This project is directly clone from
https://github.com/willblaschko/AlexaAndroid
# Alexa Voice Library
=======
*A library and sample app to abstract access to the Amazon Alexa service for Android applications.*
First and foremost, my goal with this project is to help others who have less of an understanding of Java, Android, or both, to be able to quickly and easily integrate the Amazon Alexa platform into their own applications.
For getting started with the Amazon Alexa platform, take a look over here: https://developer.amazon.com/appsandservices/solutions/alexa/alexa-voice-service/getting-started-with-the-alexa-voice-service
Library updated with functionality for the Alexa [v20160207 API version](https://developer.amazon.com/public/solutions/alexa/alexa-voice-service/content/avs-api-overview).
## Applications
### Sample Application
Curious about what the library can do? A quick example of the three main functions (live recorded audio events, text-to-speech intents, prerecorded audio intents), plus sample code can be found in the [sample app](https://play.google.com/store/apps/details?id=com.willblaschko.android.alexavoicelibrary)
#### Compiling and running the sample application
* Follow the process for creating a connected device detailed at the Amazon link at the top of the Readme.
* Add your api_key.txt file (part of the Amazon process) to the app/src/main/assets folder
* Change [PRODUCT_ID](https://github.com/willblaschko/AlexaAndroid/blob/master/app/src/main/java/com/willblaschko/android/alexavoicelibrary/global/Constants.java#L8) to the value for my Alexa application that you configured for "Application Type Id" above.
* Build and run the sample app using Gradle from the command line or Android Studio!
