package com.youngch.common.myapplication.mqtt.service;
import android.annotation.SuppressLint;
import android.os.Bundle;
import android.os.PowerManager;
import android.util.Log;
import org.eclipse.paho.client.mqttv3.DisconnectedBufferOptions;
import org.eclipse.paho.client.mqttv3.IMqttActionListener;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.IMqttMessageListener;
import org.eclipse.paho.client.mqttv3.IMqttToken;
import org.eclipse.paho.client.mqttv3.MqttAsyncClient;
import org.eclipse.paho.client.mqttv3.MqttCallbackExtended;
import org.eclipse.paho.client.mqttv3.MqttClientPersistence;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.eclipse.paho.client.mqttv3.persist.MqttDefaultFilePersistence;
import com.youngch.common.myapplication.mqtt.service.MessageStore.StoredMessage;
import java.io.File;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import android.os.PowerManager.WakeLock;
/**
* Created by ZhangHs on 2018/4/20.
*/
public class MqttConnection implements MqttCallbackExtended {
private static final String TAG = "MqttConnection";
private static final String NOT_CONNECTED = "not connected";
private String serverURI;
private String clientId;
private MqttClientPersistence persistence = null;
private MqttConnectOptions connectOptions;
private String clientHandle;
private String reconnectActivityToken = null;
private MqttAsyncClient myClient = null;
private AlarmPingSender alarmPingSender = null;
private MqttService service = null;
private volatile boolean disconnected = true;
private boolean cleanSession = true;
private volatile boolean isConnecting = false;
private Map<IMqttDeliveryToken, String> savedTopics = new HashMap();
private Map<IMqttDeliveryToken, MqttMessage> savedSentMessages = new HashMap();
private Map<IMqttDeliveryToken, String> savedActivityTokens = new HashMap();
private Map<IMqttDeliveryToken, String> savedInvocationContexts = new HashMap();
private WakeLock wakelock = null;
private String wakeLockTag = null;
private DisconnectedBufferOptions bufferOpts = null;
public String getServerURI() {
return this.serverURI;
}
public void setServerURI(String serverURI) {
this.serverURI = serverURI;
}
public String getClientId() {
return this.clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public MqttConnectOptions getConnectOptions() {
return this.connectOptions;
}
public void setConnectOptions(MqttConnectOptions connectOptions) {
this.connectOptions = connectOptions;
}
public String getClientHandle() {
return this.clientHandle;
}
public void setClientHandle(String clientHandle) {
this.clientHandle = clientHandle;
}
MqttConnection(MqttService service, String serverURI, String clientId, MqttClientPersistence persistence, String clientHandle) {
