<?php
// include the list of project database tables
require('includes/database_tables.php');
define('USE_SEO_REDIRECT_DEBUG', 'false');
/**
* Ultimate SEO URLs Contribution - osCommerce MS-2.2
*
* Ultimate SEO URLs offers search engine optimized URLS for osCommerce
* based applications. Other features include optimized performance and
* automatic redirect script.
* @package Ultimate-SEO-URLs
* @license http://opensource.org/licenses/gpl-license.php GNU Public License
* @version 2.1
* @link http://www.oscommerce-freelancers.com/ osCommerce-Freelancers
* @copyright Copyright 2005, Bobby Easland
* @author Bobby Easland
* @filesource
*/
/**
* SEO_DataBase Class
*
* The SEO_DataBase class provides abstraction so the databaes can be accessed
* without having to use tep API functions. This class has minimal error handling
* so make sure your code is tight!
* @package Ultimate-SEO-URLs
* @license http://opensource.org/licenses/gpl-license.php GNU Public License
* @version 1.1
* @link http://www.oscommerce-freelancers.com/ osCommerce-Freelancers
* @copyright Copyright 2005, Bobby Easland
* @author Bobby Easland
*/
class SEO_DataBase{
/**
* Database host (localhost, IP based, etc)
* @var string
*/
var $host;
/**
* Database user
* @var string
*/
var $user;
/**
* Database name
* @var string
*/
var $db;
/**
* Database password
* @var string
*/
var $pass;
/**
* Database link
* @var resource
*/
var $link_id;
/**
* MySQL_DataBase class constructor
* @author Bobby Easland
* @version 1.0
* @param string $host
* @param string $user
* @param string $db
* @param string $pass
*/
function SEO_DataBase($host, $user, $db, $pass){
$this->host = $host;
$this->user = $user;
$this->db = $db;
$this->pass = $pass;
$this->ConnectDB();
$this->SelectDB();
} # end function
/**
* Function to connect to MySQL
* @author Bobby Easland
* @version 1.1
*/
function ConnectDB(){
$this->link_id = mysql_connect($this->host, $this->user, $this->pass);
} # end function
/**
* Function to select the database
* @author Bobby Easland
* @version 1.0
* @return resoource
*/
function SelectDB(){
return mysql_select_db($this->db);
} # end function
/**
* Function to perform queries
* @author Bobby Easland
* @version 1.0
* @param string $query SQL statement
* @return resource
*/
function Query($query){
return @mysql_query($query, $this->link_id);
} # end function
/**
* Function to fetch array
* @author Bobby Easland
* @version 1.0
* @param resource $resource_id
* @param string $type MYSQL_BOTH or MYSQL_ASSOC
* @return array
*/
function FetchArray($resource_id, $type = MYSQL_BOTH){
return @mysql_fetch_array($resource_id, $type);
} # end function
/**
* Function to fetch the number of rows
* @author Bobby Easland
* @version 1.0
* @param resource $resource_id
* @return mixed
*/
function NumRows($resource_id){
return @mysql_num_rows($resource_id);
} # end function
/**
* Function to fetch the last insertID
* @author Bobby Easland
* @version 1.0
* @return integer
*/
function InsertID() {
return mysql_insert_id();
}
/**
* Function to free the resource
* @author Bobby Easland
* @version 1.0
* @param resource $resource_id
* @return boolean
*/
function Free($resource_id){
return @mysql_free_result($resource_id);
} # end function
/**
* Function to add slashes
* @author Bobby Easland
* @version 1.0
* @param string $data
* @return string
*/
function Slashes($data){
return addslashes($data);
} # end function
/**
* Function to perform DB inserts and updates - abstracted from osCommerce-MS-2.2 project
* @author Bobby Easland
* @version 1.0
* @param string $table Database table
* @param array $data Associative array of columns / values
* @param string $action insert or update
* @param string $parameters
* @return resource
*/
function DBPerform($table, $data, $action = 'insert', $parameters = '') {
reset($data);
if ($action == 'insert') {
$query = 'INSERT INTO `' . $table . '` (';
while (list($columns, ) = each($data)) {
$query .= '`' . $columns . '`, ';
}
$query = substr($query, 0, -2) . ') values (';
reset($data);
while (list(, $value) = each($data)) {
switch ((string)$value) {
case 'now()':
$query .= 'now(), ';
break;
case 'null':
$query .= 'null, ';
break;
default:
$query .= "'" . $this->Slashes($value) . "', ";
break;
}
}
$query = substr($query, 0, -2) . ')';
} elseif ($action == 'update') {
$query = 'UPDATE `' . $table . '` SET ';
while (list($columns, $value) = each($data)) {
switch ((string)$value) {
case 'now()':
$query .= '`' .$columns . '`=now(), ';
break;
case 'null':
$query .= '`' .$columns .= '`=null, ';
break;
default:
$query .= '`' .$columns . "`='" . $this->Slashes($value) . "', ";
break;
}
}
$query = substr($query, 0, -2) . ' WHERE ' . $parameters;
}
return $this->Query($query);
} # end function
} # end class
/**
* Ultimate SEO URLs Installer and Configuration Class
*
* Ultimate SEO URLs installer and configuration class offers a modular
* and easy to manage method of configuration. The class enables the base
* class to be configured and installed on the fly without the hassle of
* calling additional scripts or executing SQL.
