# amCharts Export
Version: 1.1.3
## Description
This plugin adds export capabilities to all amCharts products - charts and maps.
It allows annotating and exporting chart or related data to various bitmap,
vector, document or data formats, such as PNG, JPG, PDF, SVG, JSON, XLSX and
many more.
## Important notice
Please note that due to security measures implemented in modern browsers, some
or all export options might not work if the web page is loaded locally (via
file:///) or contain images loaded from different host than the web page itself.
## Usage
### 1) Include the minified version of file of this plugin as well as the
bundled CSS file. I.e.:
```
<script src="amcharts/plugins/export/export.min.js"></script>
<link type="text/css" href="amcharts/plugins/export/export.css" rel="stylesheet">
```
Or if you'd rather use amCharts CDN:
```
<script src="//cdn.amcharts.com/lib/3/plugins/export/export.min.js"></script>
<link type="text/css" href="//cdn.amcharts.com/lib/3/plugins/export/export.css" rel="stylesheet">
```
(this needs to go after all the other amCharts includes)
### 2) Enable `export` with default options:
```
AmCharts.makeChart( "chartdiv", {
...,
"export": {
"enabled": true
}
} );
```
### ... OR set your own custom options:
```
AmCharts.makeChart( "chartdiv", {
...,
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download",
"menu": [ "PNG", "JPG", "CSV" ]
}, {
"label": "Annotate",
"action": "draw",
"menu": [ {
"class": "export-drawing",
"menu": [ "PNG", "JPG" ]
} ]
} ]
} ]
}
} );
```
## Loading external libraries needed for operation of this plugin
The plugin relies on a number of different libraries, to export images, draw
annotations or generate download files.
Those libraries need to be loaded for the plugin to work properly.
There are two ways to load them. Choose the one that is right:
### 1) Automatic (preferred)
All libraries required for plugin operation are included withing plugins */libs*
subdirectory.
If you want the plugin to load them on-demand (when it's needed for a certain
operation), make sure you've set the [`path`](http://docs.amcharts.com/3/javascriptcharts/AmSerialChart#path) property in your chart setup.
If you are using relative url, note that it is relative to the web page you are
displaying your chart on, not the export.js library.
In case you've moved the libs folder you need to tell the plugin where it is
`"libs": { "path": "../libs/" }`
### 2) Manual
You can also load all those JavaScript libraries by `<script>` tags. Since
loading of libraries is on by default you will need to turn it off by setting
`"libs": { "autoLoad": false }`
Here is a full list of the files that need to be loaded for each operation:
File | Located in | Required for
---- | ---------- | ------------
fabric.min.js | libs/fabric.js/ | Any export operation
FileSaver.js | libs/FileSaver.js/ | Used to offer download files
pdfmake.min.js | libs/pdfmake/ | Export to PDF format
vfs_fonts.js | libs/pdfmake/ | Export to PDF format
jszip.js | libs/jszip/ | Export to XLSX format
xlsx.js | libs/xlsx/ | Export to XLSX format
## Complete list of available export settings
Property | Default | Description
-------- | ------- | -----------
backgroundColor | #FFFFFF | RGB code of the color for the background of the exported image
enabled | true | Enables or disables export functionality
divId | | ID or a reference to div object in case you want the menu in a separate container.
fabric | {} | Overwrites the default drawing settings (fabricJS library)
fallback | {} | Holds the messages to guide the user to copy the generated output; `false` will disable the fallback feature
fileName | amCharts | A file name to use for generated export files (an extension will be appended to it based on the export format)
legend | {} | Places the legend in case it is within an external container
libs | | 3rd party required library settings (see the above section)
menu | [] | A list of menu or submenu items (see the next chapter for details)
pdfMake | {} | Overwrites the default settings for PDF export (pdfMake library)
position | top-right | A position of export icon. Possible values: "top-left", "top-right" (default), "bottom-left", "bottom-right"
removeImages | true | If true export checks for and removes "tainted" images that area lodead from different domains
## Configuring export menu
Plugin includes a way to completely control what is displayed on export menu.
You can set up menus, sub-menus, down to any level. You can even add custom
items there that execute your arbitrary code on click. It's so configurable
it makes us sick with power ;)
The top-level menu is configured via `menu` property under `export`. It should
always be an array, even if you have a single item in it.
