springboot整合kafka,指定分区发送,批量消费,指定topic分区消费
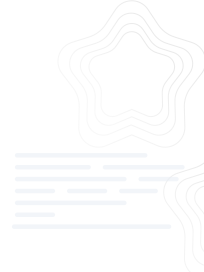


在本文中,我们将深入探讨如何在Spring Boot 2.x应用程序中整合Apache Kafka,重点是实现指定分区发送、批量消费以及指定topic分区消费的功能。Apache Kafka是一个分布式流处理平台,广泛用于构建实时数据管道和流应用。 ### 1. Spring Boot与Kafka整合 Spring Boot简化了在Java应用中配置Kafka的流程。我们需要在`pom.xml`文件中添加Spring Boot对Kafka的依赖: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>org.springframework.kafka</groupId> <artifactId>spring-kafka</artifactId> </dependency> ``` ### 2. 自定义分区发送 在Kafka中,消息发送到特定分区可以通过`ProducerRecord`类的构造函数来指定。在Spring Boot中,我们可以通过`KafkaTemplate`的`send()`方法实现这一功能: ```java @Autowired private KafkaTemplate<String, String> kafkaTemplate; public void sendMessageToPartition(String topic, int partition, String message) { ProducerRecord<String, String> record = new ProducerRecord<>(topic, partition, null, message); kafkaTemplate.send(record); } ``` ### 3. 发送后的回调函数 Spring Boot提供了`ListenableFuture`接口,允许我们在消息发送成功或失败时执行回调操作: ```java @Autowired private KafkaTemplate<String, String> kafkaTemplate; public void sendMessageWithCallback(String topic, String message) { ListenableFuture<SendResult<String, String>> future = kafkaTemplate.send(topic, message); future.addCallback(new ListenableFutureCallback<SendResult<String, String>>() { @Override public void onSuccess(SendResult<String, String> result) { System.out.println("Message sent successfully"); } @Override public void onFailure(Throwable ex) { System.out.println("Failed to send message: " + ex.getMessage()); } }); } ``` ### 4. 