在本文中,我们将深入探讨如何使用Node.js搭建一个静态资源服务器,主要关注`fs`模块、`util.promisify`以及`handlebars`模板引擎。让我们了解Node.js的基础知识,然后逐步解析上述提到的各个组件。 Node.js是一个基于Chrome V8引擎的JavaScript运行环境,它允许开发者使用JavaScript在服务器端编写高性能的网络应用程序。由于JavaScript是客户端脚本语言,Node.js的出现使得前后端开发可以使用同一种语言,提高了开发效率。 **1. Node.js中的fs模块** `fs`(文件系统)模块是Node.js的核心模块之一,用于处理文件和目录操作。例如,你可以读取、写入、创建、删除和监控文件或目录。在搭建静态资源服务器时,`fs`模块用于读取服务器上存储的HTML、CSS、JavaScript等静态文件,并将它们发送到客户端。 ```javascript const fs = require('fs'); fs.readFile('./index.html', 'utf8', (err, data) => { if (err) throw err; console.log(data); }); ``` **2. util.promisify** 在Node.js v8.0.0及以上版本中,`util.promisify`函数被引入,用于将旧式的异步回调函数转换为返回Promise的新风格。这在处理像`fs`模块中许多未返回Promise的方法时非常有用,因为它使代码更容易理解和维护。例如,我们可以使用`util.promisify`将`fs.readFile`转换为返回Promise的版本: ```javascript const { promisify } = require('util'); const readFile = promisify(fs.readFile); readFile('./index.html', 'utf8') .then(data => console.log(data)) .catch(err => console.error(err)); ``` **3. handlebars模板引擎** `handlebars`是一个强大的JavaScript模板引擎,它允许我们在HTML中插入动态数据。在服务器端,我们可以通过`handlebars`预编译模板并生成HTML字符串,然后发送给客户端。这样,我们可以将数据与结构分离,提高代码的可维护性。 我们需要安装`handlebars`库: ```bash npm install handlebars ``` 然后,我们可以创建一个模板文件`template.hbs`,并使用`handlebars.compile`编译模板: ```javascript const Handlebars = require('handlebars'); const fs = require('fs'); const templateSource = fs.readFileSync('./template.hbs', 'utf8'); const template = Handlebars.compile(templateSource); const data = { title: 'Hello, World!' }; const html = template(data); console.log(html); ``` **4. 构建静态资源服务器** 结合以上知识,我们可以构建一个简单的静态资源服务器,使用`http`模块创建服务器,监听请求,并使用`fs`和`util.promisify`读取静态文件。如果需要使用模板引擎,可以在接收到特定请求时动态生成HTML。 ```javascript const http = require('http'); const { promisify } = require('util'); const fs = require('fs'); const path = require('path'); const Handlebars = require('handlebars'); // 预编译模板 const templateSource = fs.readFileSync('./template.hbs', 'utf8'); const template = Handlebars.compile(templateSource); const server = http.createServer(async (req, res) => { const filePath = path.join(__dirname, req.url); try { // 如果是HTML请求,使用模板引擎 if (filePath.endsWith('.hbs')) { const data = { title: 'My Static Site' }; const html = template(data); res.writeHead(200, { 'Content-Type': 'text/html' }); res.end(html); } else { // 否则,读取静态文件 const fileStat = await promisify(fs.stat)(filePath); if (fileStat.isDirectory()) { res.writeHead(302, { Location: '/index.html' }); res.end(); } else { const fileContent = await promisify(fs.readFile)(filePath); const contentType = mime.getType(filePath); res.writeHead(200, { 'Content-Type': contentType }); res.end(fileContent); } } } catch (err) { res.writeHead(404); res.end(`File not found: ${filePath}`); } }); server.listen(3000, () => { console.log('Server running on port 3000'); }); ``` 以上代码创建了一个简单的静态资源服务器,它可以处理HTML模板请求和静态文件请求。当用户访问`.hbs`文件时,服务器会使用`handlebars`渲染模板;对于其他文件,服务器会直接返回文件内容。 总结,通过Node.js、`fs`模块、`util.promisify`和`handlebars`模板引擎,我们可以快速搭建一个静态资源服务器,为用户提供HTML、CSS、JavaScript等静态文件。这种设置在开发阶段特别有用,因为它允许快速迭代和调试,同时也适用于小型项目或作为大型应用程序的本地开发环境。
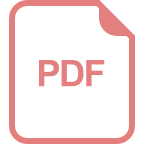
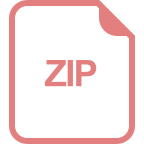
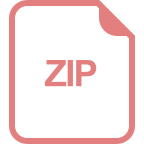
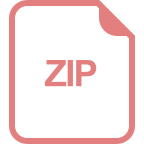
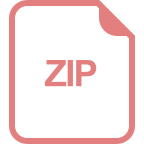
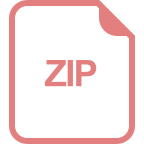
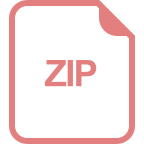
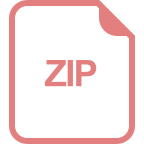
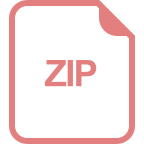
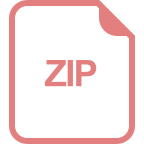
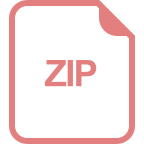
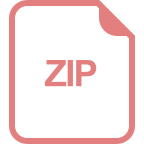
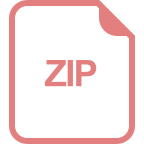
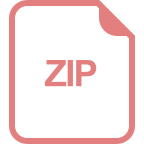
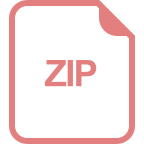
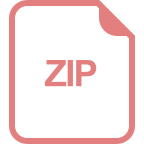
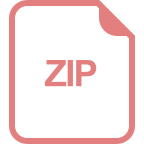
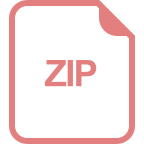
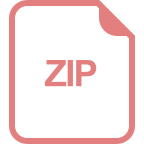
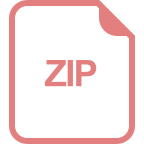
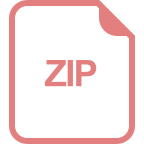
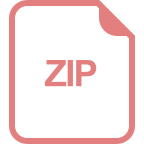
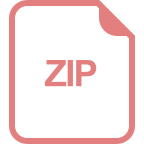
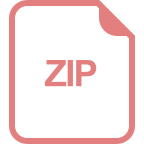
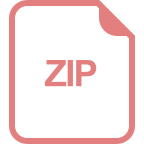
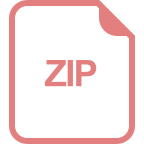