The array items could be either objects or format codes. Objects will allow you
to specify labels, action, icon, child items and even custom code to be executed
on click.
Simple format codes will assume you need an export to that format.
### Simple menu setup
Here's a sample of the simple menu setup that allows export to PNG, JPG and CSV:
```
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ "PNG", "JPG", "CSV" ]
} ]
}
```
The above will display a menu out of three options when you hover on export
icon:
* PNG
* JPG
* CSV
When clicked the plugin will trigger export to a respective format.
If that is all you need, you're all set.
Please note that we have wrapped out menu into another menu item, so that only
the icon is displayed until we roll over the icon. This means that technically
we have a two-level hierarchical menu.
If we opmitted that first step, the chart would simply display a format list
right there on the chart.
### Advanced menu setup
However, you can do so much more with the menu.
Let's add more formats and organize image and data formats into separate
submenus.
To add a submenu to a menu item, simply add a `menu` array as its own property:
```
"export": {
"enabled": true,
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "JPG", "SVG" ]
}, {
"label": "Download data",
"menu": [ "CSV", "XLSX" ]
} ]
} ]
}
```
Now we have a hierarchical menu with the following topology:
* Download as image
* PNG
* JPG
* SVG
* Download data
* CSV
* XLSX
We can mix "string" and "object" formats the way we see fit, i.e.:
```
"menu": [
"PNG",
{ "label": "JPEG",
"format": "JPG" },
"SVG"
]
```
The magic does not end here, though.
### Adding custom click events to menu items
Just like we set `label` and `format` properties for menu item, we can set
`click` as well.
This needs to be a function reference. I.e.:
```
"menu": [
"PNG",
{ "label": "JPEG",
"click": function () {
alert( "Clicked JPEG. Wow cool!" );
} },
"SVG"
]
```
### Menu item reviver
By passing the `menuReviver` callback you are to adapt or completely replace the
generated menu item before it gets appended to the list (`ul`).
It retrieves two arguments and it needs to return a valid DOM element.
```
"menuReviver": function(item,li) {
li.setAttribute("class","something special");
return li;
}
```
### Menu walker
In case you don't like our structure, go ahead and write your own recursive function
to create the menu by the given list configured through `menu`.
```
"menuWalker": function(list,container) {
// some magic to generate the nested lists using the given list
}
```
### Printing the chart
Adding menu item to print the chart or map is as easy as adding export ones. You
just use "PRINT" as `format`. I.e.:
```
"menu": [
"PNG",
"SVG",
"PRINT"
]
```
Or if you want to change the label:
```
"menu": [
"PNG",
"SVG",
{
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
