<?php
/**
* Written by David Meng <md-chinese@163.com>
* http://www.systn.com
* Version:ET.070718
*/
define("Ease Template!",TRUE);
class template{
var $ThisFile = ''; //当前文件
var $IncFile = ''; //引入文件
var $ThisValue = array(); //当前数值
var $FileList = array(); //载入文件列表
var $IncList = array(); //引入文件列表
var $ImgDir = array('images'); //图片地址目录
var $HtmDir = 'cache_htm/'; //静态存放的目录
var $HtmID = ''; //静态文件ID
var $HtmTime = '180'; //秒为单位,默认三分钟
var $AutoImage = 1; //自动解析图片目录开关默认值
var $Hacker = "<?php if(!defined('Ease Template!')){die('You are Hacker!<br>Power by Ease Template!');}?>";
var $Compile = array(
'/(\{\s*|<!--\s*)inc_php:([^\{\}]{1,100})(\s\}|\s*-->)/is',
'/<!--\s*IF(\[|\()(.+?)(\]|\))\s*-->/is',
'/<!--\s*ELSEIF(\[|\()(.+?)(\]|\))\s*-->/is',
'/<!--\s*ELSE\s*-->/is',
'/<!--\s*END\s*-->/is',
'/<!--\s*([a-zA-Z0-9_\$\[\]\'\"]{2,60})\s*(AS|as)\s*(.+?)\s*-->/',
'/<!--\s*while\:\s*(.+?)\s*-->/is',
'/(\{\s*|<!--\s*)row\:(.+?)(\s*\}|\s*-->)/eis',
'/(\{\s*|<!--\s*)color\:\s*(.+?)(\s*\}|\s*-->)/eis',
'/(\{\s*|<!--\s*)img\:([^\{\}]{1,100})(\s*\}|\s*-->)/eis',
'/(\{\s*|<!--\s*)run\:(\}|\s*-->)\s*(.+?)\s*(\{|<!--\s*)\/run(\s*\}|\s*-->)/is',
'/(\{\s*|<!--\s*)run\:(.+?)(\s*\}|\s*-->)/is',
'/\{([a-zA-Z0-9_\'\"\[\]\$]+)\}/',
);
var $Analysis = array(
'<?include_once(\'\\2\');?>',
'<?if(\\2){?>',
'<?}elseif(\\2){?>',
'<?}else{?>',
'<?}?>',
'<?\$_i=0;\\1=(is_array(\\1))?\\1:array();foreach(\\1 AS \\3){\$_i++;?>',
'<?\$_i=0;while(\\1){\$_i++;?>',
'$this->Row("\\2")',
'$this->Color("\\2")',
'$this->Image("\\2")',
'<?\\3;?>',
'<?\\2;?>',
'<?echo \$\\1;?>',
);
/**
* 声明模板用法
*/
function template(
$set = array(
'ID'=>'1', //缓存ID
'TplType'=>'htm', //模板格式
'CacheDir'=>'cache', //缓存目录
'TemplateDir'=>'template' , //模板存放目录
'AutoImage'=>'on' , //自动解析图片目录开关 on表示开放 off表示关闭
)
){
$this->TplID = (defined('TemplateID')?TemplateID:(((int)$set['ID']<=1)?1:(int)$set['ID'])).'_';
$this->CacheDir = (defined('NewCache')?NewCache:((trim($set['CacheDir'])!='')?$set['CacheDir']:'cache')).'/';
$this->TemplateDir = (defined('NewTemplate')?NewTemplate:((trim($set['TemplateDir'])!='')?$set['TemplateDir']:'template')).'/';
$this->Ext = ($set['TplType']!='')?$set['TplType']:'htm';
$this->AutoImage = ($set['AutoImage']=='off')?0:1;
//缓存目录检测以及运行模式
$this->RunType = (@substr(@sprintf('%o', @fileperms($this->CacheDir)), -3)!=777)?'Replace':'Cache';
}
/**
* 设置模板文件
* set_file(文件名,设置数组);
*/
function set_file(
$FileName,
$NewDir=''
){
$this->ThisFile = $FileName.'.'.$this->Ext;
$this->IncList[] = $this->TemplateDir.$this->ThisFile;
if($NewDir!='')
$this->NewDir = $NewDir.'/';
}
/**
* 设置数值
* set_var(变量名或是数组,设置数值);
*/
function set_var(
$name,
$var = ''
){
if (is_array($name)){
$this->ThisValue = @array_merge($this->ThisValue,$name); //merge two or more arrays
}else{
$this->ThisValue[$name] = $var;
}
}
/**
* 改变静态刷新时间
*/
function htm_time($times=0){
if((int)$times>0){
$this->HtmTime = (int)$times;
}
}
/**
* 静态文件存放的绝对目录
*/
function htm_dir($name=''){
if(trim($name)){
$this->HtmDir = trim($name).'/';
}
}
/**
* 产生静态文件输出
*/
function HtmCheck(
$name=''
){
$this->HtmID = md5(trim($name)? trim($name).'.php' : $GLOBALS['_SERVER']['REQUEST_URI'].'.php' );
//检测时间
if(is_file($this->HtmDir.$this->HtmID) && (time() - @filemtime($this->HtmDir.$this->HtmID)<=$this->HtmTime)){
ob_start();
include_once $this->HtmDir.$this->HtmID;
$HtmContent = ob_get_contents();
ob_end_clean();
return $HtmContent;
}
}
/**
* 打印静态内容
*/
function htm_p($name=''){
$HtmData = $this->HtmCheck($name);
if($HtmData){
die($HtmData);
}
}
/**
* 输出静态内容
*/
function htm_r($name=''){
return $this->HtmCheck($name);
}
//编译缓存文件包括连载功能
function Compile(
$FileName = '',
$kind='',
$temp=''
){
if($kind!='' && count($this->FileList)>0 && !file_exists($this->CacheDir.$kind.'.php')){
foreach($this->FileList AS $k=>$v){ $KindData .= $this->CompilePHP($this->Compile($v.$k,'',1)); }
