English | [中文](./README-CN.md)
# Swoole
[](https://github.com/swoole/swoole-src/releases)
[](https://travis-ci.org/swoole/swoole-src)
[](LICENSE)
[](https://gitter.im/swoole/swoole-src?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://scan.coverity.com/projects/swoole-swoole-src)
[](#backers)
[](#sponsors)

**Swoole is an event-driven asynchronous & coroutine-based concurrency networking communication engine with high performance written in C and C++ for PHP.**
## ✨Event-based
The network layer in Swoole is event-based and takes full advantage of the underlying epoll/kqueue implementation, making it really easy to serve millions of requests.
Swoole 4.x uses a brand new engine kernel and now it has a full-time developer team, so we are entering an unprecedented period in PHP history which offers a unique possibility for rapid evolution in performance.
## ⚡️Coroutine
Swoole 4.x or later supports the built-in coroutine with high availability, and you can use fully synchronized code to implement asynchronous performance. PHP code without any additional keywords, the underlying automatic coroutine-scheduling.
Developers can understand coroutines as ultra-lightweight threads, and you can easily create thousands of coroutines in a single process.
### MySQL
Concurrency 10K requests to read data from MySQL takes only 0.2s!
```php
$s = microtime(true);
Co\run(function() {
for ($c = 100; $c--;) {
go(function () {
$mysql = new Swoole\Coroutine\MySQL;
$mysql->connect([
'host' => '127.0.0.1',
'user' => 'root',
'password' => 'root',
'database' => 'test'
]);
$statement = $mysql->prepare('SELECT * FROM `user`');
for ($n = 100; $n--;) {
$result = $statement->execute();
assert(count($result) > 0);
}
});
}
});
echo 'use ' . (microtime(true) - $s) . ' s';
```
### Mixed server
You can create multiple services on the single event loop: TCP, HTTP, Websocket and HTTP2, and easily handle thousands of requests.
```php
function tcp_pack(string $data): string
{
return pack('N', strlen($data)) . $data;
}
function tcp_unpack(string $data): string
{
return substr($data, 4, unpack('N', substr($data, 0, 4))[1]);
}
$tcp_options = [
'open_length_check' => true,
'package_length_type' => 'N',
'package_length_offset' => 0,
'package_body_offset' => 4
];
```
```php
$server = new Swoole\WebSocket\Server('127.0.0.1', 9501, SWOOLE_BASE);
$server->set(['open_http2_protocol' => true]);
// http && http2
$server->on('request', function (Swoole\Http\Request $request, Swoole\Http\Response $response) {
$response->end('Hello ' . $request->rawcontent());
});
// websocket
$server->on('message', function (Swoole\WebSocket\Server $server, Swoole\WebSocket\Frame $frame) {
$server->push($frame->fd, 'Hello ' . $frame->data);
});
// tcp
$tcp_server = $server->listen('127.0.0.1', 9502, SWOOLE_TCP);
$tcp_server->set($tcp_options);
$tcp_server->on('receive', function (Swoole\Server $server, int $fd, int $reactor_id, string $data) {
$server->send($fd, tcp_pack('Hello ' . tcp_unpack($data)));
});
$server->start();
```
### Coroutine clients
Whether you DNS query or send requests or receive responses, all of these are scheduled by coroutine automatically.
```php
go(function () {
// http
$http_client = new Swoole\Coroutine\Http\Client('127.0.0.1', 9501);
assert($http_client->post('/', 'Swoole Http'));
var_dump($http_client->body);
// websocket
$http_client->upgrade('/');
$http_client->push('Swoole Websocket');
var_dump($http_client->recv()->data);
});
go(function () {
// http2
$http2_client = new Swoole\Coroutine\Http2\Client('localhost', 9501);
$http2_client->connect();
$http2_request = new Swoole\Http2\Request;
$http2_request->method = 'POST';
$http2_request->data = 'Swoole Http2';
$http2_client->send($http2_request);
$http2_response = $http2_client->recv();
var_dump($http2_response->data);
});
go(function () use ($tcp_options) {
// tcp
$tcp_client = new Swoole\Coroutine\Client(SWOOLE_TCP);
$tcp_client->set($tcp_options);
$tcp_client->connect('127.0.0.1', 9502);
$tcp_client->send(tcp_pack('Swoole Tcp'));
var_dump(tcp_unpack($tcp_client->recv()));
});
```
### Channel
Channel is the only way for exchanging data between coroutines, the development combination of the `Coroutine + Channel` is the famous CSP programming model.