使用到的技术:redis、amcharts、maven、html5、ajax、js、jquery以及css,关系型数据库采用的是mysql。 文件夹中有可以直接导入使用的数据库,以及可以导入试卷的excel表格格式. 该项目分为学生模块,和教师模块。 教师模块:教师可以通过导入Excel表格的方式进行添加试卷,如果Excel表中有不合法的数据,会在前台提醒哪一行哪一列出了什么问题,添加试卷后,教师可以发布试卷,试卷发布后,学生就可以答题,每张试卷都有作答时长,作答时间结束,将会自动提交试卷。考试结束后,教师可以发布答案。对于修改试卷,教师可以先选择所要修改的试卷,对于试卷可以修改试卷的名称以及考试时长,要想修改试题可以点击编辑试题,进行批量修改。 学生模块:注册登录进入学生考试平台,选择考卷,进行作答,试卷分为单选题、多选题以及判断题,分值各不相同,对于多选题错答不得分,漏答得一半的分。在作答期间,学生可以先保存,保存的内容存储在Redis中。若点击提交,提交后直接显示成绩。提交后就不能再进入考试。要想看正确答案,得等到考试结束,教师发布成绩后,才可以看到。 学生可以看到自己的作答历史,每道题之前学生的答案以及该题正确的答案都很清晰的标注出来。为了方便学生统计自己的成绩,本系统采用了amcharts技术根据学生的历次成绩制作了柱状图和折线图结合的图表。学生可以很直观地看到自己成绩的波动。
资源推荐
资源详情
资源评论
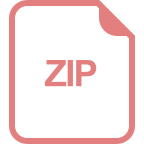
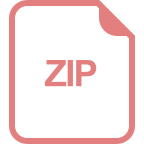
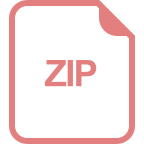
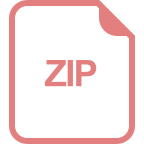
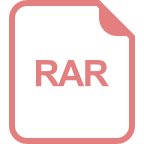
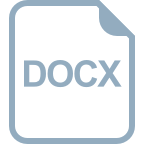
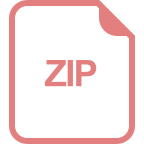
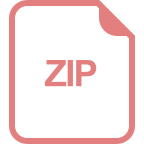
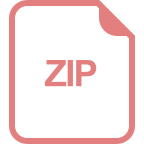
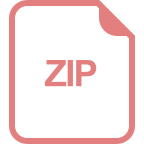
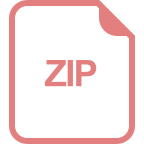
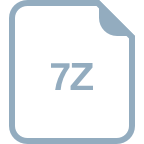
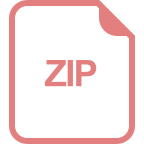
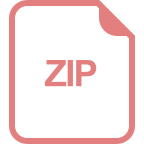
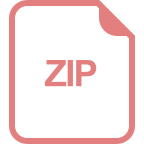
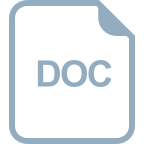
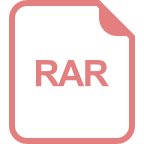
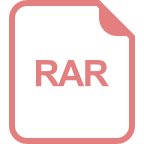
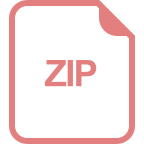
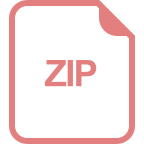
收起资源包目录

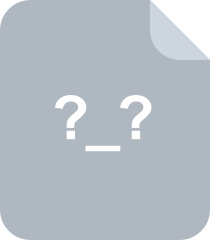
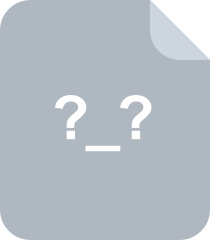
