package com.yarin.android.FileManager;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.app.AlertDialog.Builder;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.DialogInterface.OnClickListener;
import android.graphics.drawable.Drawable;
import android.net.Uri;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
public class FileManager extends ListActivity
{
private List<IconifiedText> directoryEntries = new ArrayList<IconifiedText>();
private File currentDirectory = new File("/");
private File myTmpFile = null;
private int myTmpOpt = -1;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle icicle)
{
super.onCreate(icicle);
browseToRoot();
this.setSelection(0);
}
//浏览文件系统的根目录
private void browseToRoot()
{
browseTo(new File("/"));
}
//返回上一级目录
private void upOneLevel()
{
if(this.currentDirectory.getParent() != null)
this.browseTo(this.currentDirectory.getParentFile());
}
//浏览指定的目录,如果是文件则进行打开操作
private void browseTo(final File file)
{
System.out.println(file.getAbsolutePath());
this.setTitle(file.getAbsolutePath());
if (file.isDirectory())
{
this.currentDirectory = file;
fill(file.listFiles());
}
else
{
fileOptMenu(file);
}
}
//打开指定文件
protected void openFile(File aFile)
{
Intent intent = new Intent();
intent.setAction(android.content.Intent.ACTION_VIEW);
File file = new File(aFile.getAbsolutePath());
// 取得文件名
String fileName = file.getName();
// 根据不同的文件类型来打开文件
if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingImage)))
{
intent.setDataAndType(Uri.fromFile(file), "image/*");
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingAudio)))
{
intent.setDataAndType(Uri.fromFile(file), "audio/*");
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingVideo)))
{
intent.setDataAndType(Uri.fromFile(file), "video/*");
}
startActivity(intent);
}
//这里可以理解为设置ListActivity的源
private void fill(File[] files)
{
//清空列表
this.directoryEntries.clear();
//添加一个当前目录的选项
this.directoryEntries.add(new IconifiedText(getString(R.string.current_dir), getResources().getDrawable(R.drawable.folder)));
//如果不是根目录则添加上一级目录项
if (this.currentDirectory.getParent() != null)
this.directoryEntries.add(new IconifiedText(getString(R.string.up_one_level), getResources().getDrawable(R.drawable.uponelevel)));
Drawable currentIcon = null;
for (File currentFile : files)
{
//判断是一个文件夹还是一个文件
if (currentFile.isDirectory())
{
currentIcon = getResources().getDrawable(R.drawable.folder);
}
else
{
//取得文件名
String fileName = currentFile.getName();
//根据文件名来判断文件类型,设置不同的图标
if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingImage)))
{
currentIcon = getResources().getDrawable(R.drawable.image);
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingWebText)))
{
currentIcon = getResources().getDrawable(R.drawable.webtext);
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingPackage)))
{
currentIcon = getResources().getDrawable(R.drawable.packed);
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingAudio)))
{
currentIcon = getResources().getDrawable(R.drawable.audio);
}
else if (checkEndsWithInStringArray(fileName, getResources().getStringArray(R.array.fileEndingVideo)))
{
currentIcon = getResources().getDrawable(R.drawable.video);
}
else
{
currentIcon = getResources().getDrawable(R.drawable.text);
}
}
//确保只显示文件名、不显示路径如:/sdcard/111.txt就只是显示111.txt
int currentPathStringLenght = this.currentDirectory.getAbsolutePath().length();
this.directoryEntries.add(new IconifiedText(currentFile.getAbsolutePath().substring(currentPathStringLenght), currentIcon));
