package edu.hnnu.sport_event.entity;
import java.util.Date;
import java.util.List;
public class Event {
private Integer id; // ID
private String name; // 比赛名称
private Byte quota; // 总名额
private Byte participants; // 报名人数
private Date time; // 比赛开始时间
private String location; // 比赛地点
private Integer score; // 比赛分数
private List<Student> participantsList;
public Event() {
}
public Integer getId() {
return this.id;
}
public String getName() {
return this.name;
}
public Byte getQuota() {
return this.quota;
}
public Byte getParticipants() {
return this.participants;
}
public Date getTime() {
return this.time;
}
public String getLocation() {
return this.location;
}
public Integer getScore() {
return this.score;
}
public List<Student> getParticipantsList() {
return this.participantsList;
}
public void setId(Integer id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setQuota(Byte quota) {
this.quota = quota;
}
public void setParticipants(Byte participants) {
this.participants = participants;
}
public void setTime(Date time) {
this.time = time;
}
public void setLocation(String location) {
this.location = location;
}
public void setScore(Integer score) {
this.score = score;
}
public void setParticipantsList(List<Student> participantsList) {
this.participantsList = participantsList;
}
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Event)) return false;
final Event other = (Event) o;
if (!other.canEqual((Object) this)) return false;
final Object this$id = this.getId();
final Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final Object this$name = this.getName();
final Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final Object this$quota = this.getQuota();
final Object other$quota = other.getQuota();
if (this$quota == null ? other$quota != null : !this$quota.equals(other$quota)) return false;
final Object this$participants = this.getParticipants();
final Object other$participants = other.getParticipants();
if (this$participants == null ? other$participants != null : !this$participants.equals(other$participants))
return false;
final Object this$time = this.getTime();
final Object other$time = other.getTime();
if (this$time == null ? other$time != null : !this$time.equals(other$time)) return false;
final Object this$location = this.getLocation();
final Object other$location = other.getLocation();
if (this$location == null ? other$location != null : !this$location.equals(other$location)) return false;
final Object this$score = this.getScore();
final Object other$score = other.getScore();
if (this$score == null ? other$score != null : !this$score.equals(other$score)) return false;
final Object this$participantsList = this.getParticipantsList();
final Object other$participantsList = other.getParticipantsList();
if (this$participantsList == null ? other$participantsList != null : !this$participantsList.equals(other$participantsList))
return false;
return true;
}
protected boolean canEqual(final Object other) {
return other instanceof Event;
}
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final Object $quota = this.getQuota();
result = result * PRIME + ($quota == null ? 43 : $quota.hashCode());
final Object $participants = this.getParticipants();
result = result * PRIME + ($participants == null ? 43 : $participants.hashCode());
final Object $time = this.getTime();
result = result * PRIME + ($time == null ? 43 : $time.hashCode());
final Object $location = this.getLocation();
result = result * PRIME + ($location == null ? 43 : $location.hashCode());
final Object $score = this.getScore();
result = result * PRIME + ($score == null ? 43 : $score.hashCode());
final Object $participantsList = this.getParticipantsList();
result = result * PRIME + ($participantsList == null ? 43 : $participantsList.hashCode());
return result;
}
public String toString() {
return "Event(id=" + this.getId() + ", name=" + this.getName() + ", quota=" + this.getQuota() + ", participants=" + this.getParticipants() + ", time=" + this.getTime() + ", location=" + this.getLocation() + ", score=" + this.getScore() + ", participantsList=" + this.getParticipantsList() + ")";
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于springboot+mybatis+sqlserver+html运动会报名管理系统(高分课设)已获导师指导并通过的95分的高分期末大作业项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。 系统主要功能: 运动员:项目列表、报名列表 管理员:项目列表、添加项目、查询运动员报名名单 详情:https://blog.csdn.net/qq_33037637/article/details/124967608
资源推荐
资源详情
资源评论
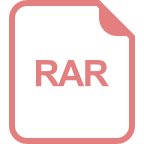
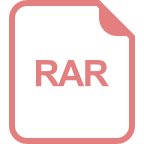
