function [x,endPop,bPop,traceInfo] = ga(bounds,evalFN,evalOps,startPop,opts,...
termFN,termOps,selectFN,selectOps,xOverFNs,xOverOps,mutFNs,mutOps)
% GA run a genetic algorithm
% function [x,endPop,bPop,traceInfo]=ga(bounds,evalFN,evalOps,startPop,opts,
% termFN,termOps,selectFN,selectOps,
% xOverFNs,xOverOps,mutFNs,mutOps)
%
% Output Arguments:
% x - the best solution found during the course of the run
% endPop - the final population
% bPop - a trace of the best population
% traceInfo - a matrix of best and means of the ga for each generation
%
% Input Arguments:
% bounds - a matrix of upper and lower bounds on the variables
% evalFN - the name of the evaluation .m function
% evalOps - options to pass to the evaluation function ([NULL])
% startPop - a matrix of solutions that can be initialized
% from initialize.m
% opts - [epsilon prob_ops display] change required to consider two
% solutions different, prob_ops 0 if you want to apply the
% genetic operators probabilistly to each solution, 1 if
% you are supplying a deterministic number of operator
% applications and display is 1 to output progress 0 for
% quiet. ([1e-6 1 0])
% termFN - name of the .m termination function (['maxGenTerm'])
% termOps - options string to be passed to the termination function
% ([100]).
% selectFN - name of the .m selection function (['normGeomSelect'])
% selectOpts - options string to be passed to select after
% select(pop,#,opts) ([0.08])
% xOverFNS - a string containing blank seperated names of Xover.m
% files (['arithXover heuristicXover simpleXover'])
% xOverOps - A matrix of options to pass to Xover.m files with the
% first column being the number of that xOver to perform
% similiarly for mutation ([2 0;2 3;2 0])
% mutFNs - a string containing blank seperated names of mutation.m
% files (['boundaryMutation multiNonUnifMutation ...
% nonUnifMutation unifMutation'])
% mutOps - A matrix of options to pass to Xover.m files with the
% first column being the number of that xOver to perform
% similiarly for mutation ([4 0 0;6 100 3;4 100 3;4 0 0])
% Binary and Real-Valued Simulation Evolution for Matlab
% Copyright (C) 1996 C.R. Houck, J.A. Joines, M.G. Kay
%
% C.R. Houck, J.Joines, and M.Kay. A genetic algorithm for function
% optimization: A Matlab implementation. ACM Transactions on Mathmatical
% Software, Submitted 1996.
%
% This program is free software; you can redistribute it and/or modify
% it under the terms of the GNU General Public License as published by
% the Free Software Foundation; either version 1, or (at your option)
% any later version.
%
% This program is distributed in the hope that it will be useful,
% but WITHOUT ANY WARRANTY; without even the implied warranty of
% MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
% GNU General Public License for more details. A copy of the GNU
% General Public License can be obtained from the
% Free Software Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
%%$Log: ga.m,v $
%Revision 1.10 1996/02/02 15:03:00 jjoine
% Fixed the ordering of imput arguments in the comments to match
% the actual order in the ga function.
%
%Revision 1.9 1995/08/28 20:01:07 chouck
% Updated initialization parameters, updated mutation parameters to reflect
% b being the third option to the nonuniform mutations
%
%Revision 1.8 1995/08/10 12:59:49 jjoine
%Started Logfile to keep track of revisions
%
n=nargin;
if n<2 | n==6 | n==10 | n==12
disp('Insufficient arguements')
end
if n<3 %Default evalation opts.
evalOps=[];
end
if n<5
opts = [1e-6 1 0];
end
if opts==[]
opts = [1e-6 1 0];
end
if any(evalFN<48) %Not using a .m file
if opts(2)==1 %Float ga
e1str=['x=c1; c1(xZomeLength)=', evalFN ';'];
e2str=['x=c2; c2(xZomeLength)=', evalFN ';'];
else %Binary ga
e1str=['x=b2f(endPop(j,:),bounds,bits); endPop(j,xZomeLength)=',...
evalFN ';'];
end
else %Are using a .m file
if opts(2)==1 %Float ga
e1str=['[c1(xZomeLength) c1]=' evalFN '(c1,[gen evalOps]);'];
e2str=['[c2(xZomeLength) c2]=' evalFN '(c2,[gen evalOps]);'];
else %Binary ga
e1str=['x=b2f(endPop(j,:),bounds,bits);[v x]=' evalFN ...