}
Collections.sort(this.directoryEntries);
IconifiedTextListAdapter itla = new IconifiedTextListAdapter(this);
//将表设置到ListAdapter中
itla.setListItems(this.directoryEntries);
//为ListActivity添加一个ListAdapter
this.setListAdapter(itla);
}
protected void onListItemClick(ListView l, View v, int position, long id)
{
super.onListItemClick(l, v, position, id);
// 取得选中的一项的文件名
String selectedFileString = this.directoryEntries.get(position).getText();
if (selectedFileString.equals(getString(R.string.current_dir)))
{
//如果选中的是刷新
this.browseTo(this.currentDirectory);
}
else if (selectedFileString.equals(getString(R.string.up_one_level)))
{
//返回上一级目录
this.upOneLevel();
}
else
{
File clickedFile = null;
clickedFile = new File(this.currentDirectory.getAbsolutePath()+ this.directoryEntries.get(position).getText());
if(clickedFile != null)
this.browseTo(clickedFile);
}
}
//通过文件名判断是什么类型的文件
private boolean checkEndsWithInStringArray(String checkItsEnd,
String[] fileEndings)
{
for(String aEnd : fileEndings)
{
if(checkItsEnd.endsWith(aEnd))
return true;
}
return false;
}
public boolean onCreateOptionsMenu(Menu menu)
{
super.onCreateOptionsMenu(menu);
menu.add(0, 0, 0, "新建目录").setIcon(R.drawable.addfolderr);
menu.add(0, 1, 0, "删除目录").setIcon(R.drawable.delete);
menu.add(0, 2, 0, "粘贴文件").setIcon(R.drawable.paste);
menu.add(0, 3, 0, "根目录").setIcon(R.drawable.goroot);
menu.add(0, 4, 0, "上一级").setIcon(R.drawable.uponelevel);
return true;
}
public boolean onOptionsItemSelected(MenuItem item)
{
super.onOptionsItemSelected(item);
switch (item.getItemId())
{
case 0:
Mynew();
break;
case 1:
//注意:删除目录,谨慎操作,该例子提供了
//deleteFile(删除文件)和deleteFolder(删除整个目录)
MyDelete();
break;
case 2:
MyPaste();
break;
case 3:
this.browseToRoot();
break;
case 4:
this.upOneLevel();
break;
}
return false;
}
@Override
public boolean onPrepareOptionsMenu(Menu menu)
{
return super.onPrepareOptionsMenu(menu);
}
//粘贴操作
public void MyPaste()
{
if ( myTmpFile == null )
{
Builder builder = new Builder(FileManager.this);
builder.setTitle("提示");
builder.setMessage("没有复制或剪切操作");
builder.setPositiveButton(android.R.string.ok,
new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
builder.setCancelable(false);
builder.create();
builder.show();
}
else
{
if ( myTmpOpt == 0 )//复制操作
{
if(new File(GetCurDirectory()+"/"+myTmpFile.getName()).exists())
{
Builder builder = new Builder(FileManager.this);
builder.setTitle("粘贴提示");
builder.setMessage("该目录有相同的文件,是否需要覆盖?");
builder.setPositiveButton(android.R.string.ok,
new AlertDialog.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
copyFile(myTmpFile,new File(GetCurDirec
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
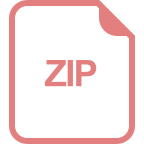
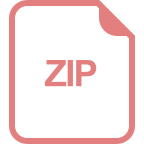
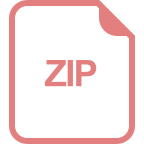
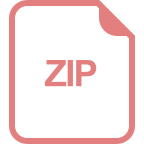
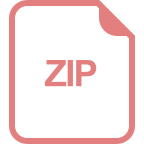
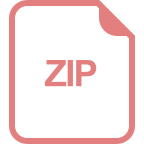
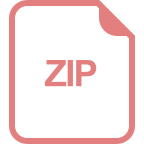
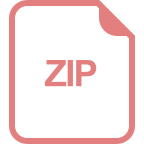
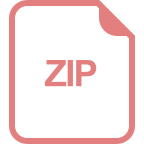
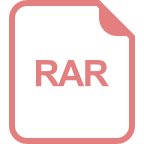
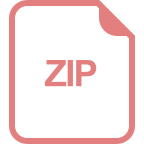
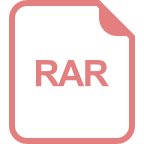
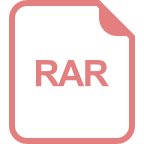
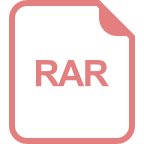
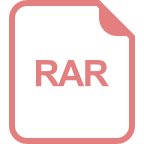
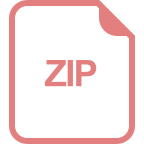
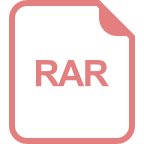
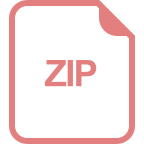
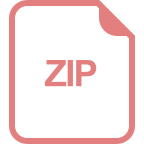
收起资源包目录




