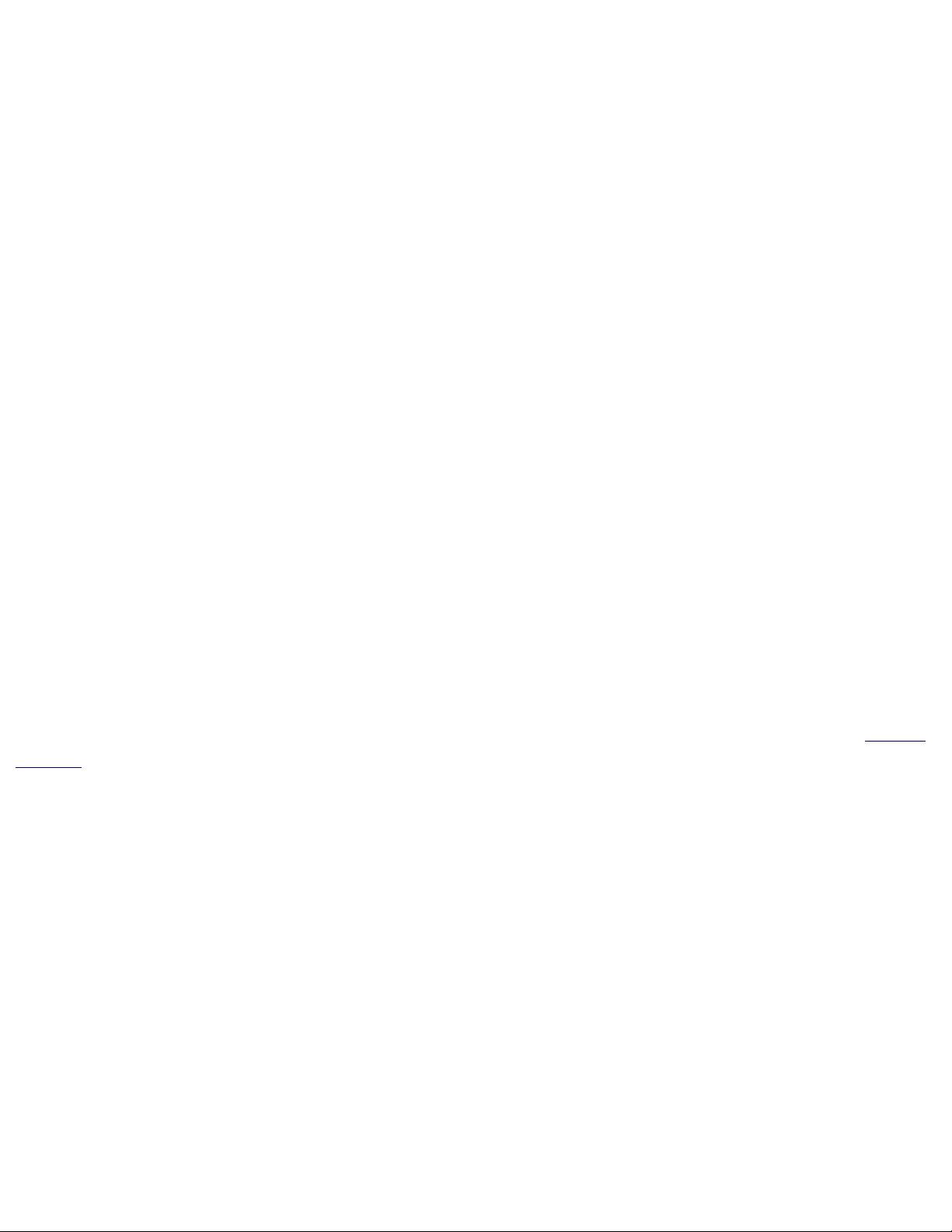
Programming Assignment 3: Implementing a Reliable Transport Protocol
Programming Assignment 5: Implementing a Reliable
Transport Protocol
Overview
In this laboratory programming assignment, you will be writing the sending and receiving transport-level
code for implementing a simple reliable data transfer protocol. There are two versions of this lab, the
Alternating-Bit-Protocol version and the Go-Back-N version. This lab should be fun since your
implementation will differ very little from what would be required in a real-world situation.
Since you probably don't have standalone machines (with an OS that you can modify), your code will have
to execute in a simulated hardware/software environment. However, the programming interface provided
to your routines, i.e., the code that would call your entities from above and from below is very close to
what is done in an actual UNIX environment. (Indeed, the software interfaces described in this
programming assignment are much more realistic that the infinite loop senders and receivers that many
texts describe). Stopping/starting of timers are also simulated, and timer interrupts will cause your timer
handling routine to be activated.
The routines you will write
The procedures you will write are for the sending entity (A) and the receiving entity (B). Only unidirectional
transfer of data (from A to B) is required. Of course, the B side will have to send packets to A to
acknowledge (positively or negatively) receipt of data. Your routines are to be implemented in the form of
the procedures described below. These procedures will be called by (and will call) procedures that I have
written which emulate a network environment. The overall structure of the environment is shown in Figure
Lab.3-1 (structure of the emulated environment):
The unit of data passed between the upper layers and your protocols is a message, which is declared as:
struct msg {
char data[20];
};
This declaration, and all other data structure and emulator routines, as well as stub routines (i.e., those
you are to complete) are in the file, prog2.c, described later. Your sending entity will thus receive data in
20-byte chunks from layer5; your receiving entity should deliver 20-byte chunks of correctly received data
to layer5 at the receiving side.
The unit of data passed between your routines and the network layer is the packet, which is declared as:
struct pkt {
http://media.pearsoncmg.com/aw/aw_kurose_network_3/labs/lab5/lab5.html (1 of 6)2006-12-3 6:35:33