* @package Ultimate-SEO-URLs
* @license http://opensource.org/licenses/gpl-license.php GNU Public License
* @version 1.1
* @link http://www.oscommerce-freelancers.com/ osCommerce-Freelancers
* @copyright Copyright 2005, Bobby Easland
* @author Bobby Easland
*/
class SEO_URL_INSTALLER{
/**
* The default_config array has all the default settings which should be all that is needed to make the base class work.
* @var array
*/
var $default_config;
/**
* Database object
* @var object
*/
var $DB;
/**
* $attributes array holds information about this instance
* @var array
*/
var $attributes;
/**
* SEO_URL_INSTALLER class constructor
* @author Bobby Easland
* @version 1.1
*/
function SEO_URL_INSTALLER(){
$this->attributes = array();
$x = 0;
$this->default_config = array();
$this->default_config['SEO_ENABLED'] = array('DEFAULT' => 'true',
'QUERY' => "INSERT INTO `".TABLE_CONFIGURATION."` VALUES ('', 'Enable SEO URLs?', 'SEO_ENABLED', 'false', 'Enable the SEO URLs? This is a global setting and will turn them off completely.', 16, ".$x.", NOW(), NOW(), NULL, 'tep_cfg_select_option(array(''true'', ''false''),')"
);
$x++;
$this->default_config['SEO_ADD_CPATH_TO_PRODUCT_URLS'] = array('DEFAULT' => 'false',