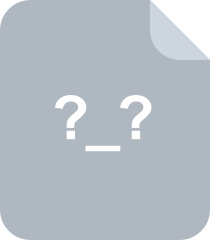


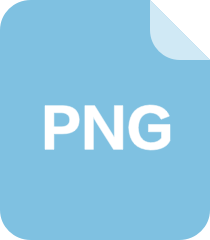
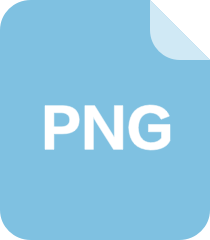
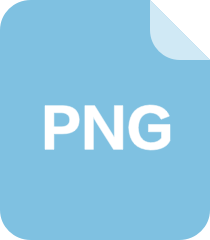
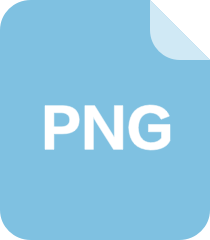
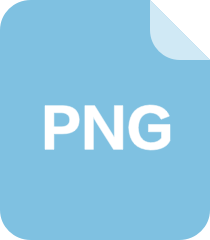
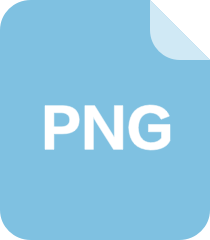
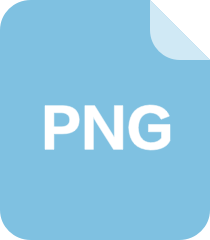
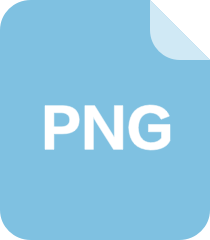
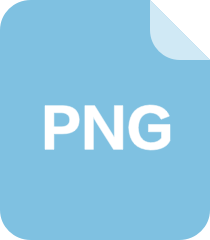
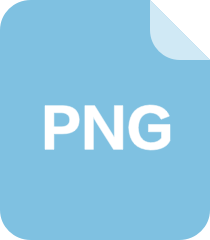
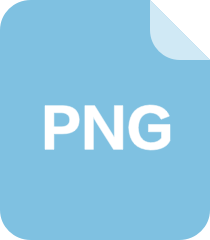
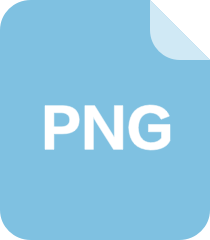
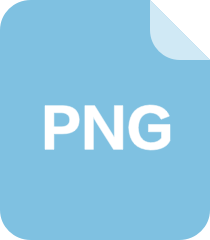
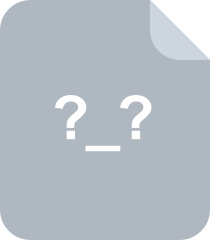