### Production Application
Or see what the library can do when converted into a full package, complete with optional always-on listener: [Alexa Listens](https://play.google.com/store/apps/details?id=com.willblaschko.android.alexalistens)
## Using the Library
Most of the library can be accessed through the [AlexaManager](http://willblaschko.github.io/AlexaAndroid/com/willblaschko/android/alexa/AlexaManager.html) and [AlexaAudioPlayer](http://willblaschko.github.io/AlexaAndroid/com/willblaschko/android/alexa/avs/AlexaAudioPlayer.html) classes, both of which are singletons.
### Installation
* Ensure you're pulling from jcenter() for your project (project-level build.gradle):
```java
buildscript {
repositories {
jcenter()
}
...
}
allprojects {
repositories {
jcenter()
}
}
```
* Add the library to your imports (application-level build.gradle):
```java
compile 'com.willblaschko.android.alexa:AlexaAndroid:2.4.2'
```
* Follow the process for creating a connected device detailed in the Amazon link at the top of the Readme.
* Follow the instructions for adding your key and preparing the Login with Amazon activity from the ['Login with Amazon' Android Project guide](https://developer.amazon.com/public/apis/engage/login-with-amazon/docs/create_android_project.html)
* Add your api_key.txt file (part of the Login with Amazon process detailed in the link above) to the app/src/main/assets folder.
* Start integration and testing!
### Library Instantiation and Basic Return Parsing
```java
private AlexaManager alexaManager;
private AlexaAudioPlayer audioPlayer;
private List<AvsItem> avsQueue = new ArrayList<>();
private void initAlexaAndroid(){
//get our AlexaManager instance for convenience
alexaManager = AlexaManager.getInstance(this, PRODUCT_ID);
//instantiate our audio player
audioPlayer = AlexaAudioPlayer.getInstance(this);
//Callback to be able to remove the current item and check queue once we've finished playing an item