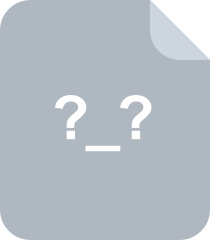
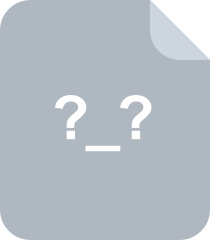
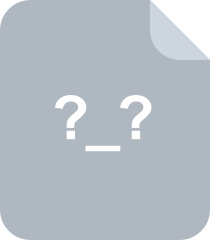
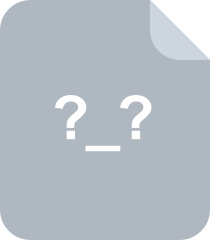
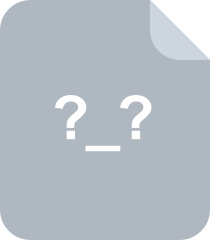
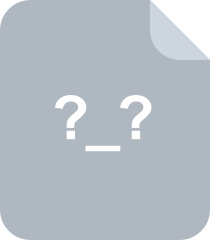
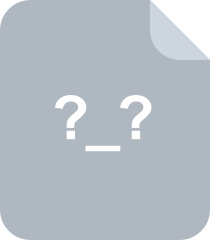
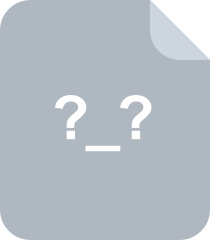
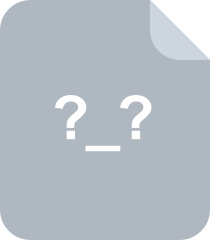
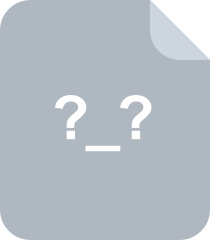
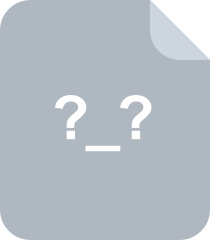
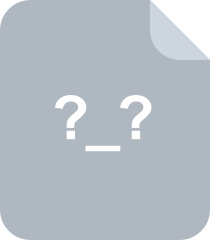
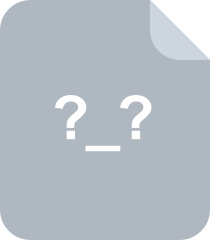
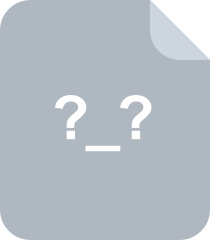
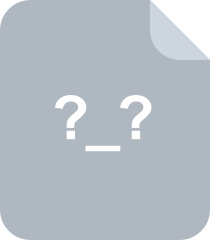
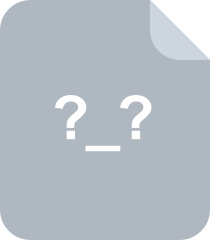
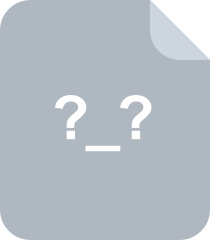
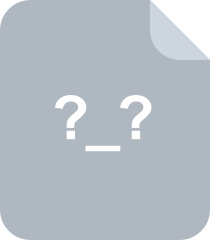
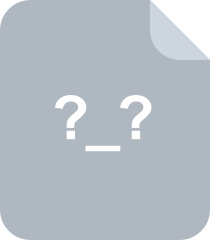
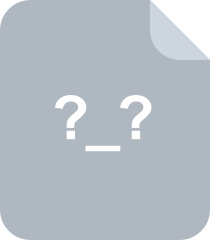
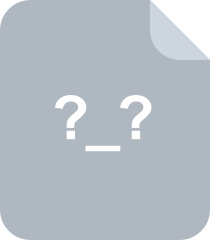
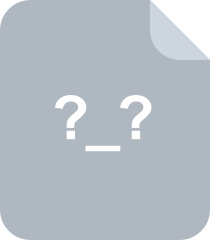
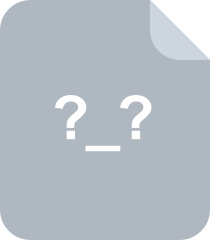
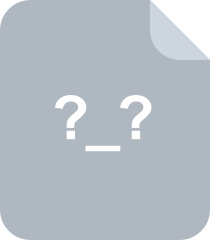
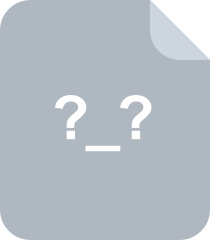
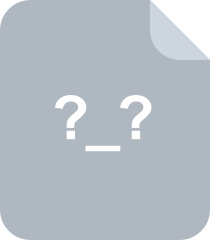
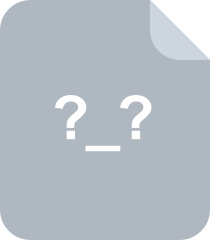
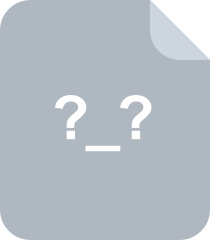
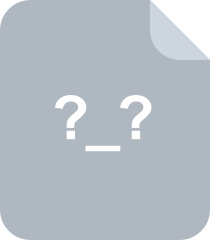
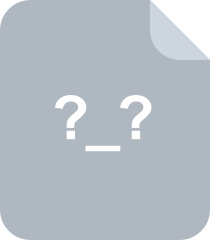
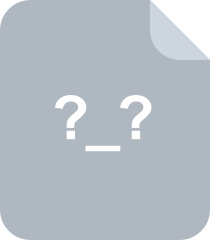
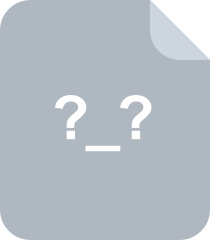
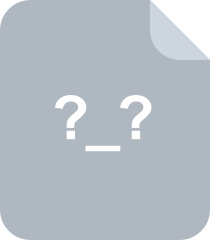
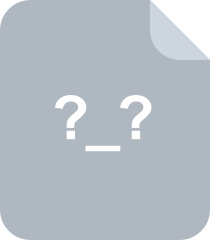
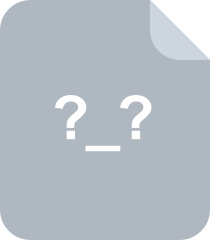
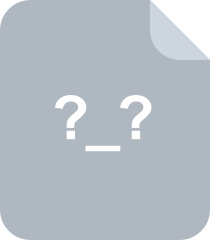
