<?php
require_once('msnpauth.php');
define('ERR_AUTHENTICATION_FAILED', 911);
define('ERR_SERVER_UNAVAILABLE', 600);
define('ERR_USER_OFFLINE', 217);
define('ERR_TOO_MANY_SESSIONS', 800);
define('OK', 1);
class sendMsg
{
var $_passport;
var $_password;
var $_account;
var $_sockets;
var $_msg;
var $result;
var $error;
function simpleSend($passport, $password, $recipient, $message)
{
$this->login($passport, $password);
if ($this->result > 1)
{
return;
}
$this->createSession($recipient);
$this->sendMessage($message);
}
function login($passport, $password)
{
$this->_passport = $passport;
$this->_password = $password;
$this->_connect('NS');
$this->_send_data('NS', 'VER 0 MSNP8');
$this->_read();
}
function createSession($account)
{
// Stop if login failed.
if ($this->result > 1)
{
return;
}
$this->_account = $account;
$this->_send_data('NS', 'XFR 5 SB');
$this->_read();
}
function sendMessage($message, $font = NULL, $color = NULL)
{
// Stop if login failed.
if ($this->result > 1)
{
return;
}
$head = "MIME-Version: 1.0\r\nContent-Type: text/plain; charset=UTF-8\r\n";
if (isset($font))
{
$font = rawurlencode($font);
$head .= "X-MMS-IM-Format: FN=$font; EF=; CO=$color; CS=0; PF=22\r\n";
}
$head .= "\r\n";
$len = strlen($head.$message);
$this->_send_data('SB', "MSG 3 U $len\r\n$head$message");
$this->result = OK;
}
function _connect($socket, $server = 'messenger.hotmail.com')
{
// This part does a DNS lookup on the server, and fails with error if it is not found.
// fsockopen doesn't return an error on DNS lookup failures, instead it outputs a warning (which we want to suppress).
if ((gethostbyname($server) == $server) && !is_numeric(str_replace('.', '', $server)))
{
$this->result = ERR_SERVER_UNAVAILABLE;
$this->error = 'Host not found'.$server;
return false;
}
ini_set('default_socket_timeout', 2);
$this->_sockets[$socket] = @fsockopen($server, 1863, $errno, $errstr, 2);
if ($this->_sockets[$socket] == false)
{
$this->result = $errno;
$this->error = $errstr;
return false;
}
return true;
}
function _read($socket = 'NS')
{
$r = false;
while ($this->_sockets[$socket] && !feof($this->_sockets[$socket]) && !$r)
{
$data = fgets($this->_sockets[$socket], 1024);
if (!$data)
{
continue;
}
$data = substr($data, 0, -2);
$r = $this->_process_data($data);
if ($r)
{
return;
}
}
}
function _send_data($socket, $data)
{
if (substr($data, 0, 3) == 'MSG')
{
// Don't send appending new line if it's a payload command. (MSG)
fputs($this->_sockets[$socket], $data);
}
else
{
fputs($this->_sockets[$socket], "$data\r\n");
}
}
function _process_data($data)
{
$params = explode(' ', $data);
switch ($params[0])
{
case 'VER':
$this->_send_data('NS', 'CVR 1 0x0409 winnt 5.1 i386 MSNMSGR 6.0.0254 MSMSGS '.$this->_passport);
break;
case 'CVR':
$this->_send_data('NS', 'USR 2 TWN I '.$this->_passport);
break;
case 'XFR':
$subParams = explode(':', $params[3]);
$r = $this->_connect($params[2], $subParams[0]);
if (!$r)
{
return true;
}
if ($params[2] == 'NS')
{
$this->_send_data('NS', 'VER 0 MSNP8');
}
elseif ($params[2] == 'SB')
{
$this->_send_data('SB', 'USR 1 '.$this->_passport.' '.$params[5]);
}
$this->_read($params[2]);
return true;
break;
case 'USR':
if ($params[2] == 'TWN')
{
$msnpauth = new MSNPAuth($this->_passport, $this->_password, $params[4]);
$hash = $msnpauth->getKey();
if (!$hash)
{
$this->result = ERR_AUTHENTICATION_FAILED;
$this->_send_data('NS', 'OUT');
return false;
}
$this->_send_data('NS', 'USR 3 TWN S '.$hash);
}
elseif ($params[2] == 'OK')
{
if (count($params) == 7)
{
$this->_send_data('NS', 'CHG 4 NLN');
return true;
}
else
{
$this->_send_data('SB', 'CAL 0 '.$this->_account);
}
}
break;
case 'JOI':
return true;
break;
// Error code handling
case '500':
case '600':
case '601':
case '910':
case '911':
case '921':
case '928':
$this->result = ERR_SERVER_UNAVAILABLE;
return true;
break;
case '800':
$this->result = ERR_TOO_MANY_SESSIONS;
return true;
break;
case '217':
$this->result = ERR_USER_OFFLINE;
return true;
break;
}
return false;
}
}
?>
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
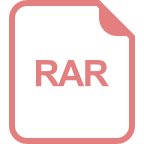
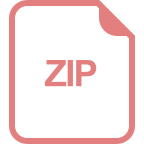
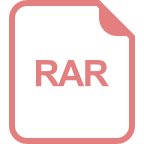
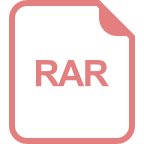
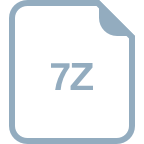
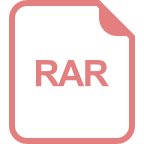
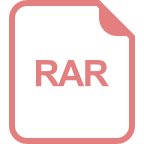
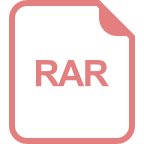
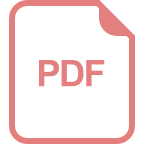
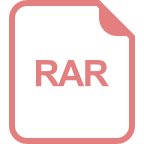
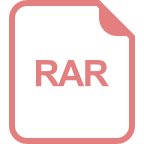
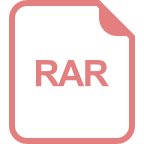
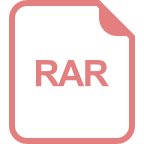
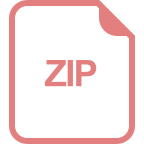
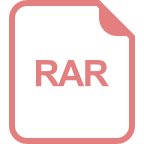
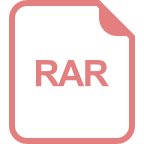
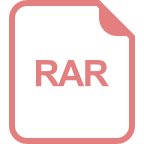
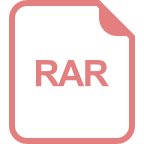
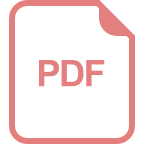
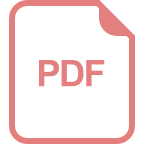
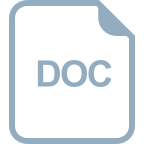
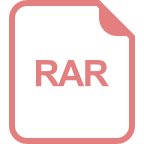
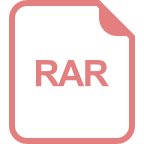
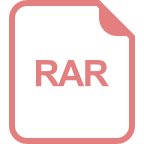
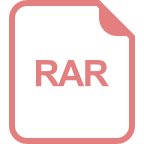
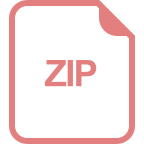
收起资源包目录



