<?php
/*
本代码由 小宜技术猫 www.azpay.cn 创建
严禁反编译、逆向等任何形式的侵权行为,违者将追究法律责任
*/
class App
{
/*高德地图开发者key*/
private $key = 'c6f191bab17453c6571eab1c63430595';
/*是否允许空refer,0为不允许,1为允许*/
public $referopen = "1";
/**路径 */
private $path = __DIR__ . "/";
// 浏览器信息
public $browser = null;
// 系统信息
public $os = null;
// 访问者IP
public $ip = null;
// 城市代码
public $adcode = null;
// 天气
public $weather = null;
// 图片
public $drawIm = null;
// 先定义一个数组
public $weekarray = array("日", "一", "二", "三", "四", "五", "六");
// 城市
public $city = "";
// 省份
public $province = "";
// 初始化
public function init()
{
// 获取浏览器信息
$this->browser = $this->getBrowser();
// 获取系统信息
$this->os = $this->getOs();
// 访问者IP
$this->ip = $_SERVER["REMOTE_ADDR"];
// $this->ip = '222.216.222.168';
// 获取访问者信息
$this->getAddress();
// 绘制图片
$this->picInit()->setWeather()->setIcon()->setInfo();
//
return $this;
}
/**
* 设置信息
*/
public function setInfo()
{
/*基本项文字位置*/
//标语
$by_text = "欢迎访问";
$by_size = "12"; //文字大小
$by_angle = "0"; //角度
$by_x = "60";
$by_y = "45";
$by_font = $this->path . "font/msyh.ttf"; //字体位置
$by_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//自定义文字
$diy_size = "20"; //文字大小
$diy_angle = "0"; //角度
$diy_x = "125";
$diy_y = "45";
$diy_font = $this->path . "font/msyh.ttf"; //字体位置
$diy_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//日期
$time_size = "10"; //文字大小
$time_angle = "0"; //角度
$time_x = "167";
$time_y = "194";
$time_font = $this->path . "font/msyh.ttf"; //字体位置
$time_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//位置
$local_size = "10"; //文字大小
$local_angle = "0"; //角度
$local_x = "165";
$local_y = "212";
$local_font = $this->path . "font/msyh.ttf"; //字体位置
$local_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//IP
$ip_size = "10"; //文字大小
$ip_angle = "0"; //角度
$ip_x = "165";
$ip_y = "232";
$ip_font = $this->path . "font/msyh.ttf"; //字体位置
$ip_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//系统
$system_size = "10"; //文字大小
$system_angle = "0"; //角度
$system_x = "278";
$system_y = "212";
$system_font = $this->path . "font/msyh.ttf"; //字体位置
$system_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
//浏览器
$bro_size = "10"; //文字大小
$bro_angle = "0"; //角度
$bro_x = "278";
$bro_y = "232";
$bro_font = $this->path . "font/msyh.ttf"; //字体位置
$bro_color = imagecolorallocate($this->drawIm, 0, 51, 78); //这里可以自定义自己需要的颜色,但需要转为RGB值,可将0,51,78修改为自己需要的颜色的RGB值
imagettftext($this->drawIm, $time_size, $time_angle, $time_x, $time_y, $time_color, $time_font, date('Y年n月j日') . " 星期" . $this->weekarray[date("w")]);
imagettftext($this->drawIm, $local_size, $local_angle, $local_x, $local_y, $local_color, $local_font, $this->province . '-' . $this->city);
imagettftext($this->drawIm, $by_size, $by_angle, $by_x, $by_y, $by_color, $by_font, $by_text); //标语添加到图片
$_diy = isset($_GET["diy"]) ? $_GET["diy"] : "";
imagettftext($this->drawIm, $diy_size, $diy_angle, $diy_x, $diy_y, $diy_color, $diy_font, base64_decode($_diy)); //自定义文字添加到图片