In Swoole development, Channel is usually used for implementing connection pool or scheduling coroutine concurrent.
#### The simplest example of a connection pool
In the following example, we have a thousand concurrently requests to redis. Normally, this has exceeded the maximum number of Redis connections setting and will throw a connection exception, but the connection pool based on Channel can perfectly schedule requests. We don't have to worry about connection overload.
```php
class RedisPool
{
/**@var \Swoole\Coroutine\Channel */
protected $pool;
/**
* RedisPool constructor.
* @param int $size max connections
*/
public function __construct(int $size = 100)
{
$this->pool = new \Swoole\Coroutine\Channel($size);
for ($i = 0; $i < $size; $i++) {
$redis = new \Swoole\Coroutine\Redis();
$res = $redis->connect('127.0.0.1', 6379);
if ($res == false) {
throw new \RuntimeException("failed to connect redis server.");
} else {
$this->put($redis);
}
}
}
public function get(): \Swoole\Coroutine\Redis
{
return $this->pool->pop();
}
public function put(\Swoole\Coroutine\Redis $redis)
{
$this->pool->push($redis);
}
public function close(): void
{
$this->pool->close();
$this->pool = null;
}
}
go(function () {
$pool = new RedisPool();
// max concurrency num is more than max connections
// but it's no problem, channel will help you with scheduling
