<?php
// Set flag that this is a parent file
define( '_FEXEC', 1 );
require('includes/application_top.php');
require(DIR_WS_CLASSES . 'currencies.php');
include(DIR_WS_FUNCTIONS . 'events_sessions.php');
include(DIR_WS_LANGUAGES . $FSESSION->language . "/" . FILENAME_CHECKOUT_ATTENDEES);
define('BLOCKED_USER_ERROR','Blocked User');
define('SUSPENDED_USER_ERROR','User Suspended Temporarily');
$server_date = getServerDate(true);
$command=$FREQUEST->getvalue('command');
$step=$FREQUEST->getvalue('step');
$customers_id=$FREQUEST->postvalue('customers_id','int',0);
if($customers_id==0){
$customers_id=$FREQUEST->getvalue('cID','int',0);
}
$currency=DEFAULT_CURRENCY;
$currencies=new currencies;
$action = $FREQUEST->postvalue('action');
if($action=='event_browse'){
$FSESSION->set('param_event',$FREQUEST->postvalue('param_event'));
$FSESSION->set('param_session',$FREQUEST->postvalue('param_session'));
}
//ajax start
if($command!="")
{
switch($command){
case 'show_state':
$zone_ids="";
$zone_name="";
$country=$FREQUEST->getvalue('country_id','int',0);
$zones_query = tep_db_query("select zone_id,zone_name from " . TABLE_ZONES . " where zone_country_id = '" . tep_db_input($country) . "' order by zone_name");
while ($zones_values = tep_db_fetch_array($zones_query)) {
$zone_ids.=$zones_values['zone_id'].",";
$zone_name.=$zones_values['zone_name'].",";
}
$cnt=$FREQUEST->getvalue('cnt');
if($cnt!='') $content= 'show_attendee_state||'.substr($zone_ids,0,-1)."^".substr($zone_name,0,-1)."^".$zone."^".$cnt;
else $content='show_state||'.substr($zone_ids,0,-1)."^".substr($zone_name,0,-1);
echo $content;
break;
/* case 'update_cart':
echo 'updates';
break;
*/
case 'fetch_customers_details':
$cnt= $FREQUEST->getvalue('cnt');
$cust_id= $FREQUEST->getvalue('cust_id');
$customer_query=tep_db_query("select c.customers_firstname,c.customers_lastname,c.customers_email_address as email_address,a.entry_postcode as postcode,a.entry_city as city,a.entry_zone_id as state,a.entry_country_id as country,a.entry_street_address as street_address,a.entry_state from ".TABLE_CUSTOMERS ." c LEFT JOIN ".TABLE_ADDRESS_BOOK." a on(c.customers_id=a.customers_id) where c.customers_id='".tep_db_input($cust_id)."'");
if(tep_db_num_rows($customer_query)>0){
$customer=tep_db_fetch_array($customer_query);
$first_name=$customer['customers_firstname'];
$last_name=$customer['customers_lastname'];
$email_address=$customer['email_address'];
$street_address=$customer['street_address'];
$city=$customer['city'];
$post_code=$customer['postcode'];
$state=tep_get_zone_name($customer['country'],$customer['state'],$customer['entry_state']);
$country=$customer['country'];
$customer_list=$first_name."^".$last_name."^".$email_address."^".$street_address."^".$city ."^".$customer['postcode']."^".$state."^".$country;
}
echo 'customer_list||'.$customer_list.'##'.$cnt;
break;
case 'show_state_billing':
$type=$FREQUEST->getvalue('type','string','B');
$country=$FREQUEST->getvalue('country_id','int',0);
$id=$FREQUEST->getvalue('id','int','');
$zone_ids="";
$zone_name="";
$zones_query = tep_db_query("select zone_id,zone_name from " . TABLE_ZONES . " where zone_country_id = '" . tep_db_input($country) . "' order by zone_name");
while ($zones_values = tep_db_fetch_array($zones_query)) {
$zone_ids.=$zones_values['zone_id'].",";
$zone_name.=$zones_values['zone_name'].",";
}
$content='show_state_billing||'.substr($zone_ids,0,-1)."^".tep_output_string(substr($zone_name,0,-1))."^".$zone."^".$type;
echo $content;
break;
case 'update_cart_details':
break;
case 'show_customer_details':
$customers_id=(int)$FREQUEST->getvalue('cID');
echo $command ."||";
out_customer_details($customers_id);
break;
case 'update_customer':
tep_update_customers($customers_id);
break;
case 'new_product':
require(FILENAME_ADDPRODUCTS_NEW);
break;
case 'new_product_step2':
$prd_id=(int)$FREQUEST->getvalue('add_product_products_id');
$products_query=tep_db_query("select is_attributes,products_price_break from " . TABLE_PRODUCTS . " where products_id='" . $prd_id . "'");
$products_result=tep_db_fetch_array($products_query);
$pattrb='{enabled:false,count:0}';