imagettftext($this->drawIm, $ip_size, $ip_angle, $ip_x, $ip_y, $ip_color, $ip_font, $this->ip); //IP添加到图片
imagettftext($this->drawIm, $system_size, $system_angle, $system_x, $system_y, $system_color, $system_font, $this->os); //系统添加到图片
imagettftext($this->drawIm, $bro_size, $bro_angle, $bro_x, $bro_y, $bro_color, $bro_font, $this->browser); //浏览器添加到图片
return $this;
}
/**
* 初始化画布
*/
public function picInit()
{
//背景图片名称(名称.格式,如"bg.png")
$dst_path = $this->path . 'img/bg.png';
//传递背景图片
$this->drawIm = imagecreatefromstring(file_get_contents($dst_path));
return $this;
}
/**
* 绘制天气部分
*/
public function setWeather()
{
/*基本图标位置*/
//天气图标位置
$weather_icon_x = "65";
$weather_icon_y = "50";
//天气图标
$sunny_path = $this->path . 'icon/weather/sunny.png';
$sunny_im = imagecreatefromstring(file_get_contents($sunny_path));
list($sunny_w, $sunny_h) = getimagesize($sunny_path);
$rain_path = $this->path . 'icon/weather/rain.png';
$rain_im = imagecreatefromstring(file_get_contents($rain_path));
list($rain_w, $rain_h) = getimagesize($rain_path);
$snow_path = $this->path . 'icon/weather/snow.png';
$snow_im = imagecreatefromstring(file_get_contents($snow_path));
list($snow_w, $snow_h) = getimagesize($snow_path);
$sha_path = $this->path . 'icon/weather/sha.png';
$sha_im = imagecreatefromstring(file_get_contents($sha_path));
list($sha_w, $sha_h) = getimagesize($sha_path);
$wu_path = $this->path . 'icon/weather/wu.png';
$wu_im = imagecreatefromstring(file_get_contents($wu_path));
list($wu_w, $wu_h) = getimagesize($wu_path);
$yin_path = $this->path . 'icon/weather/yin.png';
$yin_im = imagecreatefromstring(file_get_contents($yin_path));
list($yin_w, $yin_h) = getimagesize($yin_path);
$dyun_path = $this->path . 'icon/weather/dyun.png';
$dyun_im = imagecreatefromstring(file_get_contents($dyun_path));
list($dyun_w, $dyun_h) = getimagesize($dyun_path);
$unknow_path = $this->path . 'icon/weather/unknow.png';
$unknow_im = imagecreatefromstring(file_get_contents($unknow_path));
list($unknow_w, $unknow_h) = getimagesize($unknow_path);
// 判断天气
if (strpos($this->weather['weather'], '晴') !== false) {
imagecopy($this->drawIm, $sunny_im, $weather_icon_x, $weather_icon_y, 0, 0, $sunny_w, $sunny_h);
} elseif (strpos($this->weather['weather'], '云') !== false) {
imagecopy($this->drawIm, $dyun_im, $weather_icon_x, $weather_icon_y, 0, 0

星尘库
- 粉丝: 1429
- 资源: 82
最新资源
- 410.基于SpringBoot的高校科研信息管理系统(含报告).zip
- 附件1.植物健康状态的影响指标数据.xlsx
- Windows 10 1507-x86 .NET Framework 3.5(包括.NET 2.0和3.0)安装包
- Image_1732500699692.png
- Windows 10 21h1-x86 .NET Framework 3.5(包括.NET 2.0和3.0)安装包
- VMware 是一款功能强大的虚拟化软件,它允许用户在一台物理计算机上同时运行多个操作系统
- 31万条全国医药价格与采购数据.xlsx
- SQL注入详解,SQL 注入是一种常见的网络安全漏洞,攻击者通过在输入数据中插入恶意的 SQL 语句,欺骗应用程序执行这些恶意语句,从而获取、修改或删除数据库中的数据,甚至控制数据库服务器
- 用C语言实现哈夫曼编码:从原理到实现的详细解析
- py爱心代码高级粒子!!
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


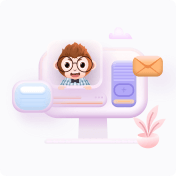