===========
NumPy C-API
===========
::
unsigned int
PyArray_GetNDArrayCVersion(void )
Included at the very first so not auto-grabbed and thus not labeled.
::
int
PyArray_SetNumericOps(PyObject *dict)
Set internal structure with number functions that all arrays will use
::
PyObject *
PyArray_GetNumericOps(void )
Get dictionary showing number functions that all arrays will use
::
int
PyArray_INCREF(PyArrayObject *mp)
For object arrays, increment all internal references.
::
int
PyArray_XDECREF(PyArrayObject *mp)
Decrement all internal references for object arrays.
(or arrays with object fields)
::
void
PyArray_SetStringFunction(PyObject *op, int repr)
Set the array print function to be a Python function.
::
PyArray_Descr *
PyArray_DescrFromType(int type)
Get the PyArray_Descr structure for a type.
::
PyObject *
PyArray_TypeObjectFromType(int type)
Get a typeobject from a type-number -- can return NULL.
New reference
::
char *
PyArray_Zero(PyArrayObject *arr)
Get pointer to zero of correct type for array.
::
char *
PyArray_One(PyArrayObject *arr)
Get pointer to one of correct type for array
::
PyObject *
PyArray_CastToType(PyArrayObject *arr, PyArray_Descr *dtype, int
is_f_order)
For backward compatibility
Cast an array using typecode structure.
steals reference to dtype --- cannot be NULL
This function always makes a copy of arr, even if the dtype
doesn't change.
::
int
PyArray_CastTo(PyArrayObject *out, PyArrayObject *mp)
Cast to an already created array.
::
int
PyArray_CastAnyTo(PyArrayObject *out, PyArrayObject *mp)
Cast to an already created array. Arrays don't have to be "broadcastable"
Only requirement is they have the same number of elements.
::
int
PyArray_CanCastSafely(int fromtype, int totype)
Check the type coercion rules.
::
npy_bool
PyArray_CanCastTo(PyArray_Descr *from, PyArray_Descr *to)
leaves reference count alone --- cannot be NULL
PyArray_CanCastTypeTo is equivalent to this, but adds a 'casting'
parameter.
::
int
PyArray_ObjectType(PyObject *op, int minimum_type)
Return the typecode of the array a Python object would be converted to
Returns the type number the result should have, or NPY_NOTYPE on error.
::
PyArray_Descr *
PyArray_DescrFromObject(PyObject *op, PyArray_Descr *mintype)
new reference -- accepts NULL for mintype
::
PyArrayObject **
PyArray_ConvertToCommonType(PyObject *op, int *retn)
This function is only used in one place within NumPy and should
generally be avoided. It is provided mainly for backward compatibility.
The user of the function has to free the returned array with PyDataMem_FREE.
::
PyArray_Descr *
PyArray_DescrFromScalar(PyObject *sc)
Return descr object from array scalar.
New reference
::
PyArray_Descr *
PyArray_DescrFromTypeObject(PyObject *type)
::
npy_intp
PyArray_Size(PyObject *op)
Compute the size of an array (in number of items)
::
PyObject *
PyArray_Scalar(void *data, PyArray_Descr *descr, PyObject *base)
Get scalar-equivalent to a region of memory described by a descriptor.
::
PyObject *
PyArray_FromScalar(PyObject *scalar, PyArray_Descr *outcode)
Get 0-dim array from scalar
0-dim array from array-scalar object
always contains a copy of the data
unless outcode is NULL, it is of void type and the referrer does
not own it either.
steals reference to outcode
::
void
PyArray_ScalarAsCtype(PyObject *scalar, void *ctypeptr)
Convert to c-type
no error checking is performed -- ctypeptr must be same type as scalar
in case of flexible type, the data is not copied
into ctypeptr which is expected to be a pointer to pointer
::
int
PyArray_CastScalarToCtype(PyObject *scalar, void
*ctypeptr, PyArray_Descr *outcode)
Cast Scalar to c-type
The output buffer must be large-enough to receive the value
Even for flexible types which is different from ScalarAsCtype
where only a reference for flexible types is returned
This may not work right on narrow builds for NumPy unicode scalars.
::
int
PyArray_CastScalarDirect(PyObject *scalar, PyArray_Descr
*indescr, void *ctypeptr, int outtype)
Cast Scalar to c-type
::
PyObject *
PyArray_ScalarFromObject(PyObject *object)
Get an Array Scalar From a Python Object
Returns NULL if unsuccessful but error is only set if another error occurred.
Currently only Numeric-like object supported.
::
PyArray_VectorUnaryFunc *
PyArray_GetCastFunc(PyArray_Descr *descr, int type_num)
Get a cast function to cast from the input descriptor to the
output type_number (must be a registered data-type).
Returns NULL if un-successful.
::
PyObject *
PyArray_FromDims(int NPY_UNUSED(nd) , int *NPY_UNUSED(d) , int
NPY_UNUSED(type) )
Deprecated, use PyArray_SimpleNew instead.
::
PyObject *
PyArray_FromDimsAndDataAndDescr(int NPY_UNUSED(nd) , int
*NPY_UNUSED(d) , PyArray_Descr
*descr, char *NPY_UNUSED(data) )
Deprecated, use PyArray_NewFromDescr instead.