audioPlayer.addCallback(alexaAudioPlayerCallback);
}
//Our callback that deals with removing played items in our media player and then checking to see if more items exist
private AlexaAudioPlayer.Callback alexaAudioPlayerCallback = new AlexaAudioPlayer.Callback() {
@Override
public void playerPrepared(AvsItem pendingItem) {
}
@Override
public void itemComplete(AvsItem completedItem) {
avsQueue.remove(completedItem);
checkQueue();
}
@Override
public boolean playerError(int what, int extra) {
return false;
}
@Override
public void dataError(Exception e) {
}
};
//async callback for commands sent to Alexa Voice
private AsyncCallback<AvsResponse, Exception> requestCallback = new AsyncCallback<AvsResponse, Exception>() {
@Override
public void start() {
//your on start code
}
@Override
public void success(AvsResponse result) {
Log.i(TAG, "Voice Success");
handleResponse(result);
}
@Override
public void failure(Exception error) {
//your on error code
}
@Override
public void complete() {
//your on complete code
}
};
/**
* Handle the response sent back from Alexa's parsing of the Intent, these can be any of the AvsItem types (play, speak, stop, clear, listen)
* @param response a List<AvsItem> returned from the mAlexaManager.sendTextRequest() call in sendVoiceToAlexa()
*/
private void handleResponse(AvsResponse response){
if(response != null){
//if we have a clear queue item in the list, we need to clear the current queue before proceeding
//iterate backwards to avoid changing our array positions and getting all the nasty errors that come
//from doing that
for(int i = response.size() - 1; i >= 0; i--){
if(response.get(i) instanceof AvsReplaceAllItem || response.get(i) instanceof AvsReplaceEnqueuedItem){
//clear our queue
avsQueue.clear();
//remove item
