package com.myutils.l;
import android.util.Log;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @author zengmiaosen
* @email 1510809124@qq.com
* @CreateDate 2016/9/22 1:39
* @Descrition 日志打印
*/
public class L {
//是否打印
private static boolean isLog = false;
//打印标签
private static String logtag = "logtag";
/**
* logcat在实现上对于message的内存分配大概是4k左右,
* 所以超过的内容都直接被丢弃,设置文本长度超过3000分多条打印
*/
private final static int LOG_MAXLENGTH = 3000;
//记录上次的logLocation
private static String lastLogMethod = "";
/**
* 打印文本
*
* @param text
*/
public static void i(String text) {
if (isLog) {
Log.i(logtag, logContent(text) + logLocation());
}
}
/**
* 打印异常文本
*
* @param text
*/
public static void e(String text) {
if (isLog) {
Log.e(logtag, logContent(text) + logLocation());
}
}
/**
* 打印异常
*
* @param msg
* @param e
*/
public static void e(String msg, Exception e) {
if (isLog) {
Log.e(logtag, msg + logLocation(), e);
}
}
/**
* 打印json格式文本
*
* @param json
*/
public static void json(String json) {
if (isLog) {
json("", json);
}
}
/**
* 打印json格式文本
*
* @param prefix 前缀文本
* @param json
*/
public static void json(String prefix, String json) {
if (isLog) {
String text = prefix + fomatJson(json);
Log.i(logtag, logContent(text) + logLocation());
}
}
/**
* 打印内容
*
* @param text
* @return
*/
private static String logContent(String text) {
if (text.length() < 50) {//内容长度不超过50,前面加空格加到50
int minLeng = 50 - text.length();
// Log.i(logtag, "leng========" + leng + " " + text.length());
if (minLeng > 0) {
StringBuilder stringBuilder = new StringBuilder();
for (int i = 0; i < minLeng; i++) {
stringBuilder.append(" ");
}
text = text + stringBuilder.toString();
}
} else if (text.length() > LOG_MAXLENGTH) {//内容超过logcat单条打印上限,分批打印
//Log.i(logtag,"text长度========="+text.length());
int logTime = text.length() / LOG_MAXLENGTH;
for (int i = 0; i < logTime; i++) {
String leng = text.substring(i * LOG_MAXLENGTH, (i + 1) * LOG_MAXLENGTH);
//提示
if (i == 0) {
Log.i(logtag, "打印分" + (logTime+1) + "条显示 :" + leng);
} else {
Log.i(logtag, "接上条↑" + leng);
}
}
text = text.substring(logTime * LOG_MAXLENGTH, text.length());
}
return text;
}
/**
* 定位打印的方法
*
* @return
*/
private static StringBuilder logLocation() {
StackTraceElement logStackTrace = getLogStackTrace();
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append(" ☞.(").append(logStackTrace.getFileName()).append(":").append(logStackTrace.getLineNumber() + ")");
//Log.i(logtag, "leng========" + stringBuilder + " " + lastLogMethod);
if (stringBuilder.toString().equals(lastLogMethod)) {
stringBuilder = new StringBuilder("");
} else {
lastLogMethod = stringBuilder.toString();
}
return stringBuilder;
}
/**
* json格式化
*
* @param jsonStr
* @return
*/
private static String fomatJson(String jsonStr) {
try {
jsonStr = jsonStr.trim();
if (jsonStr.startsWith("{")) {
JSONObject jsonObject = new JSONObject(jsonStr);
return jsonObject.toString(2);
}
if (jsonStr.startsWith("[")) {
JSONArray jsonArray = new JSONArray(jsonStr);
return jsonArray.toString(2);
}
} catch (JSONException e) {
e.printStackTrace();
}
return "Json格式有误: " + jsonStr;
}
/**
* 获取打印的方法栈
*
* @return
*/
private static StackTraceElement getLogStackTrace() {
StackTraceElement logTackTraces = null;
StackTraceElement[] stackTraces = Thread.currentThread().getStackTrace();
for (int i = 0; i < stackTraces.length; i++) {
StackTraceElement stackTrace = stackTraces[i];
if (stackTrace.getClassName().equals(L.class.getName())) {
//getLogStackTrace--logLocation--i/e/json--方法栈,所以方法栈的位置是当前方法栈后的第3个
logTackTraces = stackTraces[i + 3];
i = stackTraces.length;
}
}
return logTackTraces;
}
public static void setLogtag(String logtag) {
L.logtag = logtag;
}
public static void setIsLog(boolean isLog) {
L.isLog = isLog;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Android Log 封装
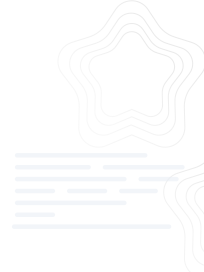
共37个文件
xml:15个
png:5个
java:5个