'QUERY' => "INSERT INTO `".TABLE_CONFIGURATION."` VALUES ('', 'Add cPath to product URLs?', 'SEO_ADD_CPATH_TO_PRODUCT_URLS', 'false', 'This setting will append the cPath to the end of product URLs (i.e. - some-product-p-1.html?cPath=xx).', 16, ".$x.", NOW(), NOW(), NULL, 'tep_cfg_select_option(array(''true'', ''false''),')"
);
$x++;
$this->default_config['SEO_ADD_CAT_PARENT'] = array('DEFAULT' => 'true',
'QUERY' => "INSERT INTO `".TABLE_CONFIGURATION."` VALUES ('', 'Add category parent to begining of URLs?', 'SEO_ADD_CAT_PARENT', 'true', 'This setting will add the category parent name to the beginning of the category URLs (i.e. - parent-category-c-1.html).', 16, ".$x.", NOW(), NOW(), NULL, 'tep_cfg_select_option(array(''true'', ''false''),')"
);
$x++;
$this->default_config['SEO_URLS_FILTER_SHORT_WORDS'] = array('DEFAULT' => '3',
'QUERY' => "INSERT INTO `".TABLE_CONFIGURATION."` VALUES ('', 'Filter Short Words', 'SEO_URLS_F
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
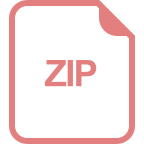
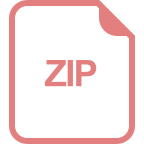
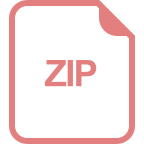
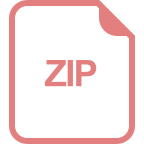
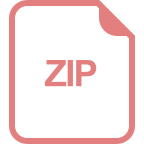
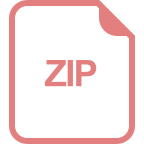
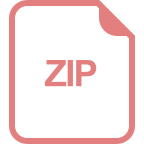
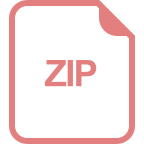
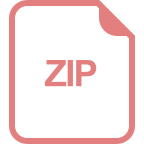