$this->writer($this->FileName($kind,$this->TplID) ,$this->Hacker.$KindData);
}elseif($temp==1){
$SourceFile = ($FileName == '') ? $this->TemplateDir.$this->NewDir.$this->ThisFile : $FileName;
if(!in_array($SourceFile,$this->FileList)){
$content = $this->reader($SourceFile);
//include
$content = $this->inc_preg($content);
return $content = @preg_replace($this->Compile, $this->Analysis, $content);
}
}else{
$SourceFile = ($FileName == '') ? $this->TemplateDir.$this->NewDir.$this->ThisFile : $FileName;
if(!in_array($SourceFile,$this->FileList)){
$content = $this->reader($SourceFile);
//include
$content = $this->inc_preg($content);
$content = $this->CompilePHP($content, 'kind');
$this->writer($this->FileName($this->ThisFile,$this->TplID) ,$this->Hacker.$content);
}
}
}
function n(){
$this->FileList[$this->ThisFile] = $this->TemplateDir;
//文件日期(用于对比最新时间更新模板)
$this->FileDate[] = @filemtime($this->TemplateDir.$this->NewDir.$this->ThisFile);
}
/**
* 输出模板内容
*/
function r(
$kind=''
){
if($this->RunType=='Cache'){
return ($kind=='include_page')?$this->ParsePHP($kind):$this->ParseHtml($kind);
}else{
if($kind){
$kind = ($kind=='include_page')?'include_page':$this->FileList;
return $this->ParseCode($kind);
}else{
return $this->ParseCode();
}
}
}
/**
* 打印模板内容
*/
function p(
$kind=''
){
if($this->RunType=='Cache'){
echo ($kind=='include_page')?$this->ParsePHP($kind):$this->ParseHtml($kind,'print');
}else{
if($kind){
$kind = ($kind=='include_page')?'include_page':$this->FileList;
echo $this->ParseCode($kind);
}else{
echo $this->ParseCode();
}
}
}
//解析替换程序
function ParseCode(
$FileList=''
){
//解析续载
if (@is_array($FileList) && $FileList!='include_page'){
$ShowTPL = '';
foreach ($FileList AS $K=>$V) {
$ShowTPL .= $this->reader($V.$K);
}
}else{
$SourceFile = $this->TemplateDir.$this->NewDir.$this->ThisFile;
if(!is_file($SourceFile)){
die('Sorry, the file '.$SourceFile.' does not exist.');
}
$ShowTPL = $this->reader($SourceFile);
if ($FileList=='include_page'){
return $ShowTPL;
}
}
//File Leng
$ReadSize = strlen($ShowTPL);
//include
$ShowTPL = $this->inc_preg($ShowTPL);
//Fix =
$ShowTPL = preg_replace('/(\{|<!--\s*)run:(\}|\s*-->)\s*=/','{run:}echo ',$ShowTPL);
$ShowTPL = preg_replace('/(\{|<!--\s*)run:\s*=/','{run:echo ',$ShowTPL);
//Fix Run 1
$ShowTPL = preg_replace('/(\{|<!--\s*)run\:(\}|\s*-->)\s*(.+?)\s*(\{|<!--\s*)\/run(\}|\s*-->)/is', '(T_T)\\3;(T_T!)',$ShowTPL);
foreach($this->Analysis AS $A_k => $A_v){
$A_v = str_replace('<?','(^_^);',$A_v);
$A_v = str_replace('?>','echo(^_^)',$A_v);
$this->Analysis[$A_k] = $A_v;
}
$ShowTPL = str_replace('\\','\\\\',$ShowTPL);
$ShowTPL = str_replace('"','\"',$ShowTPL);
$ShowTPL = @preg_replace($this->Compile, $this->Analysis, $ShowTPL);
$ShowTPL = $this->ImgCheck($ShowTPL);
//Fix javascript
$ShowTPL = str_replace('$','\$',$ShowTPL);
$ShowTPL = @preg_replace("/\(\^_\^\);(.+?)echo\(\^_\^\)/e", '$this->FixPHP(\'\\1\')', $ShowTPL);
$ShowTPL = str_replace('(^_^)','"','echo (^_^)'.$ShowTPL.'(^_^);');
//Fix Run 2
$ShowTPL = preg_replace("/\(T_T\)(.+?)\(T_T!\)/ise", '$this->FixRun(\'\\1\')', $ShowTPL);
//从数组中
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
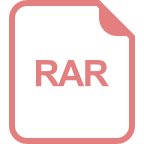
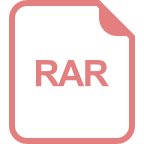
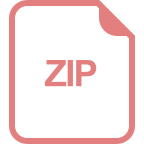
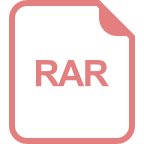
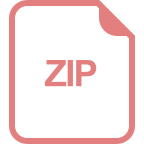
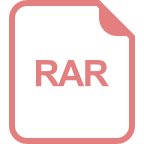
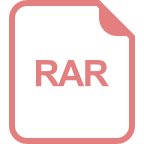
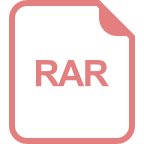
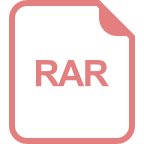
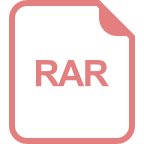
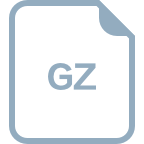
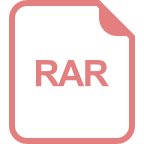
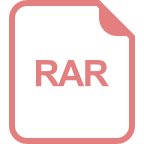
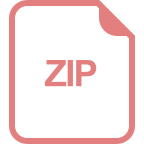
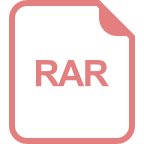
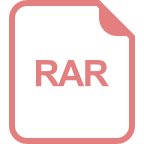
收起资源包目录



