# KakaJSON
[](https://github.com/CocoaPods/CocoaPods) [](https://github.com/Carthage/Carthage) [](https://swift.org/package-manager/)
> Fast conversion between JSON and model in Swift.
- Convert model to JSON with one line of code.(一行代码Model转JSON)
- Convert JSON to Model with one line of code.(一行代码JSON转Model)
- Archive\Unarchive object with one line of code.(一行代码实现常见数据的归档\解档)
## 中文教程
- [KakaJSON手册](https://www.cnblogs.com/mjios/p/11352776.html)
## Integration
### CocoaPods
```ruby
pod 'KakaJSON', '~> 1.1.2'
```
### Carthage
```ruby
github "kakaopensource/KakaJSON" ~> 1.1.2
```
### Swift Package Manager
To use Swift Package Manager, you should update to Xcode 11.
* Open your project.
* Click File tab
* Select Swift Packages
* Add Package Dependency, enter [KakaJSON repo's URL](https://github.com/kakaopensource/KakaJSON.git)
Or you can login Xcode with your GitHub account. just search **KakaJSON**.
## Usages
- [Coding](#coding)
- [JSON To Model_01_Basic Usage](#json-to-model_01_basic-usage)
- [Simple Model](#simple-model)
- [Class Type](#class-type)
- [Inheritance](#inheritance)
- [let](#let)
- [NSNull](#nsnull)
- [JSONString](#jsonstring)
- [JSONData](#jsondata)
- [Nested Model 1](#nested-model-1)
- [Nested Model 2](#nested-model-2)
- [Nested Model 3](#nested-model-3)
- [Recursive](#recursive)
- [Generic](#generic)
- [Model Array](#model-array)
- [Model Array In Dictionary](#model-array-in-dictionary)
- [Convert](#convert)
- [Listen](#listen)
- [JSON To Model_02_Data Type](#json-to-model_02_data-type)
- [Int](#int)
- [Float](#float)
- [Double](#double)
- [CGFloat](#cgfloat)
- [Bool](#bool)
- [String](#string)
- [Decimal](#decimal)
- [NSDecimalNumber](#nsdecimalnumber)
- [NSNumber](#nsnumber)
- [Optional](#optional)
- [URL](#url)
- [Data](#data)
- [Date](#date)
- [Enum](#enum)
- [Enum In Array](#enum-in-array)
- [Enum In Dictionary](#enum-in-dictionary)
- [Enum Array In Dictionary](#enum-array-in-dictionary)
- [Array](#array)
- [Set](#set)
- [Dictionary](#dictionary)
- [JSON To Model_03_Key Mapping](#json-to-model_03_key-mapping)
- [Basic Usage](#basic-usage)
- [Camel -> Underline](#camel---underline)
- [Underline -> Camel](#underline---camel)
- [Inheritance](#inheritance-1)
- [Override 1](#override-1)
- [Override 2](#override-2)
- [Global Config](#global-config)
- [Local Config](#local-config)
- [Config Example 1](#config-example-1)
- [Config Example 2](#config-example-2)
- [Complex](#complex)
- [JSON To Model_04_Custom Value](#json-to-model_04_custom-value)
- [Date](#date-1)
- [Unspecific Type](#unspecific-type)
- [Example](#example)
- [Other Ways](#other-ways)
- [JSON To Model_05_Dynamic Model](#json-to-model_05_dynamic-model)
- [Model To JSON](#model-to-json)
- [JSON and JSONString](#json-and-jsonstring)
- [Optional](#optional-1)
- [Enum](#enum-1)
- [Nested Model](#nested-model)
- [Any](#any)
- [Model Array](#model-array-1)
- [Model Set](#model-set)
- [Key Mapping](#key-mapping-1)
- [Custom Value](#custom-value-1)
- [Listen](#listen)
## Coding
```swift
// file path (can be String or URL)
let file = "/Users/mj/Desktop/test.data"
/****************** String ******************/
let string1 = "123"
// wrtite String to file
write(string1, to: file)
// read String from file
let string2 = read(String.self, from: file)
XCTAssert(string2 == string1)
// read Int from file
XCTAssert(read(Int.self, from: file) == 123)
/****************** Date ******************/
let date1 = Date(timeIntervalSince1970: 1565922866)
// wrtite Date to file
write(date1, to: file)
// read Date from file
let date2 = read(Date.self, from: file)
XCTAssert(date2 == date1)
// read Int from file
XCTAssert(read(Int.self, from: file) == 1565922866)
/****************** Array ******************/
let array1 = ["Jack", "Rose"]
// wrtite [String] to file
write(array1, to: file)
// read [String] from file
let array2 = read([String].self, from: file)
XCTAssert(array2 == array1)
// Also support Set\Dictionary
/****************** Model ******************/
struct Book: Convertible {
var name: String = ""
var price: Double = 0.0
}
struct Car: Convertible {
var name: String = ""
var price: Double = 0.0
}
struct Dog: Convertible {
var name: String = ""
var age: Int = 0
}
struct Person: Convertible {
var name: String = "Jack"
var car: Car? = Car(name: "Bently", price: 106.666)
var books: [Book]? = [
Book(name: "Fast C++", price: 666.6),
Book(name: "Data Structure And Algorithm", price: 666.6),
]
var dogs: [String: Dog]? = [
"dog0": Dog(name: "Wang", age: 5),
"dog1": Dog(name: "ErHa", age: 3),
]
}
// wrtite Person to file
write(Person(), to: file)
// read Person from file
let person = read(Person.self, from: file)
XCTAssert(person?.name == "Jack")
XCTAssert(person?.car?.name == "Bently")
XCTAssert(person?.car?.price == 106.666)
XCTAssert(person?.books?.count == 2)
XCTAssert(person?.dogs?.count == 2)
/****************** Model Array ******************/
struct Car: Convertible {
var name: String = ""
var price: Double = 0.0
}
let models1 = [
Car(name: "BMW", price: 100.0),
Car(name: "Audi", price: 70.0)
]
// wrtite [Car] to file
write(models1, to: file)
// read [Car] from file
let models2 = read([Car].self, from: file)
XCTAssert(models2?.count == models1.count)
XCTAssert(models2?[0].name == "BMW")
XCTAssert(models2?[0].price == 100.0)
XCTAssert(models2?[1].name == "Audi")
XCTAssert(models2?[1].price == 70.0)
/****************** Model Set ******************/
struct Car: Convertible, Hashable {
var name: String = ""
var price: Double = 0.0
}
let models1: Set<Car> = [
Car(name: "BMW", price: 100.0),
Car(name: "Audi", price: 70.0)
]
// wrtite Set<Car> to file
write(models1, to: file)
// read Set<Car> from file
let models2 = read(Set<Car>.self, from: file)!