for ($c = 0; $c < 1000; $c++) {
go(function () use ($pool, $c) {
for ($n = 0; $n < 100; $n++) {
$redis = $pool->get();
assert($redis->set("awesome-{$c}-{$n}", 'swoole'));
assert($redis->get("awesome-{$c}-{$n}") === 'swoole');
assert($redis->delete("awesome-{$c}-{$n}"));
$pool->put($redis);
}
});
}
});
```
#### Producer and consumers
Some Swoole's clients implement the defer mode for concurrency, but you can still implement it flexible with a combination of coroutines and channels.
```php
go(function () {
// User: I need you to bring me some information back.
// Channel: OK! I will be responsible for scheduling.
$channel = new Swoole\Coroutine\Channel;
go(function () use ($channel) {
// Coroutine A: Ok! I will show you the github addr info
$addr_info = Co::getaddrinfo('github.com');
$channel->push(['A', json_encode($addr_info, JSON_PRETTY_PRINT)]);
});
go(function () use ($channel) {
// Coroutine B: Ok! I will show you what your code look like
$mirror = Co::readFile(__FILE__
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
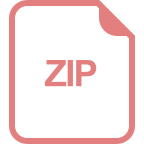
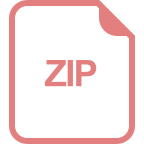
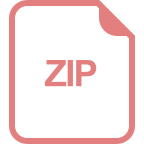
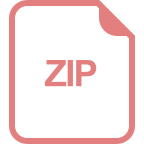
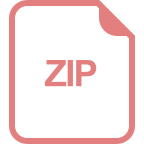
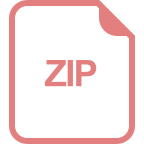
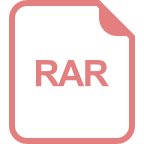
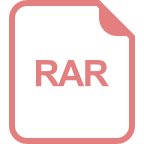