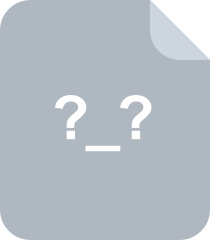
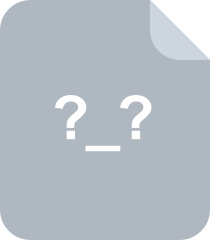
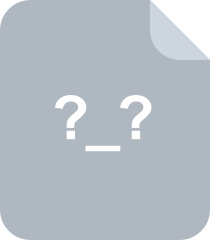
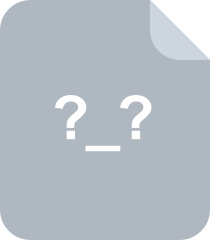
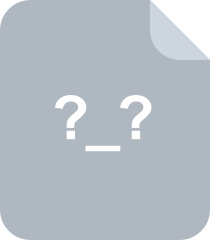

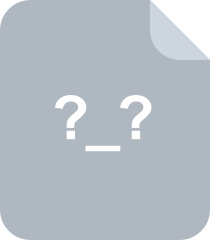
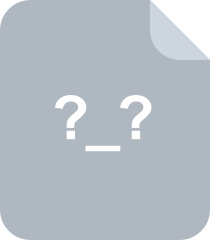

