没有合适的资源?快使用搜索试试~ 我知道了~
Chapter 13_ Data Quality Bitmasks Google Earth Engine.pdf
0 下载量 108 浏览量
2024-11-04
10:22:17
上传
评论
收藏 355KB PDF 举报
温馨提示
Google Earth Engine训练教程
资源推荐
资源详情
资源评论
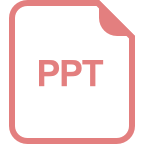
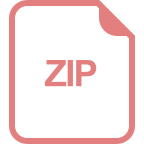
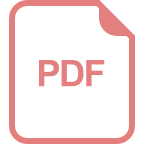
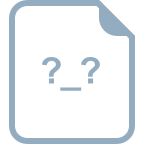
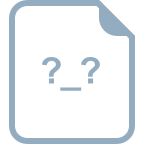
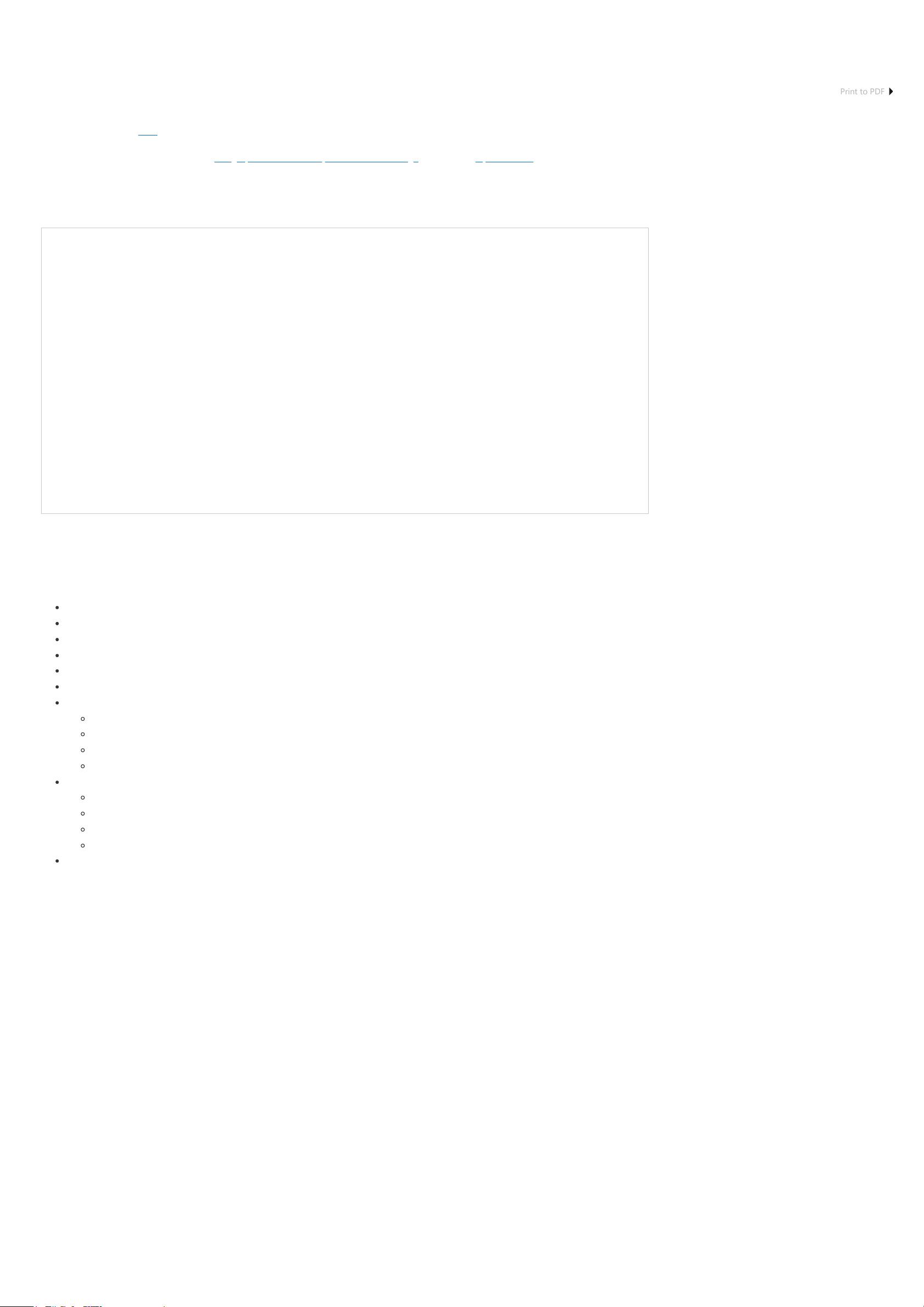
Chapter 13: Data Quality Bitmasks
This chapter provides a workow to explore quality ag bitmasks for imagery in Rocky Mountain National Park, Colorado, United States. The full
GEE code can be found here.
Code for functions was adapted from this Geographic Information Systems Stack Exchange post and this Open Geo Blog post.
Functions
Data Acquisition & Preprocessing
Landsat 8 T1 SR ‘pixel_qa’ band bitmasks:
Bit 0: Fill
Bit 1: Clear
Bit 2: Water
Bit 3: Cloud Shadow
Bit 4: Snow
Bit 5: Cloud
Bits 6-7: Cloud Condence
0: None
1: Low
2: Medium
3: High
Bits 8-9: Cirrus Condence
0: None
1: Low
2: Medium
3: High
Bit 10: Terrain Occlusion
Bitmask value of 1 for single bits means ag is set (i.e. water == 1 –> pixel is water). Bitmask value for multiple bits indicates condition (cirrus
condence of 3).
/**
* Extracts QA values from an image
* @param {ee.Image} qa_band - Single-band image of the QA layer
* @param {Integer} start_bit - Starting bit
