!function(e,t){"object"==typeof exports&&"undefined"!=typeof module?t(exports):"function"==typeof define&&define.amd?define(["exports"],t):t((e="undefined"!=typeof globalThis?globalThis:e||self).Cesium={})}(this,function(exports){"use strict";function defined(e){return null!=e}function DeveloperError(e){var t;this.name="DeveloperError",this.message=e;try{throw new Error}catch(e){t=e.stack}this.stack=t}defined(Object.create)&&(DeveloperError.prototype=Object.create(Error.prototype),DeveloperError.prototype.constructor=DeveloperError),DeveloperError.prototype.toString=function(){var e=this.name+": "+this.message;return defined(this.stack)&&(e+="\n"+this.stack.toString()),e},DeveloperError.throwInstantiationError=function(){throw new DeveloperError("This function defines an interface and should not be called directly.")};var Check={};function getUndefinedErrorMessage(e){return e+" is required, actual value was undefined"}function getFailedTypeErrorMessage(e,t,i){return"Expected "+i+" to be typeof "+t+", actual typeof was "+e}function defaultValue(e,t){return null!=e?e:t}function MersenneTwister(e){null==e&&(e=(new Date).getTime()),this.N=624,this.M=397,this.MATRIX_A=2567483615,this.UPPER_MASK=2147483648,this.LOWER_MASK=2147483647,this.mt=new Array(this.N),this.mti=this.N+1,this.init_genrand(e)}Check.typeOf={},Check.defined=function(e,t){if(!defined(t))throw new DeveloperError(getUndefinedErrorMessage(e))},Check.typeOf.func=function(e,t){if("function"!=typeof t)throw new DeveloperError(getFailedTypeErrorMessage(typeof t,"function",e))},Check.typeOf.string=function(e,t){if("string"!=typeof t)throw new DeveloperError(getFailedTypeErrorMessage(typeof t,"string",e))},Check.typeOf.number=function(e,t){if("number"!=typeof t)throw new DeveloperError(getFailedTypeErrorMessage(typeof t,"number",e))},Check.typeOf.number.lessThan=function(e,t,i){if(Check.typeOf.number(e,t),i<=t)throw new DeveloperError("Expected "+e+" to be less than "+i+", actual value was "+t)},Check.typeOf.number.lessThanOrEquals=function(e,t,i){if(Check.typeOf.number(e,t),i<t)throw new DeveloperError("Expected "+e+" to be less than or equal to "+i+", actual value was "+t)},Check.typeOf.number.greaterThan=function(e,t,i){if(Check.typeOf.number(e,t),t<=i)throw new DeveloperError("Expected "+e+" to be greater than "+i+", actual value was "+t)},Check.typeOf.number.greaterThanOrEquals=function(e,t,i){if(Check.typeOf.number(e,t),t<i)throw new DeveloperError("Expected "+e+" to be greater than or equal to"+i+", actual value was "+t)},Check.typeOf.object=function(e,t){if("object"!=typeof t)throw new DeveloperError(getFailedTypeErrorMessage(typeof t,"object",e))},Check.typeOf.bool=function(e,t){if("boolean"!