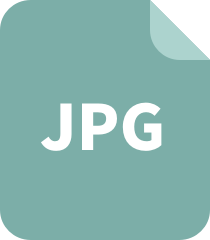
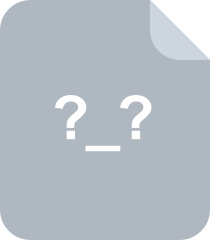
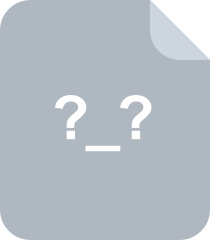
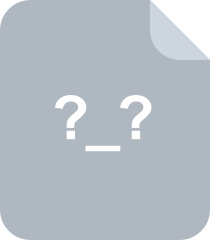
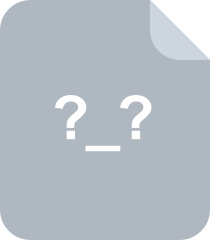
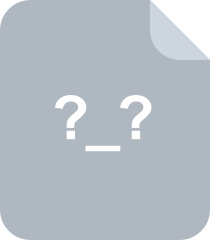
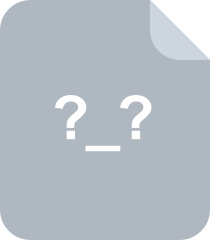
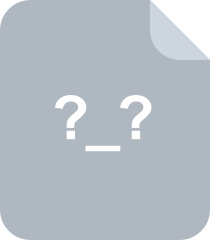
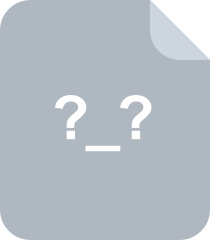
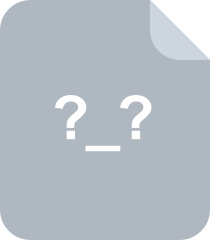
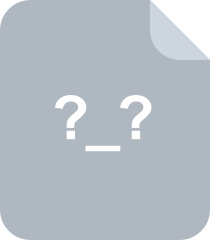
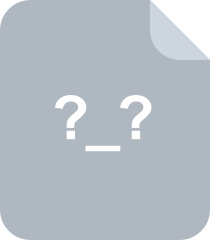
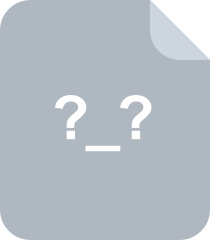
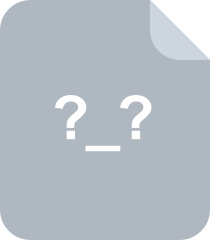
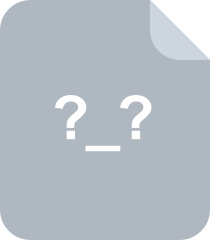
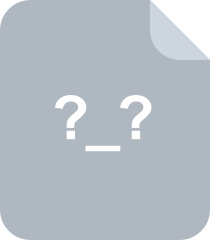

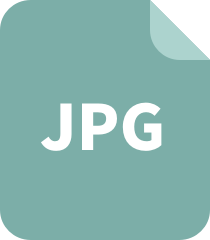

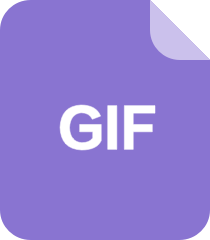
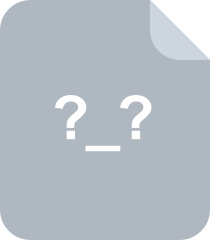
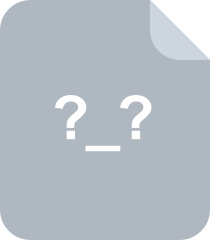
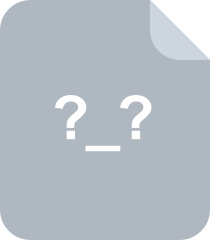
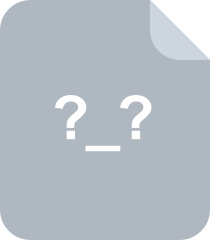
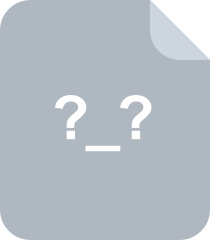
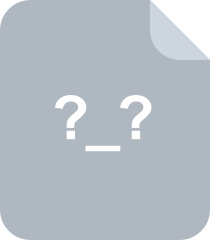