response.remove(i);
}
}
avsQueue.addAll(response);
}
checkQueue();
}
/**
* Check our current queue of items, and if we have more to parse (once we've reached a play or listen callback) then proceed to the
* next item in our list.
*
* We're handling the AvsReplaceAllItem in handleResponse() because it needs to clear everything currently in the queue, before
* the new items are added to the list, it should have no function here.
*/
private void checkQueue() {
//if we're out of things, hang up the phone and move on
if (avsQueue.size() == 0) {
return;
}
AvsItem current = avsQueue.get(0);
if (current instanceof AvsPlayRemoteItem) {
//play a URL
if (!audioPlayer.isPlaying()) {
audioPlayer.playItem((AvsPlayRemoteItem) current);
}
} else if (current instanceof AvsPlayContentItem) {
//play a URL
if (!audioPlayer.isPlaying()) {
audioPlayer.playItem((AvsPlayContentItem) current);
}
} else if (current instanceof AvsSpeakItem) {
//play a sound file
if (!audioPlayer.isPlaying()) {
audioPlayer.playItem((AvsSpeakItem) current);
}
} else if (current instanceof AvsStopItem) {
//stop our play
audioPlayer.stop();
avsQueue.remove(current);
} else if (current instanceof AvsReplaceAllItem) {
audioPlayer.stop();
avsQueue.remove(current);
} else if (current instanceof AvsReplaceEnqueuedItem) {
avsQueue.remove(current);
} else if (current instanceof AvsExpectSpeechItem) {
//listen for user input
audioPlayer.stop();
startListening();
} else if (current instanceof AvsSetVolumeItem) {
setVolume(((AvsSetVolumeItem) current).getVolume());
avsQueue.remove(current);
} else if(current instanceof AvsAdjustVolumeItem){
adjustVolume(((AvsAdjustVolumeItem) current).getAdjustment());
avsQueue.remove(current);
} else if(current instanceof AvsSetMuteItem){
setMute(((AvsSetMuteItem) current).isMute());
avsQueue.remove(current);