::
PyObject *
PyArray_FromAny(PyObject *op, PyArray_Descr *newtype, int
min_depth, int max_depth, int flags, PyObject
*context)
Does not check for NPY_ARRAY_ENSURECOPY and NPY_ARRAY_NOTSWAPPED in flags
Steals a reference to newtype --- which can be NULL
::
PyObject *
PyArray_EnsureArray(PyObject *op)
This is a quick wrapper around
PyArray_FromAny(op, NULL, 0, 0, NPY_ARRAY_ENSUREARRAY, NULL)
that special cases Arrays and PyArray_Scalars up front
It *steals a reference* to the object
It also guarantees that the result is PyArray_Type
Because it decrefs op if any conversion needs to take place
so it can be used like PyArray_EnsureArray(some_function(...))
::
PyObject *
PyArray_EnsureAnyArray(PyObject *op)
::
PyObject *
PyArray_FromFile(FILE *fp, PyArray_Descr *dtype, npy_intp num, char
*sep)
Given a ``FILE *`` pointer ``fp``, and a ``PyArray_Descr``, return an
array corresponding to the data encoded in that file.
The reference to `dtype` is stolen (it is possible that the passed in
dtype is not held on to).
The number of elements to read is given as ``num``; if it is < 0, then
then as many as possible are read.
If ``sep`` is NULL or empty, then binary data is assumed, else
text data, with ``sep`` as the separator between elements. Whitespace in
the separator matches any length of whitespace in the text, and a match
for whitespace around the separator is added.
For memory-mapped files, use the buffer interface. No more data than
necessary is read by this routine.
::
PyObject *
PyArray_FromString(char *data, npy_intp slen, PyArray_Descr
*dtype, npy_intp num, char *sep)
Given a pointer to a string ``data``, a string length ``slen``, and
a ``PyArray_Descr``, return an array corresponding to the data
encoded in that string.
If the dtype is NULL, the default array type is used (double).
If non-null, the reference is stolen.
If ``slen`` is < 0, then the end of string is used for text data.
It is an error for ``slen`` to be < 0 for binary data (since embedded NULLs
would be the norm).
The number of elements to read is given as ``num``; if it is < 0, then
then as many as possible are read.
If ``sep`` is NULL or empty, then binary data is assumed, else
text data, with ``sep`` as the separator between elements. Whitespace in
the separator matches any length of whitespace in the text, and a match
for whitespace around the separator is added.


EasySoft易软
- 粉丝: 4666
- 资源: 1704
最新资源
- VTK8.2.0-Release版本
- 好用的截图工具-Snipaste
- Plant Leaves Disease Detection
- 100kW光伏并网发电系统MATLAB仿真平均模型 采用“扰动观察P&O+积分调节器”技术的MPPT控制器 VSC并网控制 附有lunwen
- WebSocket协议详解:实现实时高效双向通信的技术指南
- Python 书店管理系统源码,有详细的功能要求、使用技术、数据库设计、用户界面搭建、扩展需求-安全控制说明
- 同步电机无传感SMO滑膜观测器模型+代码 PMSM永磁同步电机无传感器滑模观测器仿真模型(基于28035),典型的smo方案; 代码为实际应用SOP代码,非一般玩票代码可比(非ti例程);解析说明详细
- 帮助把握混合动力汽车能量管理策略当前研究热点,梳理常用算法,整理科研思路 包括基于ADMM的能量管理策略一份
- c#轻量级高并发物联网服务器接收程序源码(仅仅是接收硬件数据程序,没有web端,不是java,协议自己写,如果问及这些问题统统不回复 ),对接几万个设备没问题,数据库采用ef6+sqlite,可改e
- 基于FPGA和W5500的TCP网络通信 测试平台 zynq扩展口开发 软件平台 vivado2019.2,纯Verilog可移植 提供tcp数据环回测试模式和用户数据ram接口 测试环境 压力测试
- 免编程拖拽C#源码,可以进行二次开发,功能强大 1.支持节点连接,和删除 2.功能块任意拖拽,节点跟随,功能块属性设置输入输出和删除 3.连接节点,触发各功能块任务,可以把触发结果传给下个输入 4.功
- 锅炉控制器配套原理图+PCB+源码+文档说明 项目要求与网上搜的那些开发板的例程完全不在一个级别,也不是那些凑合性质的项目可以比拟的 项目是企业级产品的要求开发的,能够让初学者了解真实的企业项目是
- 443大神SSH2电子图书集中发布系统毕业课程源码设计
- 奇迹MU Item物品编码转换器GM工具
- 西门子S7-1500博图程序 例程,大型生产线案例,程序涵盖有机器人块,汽缸块,电机块,伺服块,可调用,扫码块,可学习参考,快速提升技能 ,编程使用的语言有SCL,LD,STL,GRAPH 非常全
- Matlab Simulimk仿真,Flyback反激式开关电源仿真
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


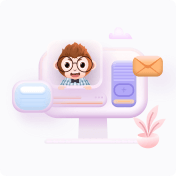