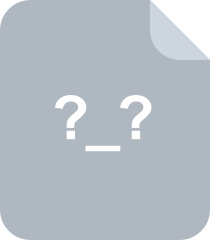
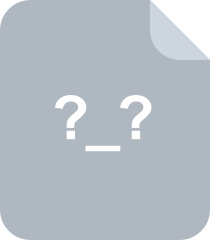
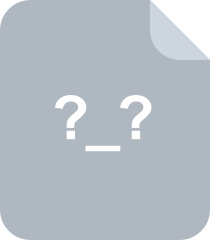

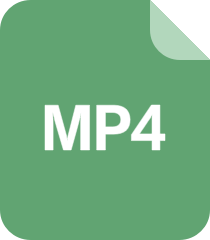

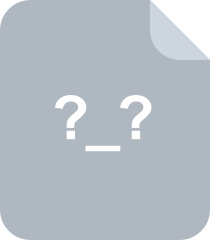

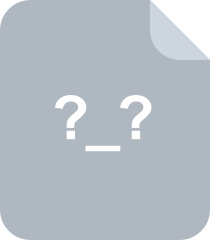

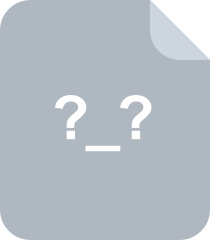

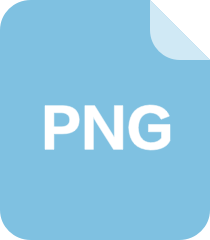
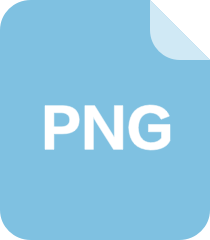
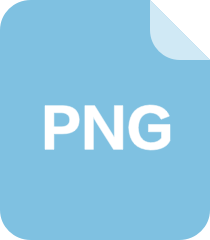
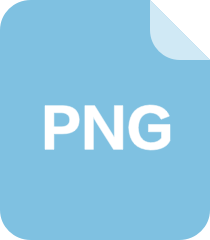
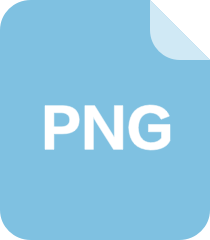
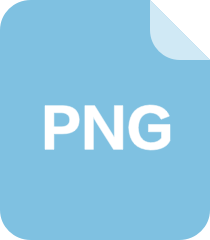
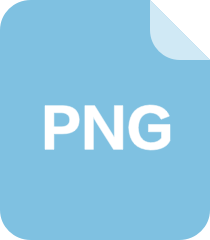
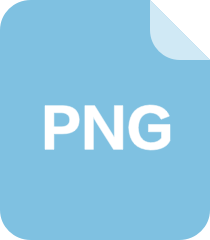
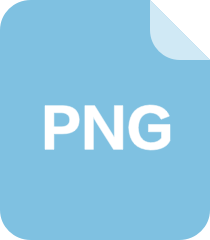

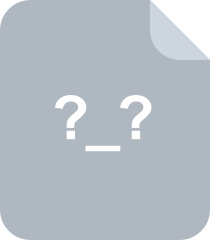

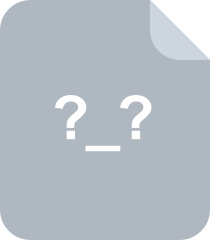
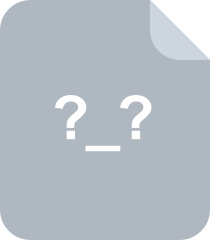
- 1
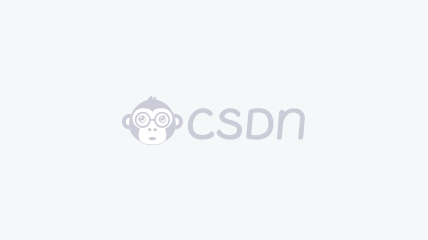

- 粉丝: 1
- 资源: 9
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

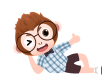
最新资源

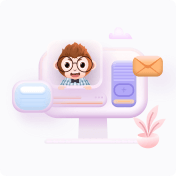