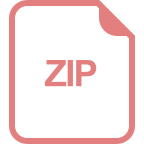
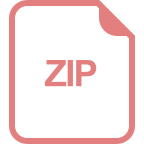
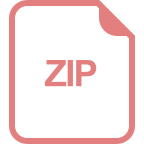
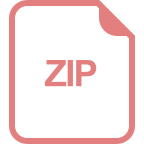
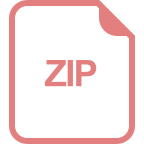
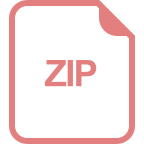
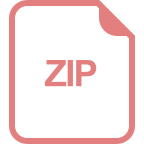
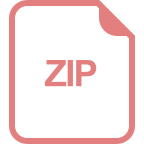
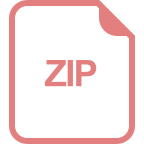
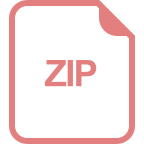
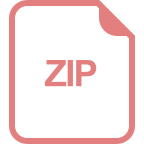
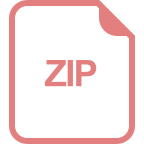
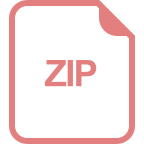
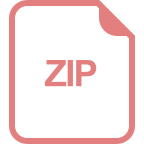
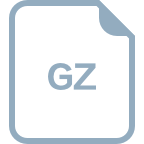
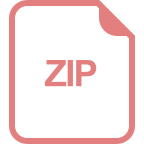
收起资源包目录

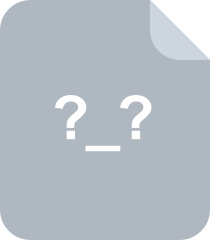
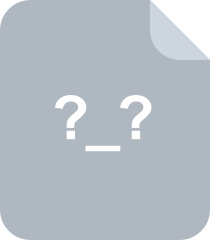
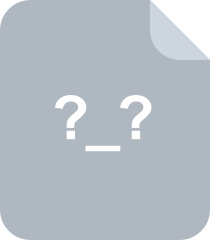
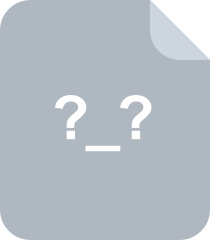
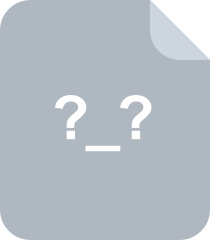
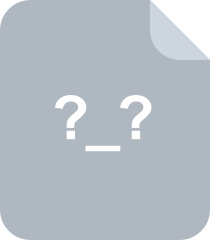
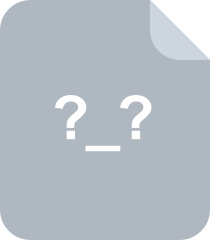
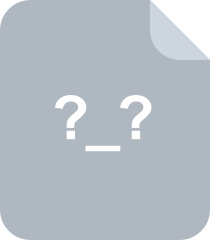
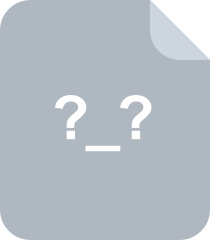
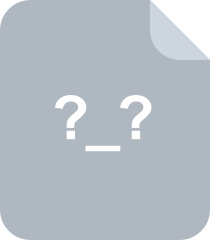
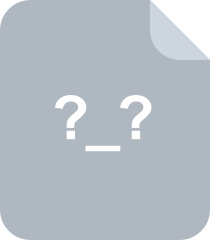
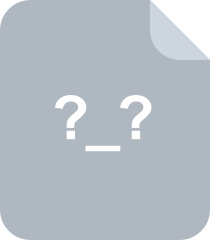
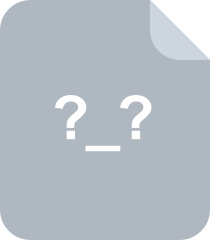
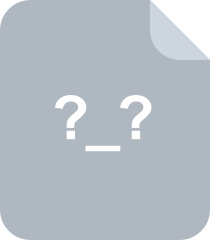
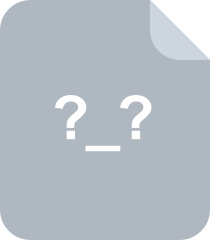