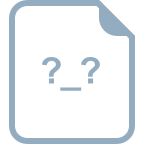
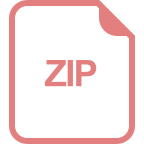
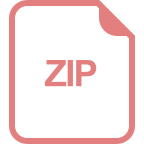
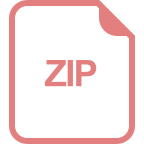
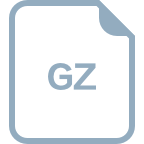
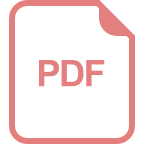
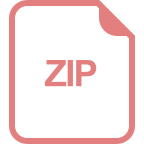
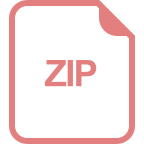
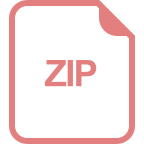
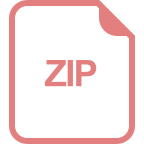
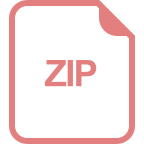
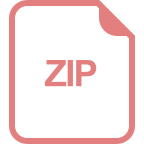
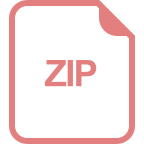
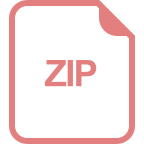
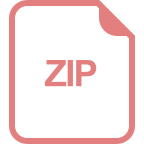
收起资源包目录

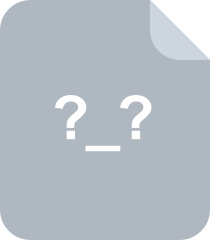
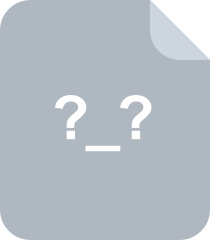
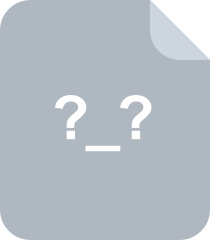
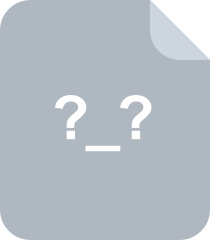
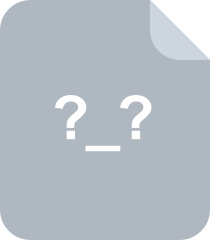
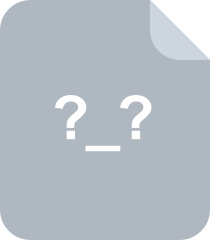
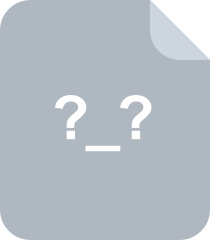
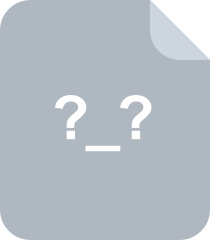
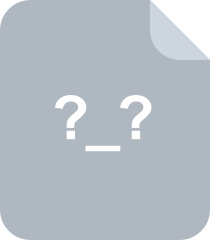
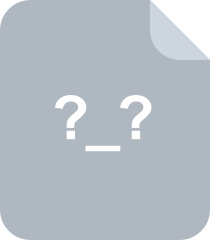
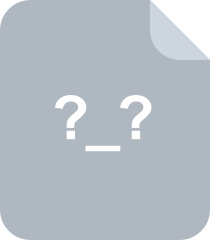
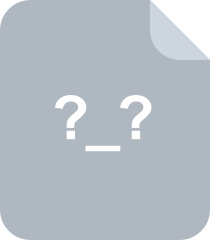
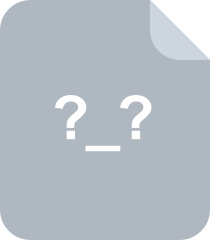
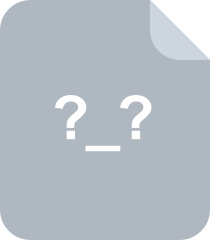
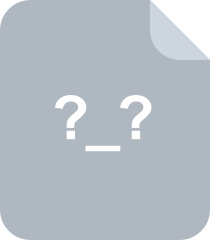
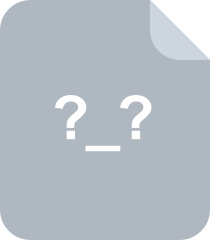
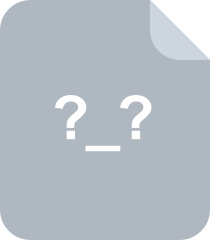
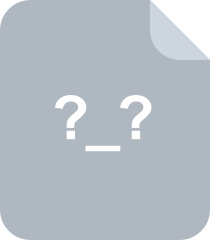