=typeof t)throw new DeveloperError(getFailedTypeErrorMessage(typeof t,"boolean",e))},Check.typeOf.number.equals=function(e,t,i,r){if(Check.typeOf.number(e,i),Check.typeOf.number(t,r),i!==r)throw new DeveloperError(e+" must be equal to "+t+", the actual values are "+i+" and "+r)},defaultValue.EMPTY_OBJECT=Object.freeze({}),MersenneTwister.prototype.init_genrand=function(e){for(this.mt[0]=e>>>0,this.mti=1;this.mti<this.N;this.mti++){e=this.mt[this.mti-1]^this.mt[this.mti-1]>>>30;this.mt[this.mti]=(1812433253*((4294901760&e)>>>16)<<16)+1812433253*(65535&e)+this.mti,this.mt[this.mti]>>>=0}},MersenneTwister.prototype.genrand_int32=function(){var e,t,i=new Array(0,this.MATRIX_A);if(this.mti>=this.N){for(this.mti==this.N+1&&this.init_genrand(5489),t=0;t<this.N-this.M;t++)e=this.mt[t]&this.UPPER_MASK|this.mt[t+1]&this.LOWER_MASK,this.mt[t]=this.mt[t+this.M]^e>>>1^i[1&e];for(;t<this.N-1;t++)e=this.mt[t]&this.UPPER_MASK|this.mt[t+1]&this.LOWER_MASK,this.mt[t]=this.mt[t+(this.M-this.N)]^e>>>1^i[1&e];e=this.mt[this.N-1]&this.UPPER_MASK|this.mt[0]&this.LOWER_MASK,this.mt[this.N-1]=this.mt[this.M-1]^e>>>1^i[1&e],this.mti=0}return e=this.mt[this.mti++],e^=e>>>11,e^=e<<7&2636928640,e^=e<<15&4022730752,(e^=e>>>18)>>>0},MersenneTwister.prototype.random=function(){return this.genrand_int32()*(1/4294967296)};var CesiumMath={EPSILON1:.1,EPSILON2:.01,EPSILON3:.001,EPSILON4:1e-4,EPSILON5:1e-5,EPSILON6:1e-6,EPSILON7:1e-7,EPSILON8:1e-8,EPSILON9:1e-9,EPSILON10:1e-10,EPSILON11:1e-11,EPSILON12:1e-12,EPSILON13:1e-13,EPSILON14:1e-14,EPSILON15:1e-15,EPSILON16:1e-16,EPSILON17:1e-17,EPSILON18:1e-18,EPSILON19:1e-19,EPSILON20:1e-20,EPSILON21:1e-21,GRAVITATIONALPARAMETER:3986004418e5,SOLAR_RADIUS:6955e5,LUNAR_RADIUS:1737400,SIXTY_FOUR_KILOBYTES:65536,FOUR_GIGABYTES:4294967296};CesiumMath.sign=defaultValue(Math.sign,function(e){return 0===(e=+e)||e!=e?e:0<e?1:-1}),CesiumMath.signNotZero=function(e){return e<0?-1:1},CesiumMath.toSNorm=function(e,t){return t=defaultValue(t,255),Math.round((.5*CesiumMath.clamp(e,-1,1)+.5)*t)},CesiumMath.fromSNorm=function(e,t){return t=defaultValue(t,255),CesiumMath.clamp(e,0,t)/t*2-1},CesiumMath.normalize=function(e,t,i){return 0===(i=Math.max(i-t,0))?0:CesiumMath.clamp((e-t)/i,0,1)},CesiumMath.sinh=defaultValue(Math.sinh,function(e){return(Math.exp(e)-Math.exp(-e))/2}),CesiumMath.cosh=defaultValue(Math.cosh,function(e){return(Math.exp(e)+Math.exp(-e))/2}),CesiumMath.lerp=function(e,t,i){return(1-i)*e+i*t},CesiumMath.PI=Math.PI,CesiumMath.ONE_OVER_PI=1/Math.PI,CesiumMath.PI_OVER_TWO=Math.PI/2,CesiumMath.PI_OVER_THREE=Math.PI/3,CesiumMath.PI_OVER_FOUR=Math.PI/4,CesiumMath.PI_OVER_SIX=Math.PI/6,CesiumMath.THREE_PI_OVER_TWO=3*Math.PI/2,CesiumMath.TWO_PI=2*Math.PI,CesiumMath.ONE_OVER_TWO_PI=1/(2*Math.PI),CesiumMath.RADIANS_PER_DEGREE=Math.PI/180,CesiumMath.DEGREES_PER_RADIAN=180/Math.PI,CesiumMath.RADIANS_PER_ARCSECOND=CesiumMath.RADIANS_PER_DEGREE/3600,CesiumMath.toRadians=function(e){return