}else if(current instanceof AvsMediaPlayCommandItem){
//fake a hardware "play" press
sendMediaButton(this, KeyEvent.KEYCODE_MEDIA_PLAY);
}else if(current instanceof AvsMediaPauseCommandItem){
//fake a hardware "pause" press
sendMediaButton(this, KeyEvent.KEYCODE_MEDIA_P
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
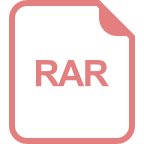
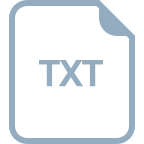
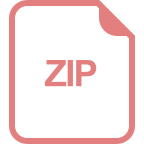
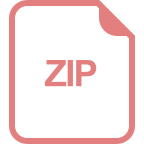
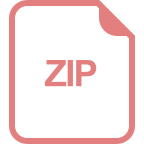
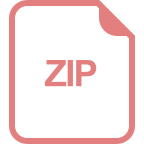
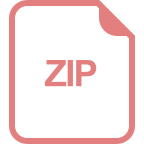
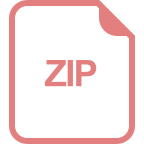
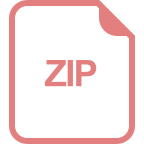
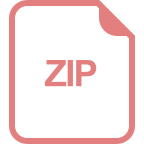
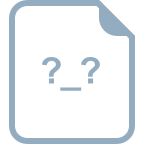
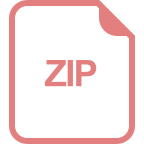
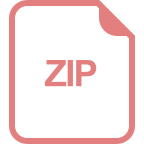
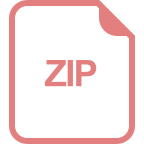
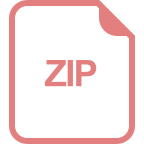
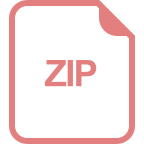
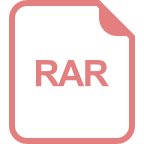
收起资源包目录

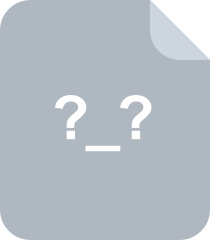
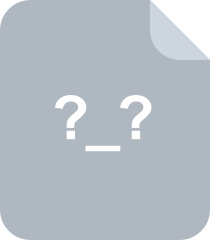
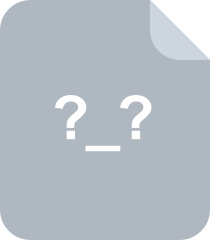
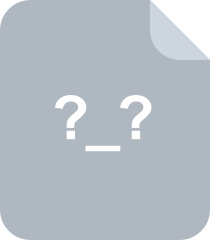
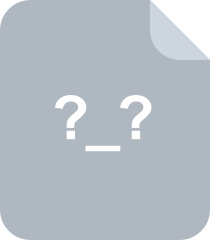
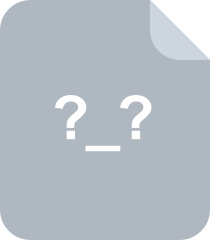
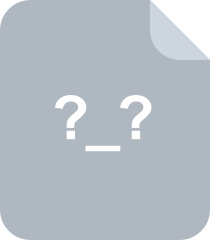
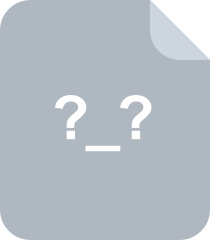
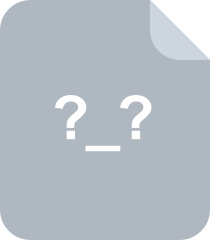
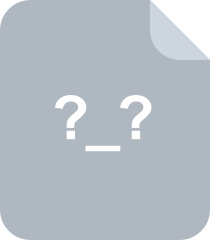
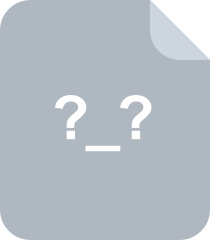
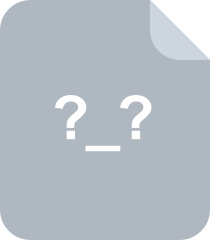
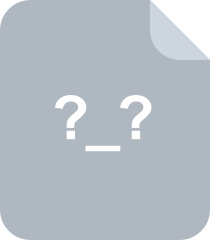
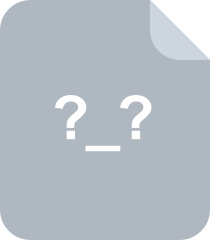
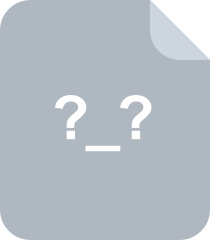
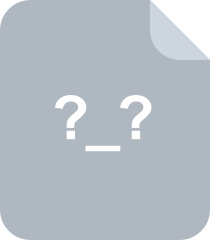
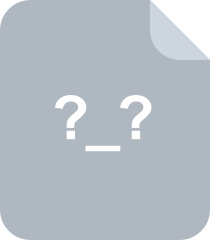
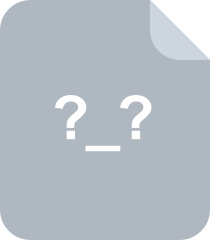
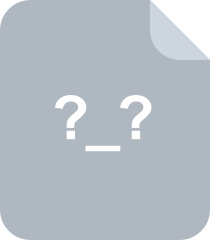