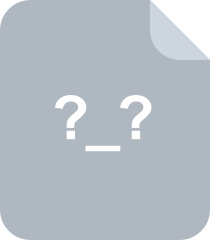
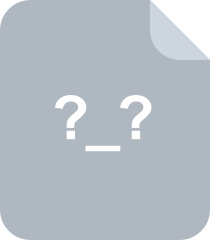
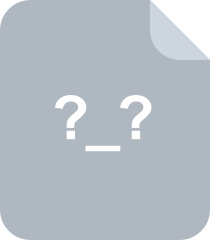
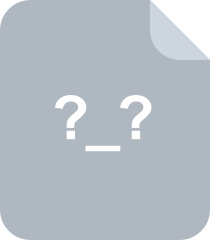
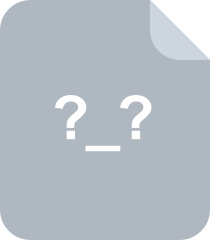
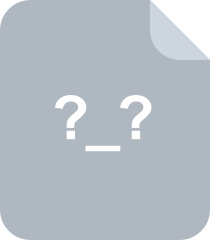
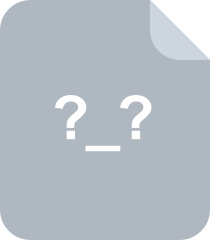
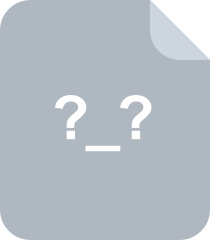
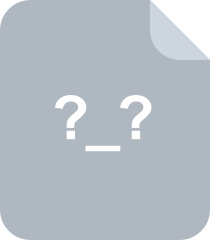
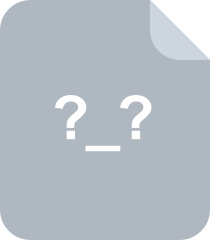
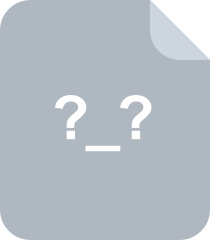
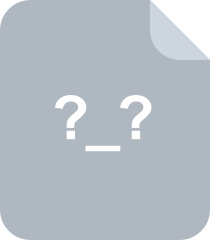
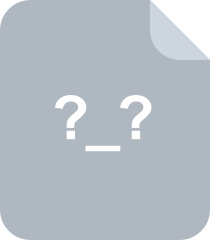
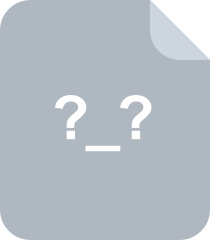
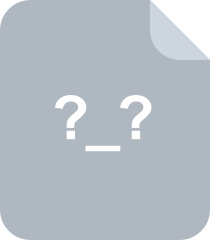
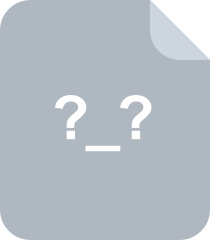
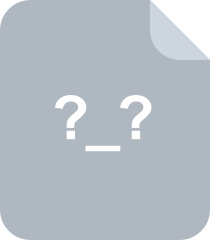
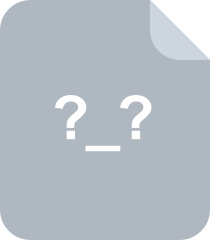
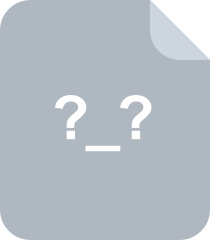
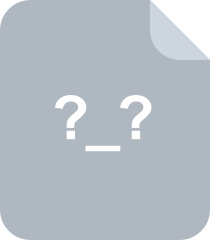
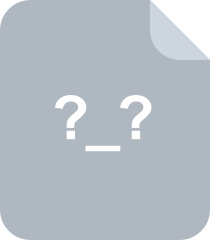
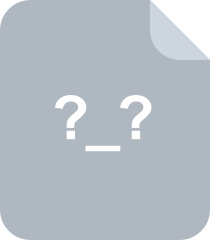
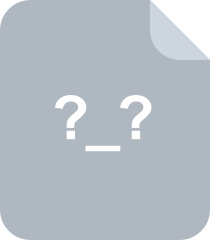
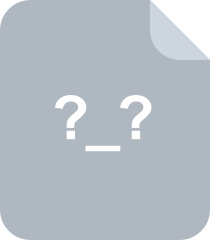
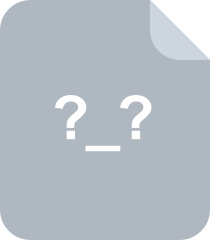
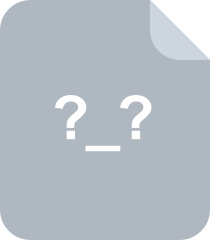
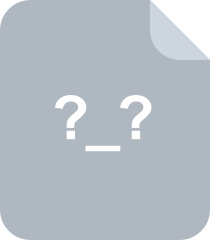
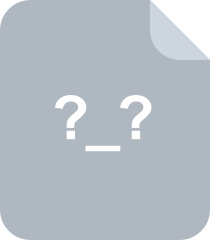
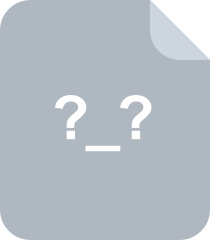
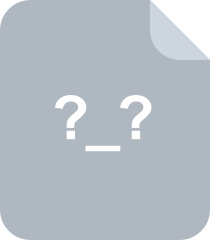
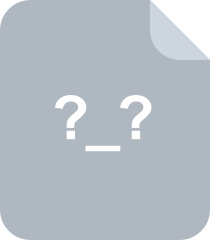
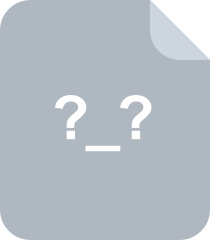
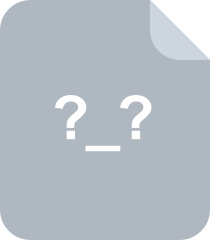