'(x,[gen evalOps]); endPop(j,:)=[f2b(x,bounds,bits) v];'];
end
end
if n<6 %Default termination information
termOps=[100];
termFN='maxGenTerm';
end
if n<12 %Default muatation information
if opts(2)==1 %Float GA
mutFNs=['boundaryMutation multiNonUnifMutation nonUnifMutation unifMutation'];
mutOps=[4 0 0;6 termOps(1) 3;4 termOps(1) 3;4 0 0];
else %Binary GA
mutFNs=['binaryMutation'];
mutOps=[0.05];
end
end
if n<10 %Default crossover information
if opts(2)==1 %Float GA
xOverFNs=['arithXover heuristicXover simpleXover'];
xOverOps=[2 0;2 3;2 0];
else %Binary GA
xOverFNs=['simpleXover'];
xOverOps=[0.6];
end
end
if n<9 %Default select opts only i.e. roullete wheel.
selectOps=[];
end
if n<8 %Default select info
selectFN=['normGeomSelect'];
selectOps=[0.08];
end
if n<6 %Default termination information
termOps=[100];
termFN='maxGenTerm';
end
if n<4 %No starting population passed given
startPop=[];
end
if startPop==[] %Generate a population at random
%startPop=zeros(80,size(bounds,1)+1);
startPop=initialize(80,bounds,evalFN,evalOps,opts(1:2));
end
if opts(2)==0 %binary
bits=calcbits(bounds,opts(1));
end
xOverFNs=parse(xOverFNs);
mutFNs=parse(mutFNs);
xZomeLength = size(startPop,2); %Length of the xzome=numVars+fittness
numVar = xZomeLength-1; %Number of variables
popSize = size(startPop,1); %Number of individuals in the pop
endPop = zeros(popSize,xZomeLength); %A secondary population matrix
c1 = zeros(1,xZomeLength); %An individual
c2 = zeros(1,xZomeLength); %An individual
numXOvers = size(xOverFNs,1); %Number of Crossover operators
numMuts = size(mutFNs,1); %Number of Mutation operators
epsilon = opts(1); %Threshold for two fittness to differ
oval = max(startPop(:,xZomeLength)); %Best value in start pop
bFoundIn = 1; %Number of times best has changed
done = 0; %Done with simulated evolution
gen = 1; %Current Generation Number
collectTrace = (nargout>3); %Should we collect info every gen
floatGA = opts(2)==1; %Probabilistic application of ops
display = opts(3); %Display progress
while(~done)
%Elitist Model
[bval,bindx] = max(startPop(:,xZomeLength)); %Best of current pop
best = startPop(bindx,:);
if collectTrace
traceInfo(gen,1)=gen; %current generation
traceInfo(gen,2)=startPop(bindx,xZomeLength); %Best fittness
traceInfo(gen,3)=mean(startPop(:,xZomeLength)); %Avg fittness
end
if ( (abs(bval - oval)>epsilon) | (gen==1)) %If we have a new best sol
if display
fprintf(1,'\n%d %f\n',gen,bval); %Update the display
end
if floatGA
bPop(bFoundIn,:)=[gen startPop(bindx,:)]; %Update bPop Matrix
else
bPop(bFoundIn,:)=[gen b2f(startPop(bindx,1:numVar),bounds,bits)...