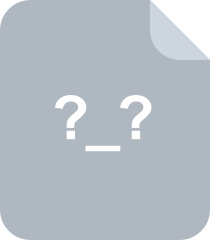
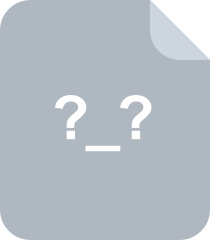
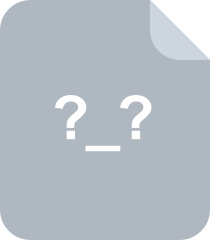
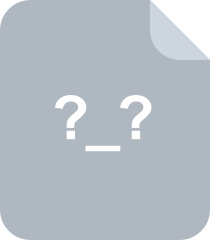
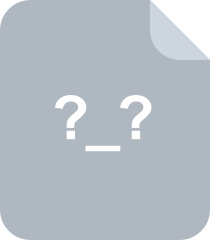
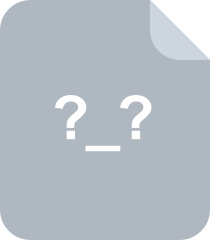
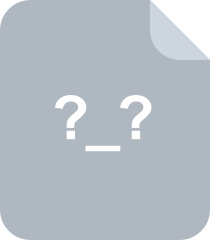
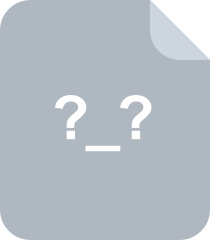
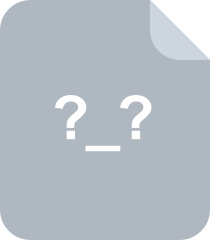
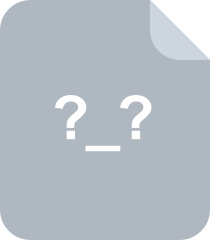
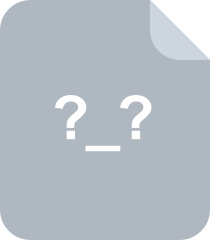
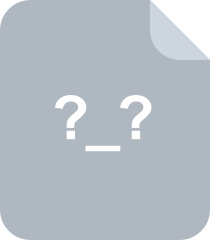
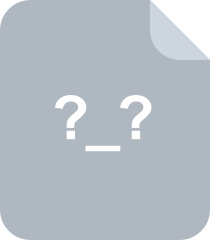
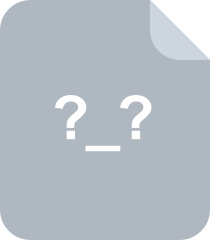
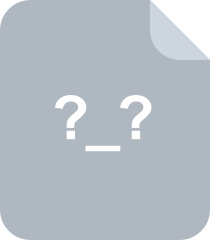
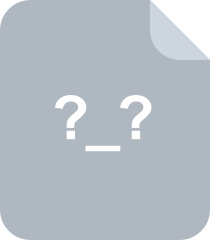
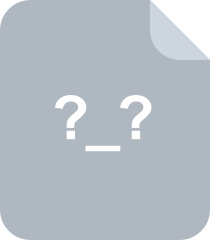
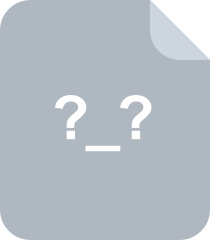
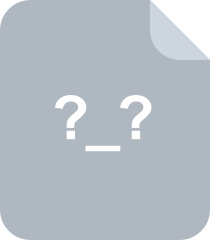
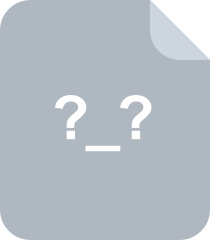
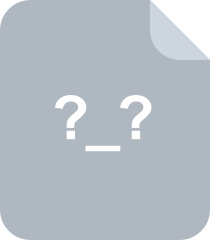
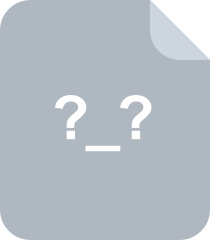
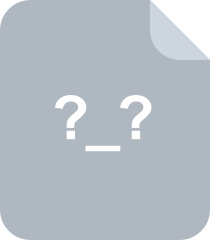
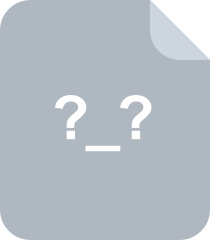
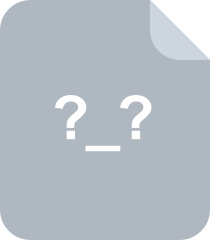
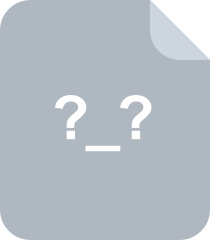
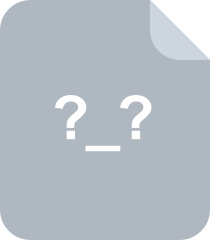
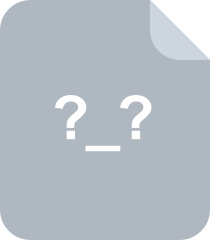
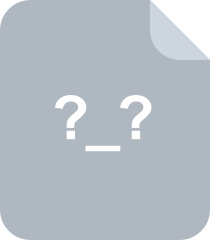
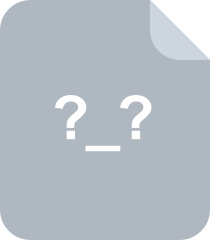
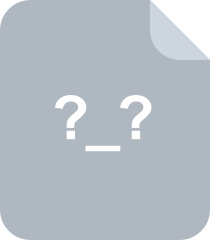