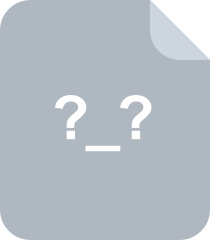
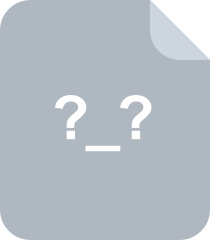
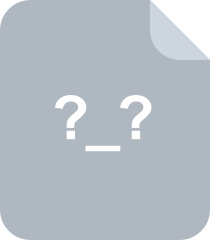
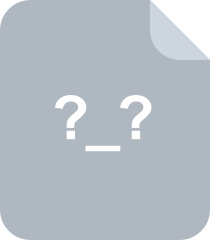
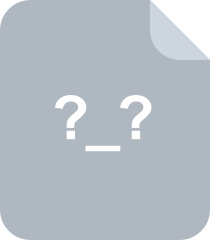
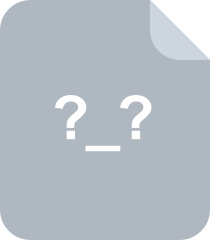
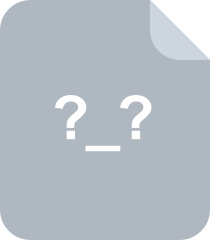
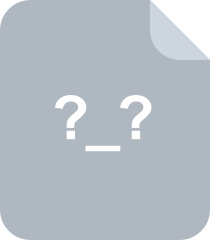
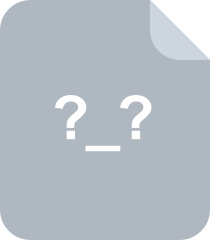
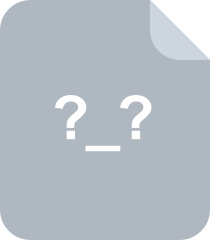
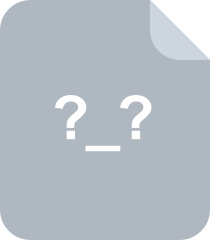
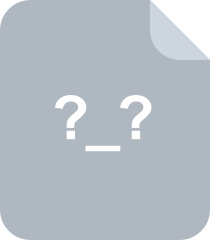
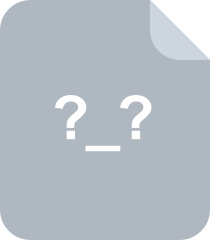
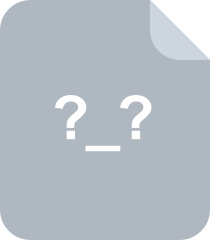
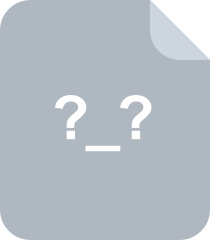
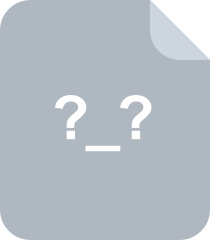
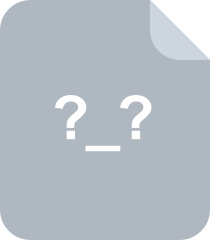
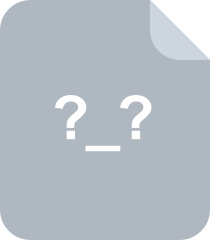
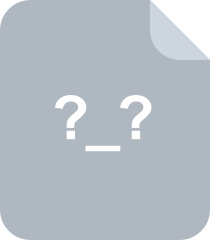
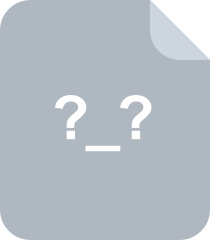
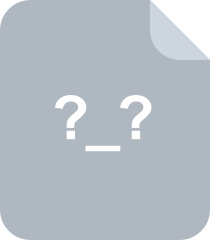
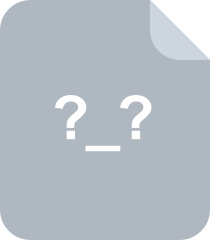
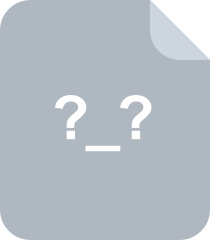
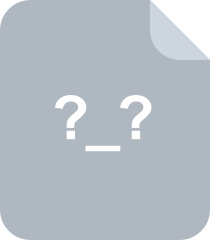
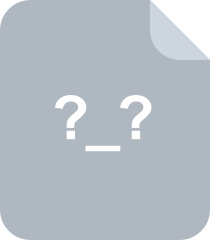
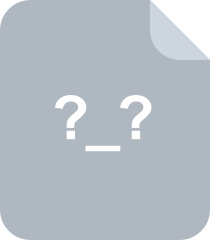
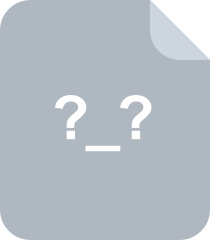
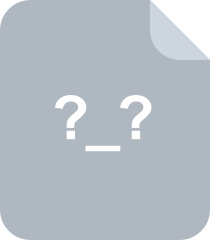
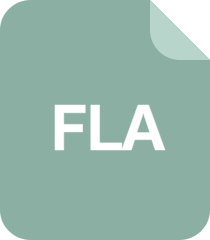
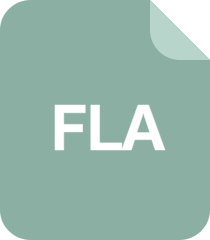
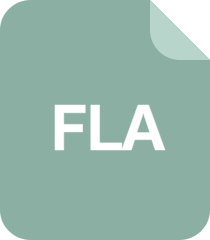
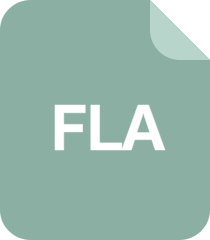
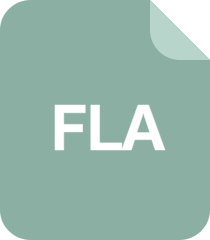
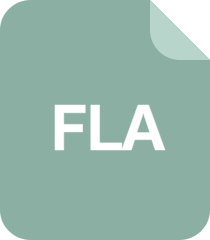
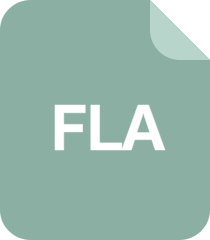
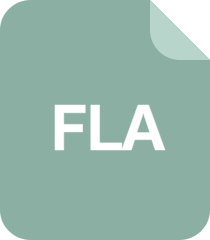
