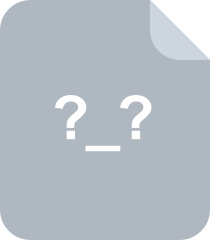
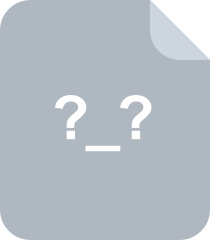
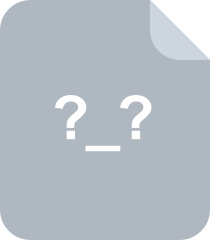
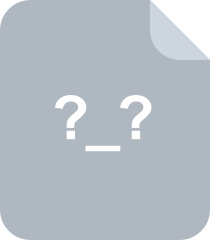
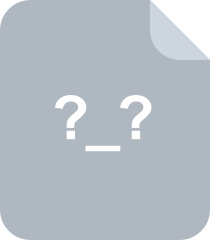
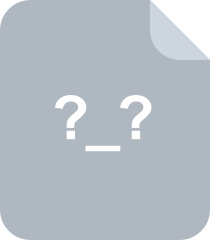
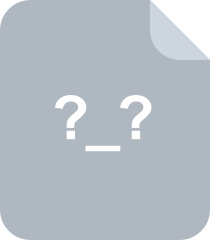
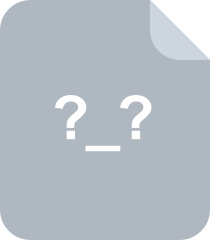
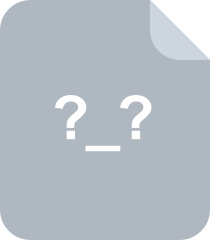
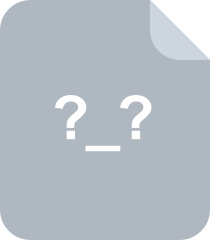
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
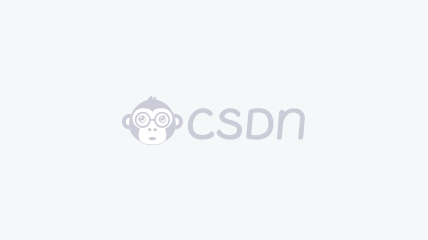
- jdjiadian2019-10-28不完整,BUG很多

现实分享
- 粉丝: 3
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

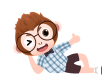
最新资源
- HTML5+CSS3综合案例-智慧教辅
- 打板-抓涨停板工具(炒股辅助表格)-每隔1分钟获取一次网页数据-股票基金
- 软件测试之弱网测试分享文件
- Java实现数据库连接及数据操作教程
- 视频下载工具,v如i果p有,充一次,看多次
- 食物检测54-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 系统集成项目管理工程师备考资料-必背100题
- Swift 6从应用到嵌入式
- 东北大学计算机科学与技术专业四年的课程设计和实验报告.zip
- 高压数字测量系统课程设计 的 报告.zip
- 电子课程设计小车调试代码日志报告仓库.zip
- 河南工业大学计科课程设计及报告.zip
- 航空客运订票系统采用C用链表存储航班信息包含配套开题报告答辩ppt课程设计报告等.zip
- 合肥工业大学信息论与编码课程设计含代码可视化界面课设报告.zip
- 华中科技大学大学CS课程其它报告.zip
- 2021春季学期 操作系统 课程设计报告.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


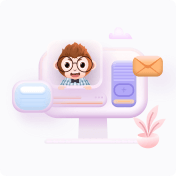
安全验证
文档复制为VIP权益,开通VIP直接复制