XCTAssert(models2.count == models1.count)
for car in models2 {
XCTAssert(["BMW", "Audi"].contains(car.name))
XCTAssert([100.0, 70.0].contains(car.price))
}
/****************** Model Dictionary ******************/
struct Car: Convertible {
var name: String = ""
var price: Double = 0.0
}
let models1 = [
"car0": Car(name: "BMW", price: 100.0),
"car1": Car(name: "Audi", price: 70.0)
]
// wrtite [String: Car] to file
write(models1, to: file)
// read [String: Car] from file
let models2 = read([String: Car].self, from: file)
XCTAssert(models2?.count == models1.count)
let car0 = models2?["car0"]
XCTAssert(car0?.name == "BMW")
XCTAssert(car0?.price == 100.0)
let car1 = models2?["car1"]
XCTAssert(car1?.name == "Audi")
XCTAssert(car1?.price == 70.0)
```
## JSON To Model_01_Basic Usage
### Simple Model
```swift
struct Cat: Convertible {
var name: String = ""
var weight: Double = 0.0
}
// json can also be NSDictionary, NSMutableDictionary
let json: [String: Any] = [
"name": "Miaomiao",
"weight": 6.66
]
let cat1 = json.kj.model(Cat.self)
XCTAssert(cat1.name == "Miaomiao")
XCTAssert(cat1.weight == 6.66)
// you can call global function `model`
let cat2 = model(from: json, Cat.self)
// support type variable
var type: Convertible.Type = Cat.self
let cat3 = json.kj.model(type: type) as? Cat
let cat4 = model(from: json, type: type) as? Cat
```
### Class Type
```swift
class Cat: Convertible {
var weight: Double = 0.0
var name: String = ""
// The protocol `Convertible` required an init constructor
// for initializing an instance completely.
required init() {}
}
let json = ...
let cat = json.kj.model(Cat.self)
// a class inh


十小大
- 粉丝: 1w+
- 资源: 1529
最新资源
- python项目,Python爬虫教学的项目,包括:Python基础,爬虫原理和网页构造,我们的第一个爬虫,正则表达式,多进程爬虫等
- 基于MATLAB Simulink的双馈风机机侧与网侧协同控制策略研究,【探究MATLAB Simulink中双馈风机控制系统的设计与实现】:深入理解机侧与网侧控制的重要性与运用,dfig0522
- 两相交错并联同步整流双向Buck Boost变换器仿真研究:实现ZVs软开关与开关频率分析,两相交错并联同步整流双向Buck Boost变换器仿真研究:开关管ZVs软开关技术实现及性能分析,两相交错并
- python资源,Python项目资源: 基础入门、数据分析、爬虫实践,包括:Python编程[从入门到实战],Python数据分析,Python网络爬虫[从入门到实战]等
- 结构光CUDA多线程编程在相位单目双目结构光三维重建系统中的应用:高效0.5秒500万像素三维重建技术,结构光CUDA多线程编程在相位单目双目结构光三维重建系统中的应用:高速500万像素三维重建技术
- python,python同花顺资源,包括:将文本转换为列表的程序,统计一周的每一天的打板溢价率,股票数据格式等
- 2501.DeepSeek-R1.pdf
- DeepSeek 爆火详细报告.pptx
- 2025年DeepSeek 保姆级教程:含7大场景+50大案例+全套提示词 从入门到精通.docx
- Deepseek-R1开源推动AI应用发展,头部AI厂支持Deepseek.pdf
- BSOO_ 二元恒星振荡优化器附Matlab代码.rar
- - 一种用于获取非线性系统(定义为ODE)的基本分叉分析类型的工具.rar
- 智能手表电磁辐射SAR仿真与测试:基于FDTD和HFSS的Python/Matlab实现及其误差分析(含代码及详细解释)
- 【信道估计】MIMO-OFDM系统的自适应信道SVD估计python代码.rar
- FM-CW雷达系统的设计方法simulink.rar
- GPS导航Matlab工具箱.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


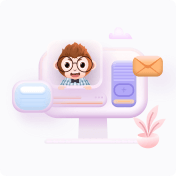