startPop(bindx,xZomeLength)];
end
bFoundIn=bFoundIn+1; %Update number of changes
oval=bval; %Update the best val
else
if display
fprintf(1,'%d ',gen); %Otherwise just update num gen
end
end
endPop = feval(selectFN,startPop,[gen selectOps]); %Select
if floatGA %Running with the model where the para
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
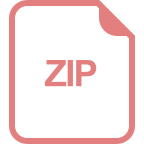
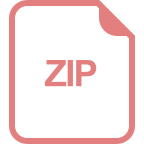
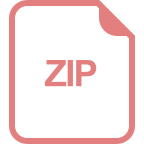
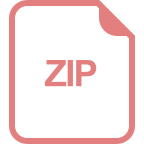
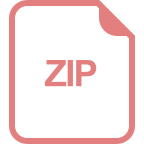
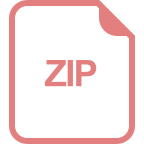
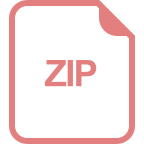
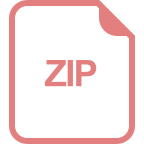
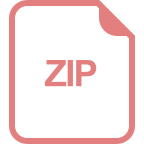
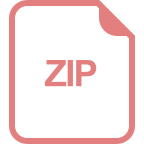
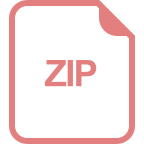
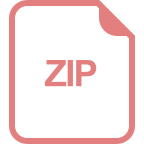
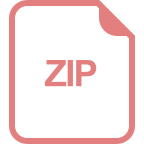
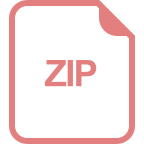
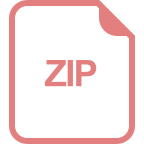
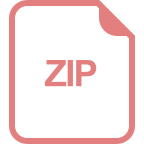
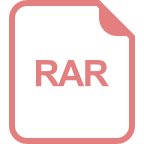
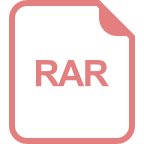
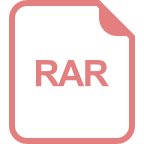
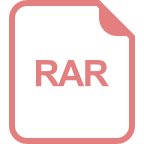
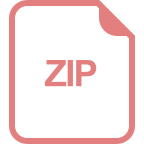
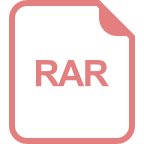