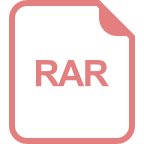
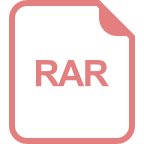
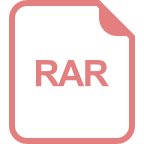
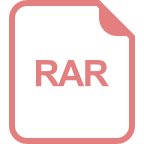
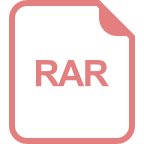
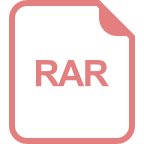
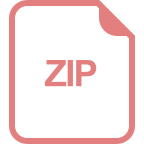
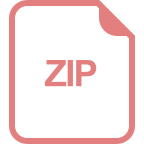
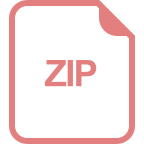
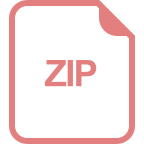
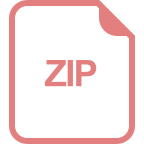
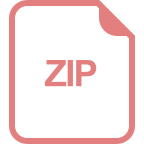
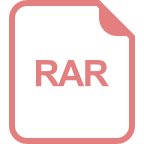
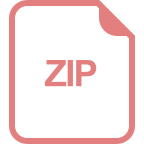
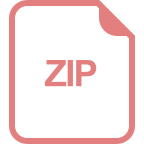
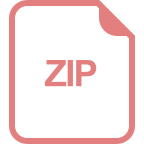
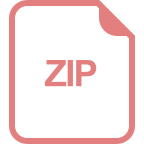
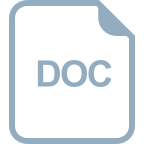
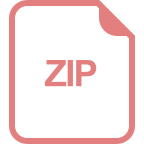
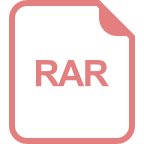
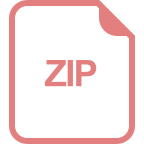
收起资源包目录

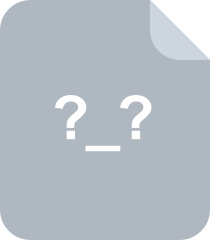
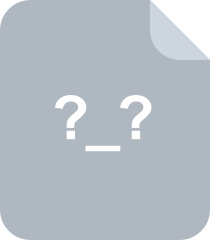
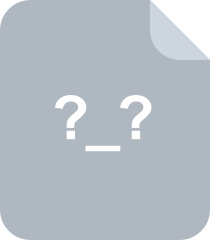
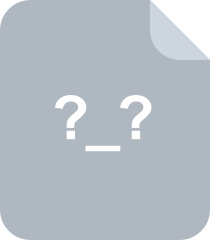
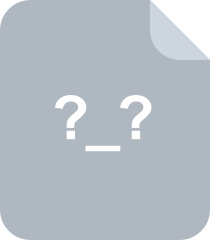
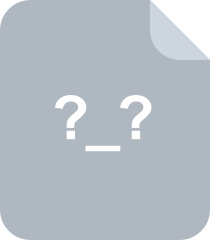
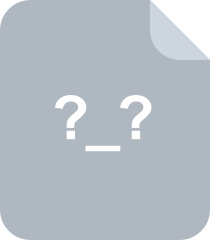
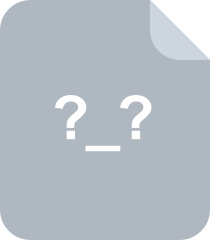
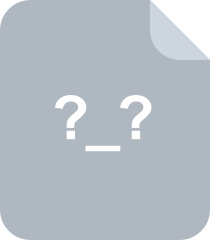
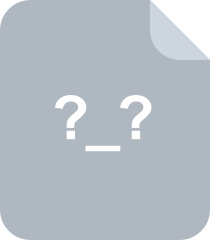
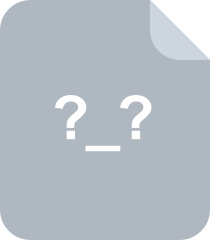
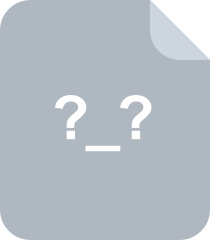
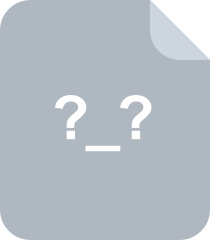
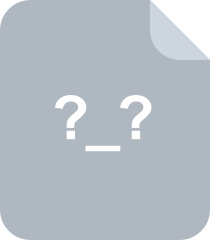
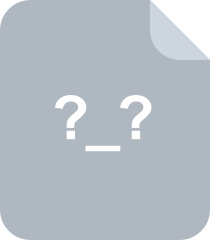
