Option Explicit
'Download by http://www.codefans.net
Private Declare Function mciSendString Lib "winmm.dll" Alias "mciSendStringA" (ByVal lpstrCommand As String, ByVal lpstrReturnString As String, ByVal uReturnLength As Long, ByVal hwndCallback As Long) As Long
Private Declare Function mciGetErrorString Lib "winmm.dll" Alias "mciGetErrorStringA" (ByVal dwError As Long, ByVal lpstrBuffer As String, ByVal uLength As Long) As Long
Dim ReturnString As String * 256
Dim RetValue As Long
Dim errorstring As String * 1024
Private Sub cmdPlay_Click()
Dim CommandString As String
If Dir(App.Path & "\Test.Wav") <> "" Then
RetValue = mciSendString("open Test.Wav alias sounds1", ReturnString, 256, 0)
mciSendString "play sounds1", ReturnString, 256, 0
Else
MsgBox "没有声音文件 Test.Wav", vbOKOnly, "提示"
End If
End Sub
Private Sub cmdRecord_Click()
RetValue = mciSendString("open new type waveaudio alias sounds1", ReturnString, 256, 0)
If RetValue = 0 Then
'开始录音...
RetValue = mciSendString("record sounds1", ReturnString, 256, 0)
cmdStop.Enabled = True
Else
mciGetErrorString RetValue, errorstring, 1024
MsgBox errorstring
End If
End Sub
Private Sub cmdStop_Click()
RetValue = mciSendString("stop sounds1", ReturnString, 256, 0)
RetValue = mciSendString("save sounds1 Test.Wav", ReturnString, 256, 0)
If RetValue = 0 Then
Else
mciGetErrorString RetValue, errorstring, 1024
MsgBox errorstring
End If
RetValue = mciSendString("close sounds1", ReturnString, 256, 0)
cmdStop.Enabled = False
End Sub
Private Sub Form_Unload(Cancel As Integer)
mciSendString "close all", ReturnString, 256, 0
End Sub
改编以上程序代码,改编成VB.NET版本的程序代码。
以下是将您提供的 VB 代码改编为 VB.NET 版本的示例:
```vb
Imports System.Runtime.InteropServices
Module Module1
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciSendString")>
Function mciSendString(lpstrCommand As String, ByRef lpstrReturnString As StringBuilder, uReturnLength As Integer, hwndCallback As Integer) As Integer
End Function
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciGetErrorString")>
Function mciGetErrorString(dwError As Integer, ByRef lpstrBuffer As StringBuilder, uLength As Integer) As Integer
End Function
Dim ReturnString As New StringBuilder(256)
Dim RetValue As Integer
Dim errorstring As New StringBuilder(1024)
Private Sub cmdPlay_Click()
Dim CommandString As String
If IO.File.Exists(Application.StartupPath & "\Test.Wav") Then
RetValue = mciSendString("open Test.Wav alias sounds1", ReturnString, 256, 0)
mciSendString("play sounds1", ReturnString, 256, 0)
Else
MessageBox.Show("没有声音文件 Test.Wav", "提示", MessageBoxButtons.OK)
End If
End Sub
Private Sub cmdRecord_Click()
RetValue = mciSendString("open new type waveaudio alias sounds1", ReturnString, 256, 0)
If RetValue = 0 Then
'开始录音...
RetValue = mciSendString("record sounds1", ReturnString, 256, 0)
cmdStop.Enabled = True
Else
mciGetErrorString(RetValue, errorstring, 1024)
MessageBox.Show(errorstring.ToString)
End If
End Sub
Private Sub cmdStop_Click()
RetValue = mciSendString("stop sounds1", ReturnString, 256, 0)
RetValue = mciSendString("save sounds1 Test.Wav", ReturnString, 256, 0)
If RetValue = 0 Then
Else
mciGetErrorString(RetValue, errorstring, 1024)
MessageBox.Show(errorstring.ToString)
End If
RetValue = mciSendString("close sounds1", ReturnString, 256, 0)
cmdStop.Enabled = False
End Sub
Private Sub Form_Unload(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs)
mciSendString("close all", ReturnString, 256, 0)
End Sub
End Module
```
在这个改编后的代码中:
- 使用了 `System.Runtime.InteropServices` 命名空间来导入 Windows API 函数。
- 将固定长度的字符串缓冲区改为 `StringBuilder` 类型。
- 使用 `IO.File.Exists` 来检查文件是否存在。
- 在显示消息框时,直接使用 `MessageBox.Show` 方法。
答案2
```vb
Imports System.Runtime.InteropServices
Module Module1
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciSendString")>
Function mciSendString(lpstrCommand As String, ByRef lpstrReturnString As StringBuilder, uReturnLength As Integer, hwndCallback As Integer) As Integer
End Function
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciGetErrorString")>
Function mciGetErrorString(dwError As Integer, ByRef lpstrBuffer As StringBuilder, uLength As Integer) As Integer
End Function
Dim ReturnString As New StringBuilder(256)
Dim RetValue As Integer
Dim errorstring As New StringBuilder(1024)
Private Sub cmdPlay_Click(sender As Object, e As EventArgs)
Dim CommandString As String
If System.IO.File.Exists(System.AppDomain.CurrentDomain.BaseDirectory & "\Test.Wav") Then
RetValue = mciSendString("open Test.Wav alias sounds1", ReturnString, 256, 0)
mciSendString("play sounds1", ReturnString, 256, 0)
Else
System.Windows.Forms.MessageBox.Show("没有声音文件 Test.Wav", "提示", System.Windows.Forms.MessageBoxButtons.OK)
End If
End Sub
Private Sub cmdRecord_Click(sender As Object, e As EventArgs)
RetValue = mciSendString("open new type waveaudio alias sounds1", ReturnString, 256, 0)
If RetValue = 0 Then
'开始录音...
RetValue = mciSendString("record sounds1", ReturnString, 256, 0)
cmdStop.Enabled = True
Else
mciGetErrorString(RetValue, errorstring, 1024)
System.Windows.Forms.MessageBox.Show(errorstring.ToString)
End If
End Sub
Private Sub cmdStop_Click(sender As Object, e As EventArgs)
RetValue = mciSendString("stop sounds1", ReturnString, 256, 0)
RetValue = mciSendString("save sounds1 Test.Wav", ReturnString, 256, 0)
If RetValue = 0 Then
Else
mciGetErrorString(RetValue, errorstring, 1024)
System.Windows.Forms.MessageBox.Show(errorstring.ToString)
End If
RetValue = mciSendString("close sounds1", ReturnString, 256, 0)
cmdStop.Enabled = False
End Sub
Private Sub Form_Unload(sender As Object, e As System.ComponentModel.CancelEventArgs)
mciSendString("close all", ReturnString, 256, 0)
End Sub
End Module
```
Imports System.Runtime.InteropServices
Public Class Form1
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciSendString")>
Function mciSendString(ByVal lpstrCommand As String, ByRef lpstrReturnString As StringBuilder, ByVal uReturnLength As Integer, ByVal hwndCallback As Integer) As Integer
End Function
<DllImport("winmm.dll", CharSet:=CharSet.Ansi, EntryPoint:="mciGetErrorString")>
Function mciGetErrorString(ByVal dwError As Integer, ByRef lpstrBuffer As StringBuilder, ByVal uLength As Integer) As Integer
End Function
Dim ReturnString As New StringBuilder(256)
Dim RetValue As Integer
Dim errorstring As New StringBuilder(1024)
Private Sub Form_Unload(ByVal sender As Object, ByVal e As System.ComponentModel.CancelEventArgs)
mciSendString("close all", ReturnString, 256, 0)
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
RetValue = mciSendString("stop sounds1", ReturnStri
没有合适的资源?快使用搜索试试~ 我知道了~
Visual Studio 2010旗舰版的vb.net版本录音程序源代码2024-7-21QZQ.zip
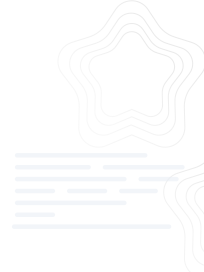
共69个文件
vb:12个
txt:7个
cache:6个