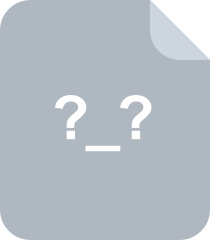
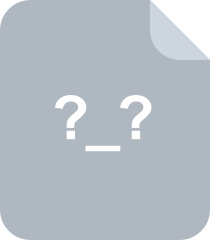
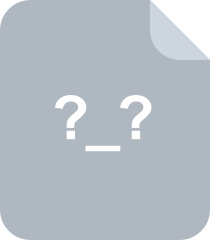
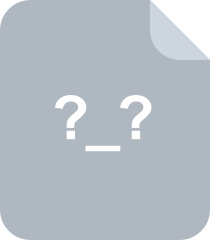
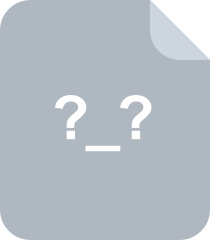
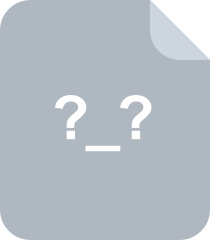
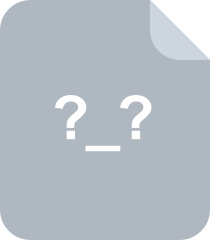
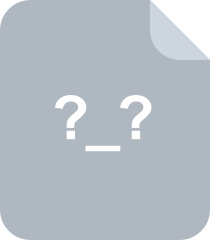
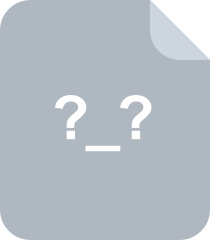
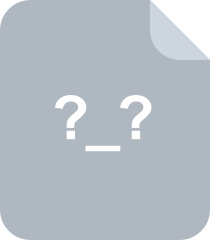
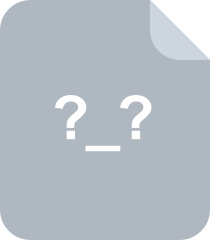
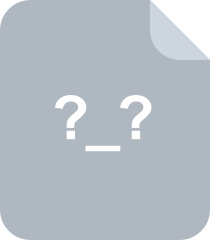
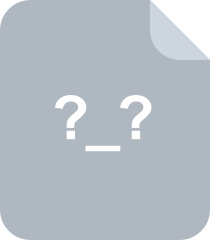
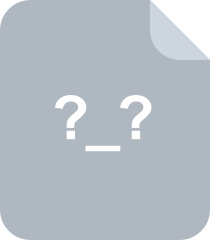
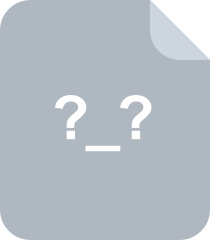
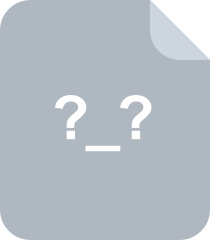
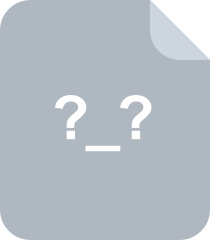
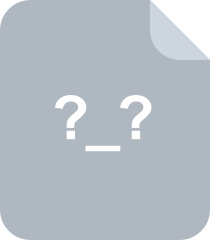
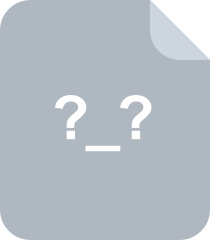
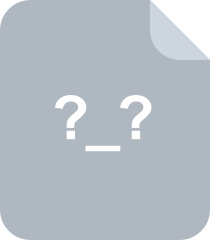
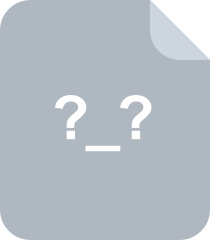
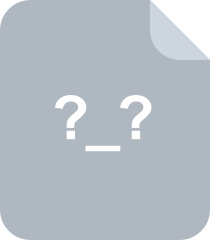
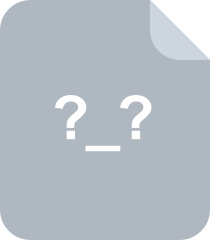
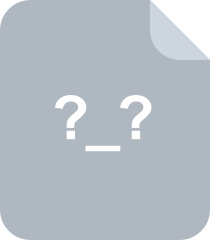
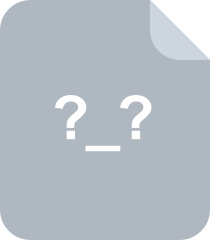
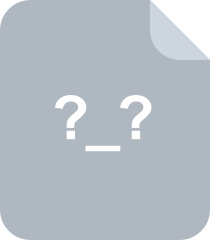
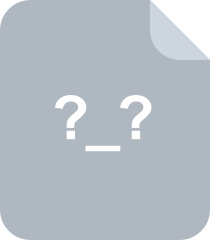
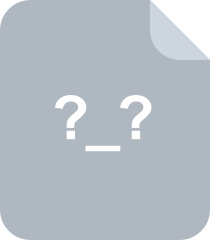
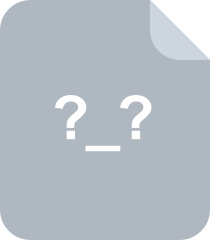
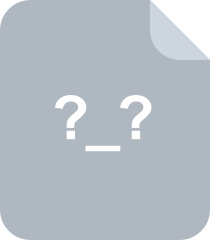
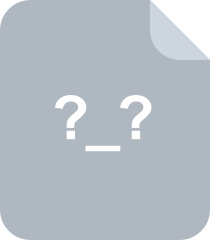
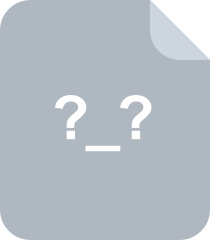
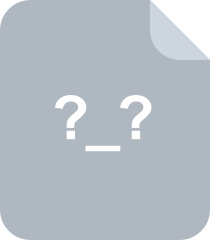
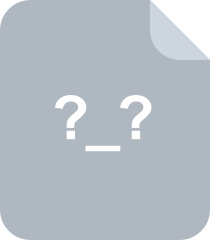
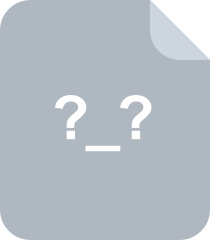
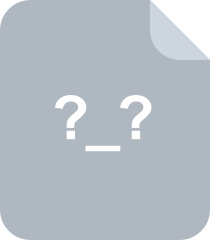





