package com.jxd.bank.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Scanner;
import util.DBUtil;
import com.jxd.bank.entity.BankAccount;
import com.jxd.bank.entity.Record;
public class BankAccountDaoImpl implements BankAccountDao {
private static Scanner input = new Scanner(System.in);
private static List<Map<BankAccount,Record>> list = new ArrayList<Map<BankAccount,Record>>();
private static BankAccount bac = new BankAccount();
private static Record r = new Record();
private static Map<BankAccount,Record> map = new HashMap<BankAccount, Record>();
private static Connection conn = null;
private static PreparedStatement pst = null;
private static ResultSet rs = null;
/**
* 开户
*/
public BankAccount openAccount() {
System.out.println("欢迎您开办新账户!请根据系统提示输入!\n");
System.out.println("请输入姓名:");
String name = input.next();
System.out.println("请输入密码:");
String pwd = input.next();
System.out.println("请输入初始余额");
double balance = input.nextInt();
System.out.println("请稍后,正在开户中……");
System.out.println("---------------------------");
try {
conn = DBUtil.getConnection();
String sql = "insert into bankaccount(cardid,customername,password,oldpassword,balance,opendate) "
+ "values(seq_bankaccount.nextval,?,?,?,?,sysdate)";
pst = conn.prepareStatement(sql);
pst.setString(1, name);
pst.setString(2, pwd);
pst.setString(3, pwd);
pst.setDouble(4, balance);
pst.executeUpdate();
String sql1 = "select * from bankaccount where customername = ? and password = ? order by cardid desc";
pst = conn.prepareStatement(sql1);
pst.setString(1, name);
pst.setString(2, pwd);
rs = pst.executeQuery();
if (rs.next()) {
String sql2 = "insert into record(id,cardid,transtype,transmoney,transdate) "
+ "values(seq_record.nextval,?,'1',?,sysdate)";
pst = conn.prepareStatement(sql2);
pst.setInt(1, rs.getInt("cardid"));
pst.setDouble(2, rs.getDouble("balance"));
pst.executeUpdate();
r.setCardid(rs.getInt("cardid"));
r.setTranstype("1");
r.setDate(rs.getString("opendate"));
r.setTransmoney(rs.getDouble("balance"));
bac.setCardid(rs.getInt("cardid"));
bac.setCustomername(name);
bac.setPassword(pwd);
bac.setOldpassword(pwd);
bac.setBalance(balance);
bac.setDate(rs.getDate("opendate") + " " + rs.getTime("opendate"));
map.put(bac, r);
list.add(map);
} else {
System.out.println("密码长度过长,开户失败");
}
System.out.println("---------------------------");
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pst, rs);
}
return bac;
}
/**
* 取款
*/
public List<Record> takeMoney() {
System.out.println("欢迎来办理取款业务!请根据提示输入!");
System.out.println("请输入账号:");
int cardid = input.nextInt();
System.out.println("请输入密码,有3次输入机会");
try {
conn = DBUtil.getConnection();
for (int i = 0; i < 3; i++) {
String pwd = input.next();
String sql = "select * from bankaccount where cardid = ? and password = ?";
pst = conn.prepareStatement(sql);
pst.setInt(1, cardid);
pst.setString(2, pwd);
rs = pst.executeQuery();
if (rs.next()) {
System.out.println("请输入取款金额:");
double balance = input.nextDouble();
if (balance <= rs.getDouble("balance")) {
System.out.println("请稍后,正在取款中……");
System.out
.println("---------------------------------------");
String sql1 = "update bankaccount set balance = balance - ? where cardid = ?";
pst = conn.prepareStatement(sql1);
pst.setDouble(1, balance);
pst.setInt(2, cardid);
pst.executeUpdate();
pst = conn.prepareStatement(sql);
pst.setInt(1, cardid);
pst.setString(2, pwd);
rs = pst.executeQuery();
if (rs.next()) {
System.out.println("恭喜你取款成功!");
System.out.println("您的余额是:"
+ rs.getDouble("balance"));
}
String sql2 = "insert into record(id,cardid,transtype,transmoney,transdate) "
