sshpk
=========
Parse, convert, fingerprint and use SSH keys (both public and private) in pure
node -- no `ssh-keygen` or other external dependencies.
Supports RSA, DSA, ECDSA (nistp-\*) and ED25519 key types, in PEM (PKCS#1,
PKCS#8) and OpenSSH formats.
This library has been extracted from
[`node-http-signature`](https://github.com/joyent/node-http-signature)
(work by [Mark Cavage](https://github.com/mcavage) and
[Dave Eddy](https://github.com/bahamas10)) and
[`node-ssh-fingerprint`](https://github.com/bahamas10/node-ssh-fingerprint)
(work by Dave Eddy), with additions (including ECDSA support) by
[Alex Wilson](https://github.com/arekinath).
Install
-------
```
npm install sshpk
```
Examples
--------
```js
var sshpk = require('sshpk');
var fs = require('fs');
/* Read in an OpenSSH-format public key */
var keyPub = fs.readFileSync('id_rsa.pub');
var key = sshpk.parseKey(keyPub, 'ssh');
/* Get metadata about the key */
console.log('type => %s', key.type);
console.log('size => %d bits', key.size);
console.log('comment => %s', key.comment);
/* Compute key fingerprints, in new OpenSSH (>6.7) format, and old MD5 */
console.log('fingerprint => %s', key.fingerprint().toString());
console.log('old-style fingerprint => %s', key.fingerprint('md5').toString());
```
Example output:
```
type => rsa
size => 2048 bits
comment => foo@foo.com
fingerprint => SHA256:PYC9kPVC6J873CSIbfp0LwYeczP/W4ffObNCuDJ1u5w
old-style fingerprint => a0:c8:ad:6c:32:9a:32:fa:59:cc:a9:8c:0a:0d:6e:bd
```
More examples: converting between formats:
```js
/* Read in a PEM public key */
var keyPem = fs.readFileSync('id_rsa.pem');
var key = sshpk.parseKey(keyPem, 'pem');
/* Convert to PEM PKCS#8 public key format */
var pemBuf = key.toBuffer('pkcs8');
/* Convert to SSH public key format (and return as a string) */
var sshKey = key.toString('ssh');
```
Signing and verifying:
```js
/* Read in an OpenSSH/PEM *private* key */
var keyPriv = fs.readFileSync('id_ecdsa');
var key = sshpk.parsePrivateKey(keyPriv, 'pem');
var data = 'some data';
/* Sign some data with the key */
var s = key.createSign('sha1');
s.update(data);
var signature = s.sign();
/* Now load the public key (could also use just key.toPublic()) */
var keyPub = fs.readFileSync('id_ecdsa.pub');
key = sshpk.parseKey(keyPub, 'ssh');
/* Make a crypto.Verifier with this key */
var v = key.createVerify('sha1');
v.update(data);
var valid = v.verify(signature);
/* => true! */
```
Matching fingerprints with keys:
```js
var fp = sshpk.parseFingerprint('SHA256:PYC9kPVC6J873CSIbfp0LwYeczP/W4ffObNCuDJ1u5w');
var keys = [sshpk.parseKey(...), sshpk.parseKey(...), ...];
keys.forEach(function (key) {
if (fp.matches(key))
console.log('found it!');
});
```
Usage
-----
## Public keys
### `parseKey(data[, format = 'auto'[, options]])`
Parses a key from a given data format and returns a new `Key` object.
Parameters
- `data` -- Either a Buffer or String, containing the key
- `format` -- String name of format to use, valid options are:
- `auto`: choose automatically from all below
- `pem`: supports both PKCS#1 and PKCS#8
- `ssh`: standard OpenSSH format,
- `pkcs1`, `pkcs8`: variants of `pem`
- `rfc4253`: raw OpenSSH wire format
- `openssh`: new post-OpenSSH 6.5 internal format, produced by
`ssh-keygen -o`
- `dnssec`: `.key` file format output by `dnssec-keygen` etc
- `putty`: the PuTTY `.ppk` file format (supports truncated variant without
all the lines from `Private-Lines:` onwards)
- `options` -- Optional Object, extra options, with keys:
- `filename` -- Optional String, name for the key being parsed
(eg. the filename that was opened). Used to generate
Error messages
- `passphrase` -- Optional String, encryption passphrase used to decrypt an
encrypted PEM file
### `Key.isKey(obj)`
Returns `true` if the given object is a valid `Key` object created by a version
of `sshpk` compatible with this one.
Parameters
- `obj` -- Object to identify
### `Key#type`
String, the type of key. Valid options are `rsa`, `dsa`, `ecdsa`.
### `Key#size`
Integer, "size" of the key in bits. For RSA/DSA this is the size of the modulus;
for ECDSA this is the bit size of the curve in use.
### `Key#comment`
Optional string, a key comment used by some formats (eg the `ssh` format).
### `Key#curve`
Only present if `this.type === 'ecdsa'`, string containing the name of the
named curve used with this key. Possible values include `nistp256`, `nistp384`
and `nistp521`.
### `Key#toBuffer([format = 'ssh'])`
Convert the key into a given data format and return the serialized key as
a Buffer.