0 下载量 30 浏览量
2024-07-21
15:00:41
上传
评论
收藏 2.53MB ZIP 举报
温馨提示
Visual Studio 2010旗舰版的vb.net版本录音程序源代码2024-7-21QZQ.zip
资源推荐
资源详情
资源评论
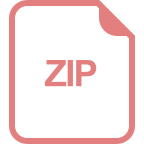
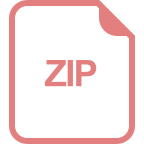
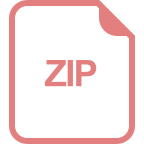
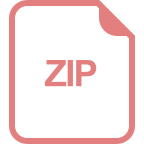
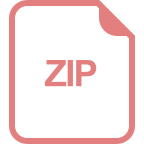
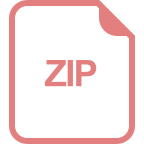
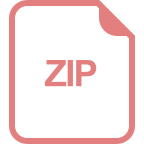
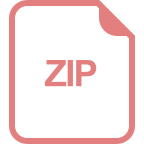
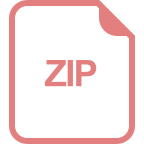
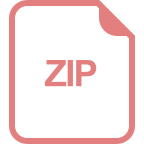
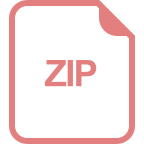
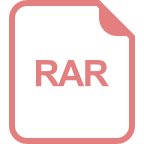
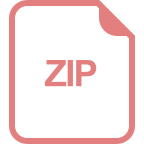
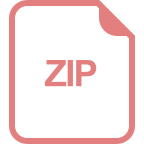
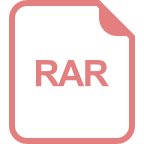
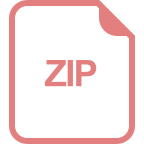
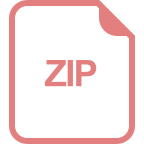
收起资源包目录