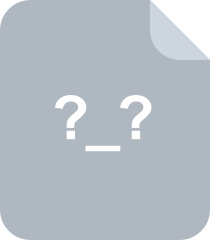
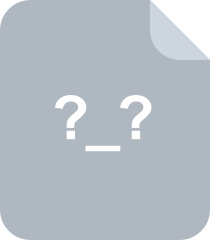
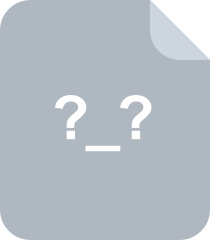
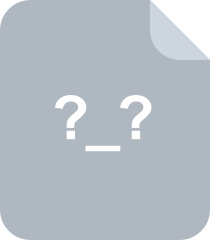
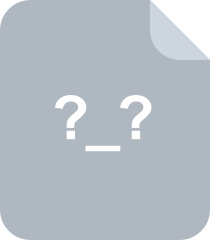
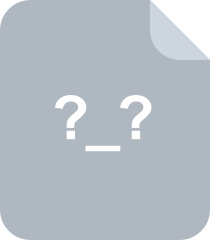
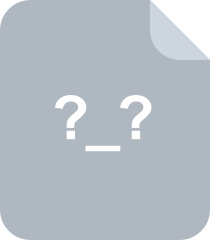
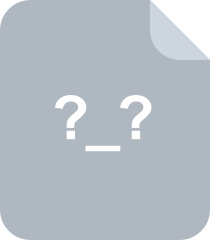
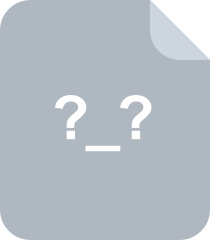
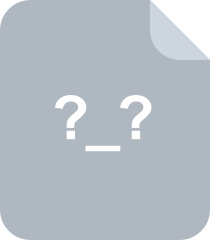
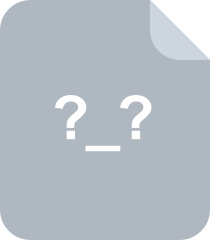
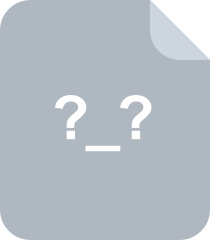
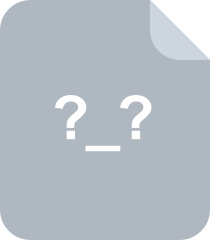
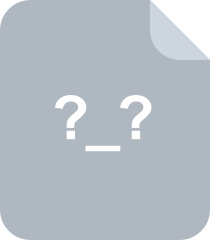
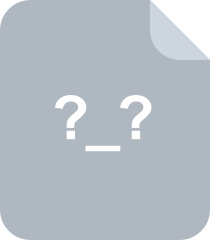
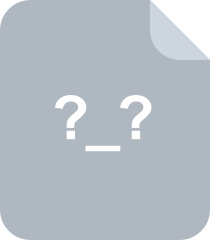
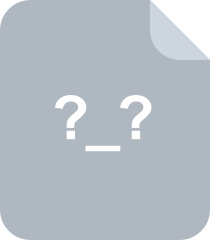
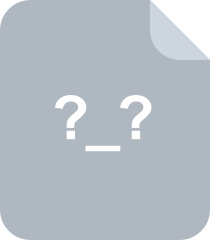
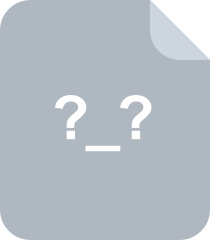
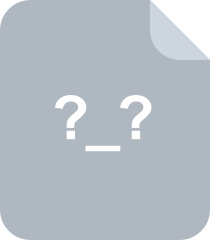
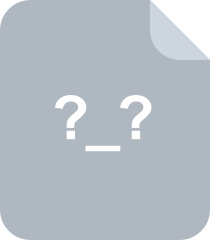
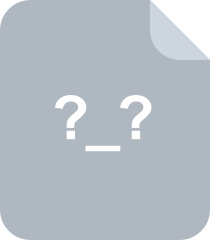
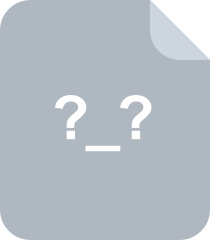
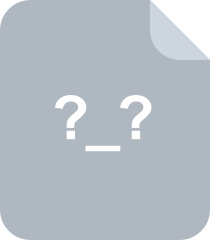
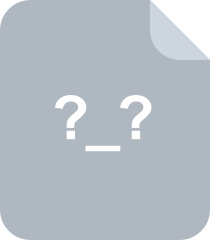
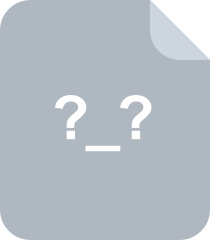
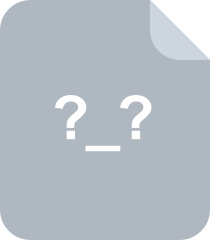
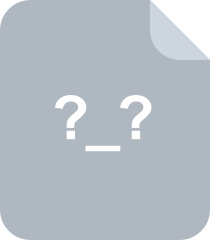
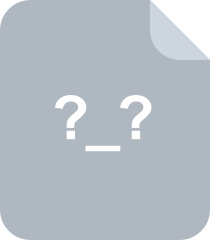
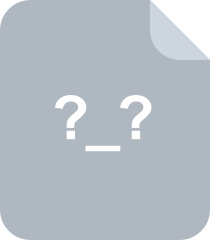
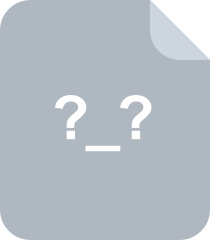
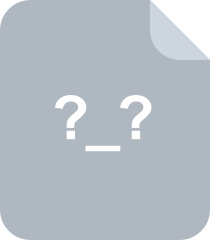
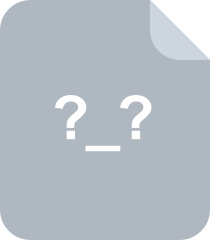