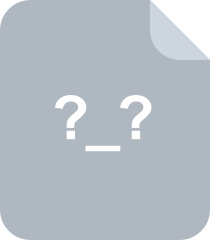
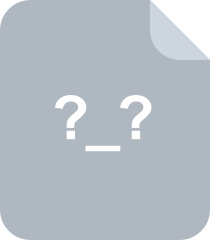
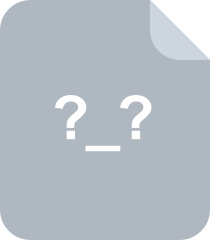
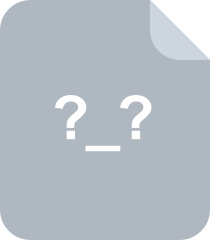
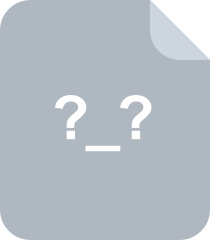
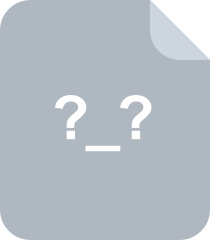
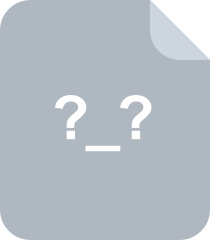
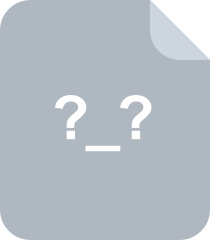
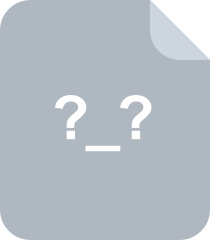
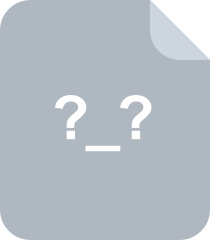
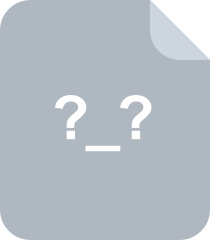
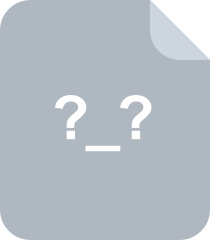
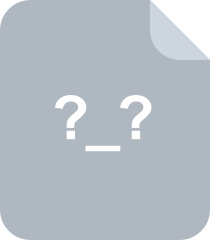
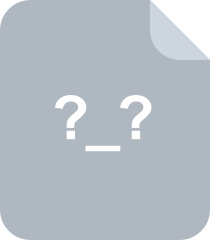
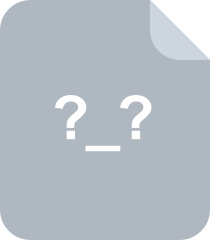
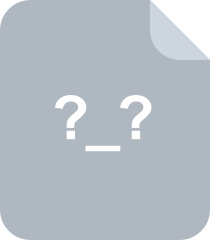
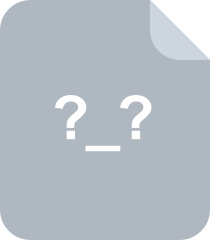
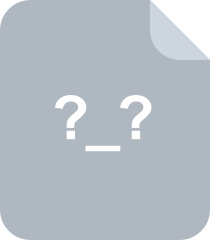
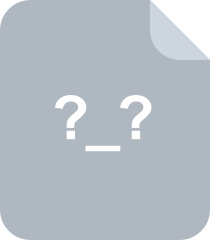
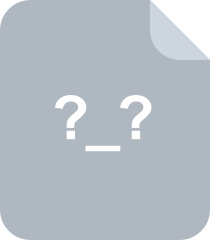
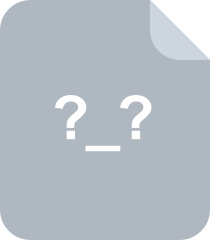
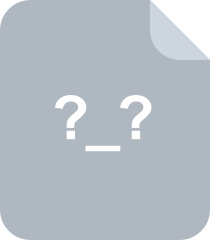
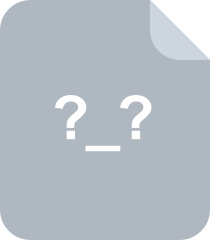
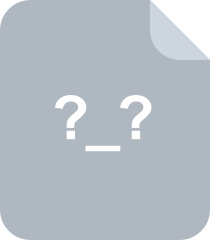
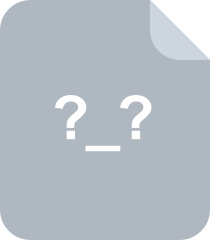
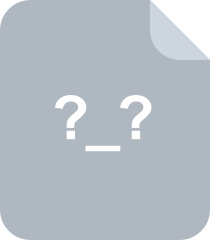
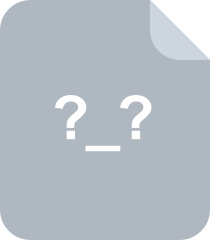
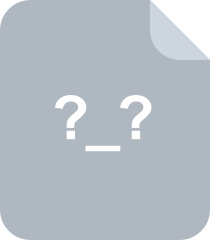
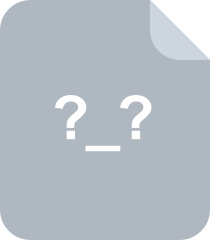
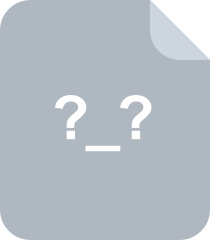
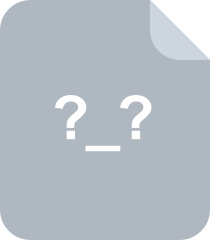
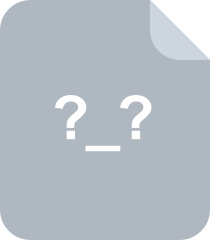