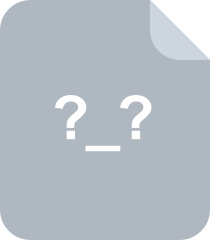

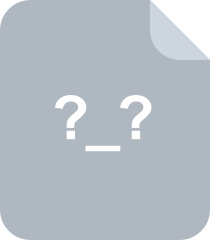

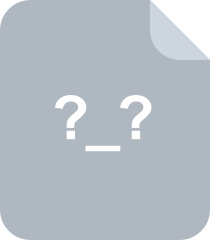
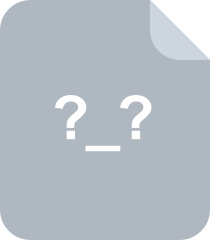
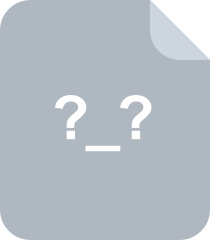
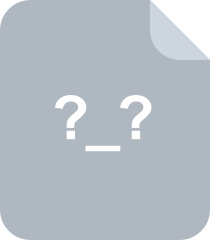
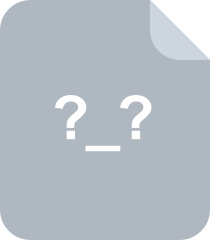
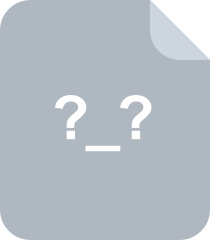
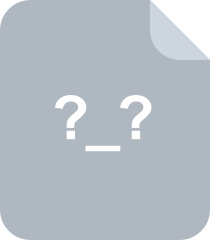




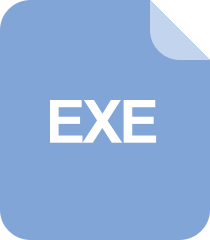
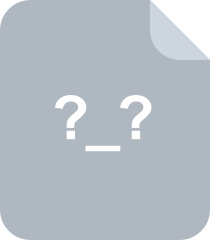
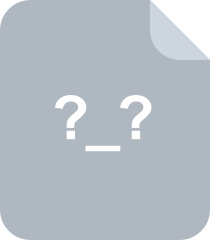
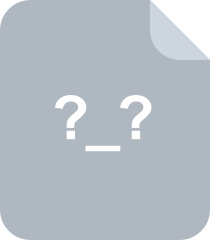