批量消费多topic 为了实现批量消费多个topic,我们可以创建一个`@KafkaListener`注解的消费者方法,同时监听多个topic: ```java @Autowired private KafkaListenerContainerFactory<ConcurrentMessageListenerContainer<String, String>> kafkaListenerContainerFactory; @KafkaListener(topics = {"topic1", "topic2"}) public void batchConsume(List<String> messages) { // 批量处理消息 for (String message : messages) { System.out.println("Received message: " + message); } } @Bean public ConcurrentMessageListenerContainer<String, String> kafkaListenerContainer() { ConcurrentMessageListenerContainer<String, String> container = kafkaListenerContainerFactory.createContainer(Arrays.asList("topic1", "topic2")); container.getContainerProperties().setGroupId("batch-consumer"); return container; } ``` ### 5. 消费指定topic的不同分区 如果我们想要针对特定topic的每个分区进行独立消费,可以创建多个`@KafkaListener`方法,每个方法监听一个分区: ```java @KafkaListener(topics = {"myTopic"}, partitions = {0}) public void consumeFromPartition0(String message) { System.out.println("Consumed from partition 0: " + message); } @KafkaListener(topics = {"myTopic"}, partitions = {1}) public void consumeFromPartition1(String message) { System.out.println("Consumed from partition 1: " + message); } ``` 通过这种方式,我们可以根据业务需求灵活地消费Kafka中的数据。结合Spring Boot的自动化配置和强大的API,可以轻松地实现在不同场景下的Kafka集成。以上代码示例仅作为参考,实际使用时需根据项目结构和需求进行调整。