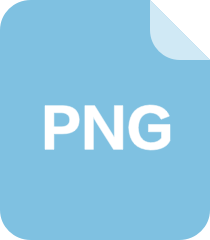
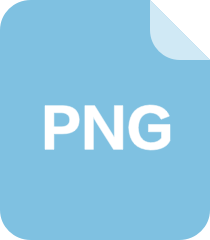
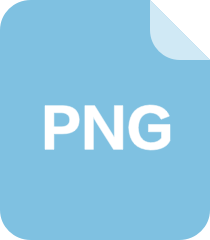
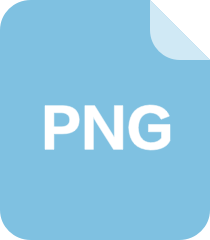
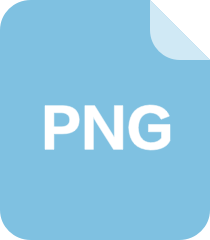
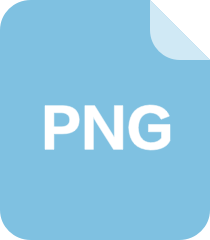
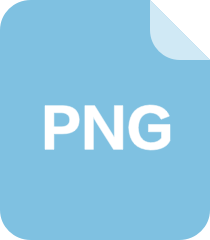
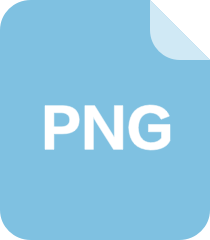
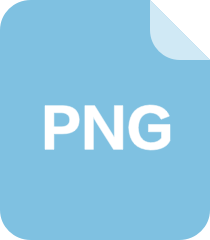
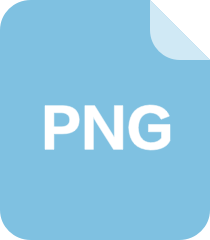
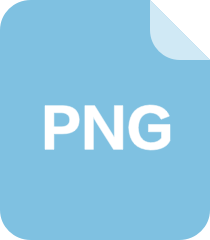
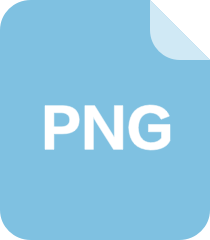
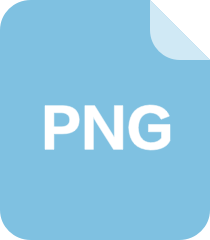