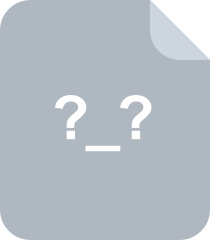
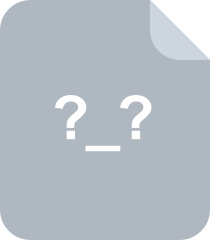
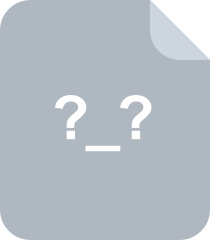
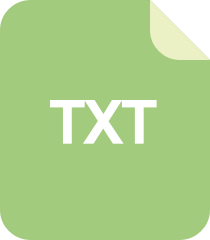
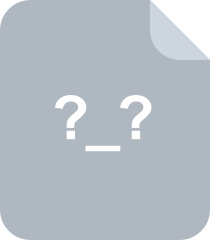
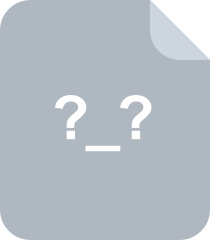
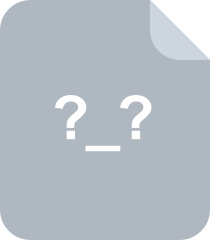



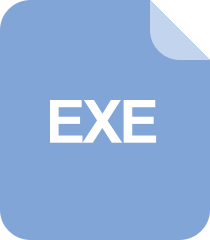
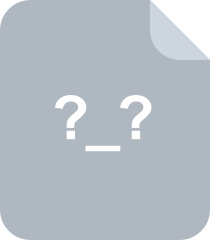
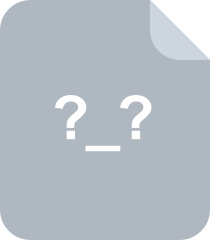
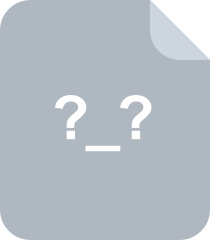
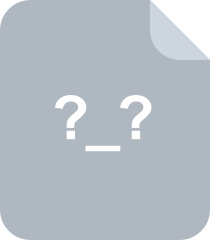
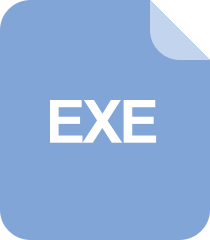
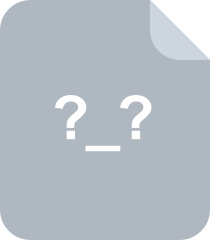
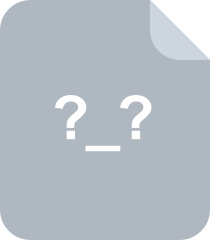
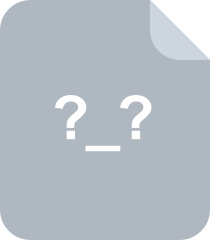
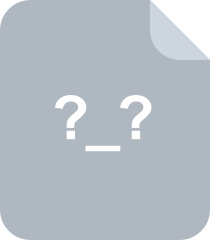
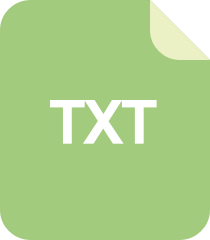
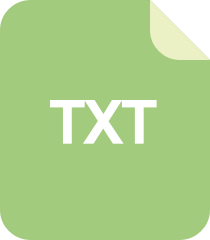