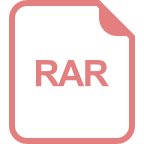
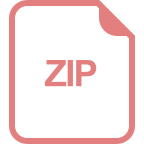
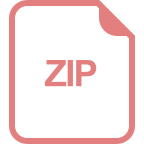
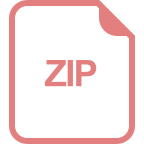
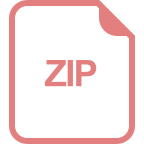
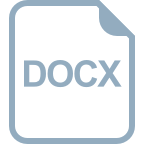
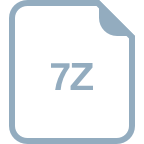
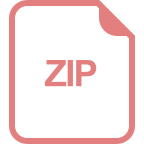
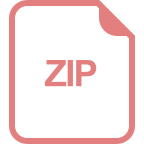
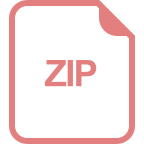
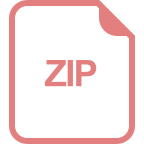
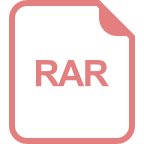
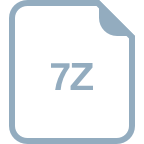
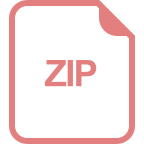
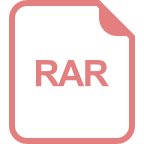
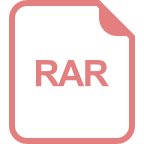
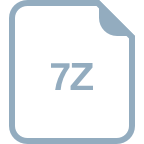
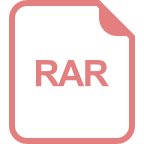
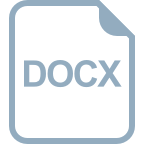
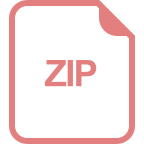
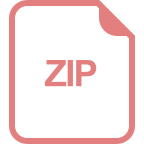
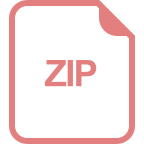
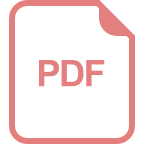
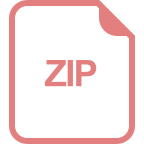
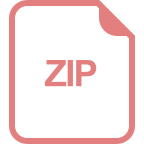
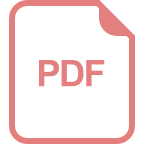

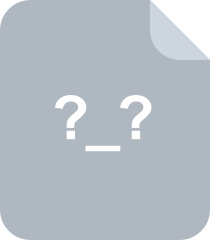
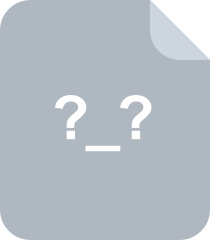
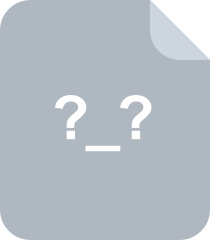
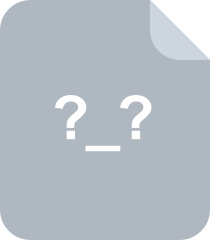
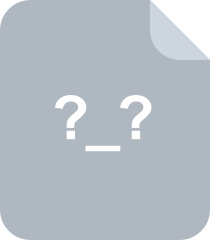
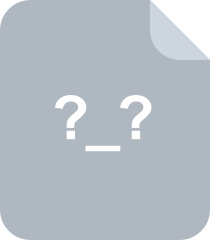