e*CesiumMath.RADIANS_PER_DEGREE},CesiumMath.toDegrees=function(e){return e*CesiumMath.DEGREES_PER_RADIAN},CesiumMath.convertLongitudeRange=function(e){var t=CesiumMath.TWO_PI,e=e-Math.floor(e/t)*t;return e<-Math.PI?e+t:e>=Math.PI?e-t:e},CesiumMath.clampToLatitudeRange=function(e){return CesiumMath.clamp(e,-1*CesiumMath.PI_OVER_TWO,CesiumMath.PI_OVER_TWO)},CesiumMath.negativePiToPi=function(e){return e>=-CesiumMath.PI&&e<=CesiumMath.PI?e:CesiumMath.zeroToTwoPi(e+CesiumMath.PI)-CesiumMath.PI},CesiumMath.zeroToTwoPi=function(e){if(0<=e&&e<=CesiumMath.TWO_PI)return e;var t=CesiumMath.mod(e,CesiumMath.TWO_PI);return Math.abs(t)<CesiumMath.EPSILON14&&Math.abs(e)>CesiumMath.EPSILON14?CesiumMath.TWO_PI:t},CesiumMath.mod=function(e,t){return CesiumMath.sign(e)===CesiumMath.sign(t)&&Math.abs(e)<Math.abs(t)?e:(e%t+t)%t},CesiumMath.equalsEpsilon=function(e,t,i,r){i=defaultValue(i,0),r=defaultValue(r,i);var n=Math.abs(e-t);return n<=r||n<=i*Math.max(Math.abs(e),Math.abs(t))},CesiumMath.lessThan=function(e,t,i){return e-t<-i},CesiumMath.lessThanOrEquals=function(e,t,i){return e-t<i},CesiumMath.greaterThan=function(e,t,i){return i<e-t},CesiumMath.greaterThanOrEquals=function(e,t,i){return-i<e-t};var factorials=[1];CesiumMath.factorial=function(e){var t=factorials.length;if(t<=e)for(var i=factorials[t-1],r=t;r<=e;r++){var n=i*r;factorials.push(n),i=n}return factorials[e]},CesiumMath.incrementWrap=function(e,t,i){return i=defaultValue(i,0),e=t<++e?i:e},CesiumMath.isPowerOfTwo=function(e){return 0!==e&&0==(e&e-1)},CesiumMath.nextPowerOfTwo=function(e){return--e,e|=e>>1,e|=e>>2,e|=e>>4,e|=e>>8,e|=e>>16,++e},CesiumMath.previousPowerOfTwo=function(e){return e|=e>>1,e|=e>>2,e|=e>>4,e|=e>>8,e|=e>>16,e=((e|=e>>32)>>>0)-(e>>>1)},CesiumMath.clamp=function(e,t,i){return e<t?t:i<e?i:e};var randomNumberGenerator=new MersenneTwister;function Cartesian3(e,t,i){this.x=defaultValue(e,0),this.y=defaultValue(t,0),this.z=defaultValue(i,0)}CesiumMath.setRandomNumberSeed=function(e){randomNumberGenerator=new MersenneTwister(e)},CesiumMath.nextRandomNumber=function(){return randomNumberGenerator.random()},CesiumMath.randomBetween=function(e,t){return CesiumMath.nextRandomNumber()*(t-e)+e},CesiumMath.acosClamped=function(e){return Math.acos(CesiumMath.clamp(e,-1,1))},CesiumMath.asinClamped=function(e){return Math.asin(CesiumMath.clamp(e,-1,1))},CesiumMath.chor


十小大
- 粉丝: 1w+
- 资源: 1529
最新资源
- 【创新无忧】基于雾凇优化算法RIME优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现光伏预测附matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


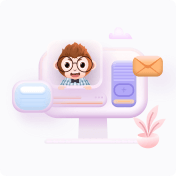