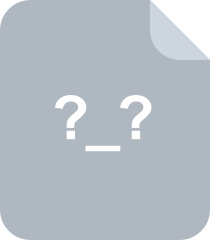

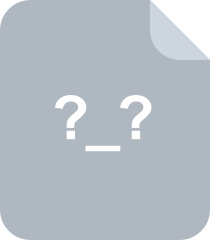

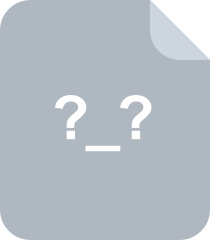
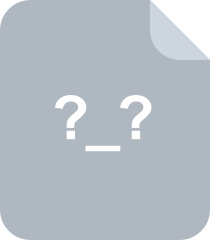
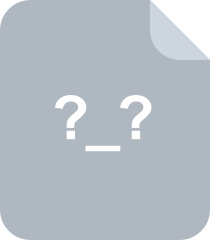
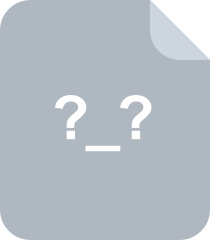
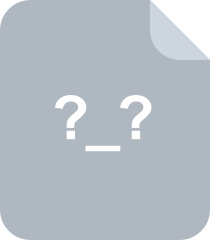
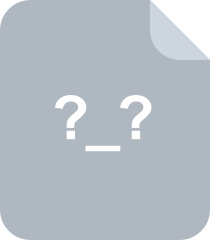
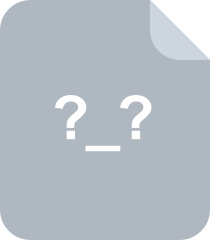




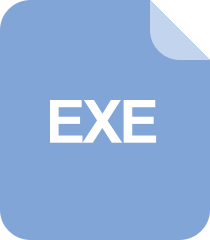
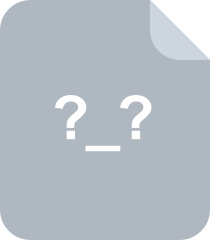
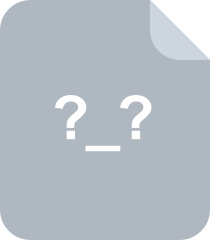
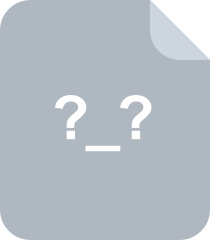

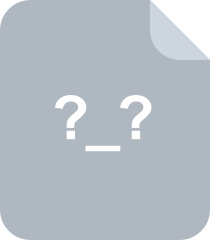
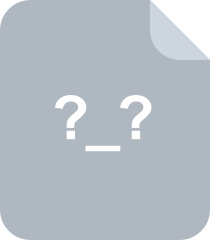
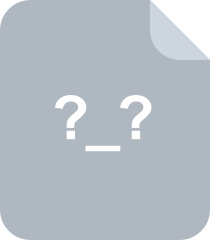
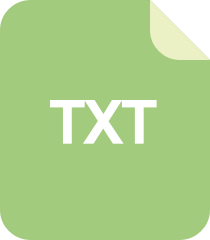
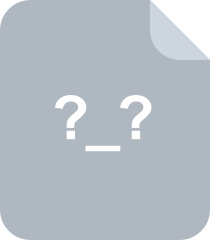
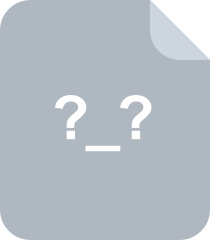
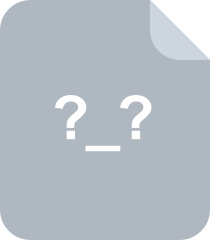