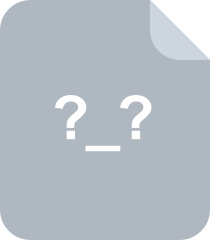
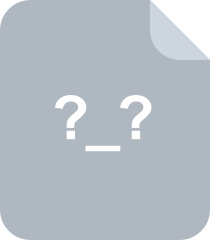
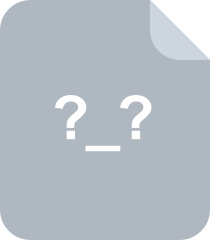
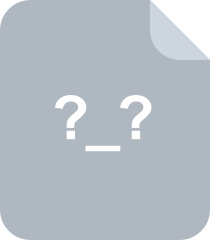
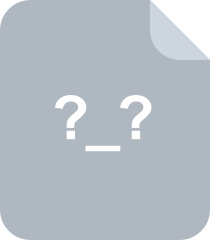
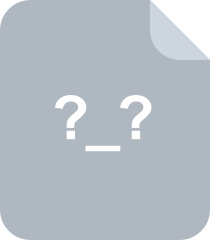
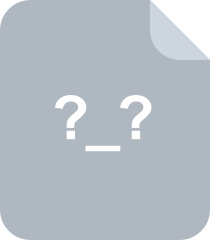
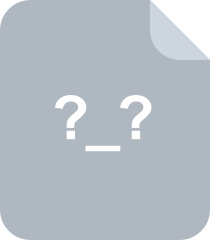
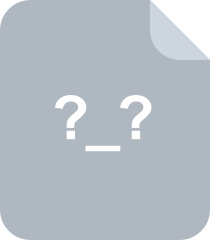
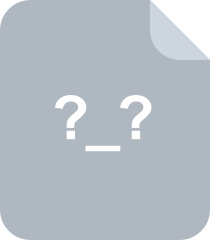
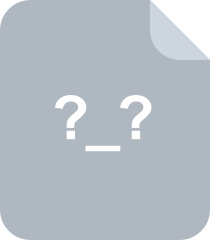
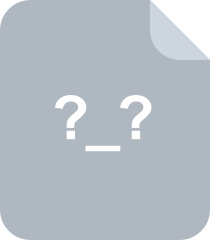
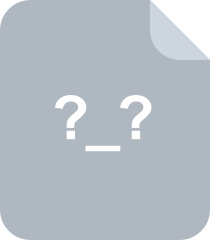
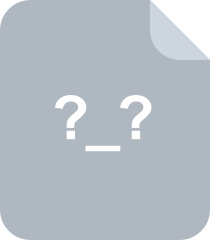
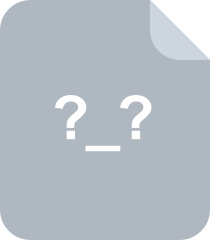
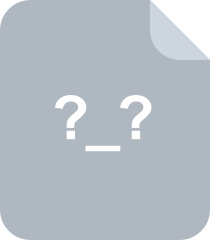
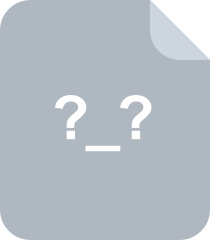
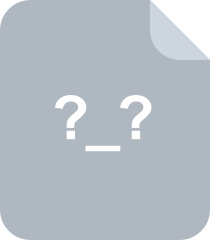
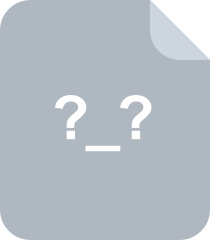
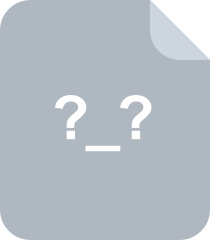
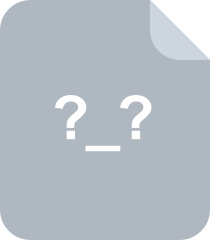
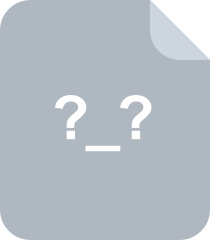
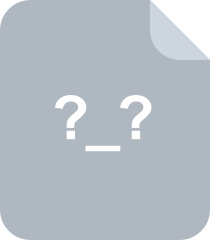
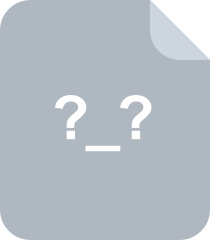
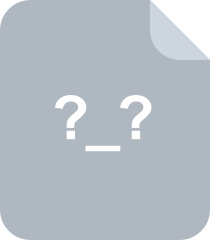
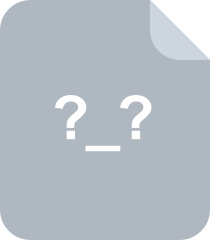
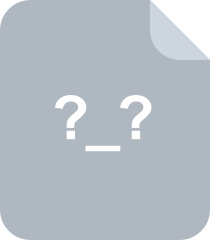
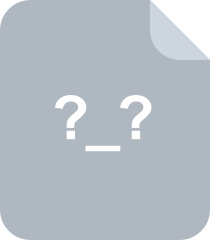
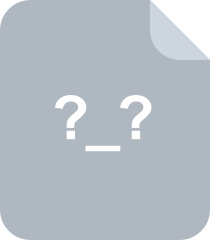
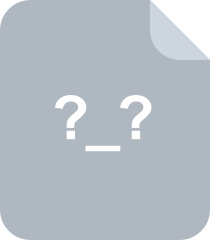
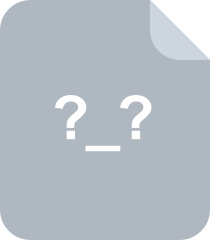
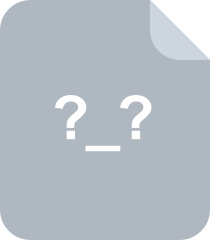
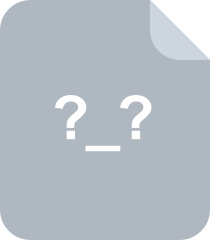