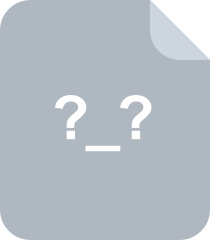
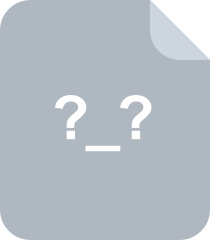
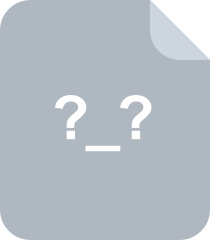
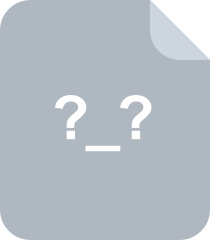
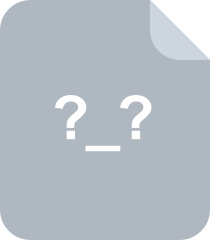
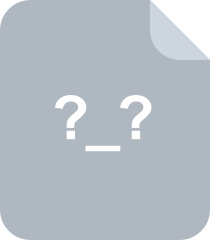
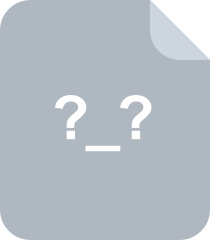
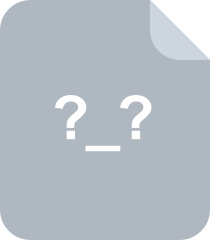
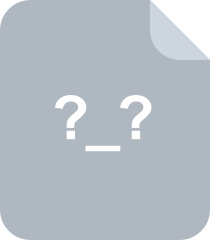
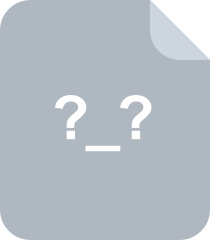
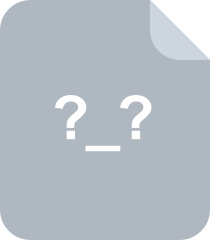
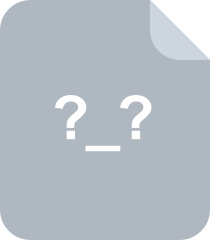
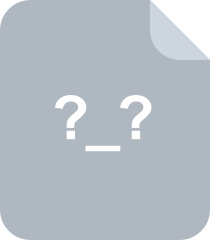
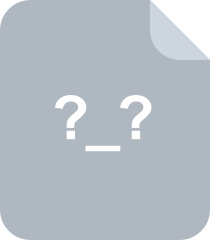
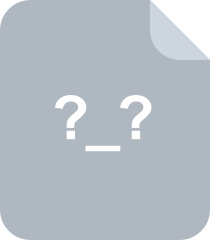
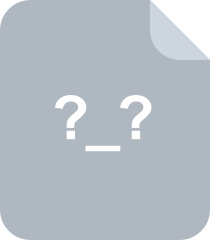
共 1665 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
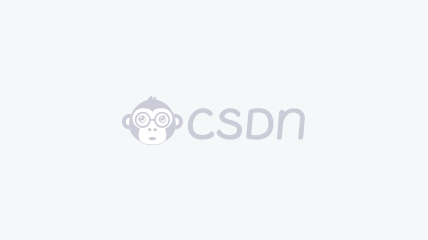

芝麻粒儿
- 粉丝: 6w+
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

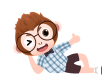
最新资源
- fed54987-3a28-4a7a-9c89-52d3ac6bc048.vsidx
- (177367038)QT实现教务管理系统.zip
- (178041422)基于springboot网上书城系统.zip
- (3127654)超级玛丽游戏源码下载
- (175717016)CTGU单总线CPU设计(变长指令周期3级时序)(HUST)(circ文件)
- (133916396)单总线CPU设计(变长指令周期3级时序)(HUST).rar
- Unity In-game Debug Console
- (3292010)Java图书管理系统(源码)
- Oracle期末复习题:选择题详解与数据库管理技术
- (176721246)200行C++代码写一个Qt俄罗斯方块
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


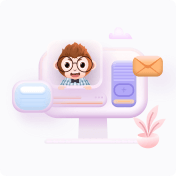
安全验证
文档复制为VIP权益,开通VIP直接复制