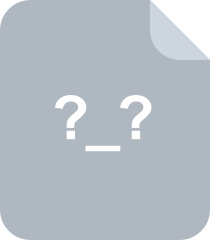
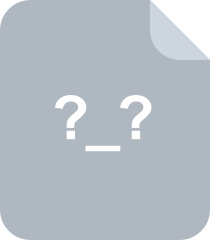


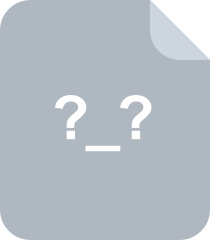
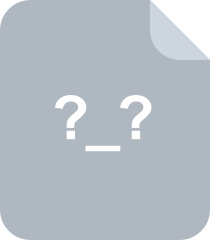






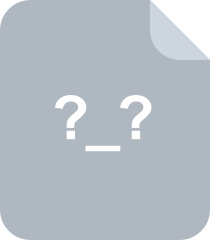
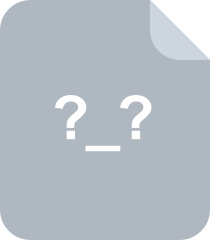

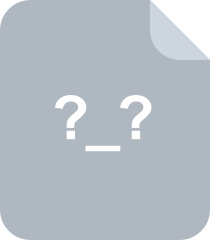





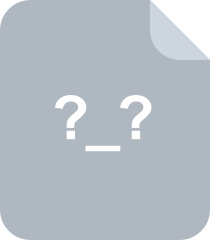
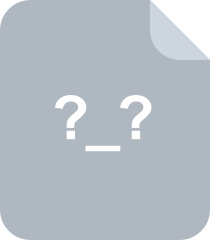
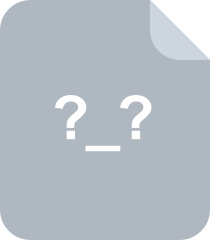
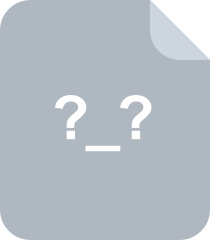
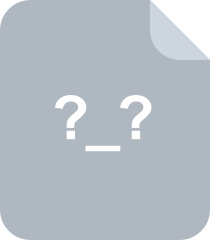
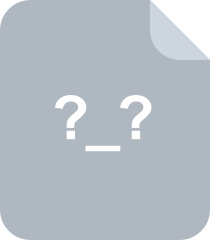
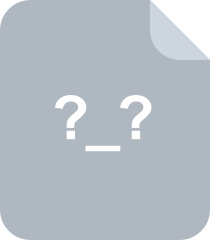
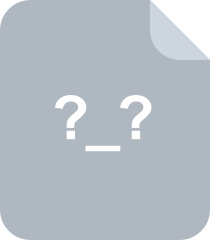
共 79 条
- 1
资源评论
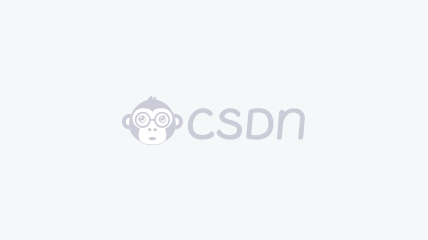

芝麻粒儿
- 粉丝: 6w+
- 资源: 2万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

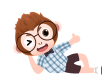
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


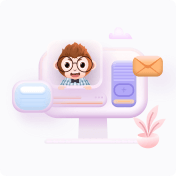
安全验证
文档复制为VIP权益,开通VIP直接复制
