(完整版)python常用函数.docx
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
### Python 常用函数详解 #### 一、`map()` 函数 `map()` 是 Python 内置的一个高阶函数,它接受一个函数 `f` 和一个列表,并通过将函数 `f` 依次作用于列表中的每一个元素,从而得到一个新的列表并返回。 **示例**: 假设有一个列表 `[1, 2, 3, 4, 5, 6, 7, 8, 9]`,我们希望将列表中的每个元素平方,可以通过 `map()` 函数轻松实现: ```python numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9] # 定义一个函数来计算平方 def square(x): return x * x # 使用 map() 函数应用到列表 squared_numbers = list(map(square, numbers)) print(squared_numbers) # 输出: [1, 4, 9, 16, 25, 36, 49, 64, 81] ``` **注意**: - `map()` 函数不会改变原有的列表,而是返回一个新的列表。 - 可以通过 `list()` 来转换 `map` 对象为列表。 - `map()` 不仅仅适用于数值列表,对于任意类型的列表,只要传入的函数能够处理该数据类型,`map()` 都能正常工作。 **示例**: 假设我们需要格式化一个名字列表,使得每个名字的首字母大写,其余部分小写: ```python names = ['adam', 'LISA', 'barT'] def format_name(s): return s[0].upper() + s[1:].lower() formatted_names = list(map(format_name, names)) print(formatted_names) # 输出: ['Adam', 'Lisa', 'Bart'] ``` #### 二、`reduce()` 函数 `reduce()` 是 Python 内置的另一个高阶函数,用于对列表中的所有元素进行累积操作,如求和、求积等。 **示例**: 假设有一个函数 `add(x, y)`,我们希望通过 `reduce()` 来计算列表 `[1, 3, 5, 7, 9]` 的所有元素之和: ```python from functools import reduce def add(x, y): return x + y result = reduce(add, [1, 3, 5, 7, 9]) print(result) # 输出: 25 ``` **使用初始值**: `reduce()` 还可以接收一个可选的第三个参数作为初始值。例如,如果我们将初始值设置为 100,则计算 `reduce(add, [1, 3, 5, 7, 9], 100)` 的结果将是 125。 ```python result_with_initial_value = reduce(add, [1, 3, 5, 7, 9], 100) print(result_with_initial_value) # 输出: 125 ``` **示例**: 现在让我们使用 `reduce()` 来求一个列表 `[2, 4, 5, 7, 12]` 的乘积: ```python def prod(x, y): return x * y product = reduce(prod, [2, 4, 5, 7, 12]) print(product) # 输出: 3360 ``` #### 三、`filter()` 函数 `filter()` 是 Python 内置的另一个有用的高阶函数,它接收一个函数 `f` 和一个列表,函数 `f` 的作用是对列表中的每个元素进行判断,返回 `True` 或 `False`。`filter()` 根据判断结果自动过滤掉不符合条件的元素,返回由符合条件的元素组成的新列表。 **示例**: 假设我们有一个列表 `[1, 4, 6, 7, 9, 12, 17]`,我们希望保留其中所有的奇数: ```python def is_odd(x): return x % 2 == 1 filtered_list = list(filter(is_odd, [1, 4, 6, 7, 9, 12, 17])) print(filtered_list) # 输出: [1, 7, 9, 17] ``` **示例**: 接下来,我们尝试删除列表中的 `None` 或者空字符串: ```python def is_not_empty(s): return s and len(s.strip()) > 0 non_empty_strings = list(filter(is_not_empty, ['test', None, '', 'str', '', 'END'])) print(non_empty_strings) # 输出: ['test', 'str', 'END'] ``` #### 四、自定义排序函数 `sorted()` Python 内置的 `sorted()` 函数可以用来对列表进行排序。 **示例**: 对一个数字列表进行排序: ```python numbers = [36, 5, 12, 9, 21] sorted_numbers = sorted(numbers) print(sorted_numbers) # 输出: [5, 9, 12, 21, 36] ``` **示例**: 对于字符串排序,默认情况下 `sorted()` 按照 ASCII 码的大小顺序进行排序: ```python words = ['bob', 'about', 'Zoo', 'Credit'] sorted_words = sorted(words) print(sorted_words) # 输出: ['Credit', 'Zoo', 'about', 'bob'] ``` **自定义排序**: 我们可以自定义排序函数,例如,忽略大小写进行排序: ```python def cmp_ignore_case(s1, s2): return (s1.lower() > s2.lower()) - (s1.lower() < s2.lower()) words = ['bob', 'About', 'zoo', 'credit'] sorted_words = sorted(words, key=cmp_to_key(cmp_ignore_case)) print(sorted_words) # 输出: ['About', 'bob', 'credit', 'zoo'] ``` 这里需要注意的是,`cmp_to_key` 是 `functools` 模块提供的函数,用于将比较函数转换成关键字函数。因为在 Python 3 中,`sorted()` 接受的关键字参数 `key` 必须是一个函数,而不是比较函数。 以上就是关于 Python 中 `map()`、`reduce()`、`filter()` 和自定义排序函数 `sorted()` 的详细介绍及示例代码。这些函数在实际编程过程中非常有用,掌握它们能够帮助我们更加高效地处理数据。
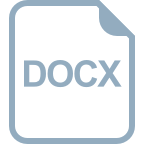
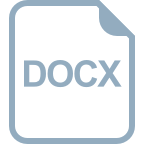
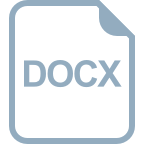
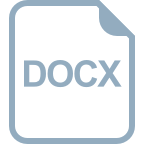
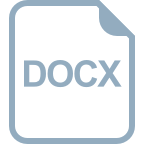
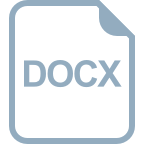
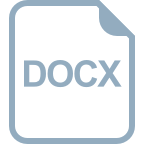
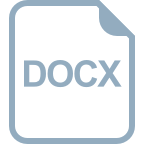
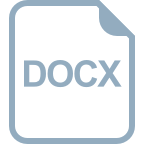
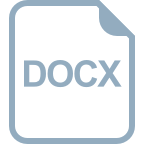
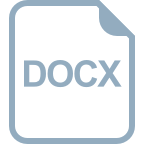
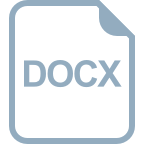
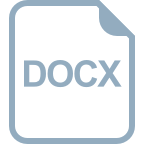
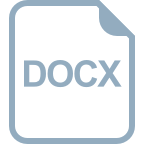
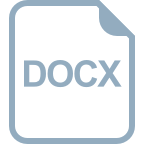
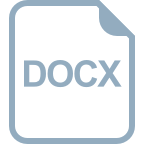
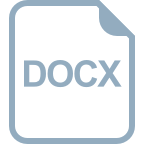
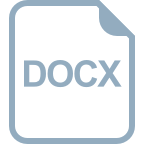
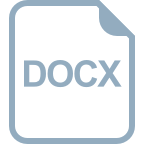
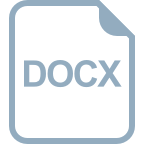
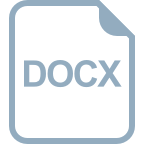
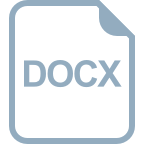
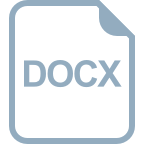
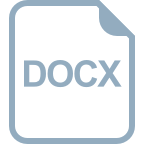
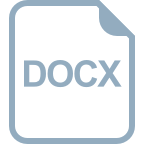
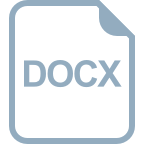
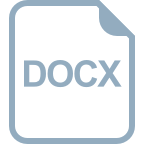
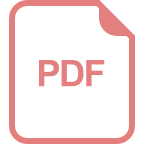
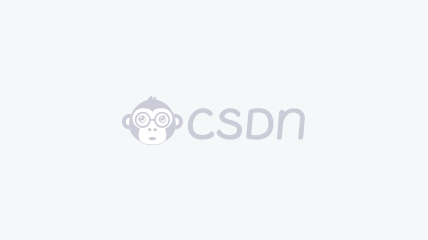

- 粉丝: 4
- 资源: 7万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

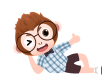
最新资源

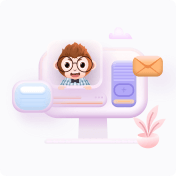