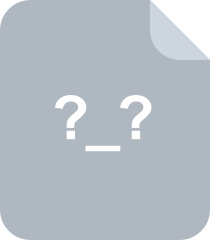
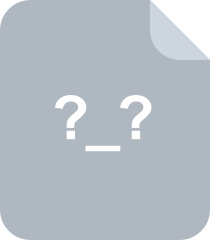
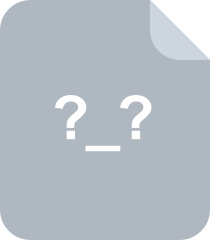
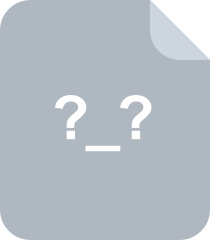
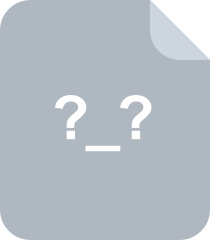
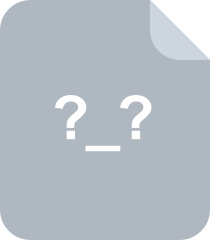
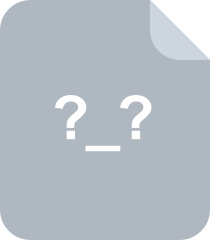
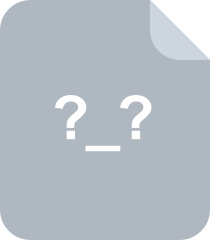
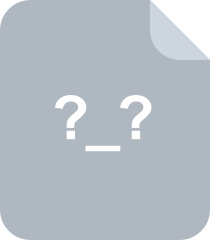
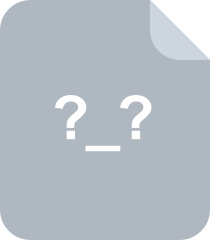
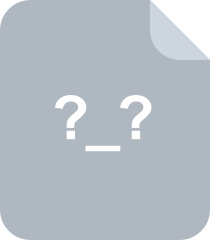
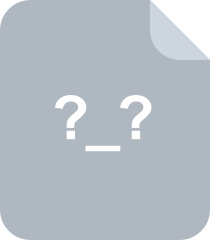
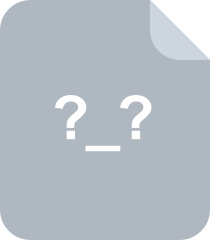
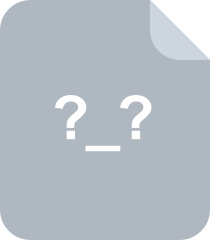
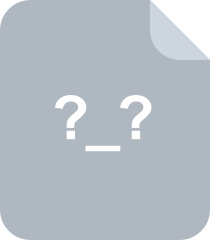
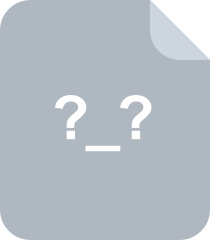
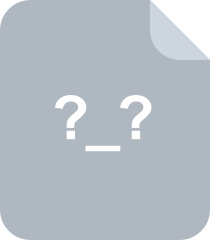
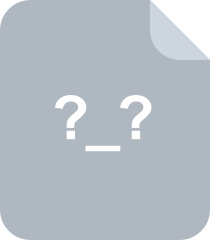
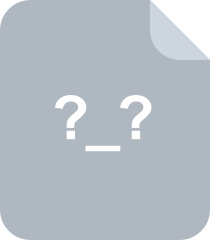
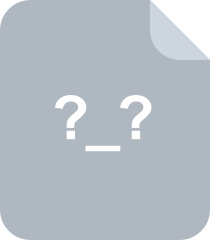
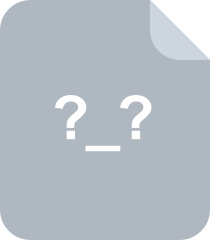
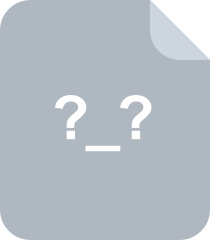
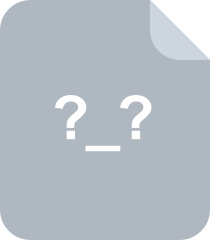
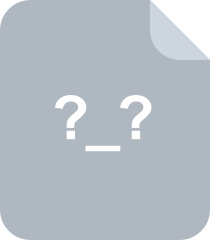
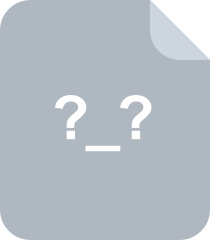
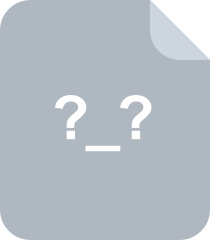
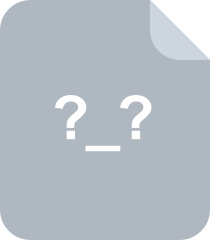
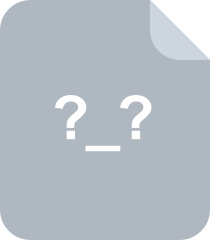
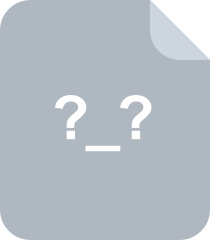
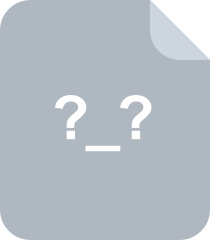
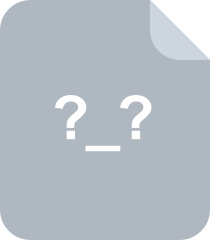
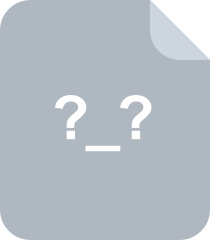
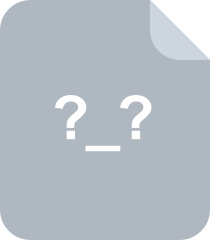