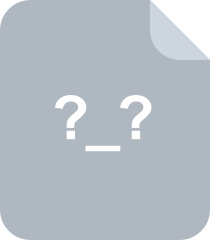
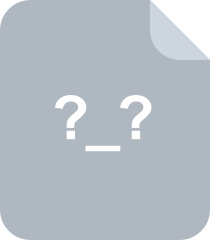
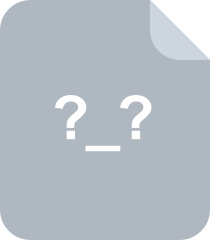
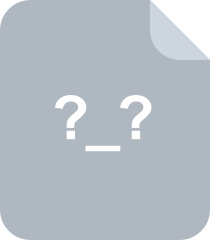
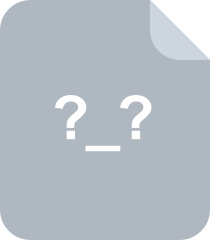
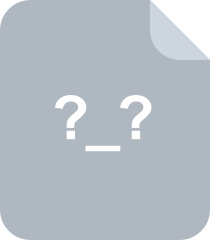
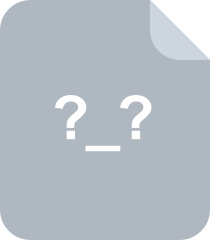
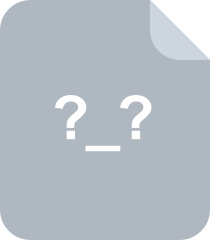
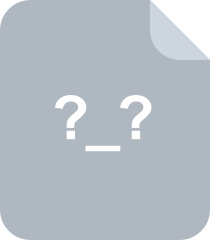
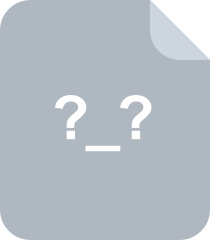
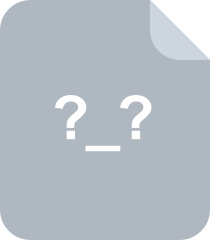
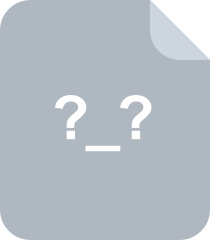
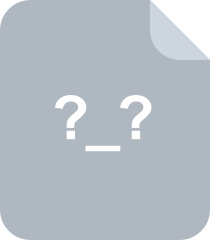
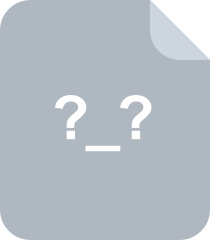
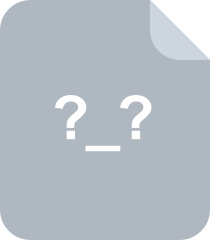
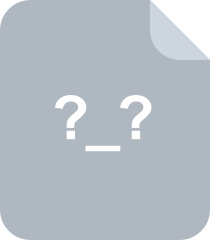
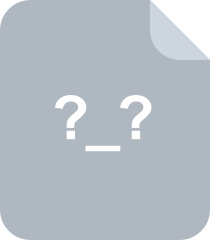
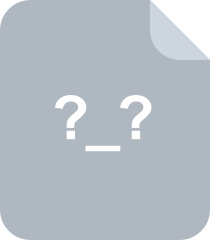
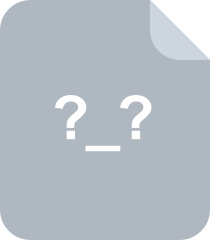
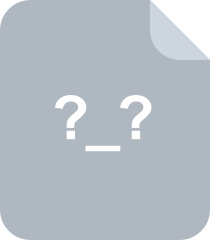
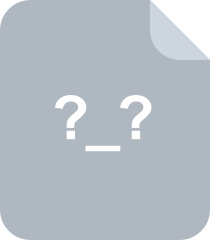
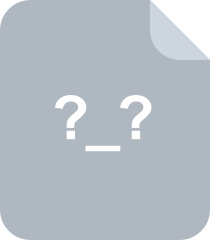
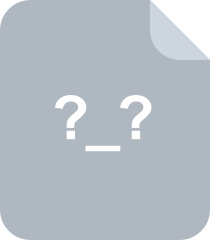
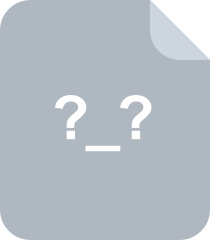
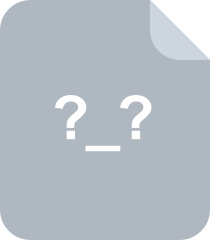
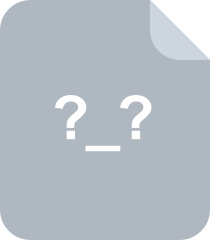
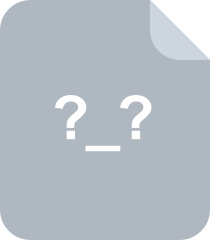
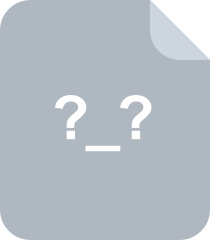
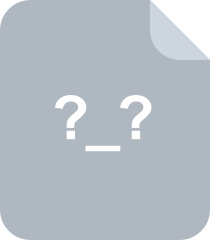
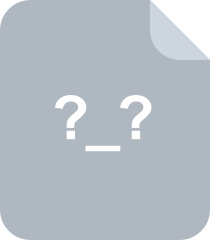
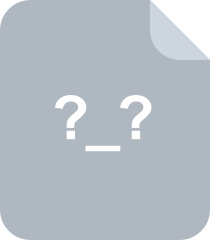
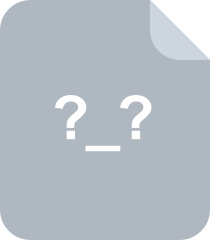
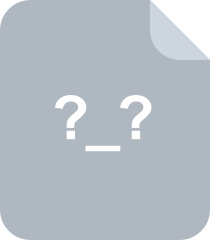
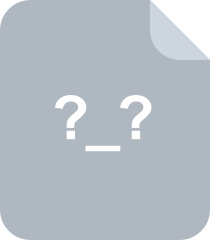
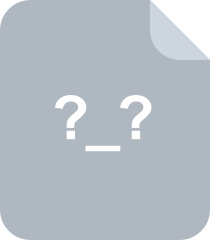
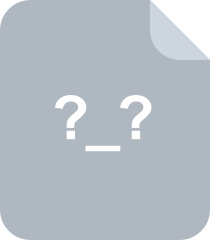
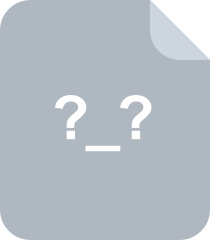
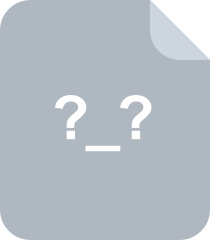
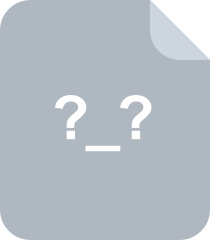
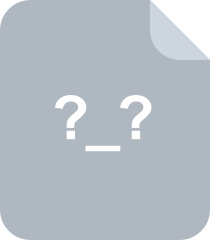
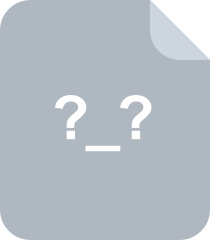
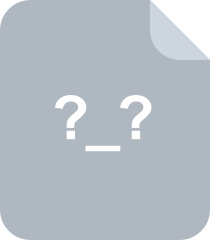
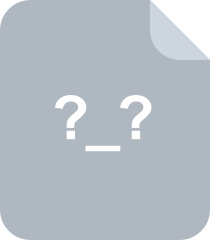
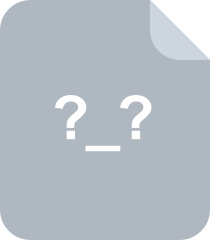
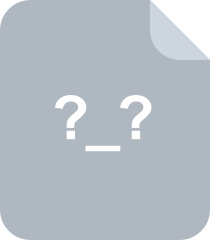