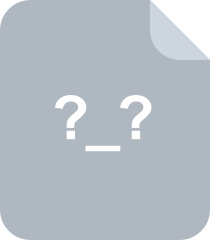
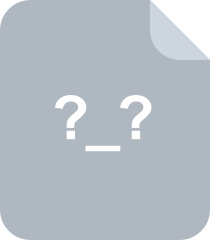
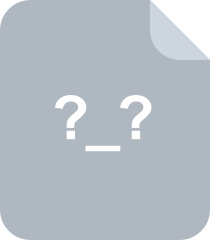
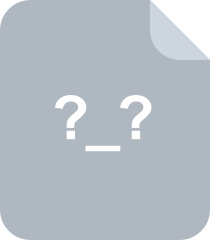
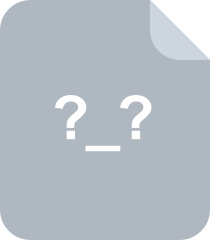
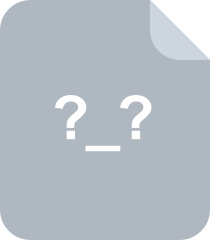
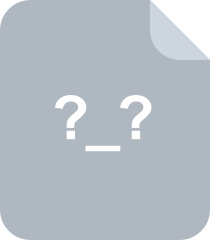
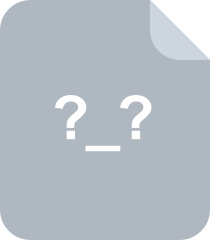
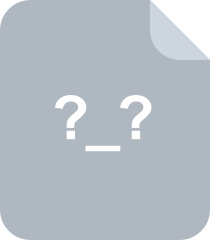
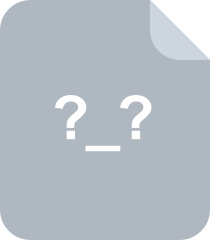
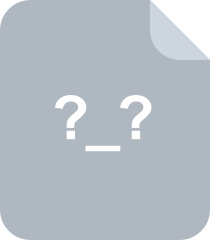
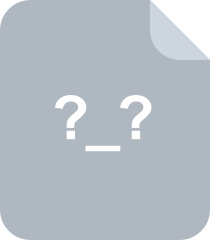
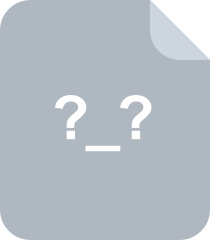
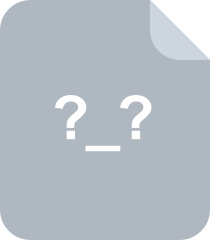
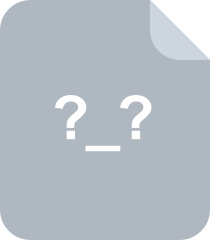
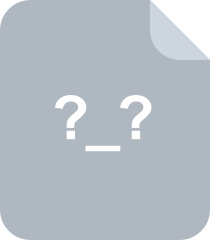

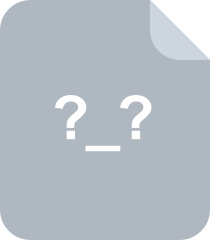
共 48 条
- 1
资源评论
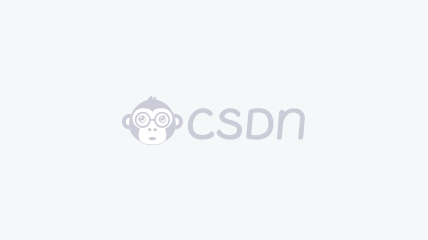
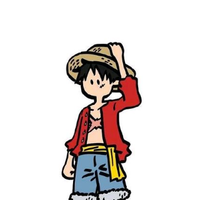
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

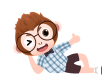
最新资源
- electron-v32.2.5-win32-x64资源包
- 日历组件使用········
- java班级管理系统(java毕业设计源码).zip
- bochb_assist_2.0.0.apk
- java无线点餐系统源码数据库 MySQL源码类型 WebForm
- 简历模板嵌入式常用知识&面试题库200M
- 常用基础元件的PCB封装库SchLib/IntLib通用原理图库接插件-脚距3.96
- 常用基础元件的PCB封装库SchLib/IntLib通用原理图库STM32 F2系列单片机
- 常用基础元件的PCB封装库SchLib/IntLib通用原理图库PIC系列单片机
- java通用后台管理系统源码数据库 MySQL源码类型 WebForm
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


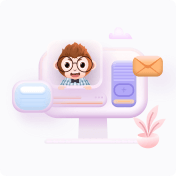
安全验证
文档复制为VIP权益,开通VIP直接复制