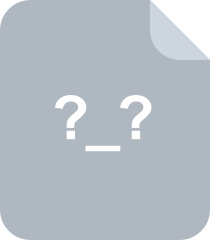
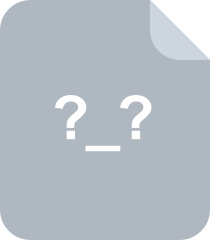
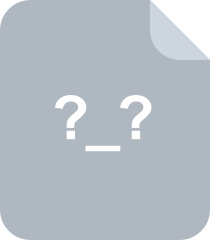
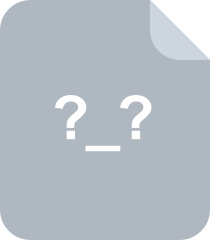
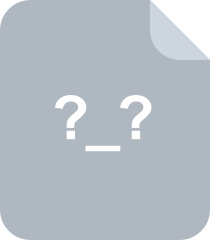
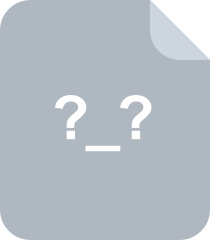
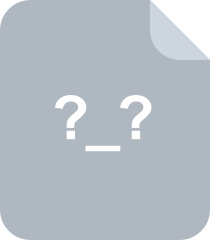
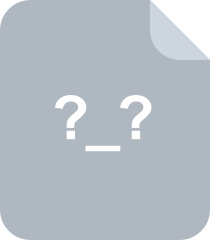
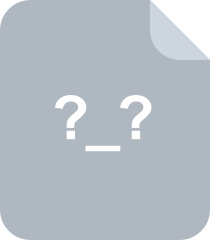
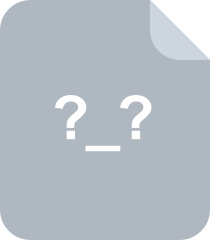
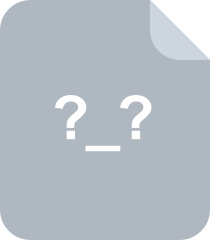
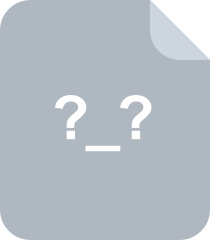
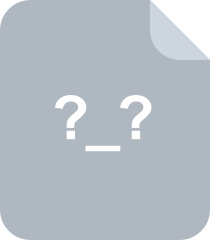
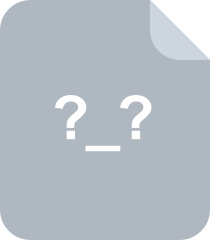
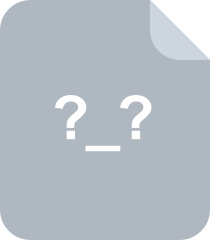
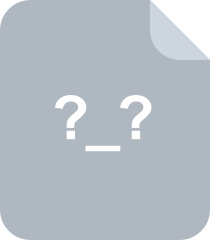
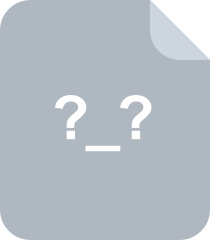
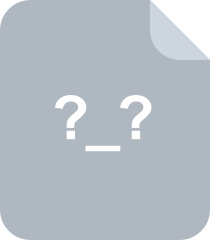
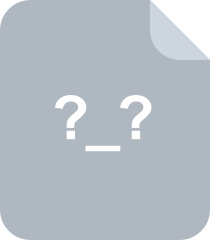
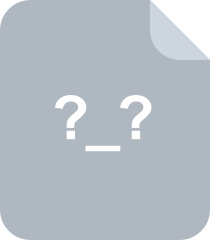
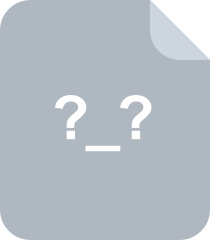
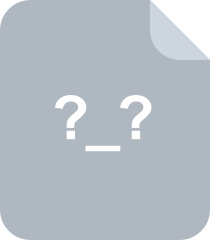
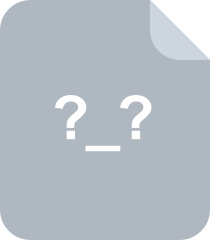
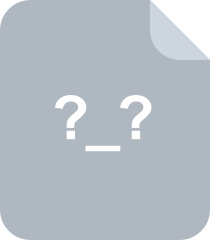
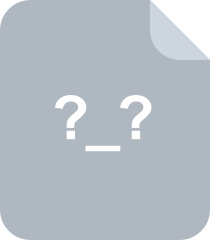
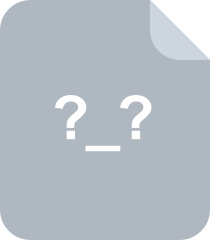
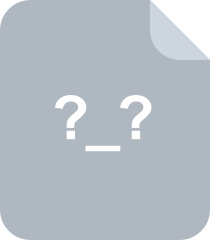
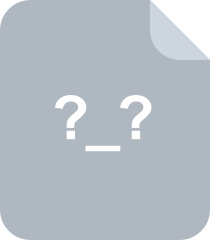
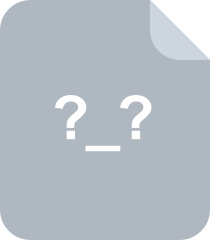
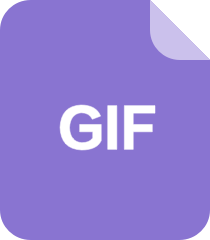
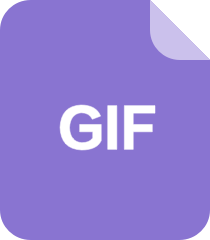
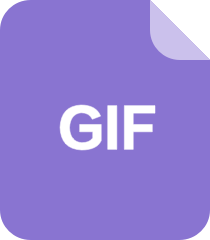
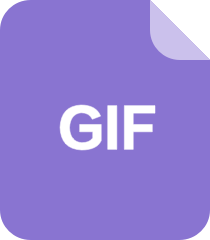
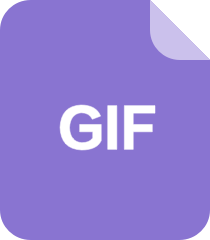
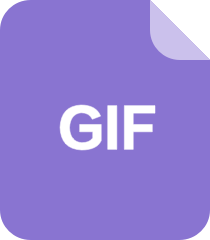
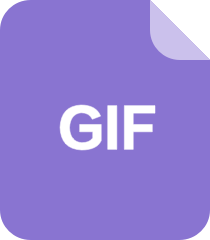