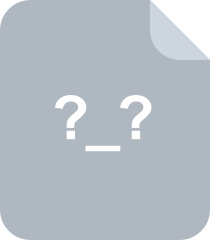
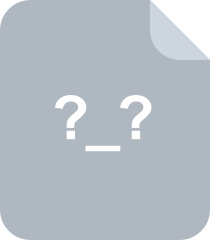
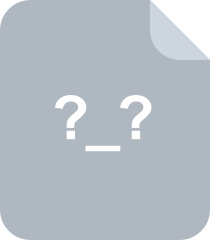
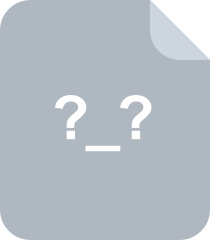
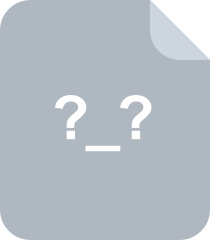
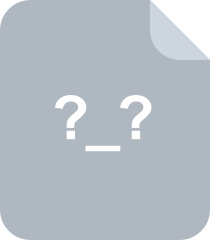
共 6 条
- 1
资源评论
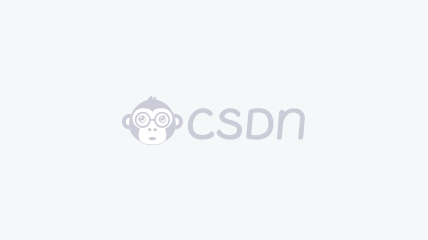
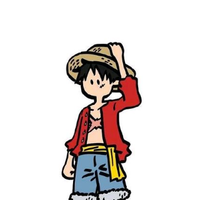
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

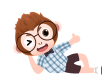
最新资源
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
- (源码)基于Spring Boot和Vue的房屋租赁管理系统.zip
- (源码)基于Android的饭店点菜系统.zip
- (源码)基于Android平台的权限管理系统.zip
- (源码)基于CC++和wxWidgets框架的LEGO模型火车控制系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


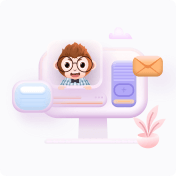
安全验证
文档复制为VIP权益,开通VIP直接复制