温馨提示
代码在github上维护:https://github.com/miaosen/L 1,文本打印,异常打印,json格式化打印 2,定位打印方法的位置,可以点击跳转到调用打印的位置 2,解决logcat打印不全问题(文本长度超过3500,分多条log打印) 3,全局的打印开关、打印标签(默认为logtag)
资源推荐
资源详情
资源评论
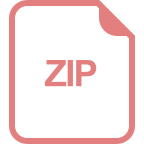
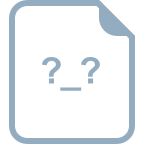
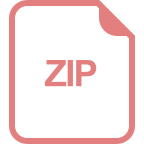
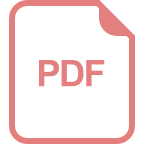
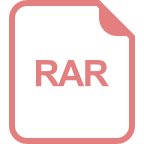
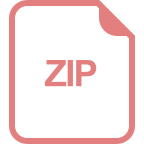
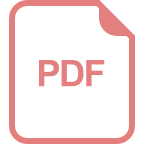
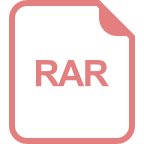
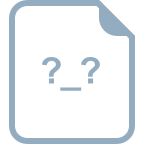
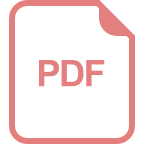
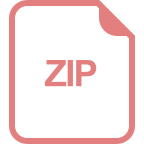
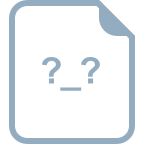
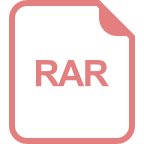
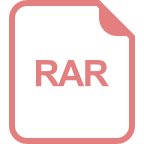
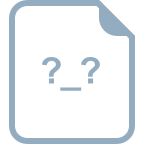
收起资源包目录


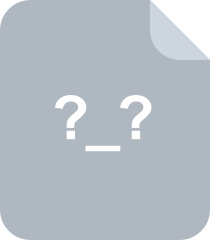
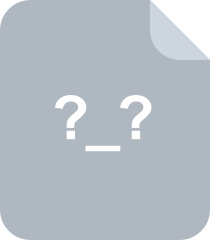

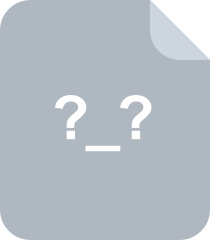
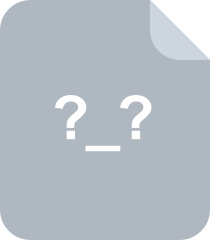
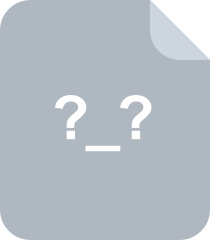
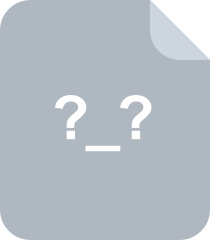
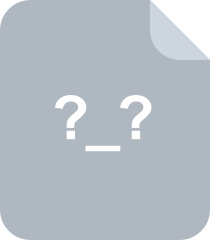
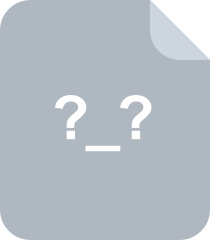
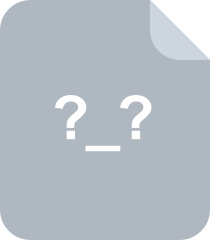

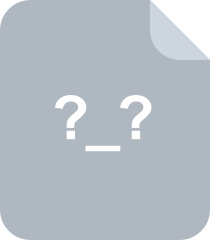
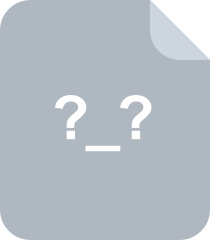


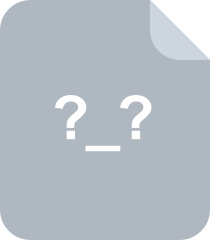
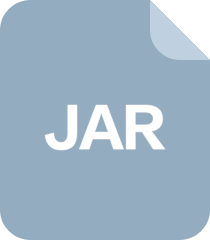
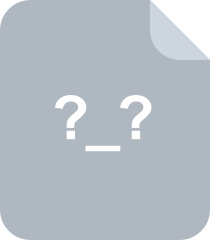
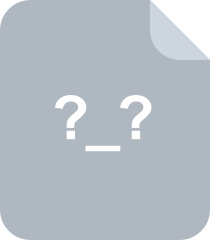
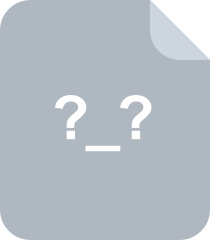