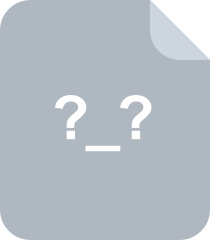
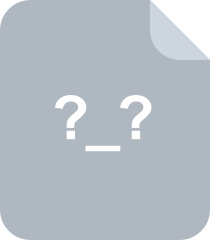
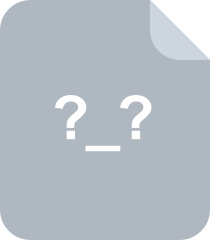
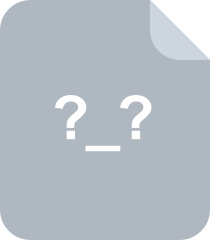
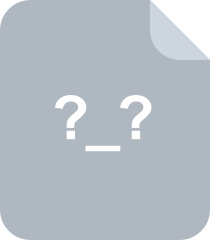
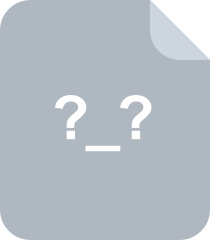
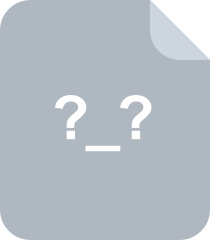
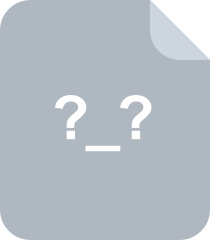
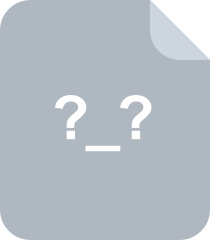
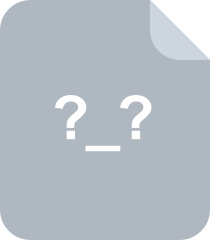
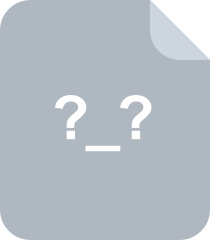
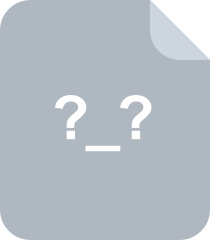
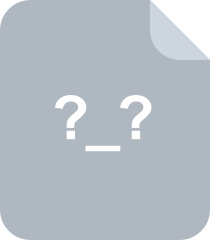
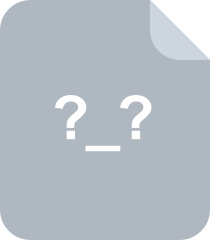
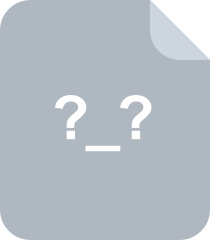
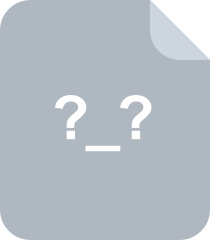
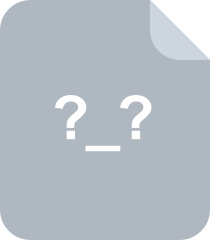
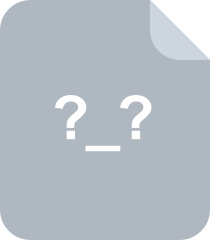
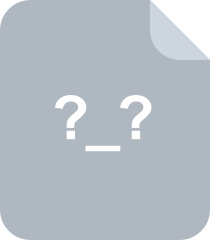
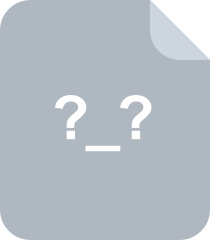
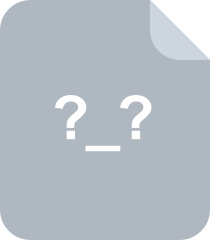
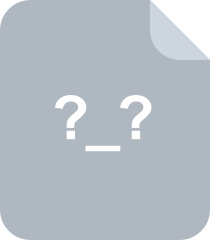
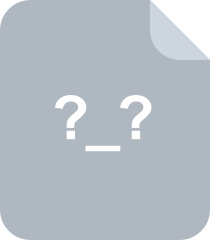
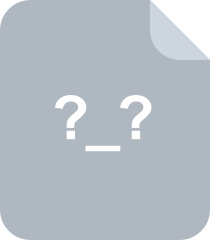
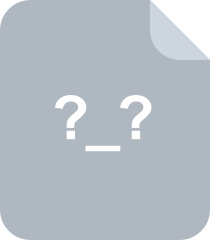
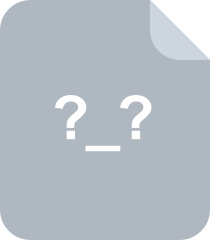
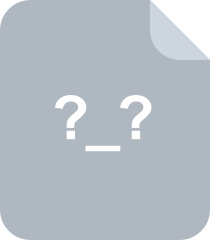
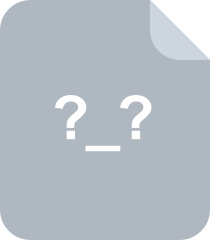
- 1
- 2
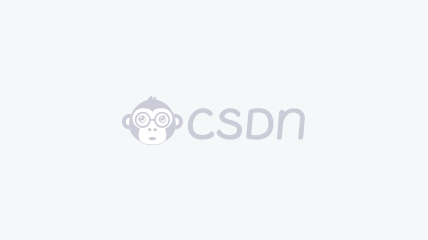
- zby7322021-06-17试过了,不错的工具

- 粉丝: 47
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

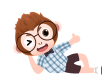
最新资源

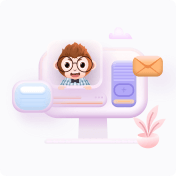