* @param {Integer} end_bit - Ending bit
* @param {String} band_name - param_description
* @return {ee.Image} - Image with extracted QA values
*/
function extract_qa_bits(qa_band, start_bit, end_bit, band_name) {
// Initialize QA bit string/pattern to check QA band against
var qa_bits = 0;
// Add each specified QA bit flag value/string/pattern to the QA bits to check/extract
for (var bit = start_bit; bit <= end_bit; bit++) {
qa_bits += Math.pow(2, bit); // Same as qa_bits += (1 << bit);
}
// Return a single band image of the extracted QA bit values
return qa_band
// Rename output band to specified name
.select([0], [band_name])
// Check QA band against specified QA bits to see what QA flag values are set
.bitwiseAnd(qa_bits)
// Get value that matches bitmask documentation
// (0 or 1 for single bit, 0-3 or 0-N for multiple bits)
.rightShift(start_bit);
}
Print to PDF
2024/11/4 10:17
Chapter 13: Data Quality Bitmasks — Remote Sensing with Google Earth Engine
https://calekochenour.github.io/remote-sensing-textbook/03-beginner/chapter13-data-quality-bitmasks.html
1/3
资源评论
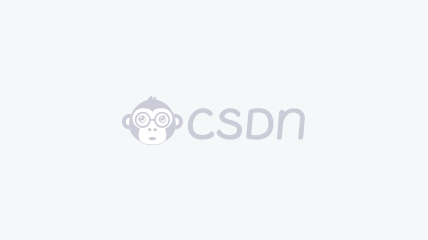


此星光明
- 粉丝: 7w+
- 资源: 1198
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

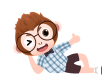
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


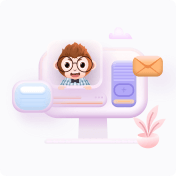
安全验证
文档复制为VIP权益,开通VIP直接复制