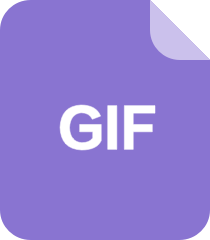
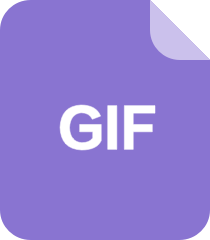
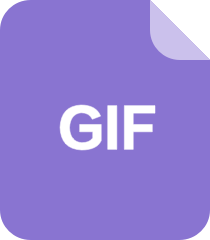
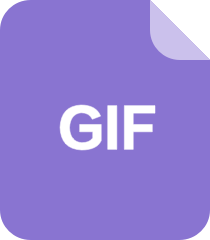
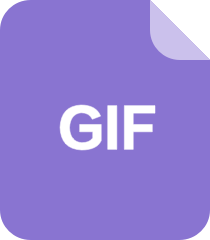
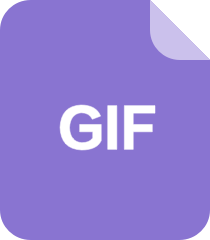
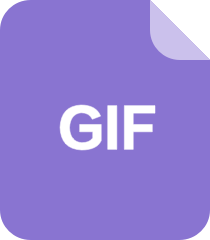
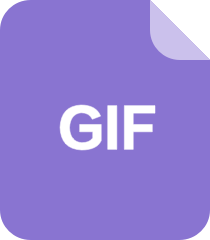
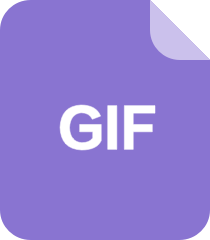
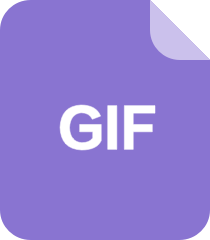
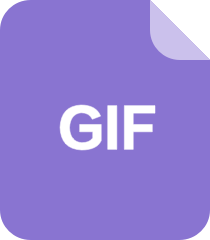
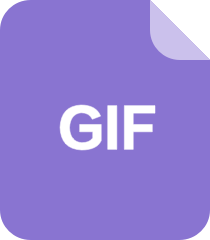
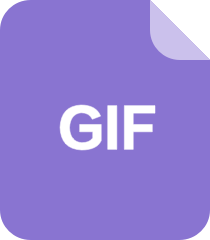
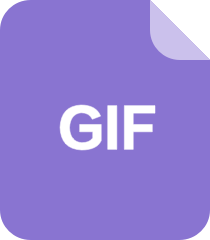
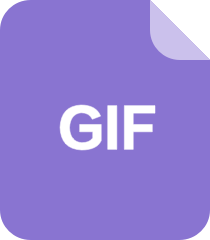
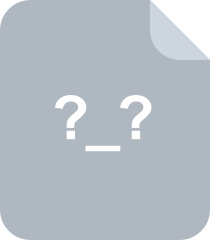
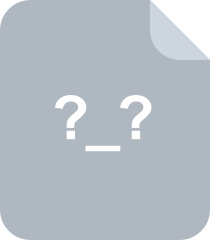
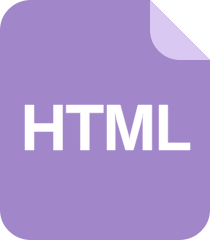
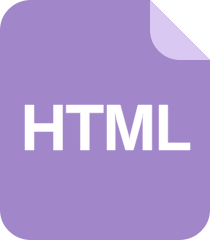
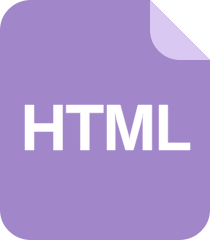
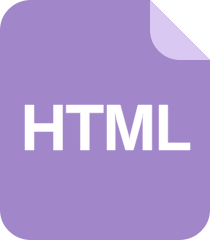
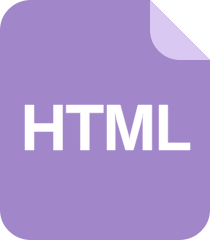
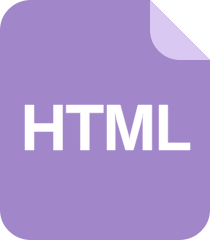
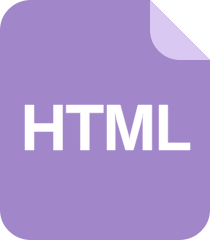
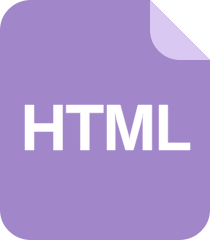
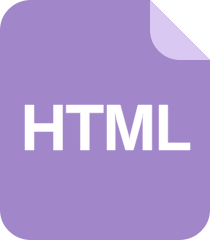
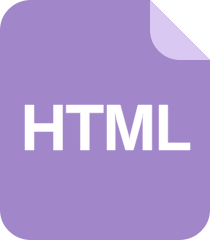
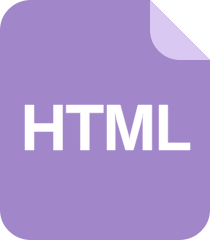
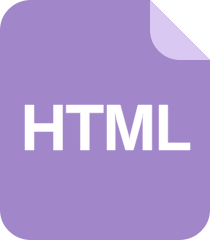
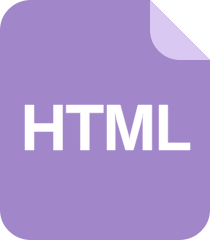
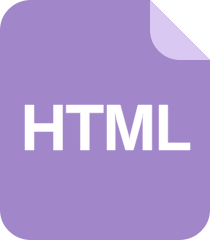
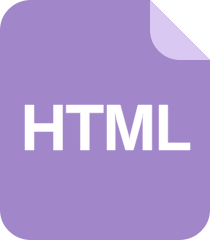
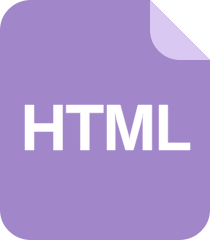
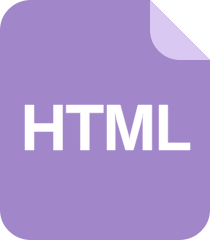
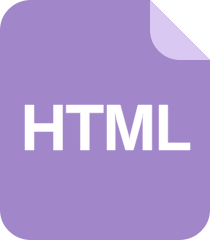
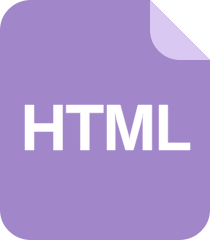
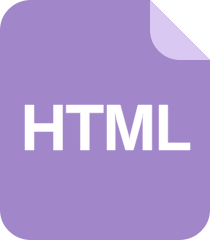