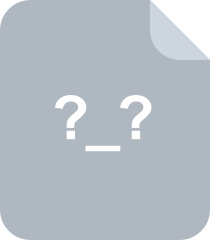
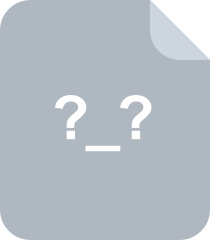
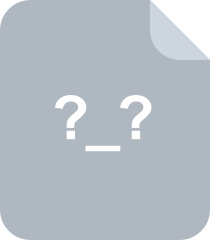
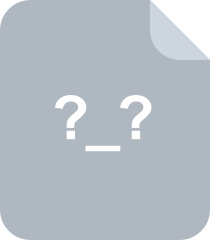
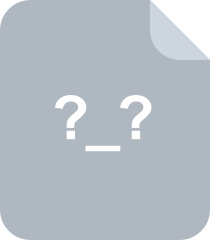
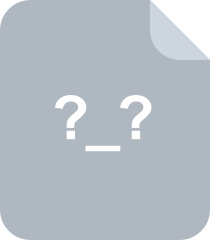
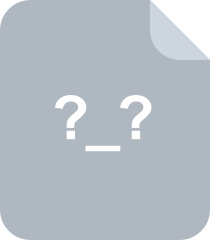
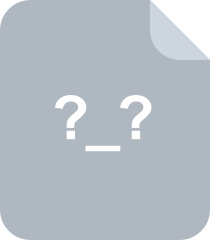
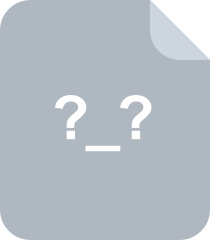
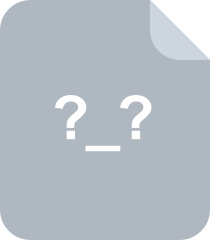
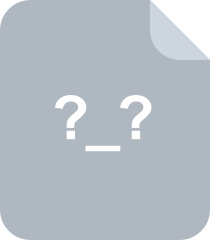
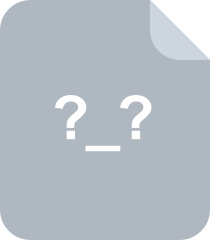
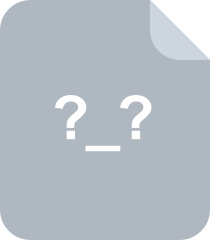
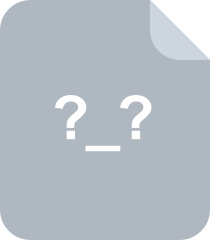
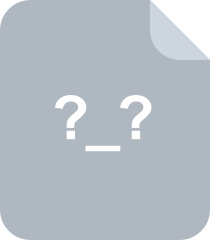
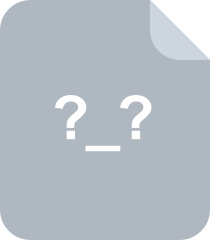
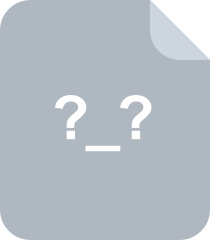
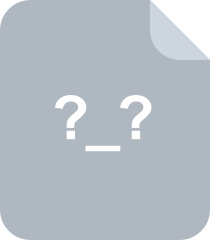
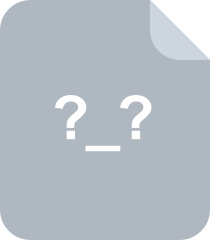
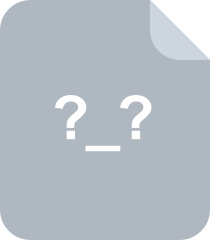
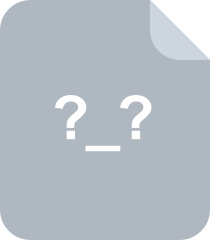
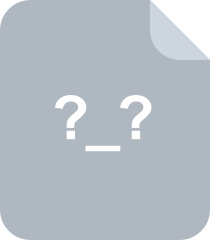
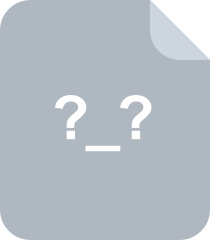
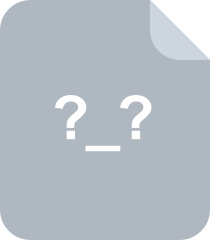
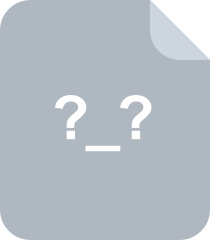
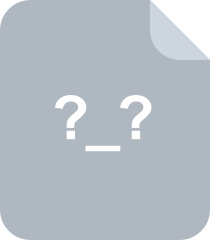
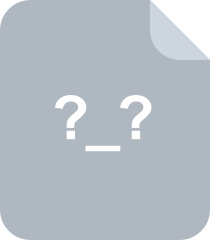
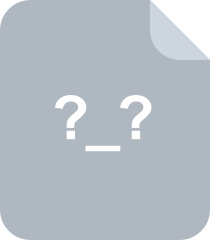
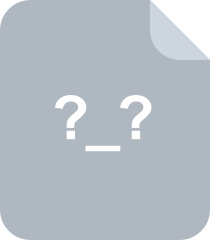
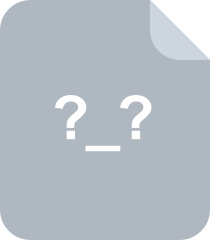
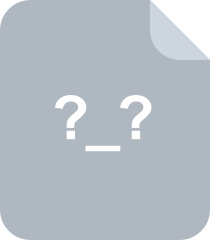
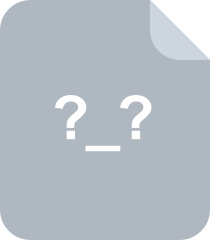
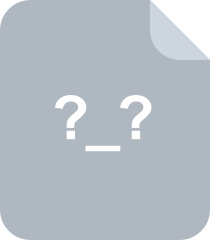
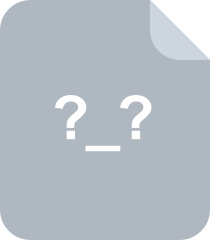
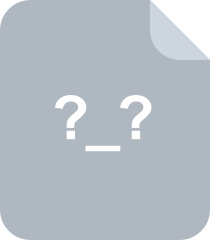
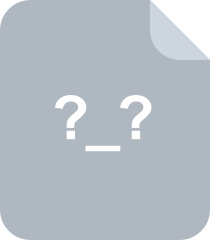
共 6098 条
- 1
- 2
- 3
- 4
- 5
- 6
- 61
资源评论
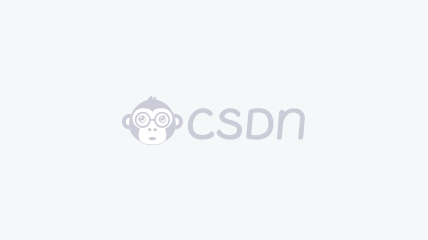

放大的EZ
- 粉丝: 889
- 资源: 59
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

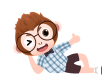
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


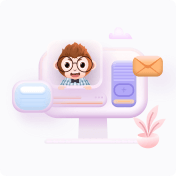
安全验证
文档复制为VIP权益,开通VIP直接复制