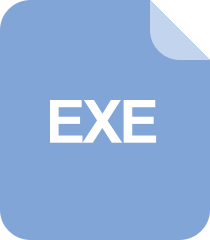
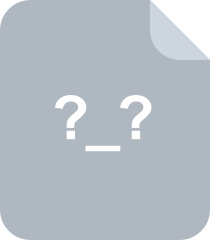
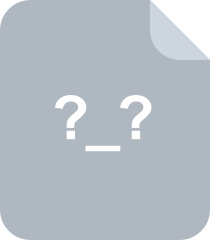
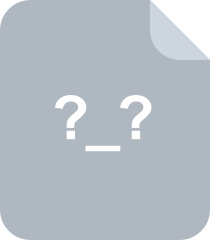
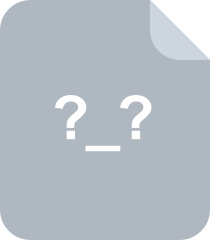
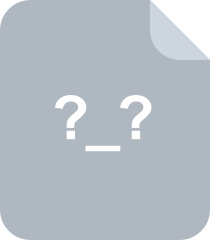
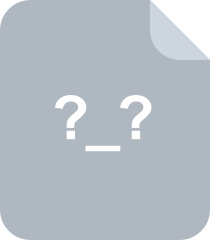
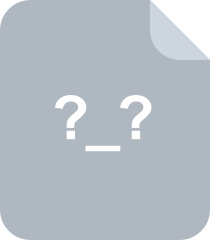
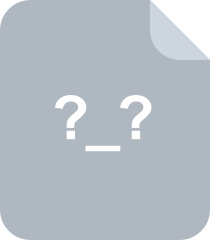
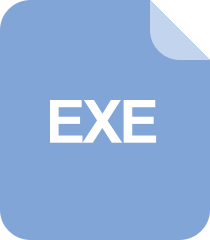
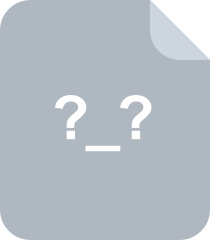
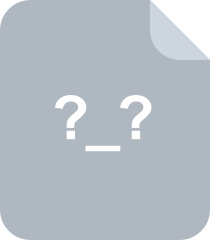
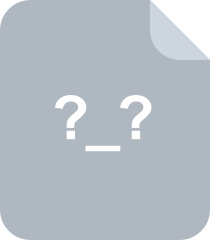
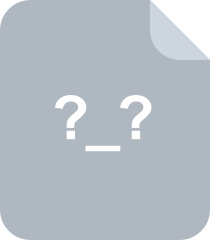
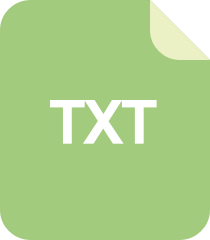
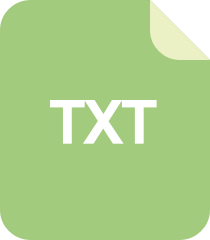
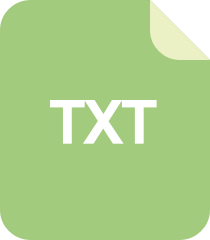
共 69 条
- 1
资源评论
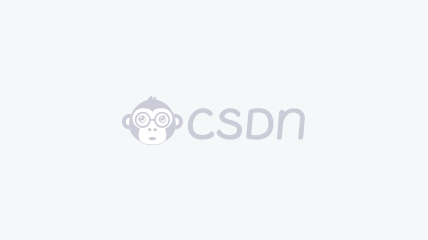


EasySoft易软
- 粉丝: 4356
- 资源: 1620
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

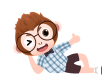
最新资源
- 全球健康统计数据,多个国家,多年的疾病、治疗数据集(100万条数据)
- 基于Springboot+Vue火锅店订餐购物管理系统-毕业源码案例设计(95分以上).zip
- 基于Springboot+Vue技术的实验室管理系统-毕业源码案例设计(高分项目).zip
- 基于Springboot+Vue华强北商城二手手机管理系统-毕业源码案例设计(源码+论文).zip
- 航空旅客满意度数据集.zip
- EXFO FIP-400B系列光纤端面检测仪介绍
- 同学聚会ppt模板,21页,风格怀旧
- c语言实现快速排序基础
- c语言实现冒泡排序基础
- 天气状况分类数据集.zip
- Delphi 12 控件之BitmapStyleDesigner.7z
- 54484-数据结构与算法(C语言篇)-源代码.zip.zip
- c语言-实现堆排序基础
- xshell , 绿色, 可用
- C#与海康VM联合开发,C#与海康visionmaster联合开发,C#基于海康视觉VM4.1/VM4.2/VM4.3的二次开发框架源码,需要安装VM及加密狗 框架保证运行
- c语言实现归并排序基础
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


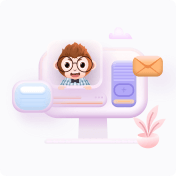
安全验证
文档复制为VIP权益,开通VIP直接复制