this.serverURI = serverURI;
this.service = service;
this.clientId = clientId;
this.persistence = persistence;
this.clientHandle = clientHandle;
StringBuilder stringBuilder = new StringBuilder(this.getClass().getCanonicalName());
stringBuilder.append(" ");
stringBuilder.append(clientId);
stringBuilder.append(" ");
stringBuilder.append("on host ");
stringBuilder.append(serverURI);
this.wakeLockTag = stringBuilder.toString();
}
public void connect(MqttConnectOptions options, String invocationContext, String activityToken) {
this.connectOptions = options;
this.reconnectActivityToken = activityToken;
if(options != null) {
this.cleanSession = options.isCleanSession();
}
if(this.connectOptions.isCleanSession()) {
this.service.messageStore.clearArrivedMessages(this.clientHandle);
}
this.service.traceDebug(TAG, "Connecting {" + this.serverURI + "} as {" + this.clientId + "}");
final Bundle resultBundle = new Bundle();
resultBundle.putString(MqttServiceConstants.CALLBACK_ACTIVITY_TOKEN, activityToken);
resultBundle.putString(MqttServiceConstants.CALLBACK_INVOCATION_CONTEXT, invocationContext);
resultBundle.putString(MqttServiceConstants.CALLBACK_ACTION, "connect");
try {
if(this.persistence == null) {
File myDir = this.service.getExternalFilesDir(TAG);
if(myDir == null) {
myDir = this.service.getDir(TAG, 0);
if(myDir == null) {
resultBundle.putString(MqttServiceConstants.CALLBACK_ERROR_MESSAGE, "Error! No external and internal storage available");
resultBundle.putSerializable(MqttServiceConstants.CALLBACK_EXCEPTION, new MqttPersistenceException());
this.service.callbackToActivity(this.clientHandle, Status.ERROR, resultBundle);
return;
}
}
this.persistence = new MqttDefaultFilePersistence(myDir.getAbsolutePath());
}
IMqttActionListener listener = new MqttConnection.MqttConnectionListener(resultBundle) {
public void onSuccess(IMqttToken asyncActionToken) {
MqttConnection.this.doAfterConnectSuccess(resultBundle);
MqttConnection.this.service.traceDebug(TAG, "connect success!");
}
public void onFailure(IMqttToken asyncActionToken, Throwable exception) {
resultBundle.putString(MqttServiceConstants.CALLBACK_ERROR_MESSAGE, exception.getLocalizedMessage());
resultBundle.putSerializable(MqttServiceConstants.CALLBACK_EXCEPTION, exception);
MqttConnection.this.service.traceError(TAG, "connect fail, call connect to reconnect.reason:" + exception.getMessage());
MqttConnection.this.doAfterConnectFail(resultBundle);
}
};
if(this.myClient != null) {
if(this.isConnecting) {
this.service.traceDebug(TAG, "myClient != null and the client is connecting. Connect return directly.");
this.service.traceDebug(TAG, "Connect return:isConnecting:" + this.isConnecting + ".disconnected:" + this.disconnected);