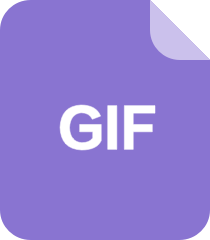
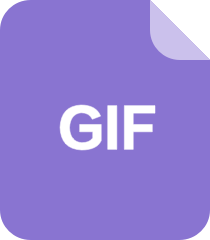
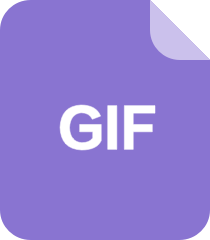
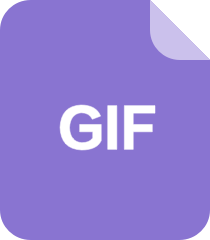
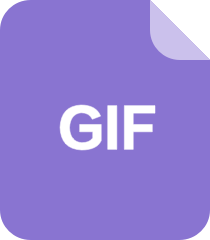
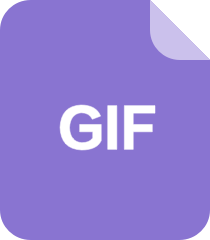
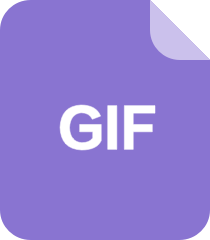
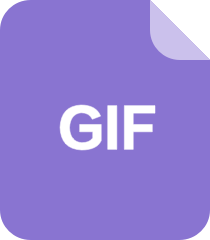
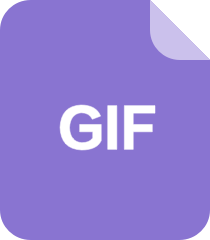
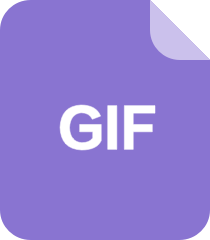
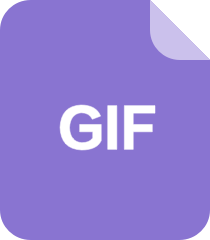
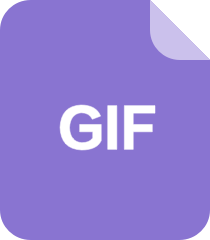
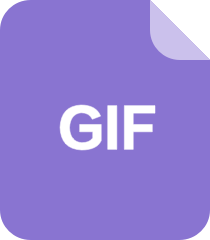
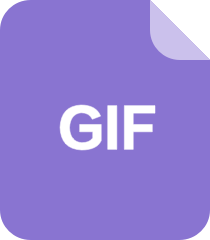
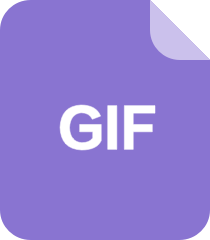
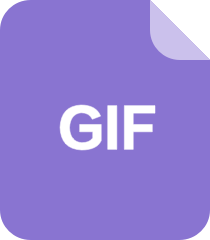
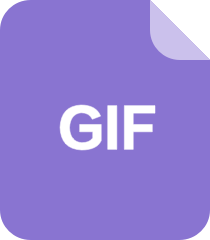
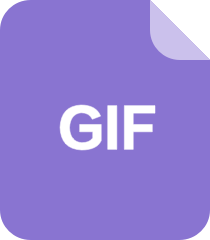
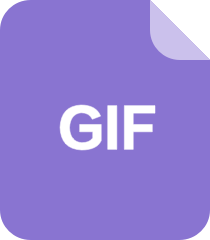
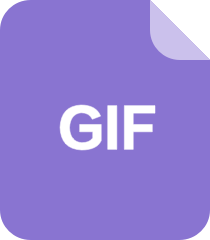
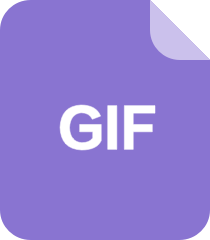
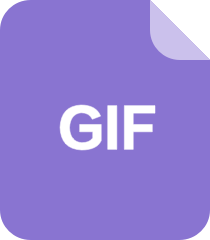
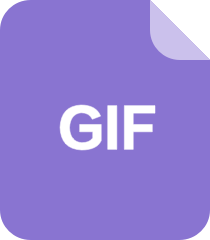
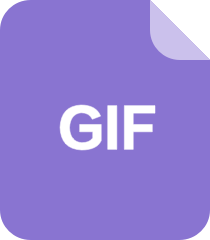
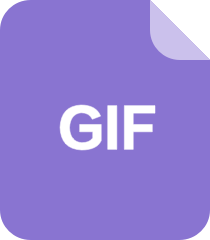
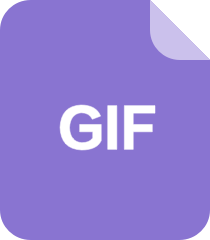
共 875 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
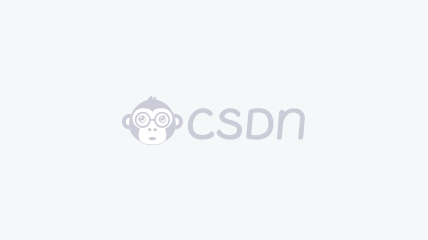
- 水小白2019-01-24楼主,缺着重写的MultipartRequest,您能发送到我邮箱吗646453883@qq.com,非常感谢!!!

初生的圣牌
- 粉丝: 4
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

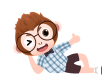
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


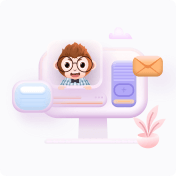
安全验证
文档复制为VIP权益,开通VIP直接复制