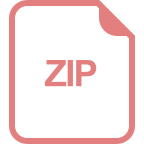
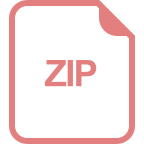
收起资源包目录



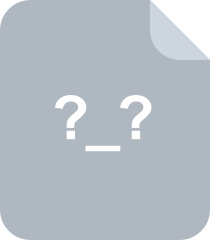
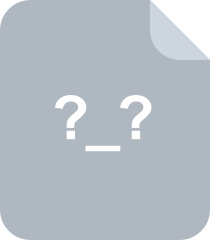
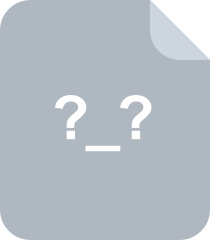
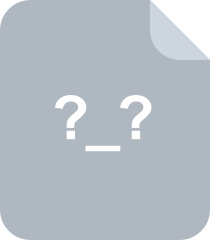
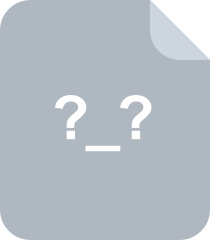
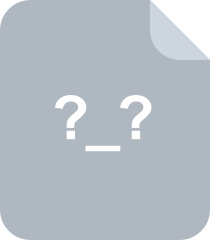
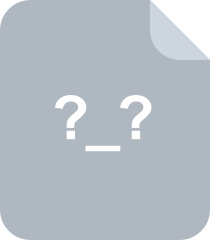
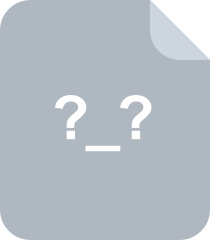
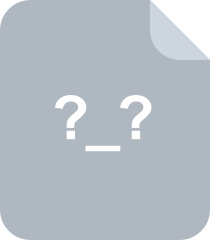
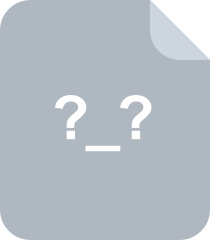
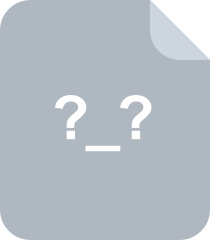
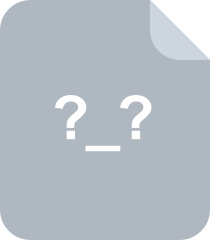
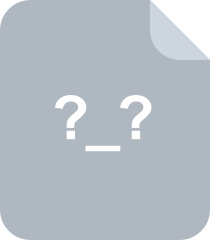
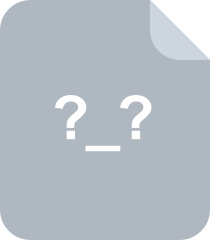
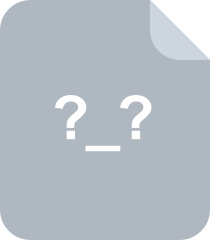
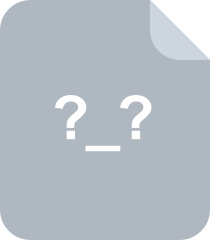
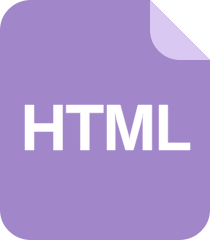
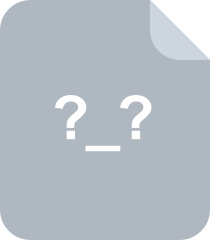
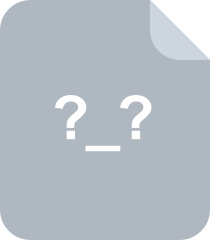
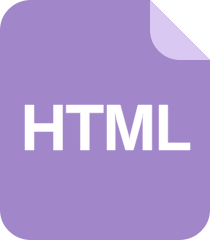
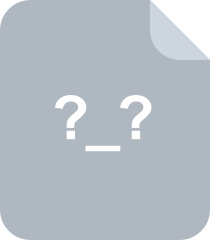
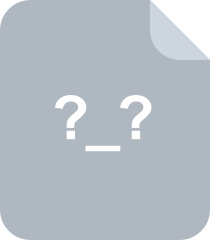
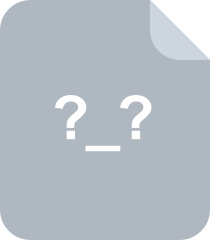
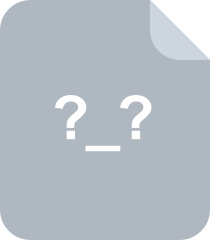
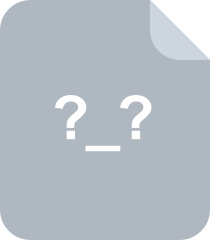
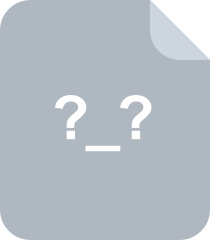
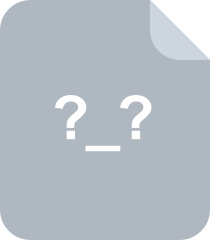
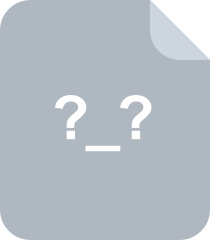