} else if(!this.disconnected) {
this.service.traceDebug(TAG, "myClient != null and the client is connected and notify!");
this.doAfterConnectSuccess(resultBundle);
} else {
this.service.traceDebug(TAG, "myClient != null and the client is not connected");
this.service.traceDebug(TAG, "Do Real connect!");
this.setConnectingState(true);
this.myClient.connect(this.connectOptions, invocationContext, listener);
}
} else {
this.alarmPingSender = new AlarmPingSender(this.service);
this.myClient = new MqttAsyncClient(this.serverURI, this.clientId, this.persistence, this.alarmPingSender);
this.myClient.setCallback(this);
this.service.traceDebug(TAG, "Do Real connect!");
this.setConnectingState(true);
this.myClient.connect(this.connectOptions, invocationContext, listener);
}
} catch (Exception var6) {
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
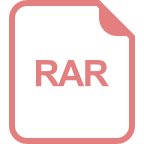
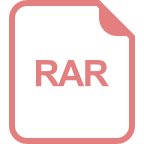
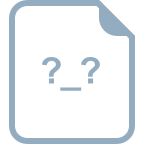
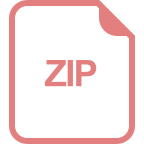
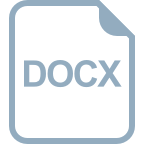
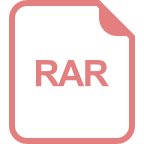
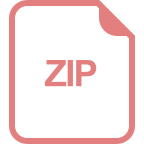
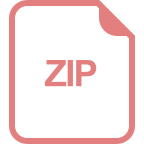
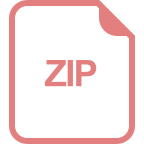
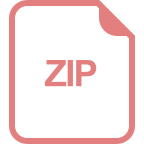
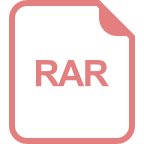
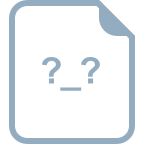
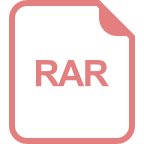
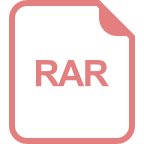
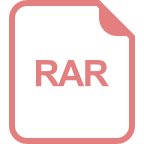
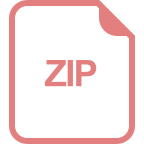
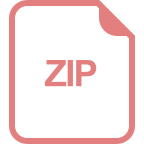
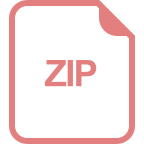
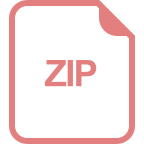
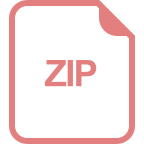
收起资源包目录


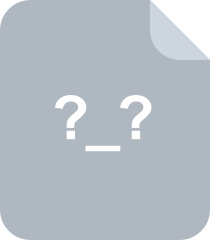

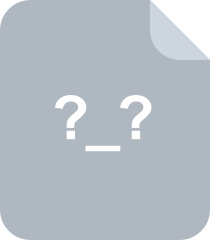
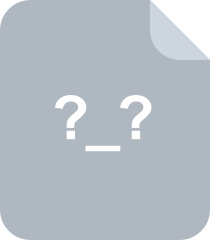
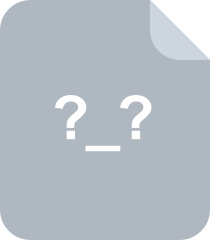
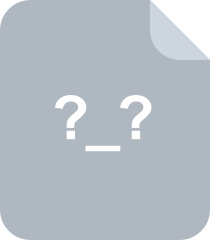
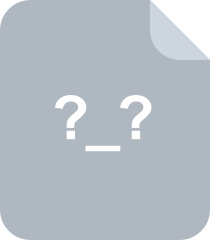
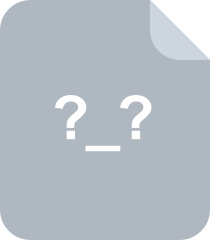
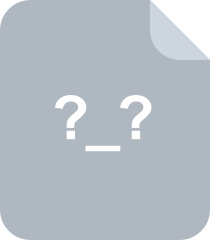
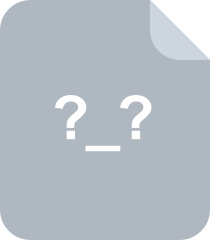
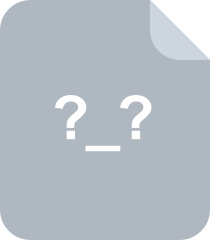
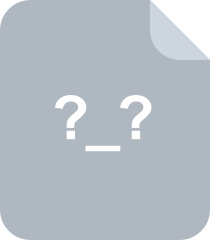
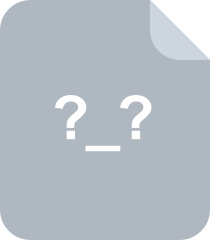
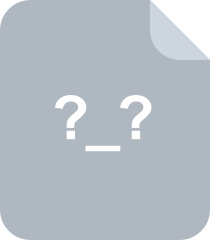
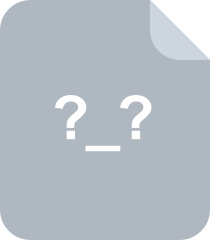
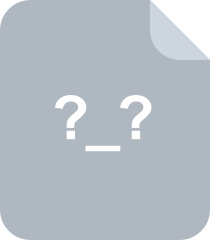
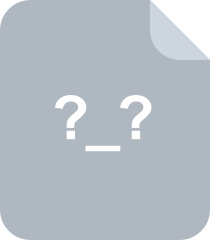
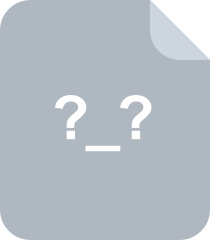
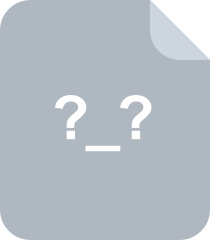
共 18 条
- 1
资源评论
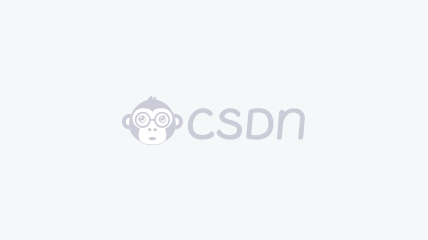
- wjfgps2019-08-12不会用,怎么办。。。。。。慈眉善目张先森2019-08-12我博客里有教程
- hngsyz2018-12-10activemq配套使用

慈眉善目张先森
- 粉丝: 25
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

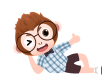
安全验证
文档复制为VIP权益,开通VIP直接复制