+ "values(seq_record.nextval,?,'3',?,sysdate)";
pst = conn.prepareStatement(sql2);
pst.setInt(1, cardid);
pst.setDouble(2, balance);
pst.executeUpdate();
String sql3 = "select * from record where cardid = ? and transmoney = ? and transtype = '3' "
+ "order by id desc";
pst = conn.prepareStatement(sql3);
pst.setInt(1, cardid);
pst.setDouble(2, balance);
rs = pst.executeQuery();
if (rs.next()) {
System.out.println("您的存款日期:"
+ rs.getString("transdate"));
r.setCardid(rs.getInt("cardid"));
r.setTranstype("3");
r.setDate(rs.getString("transdate"));
r.setTransmoney(rs.getDouble("transmoney"));
list.add(r);
}
break;
} else {
System.out.println("余额不足!!");
}
} else {
System.out.println("密码错误,请重新输入,还有" + (2 - i) + "次机会");
}
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pst, rs);
}
return list;
}
/**
* 存款
*/
public List<Record> saveMoney() {
System.out.println("欢迎您来办理存款业务!请根据提示输入!");
System.out.println("请输入账号:");
int cardid = input.nextInt();
System.out.println("请输入存款的金额:");
double balance = input.nextDouble();
System.out.println("请稍后,正在存款中……");
System.out.println("---------------------------------");
try {
conn = DBUtil.getConnection();
String sql = "select * from bankaccount where cardid = ?";
pst = conn.prepareStatement(sql);
pst.setInt(1, cardid);
rs = pst.executeQuery();
if (rs.next()) {
String sql1 = "update bankaccount set balance = balance + ? where cardid = ?";
pst = conn.prepareStatement(sql1);
pst.setDouble(1, balance);
pst.setInt(2, cardid);
pst.executeUpdate();
pst = conn.prepareStatement(sql);
pst.setInt(1, cardid);
rs = pst.executeQuery();
if (rs.next()) {
System.out.println("恭喜你存款成功!");
System.out.println("您的存款金额是:" + balance);
System.out.println("您的余额是:" + rs.getDouble("balance"));
String sql2 = "insert into record(id,cardid,transtype,transmoney,transdate) "
+ "values(seq_record.nextval,?,'2',?,sysdate)";
pst = conn.prepareStatement(sql2);
pst.setInt(1, cardid);
pst.setDouble(2, balance);
pst.executeUpdate();
String sql3 = "select * from record where cardid = ? and transmoney = ? and transtype = '2' "
+ "order by id desc";
pst = conn.prepareStatement(sql3);
pst.setInt(1, cardid);
pst.setDouble(2, balance);
rs = pst.executeQuery();
if (rs.next()) {
System.out.println("您的存款日期:"
+ rs.getString("transdate"));
r.setCardid(rs.getInt("cardid"));
r.setTranstype("2");
r.setDate(rs.getString("transdate"));
r.setTransmoney(rs.getDouble("transmoney"));
list.add(r);
}
}
} else {
System.out.println("卡号不存在");
}
System.out.println("---------------------------------");
} catch (SQLException e) {
e.printStackTrace();
} finally {
DBUtil.closeAll(conn, pst, rs);
}
return list;
}
/**
* 查询
*/
public List<Record> queryMoney() {
String type = null;
System.out.println("欢迎您来查看账户信息!请根据提示输入!");
System.out.println("请输入账号:");
int cardid = input.nextInt();
System.out.println("请输入�

qq_26826443
- 粉丝: 0
- 资源: 1
最新资源
- springboot043基于springboot的“衣依”服装销售平台的设计与实现.zip
- springboot243基于SpringBoot的小学生身体素质测评管理系统设计与实现.zip
- 多类型电动汽车 负荷预测 蒙特卡洛 SOC 基于蒙特卡洛的多种类型电动汽车负荷预测 软件:Matlab 介绍:基于蒙特卡洛模拟(MCS)抽样,四种充电汽车类型同时模拟,根据私家车、公交车、出租车、公务
- springboot045新闻推荐系统.zip
- springboot044美容院管理系统.zip
- springboot244基于SpringBoot和VUE技术的智慧生活商城系统设计与实现.zip
- springboot245科研项目验收管理系统.zip
- springboot246老年一站式服务平台.zip
- springboot046古典舞在线交流平台的设计与实现.zip
- T113S3增加串口4(Uart4)-Tina环境-board.dts文件比较
- MPU6050六轴传感器位移测算
- springboot048校园资料分享平台.zip
- springboot047大学生就业招聘系统的设计与实现.zip
- haohuan_release.apk
- springboot247人事管理系统.zip
- springboot248校园资产管理.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


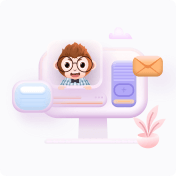