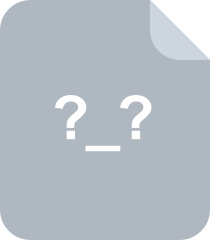





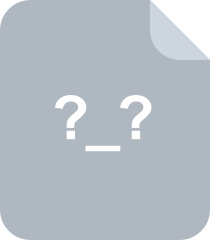

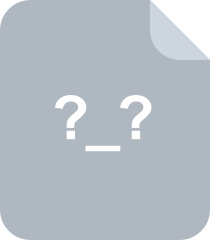


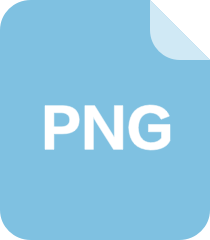

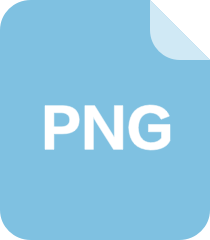

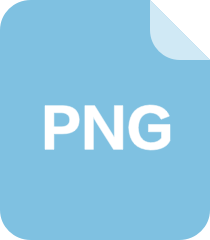

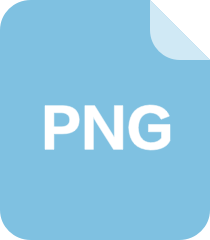

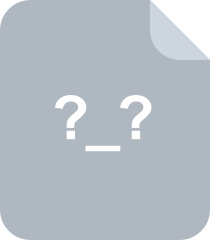

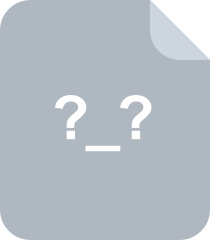

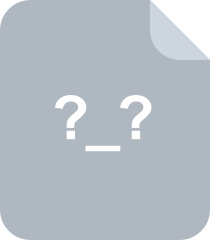
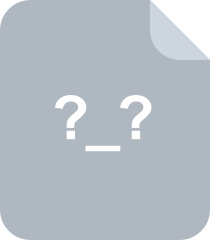
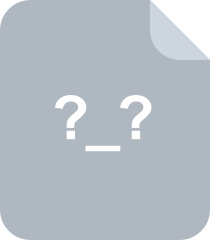
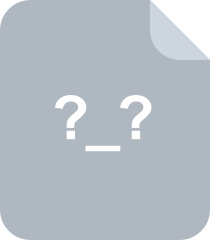

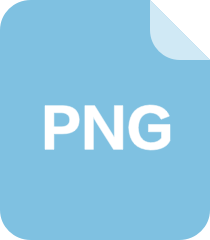




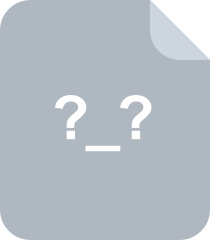
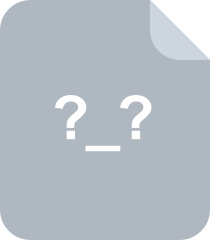
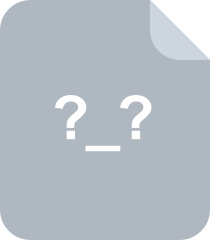
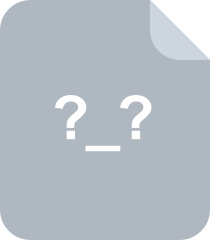
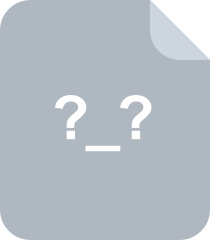
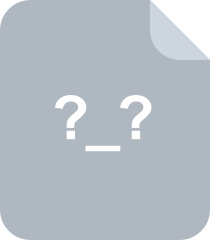
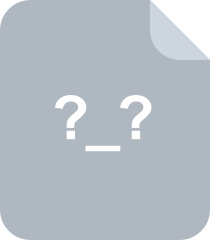
共 37 条
- 1
资源评论
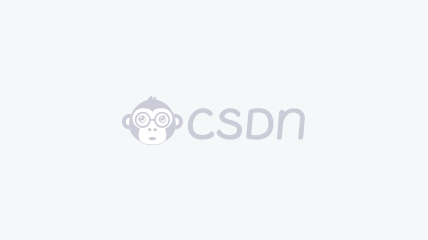
- 长安三日2020-03-21不错,学习了,代码简单易懂

淼森大帝
- 粉丝: 1
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

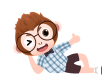
最新资源
- 动物位移小游戏Java实现,强行使用上了SQLite和MyBatis.zip
- 叠罗汉游戏,安卓java实现,自定义Framlayout,属性动画.zip
- java项目实战练习.zip
- java桌面小程序,主要为游戏.zip学习资料
- 2021级大三上学期计算机体系结构-期末大作业复现代码.zip
- ember前端框架,一键部署到云开发平台.zip
- kero is a front-end model framework. - kero是一个前端模型框架,做为MVVM架构中Model层的增强,提供多维数据模型.zip
- PandaUi 是PandaX的前端框架,PandaX 是golang(go)语言微服务开发架构.zip
- v8垃圾回收机制 一篇技术分享文章
- libre后台管理系统前端,使用vue2开发.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


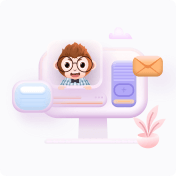
安全验证
文档复制为VIP权益,开通VIP直接复制