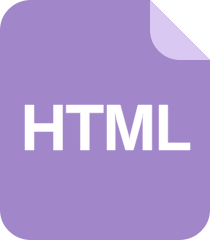
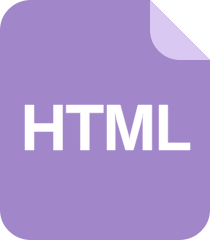
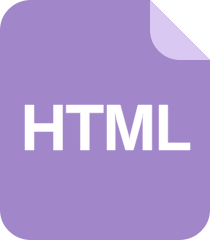
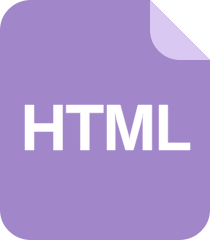
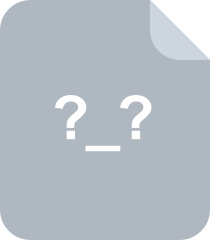
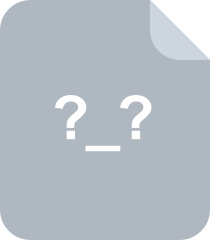
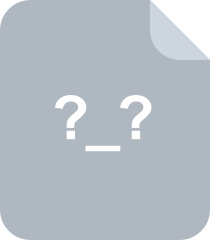
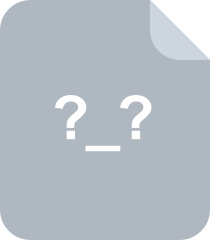
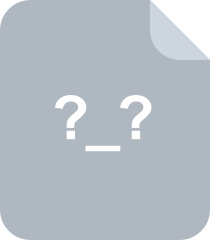
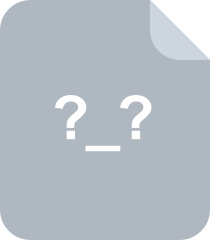
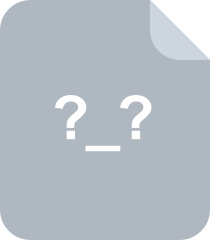
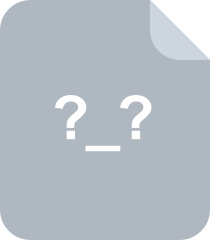
共 239 条
- 1
- 2
- 3
资源评论
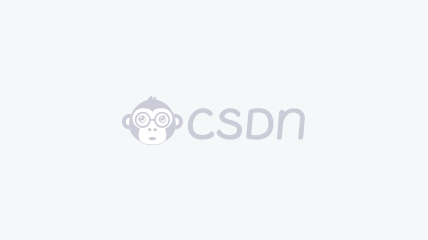


小码叔
- 粉丝: 5174
- 资源: 5326

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

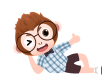
最新资源
- 纯前端js实现圣诞树特效
- 图像加解密技术及其应用场景与重要性
- 直流电机双闭环调速系统仿真模型 转速电流双闭环调速系统Matlab Simulink仿真模型 内外环均采用PI调节器,本模型具体直流电机模块、三相电源、同步6脉冲触发器、双闭环、负载、示波器模块搭建
- S7-200smart 控制台达B2伺服带直线丝杠滑台,PLC程序+触摸屏程序+CAD接线图
- 机械设计塑封机 塑封过胶压覆膜机sw21全套设计资料100%好用.zip
- 基于lqr的主动悬架控制与被动悬架的性能仿真表现(matlabsimulink)对比,模型为四分之一模型 扰动输入,有简单视频讲解,配套的本程序对应内容资料(伦纹)
- 免费Prism WPF 应用案例
- rk3588-测试-调试
- C# OPC DA 协议同步及异步读取数据,支持局域网访问其他OPC server,详细见图片
- python入门参考资料PDF
- 基于springboot的农场投入品运营线上管理系统源码(java毕业设计完整源码).zip
- 基于springboot的办公用品管理系统源码(java毕业设计完整源码).zip
- SOEM Ether CAT C语言源码
- 基于selenium+python实现京东商品爬虫淘宝店铺爬虫项目源码
- 机械手轨迹规划, 5次b样条, 七次b样条, 可显示位置,速度,加速度,加加速度曲线图 轨迹优化 基于NSGA2多目标轨迹规划, 一个可以让你直接用的代码
- 基于springboot的助农电商平台源码(java毕业设计完整源码).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


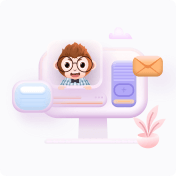
安全验证
文档复制为VIP权益,开通VIP直接复制