$ppbreaks='{enabled:false}';
if($products_result['is_attributes']=='Y')
$pattrb=set_product_values($prd_id,false);
if($products_result['products_price_break'] =='Y')
$ppbreaks=tep_get_products_breaks($prd_id);
$JS_DETAILS=array();
echo 'new_product_step2||' . $prd_id . '{@@}' . tep_get_products_stock($prd_id) . '{@@}' . $ppbreaks . '{@@}' . $pattrb . '{@@}' . "{symbolLeft:'" .$currencies->currencies[$currency]['symbol_left'] . "',symbolRight:'" . $currencies->currencies[$currency]['symbol_right'] . "'}" . '||';
out_product_details($prd_id);
echo '||' . $JS_DETAILS["SALEMAKER_DATAS"];
break;
case 'new_event':
require(FILENAME_ADDEVENTS_NEW);
break;
case 'new_event_step2':
$event_id=(int)$FREQUEST->getvalue('event_id');
$show_period=(isset($FGET["show_period"])?true:false);
$override=(isset($FGET["override_reserve"])?true:false);
$overbook=(isset($FGET["overbook_reserve"])?true:false);
$no_attendees=(int)$FREQUEST->getvalue('no_attendees','int',1);
echo 'new_event_step2||';
out_event_details($event_id,$show_period,$override,$overbook,$no_attendees);
break;
case 'show_attendees':
echo 'show_attendees||';
$no_attendees=(int)$FREQUEST->getvalue('no_attendees','int',1);
out_attendees_details($no_attendees);
break;
case 'check_attendees':
$event_id=(int)$FREQUEST->getvalue('events_id');
$type=$FREQUEST->getvalue('type','int',0);
$sessions=$FREQUEST->getvalue('session');
$no_of_sessions=$FREQUEST->getvalue('type','int',1);
$no_attendees=(int)$FREQUEST->getvalue('no_attendees','int',1);
echo 'check_attendees||';
check_dates($event_id,$no_attendees,$type,$sessions);
break;
case 'new_subscription':
require(FILENAME_ADDSUBSCRIPTIONS_NEW);
break;
case 'new_subscription_step2':
$subs_id=(int)$FREQUEST->getvalue('subscriptions_id');
echo 'new_subscription_step2||';
out_subscription_details($subs_id);
break;
case 'new_service':
require(FILENAME_ADDSERVICES_NEW);
break;
case 'new_service_step2':
$service_id=(int)$FREQUEST->getvalue('service_id');
echo 'new_service_step2||';
out_service_details($service_id);
break;
case 'new_service_step3':
$service_id=(int)$FREQUEST->getvalue('service_id');
echo 'new_service_step3||';
out_service_details_step3($service_id);
break;
case 'show_shipping_details':
case 'submit_shipping_details':
echo "checkout_shipping_details||";
require(FILENAME_CHECKOUT_SHIPPING);
break;
case 'show_payment_details':
echo "checkout_payment_details||";
require(FILENAME_CHECKOUT_PAYMENT_NEW);
break;
case 'submit_payment_details':
case 'coupon_details':
$GLOBALS["EXECUTE_PAYMENT"]=1;
echo "payment_confirm||";
require(FILENAME_CHECKOUT_CONFIRM_NEW);
break;
case 'show_payment_address':
//manual price adjustment
$modify_price_prefix=$FREQUEST->getvalue('modify_price_prefix');
$sign=$FREQUEST->getvalue('sign');
$purpose=$FREQUEST->getvalue('purpose');
$manual_option=$FREQUEST->getvalue('manual_option');
if($sign=='plus') {
$sign='+'; }
else {
$sign='-';
$modify_price_prefix=$modify_price_
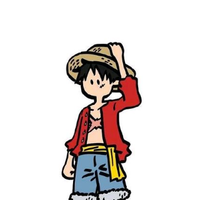
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
最新资源
- 串口转网络模块源码,uart tcp ip 以太网关模块 stm32f107主控,曾经量产过 pcb ad10工程 mcu keil工程 上位机 vc6工程 提供所有设计文件源码,可学习,可生
- MW54 微型涡喷发动机 涡轮喷气发动机 平面图纸+三维建模,文件格式是STP,通用格
- 码垛机图纸,伺料码垛机图纸,腻子粉码垛机图纸,可借鉴学习,参考设计
- 共90套左右各类污水处理设备三维模型,管道设备三维模型,石油化工设备三维模型 sw打开,大部分是可以编辑修改尺寸的 有装配体模型,有零部件模型
- springboot 集成 lemon-imui vue tio 完成即时通讯
- 30天开发操作系统 第 13 天 - 定时器 v2.0
- 基于React框架的Airbnb风格民宿租赁门户网站设计源码
- 燃料电池汽车Cruise整车仿真模型(燃料电池电电混动整车仿真模型) 1.基于Cruise与MATLAB Simulink联合仿真完成整个模型搭建,策略为多点恒功率(多点功率跟随)式控制策略,策略模
- 基于Vue3、uniapp的树洞公众号语聊搭子陪玩社交社区论坛礼物特效IM聊天系统设计源码
- comsol 离散裂隙 两相流模型
- FLAC3D后处理,将云图转为三维,可视化更强 图一为flac原图,图二图三为处理后的图 内容包括:案例文件,fish代码和matlab代码
- 汽车BCM程序源代码 国产车BCM程序源代码 外部灯光:前照灯、小灯、转向灯、前后雾灯、日间行车灯、倒车灯、制动灯、角灯、泊车灯等 内部灯光:顶灯、钥匙光圈、门灯 前后雨
- 纯电动汽车仿真、纯电动公交、纯电动客车、纯电动汽车动力性仿真、经济性仿真、续航里程仿真 模型包括电机、电池、车辆模型 有两种模型2选1: 1 完全用matlab simulink搭建的模型 2用
- 电机控制器,两种基于滑模观测器的PMSM无感矢量控制仿真(开关设置区分): 1. PLL+滑模(降低高频开关噪声); 2. arctan+滑模; 有配套算法原理资料
- 包含光热电站的综合能源系统优化运行规划(MATLAB+cplex) 采用Matlab程序Yalmip+Cplex求解 系统中包含电、热、冷、气 系统中机组有:风力,光伏,燃气轮机,P2G, 电制冷,O
- 双馈风力发电系统模型 Matlab simulink仿真运行 可直接跑
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


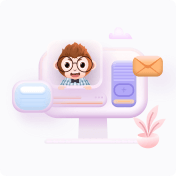