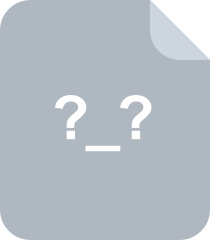
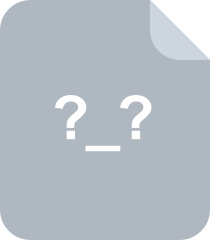
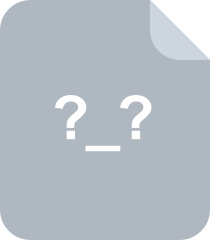
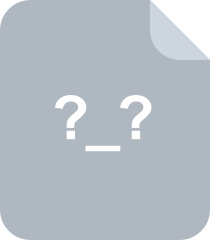

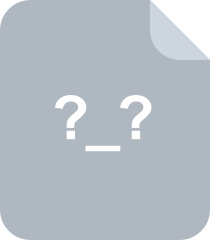
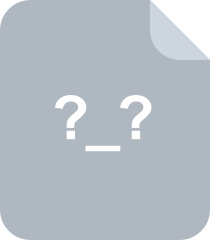
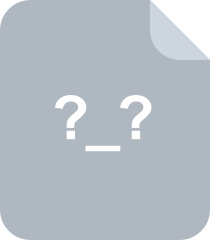
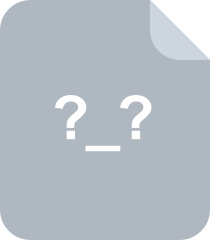
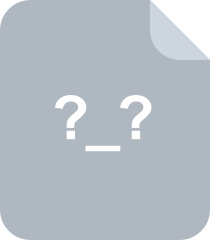

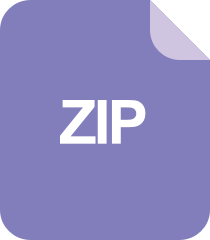
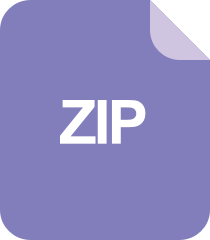
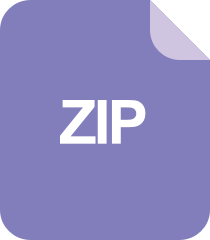
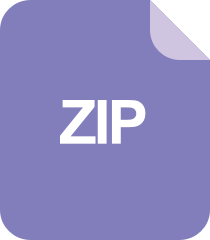
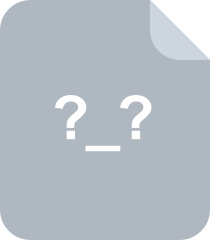
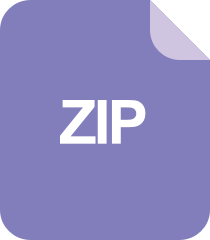
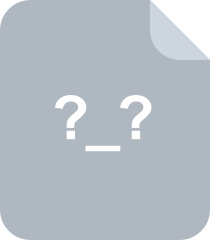
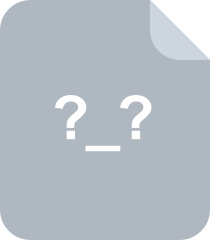
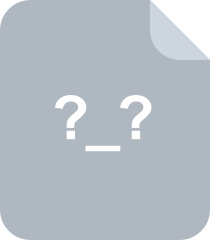
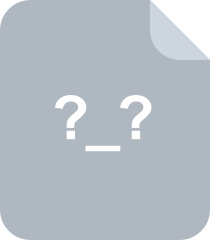
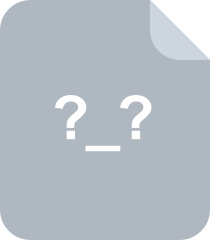
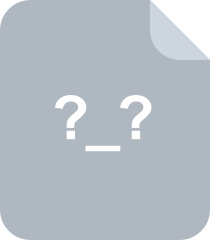
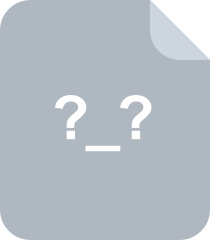
共 51 条
- 1
资源评论
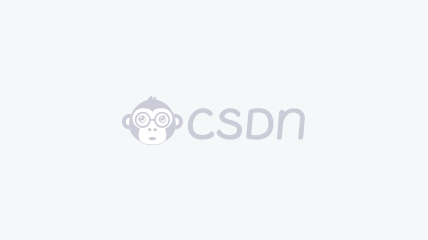
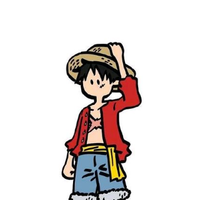
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

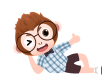
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


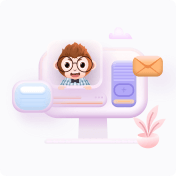
安全验证
文档复制为VIP权益,开通VIP直接复制