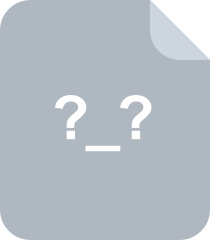
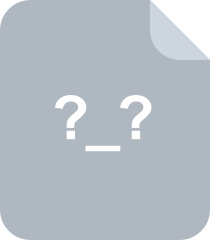
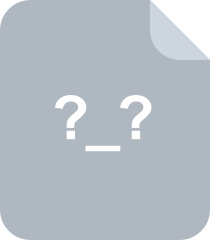
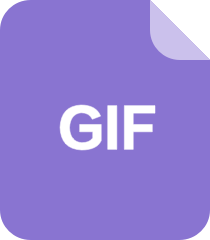
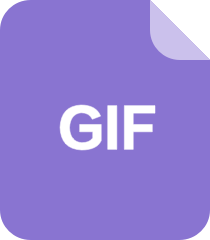
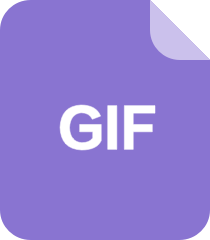
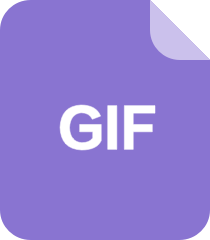
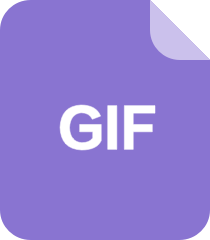
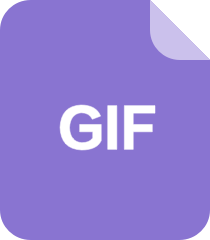
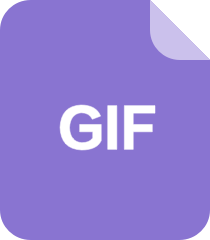
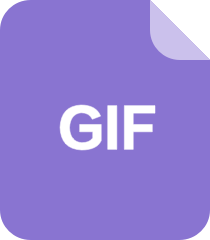
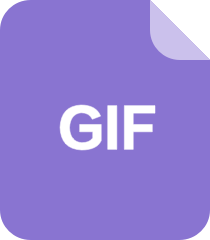
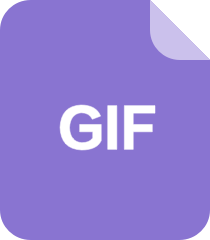
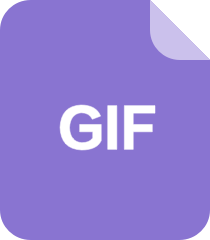
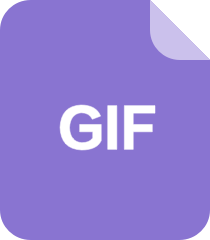
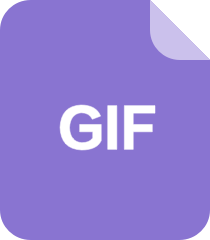
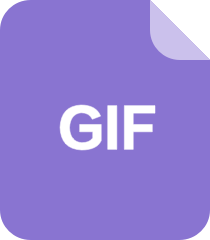
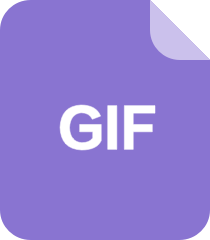
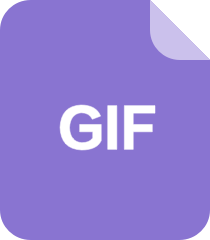
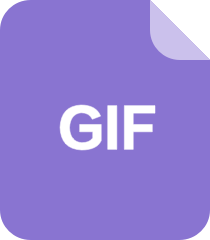
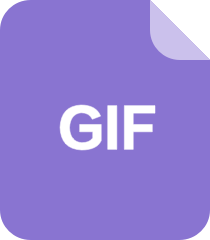
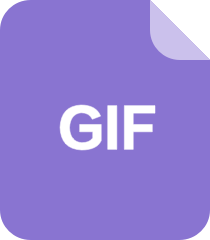
共 1667 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
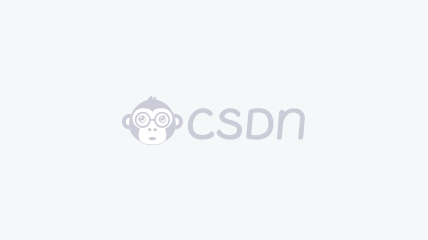
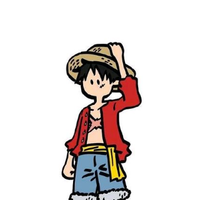
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

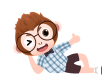
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


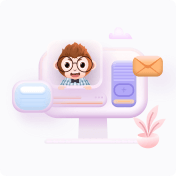
安全验证
文档复制为VIP权益,开通VIP直接复制