Parameters
- `format` -- String name of format to use, for valid options see `parseKey()`
### `Key#toString([format = 'ssh])`
Same as `this.toBuffer(format).toString()`.
### `Key#fingerprint([algorithm = 'sha256'[, hashType = 'ssh']])`
Creates a new `Fingerprint` object representing this Key's fingerprint.
Parameters
- `algorithm` -- String name of hash algorithm to use, valid options are `md5`,
`sha1`, `sha256`, `sha384`, `sha512`
- `hashType` -- String name of fingerprint hash type to use, valid options are
`ssh` (the type of fingerprint used by OpenSSH, e.g. in
`ssh-keygen`), `spki` (used by HPKP, some OpenSSL applications)
### `Key#createVerify([hashAlgorithm])`
Creates a `crypto.Verifier` specialized to use this Key (and the correct public
key algorithm to match it). The returned Verifier has the same API as a regular
one, except that the `verify()` function takes only the target signature as an
argument.
Parameters
- `hashAlgorithm` -- optional String name of hash algorithm to use, any
supported by OpenSSL are valid, usually including
`sha1`, `sha256`.
`v.verify(signature[, format])` Parameters
- `signature` -- either a Signature object, or a Buffer or String
- `format` -- optional String, name of format to interpret given String with.
Not valid if `signature` is a Signature or Buffer.
### `Key#createDiffieHellman()`
### `Key#createDH()`
Creates a Diffie-Hellman key exchange object initialized with this key and all
necessary parameters. This has the same API as a `crypto.DiffieHellman`
instance, except that functions take `Key` and `PrivateKey` objects as
arguments, and return them where indicated for.
This is only valid for keys belonging to a cryptosystem that supports DHE
or a close analogue (i.e. `dsa`, `ecdsa` and `curve25519` keys). An attempt
to call this function on other keys will yield an `Error`.
## Private keys
### `parsePrivateKey(data[, format = 'auto'[, options]])`
Parses a private key from a given data format and returns a new
`PrivateKey` object.
Parameters
- `data` -- Either a Buffer or String, containing the key
- `format` -- String name of format to use, valid options are:
- `auto`: choose automatically from all below
- `pem`: supports both PKCS#1 and PKCS#8
- `ssh`, `openssh`: new post-OpenSSH 6.5 internal format, produced by
`ssh-keygen -o`
- `pkcs1`, `pkcs8`: variants of `pem`
- `rfc4253`: raw OpenSSH wire format
- `dnssec`: `.private` format output by `dnssec-keygen` etc.
- `options` -- Optional Object, extra options, with keys:
- `filename` -- Optional String, name for the key being parsed
(eg. the filename that was opened). Used to generate
Error messages
- `passphrase` -- Optional String, encryption passphrase used to decrypt an
encrypted PEM file
### `generatePrivateKey(type[, options])`
Generates a new private key of a certain key type, from random data.
Parameters
- `type` -- String, type of key to generate. Currently supported are `'ecdsa'`
and `'ed25519'`
- `options` -- optional Object, with keys:
- `curve` -- optional String, for `'ecdsa'` keys, specifies the curve to use.
没有合适的资源?快使用搜索试试~ 我知道了~
112-加油站系统.zip
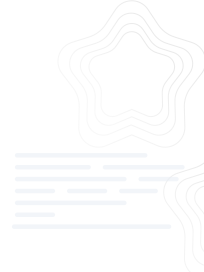
共258个文件
xml:122个
json:33个
md:30个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 17 浏览量
2024-11-26
08:58:19
上传
评论
收藏 55.23MB ZIP 举报
温馨提示
重点:所有项目都有sql文件,比其他博主项目要严谨一万倍所有项目本人亲自测试可运行使用!!有任何问题私我解决! 1、资源项目源码均已通过严格测试验证,保证能够正常运行; 2、项目问题、技术讨论,可以给博主私信或留言,博主看到后会第一时间与您进行沟通; 3、本项目比较适合计算机领域相关的毕业设计课题、课程作业等使用,尤其对于人工智能、计算机科学与技术等相关专业,更为适合; 求注关 所有9.9的项目,企业项目都有SQL文件,购买后运行部署可联系包你满意。不容易啊,谢谢大家支持!!
资源推荐
资源详情
资源评论
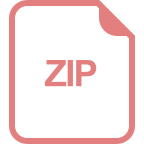
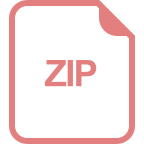
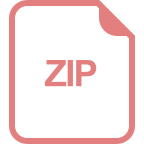
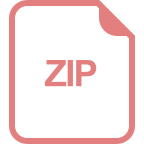
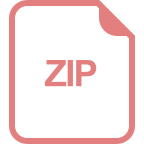
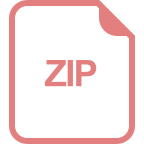
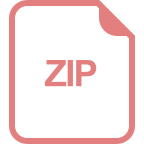
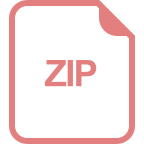
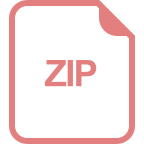
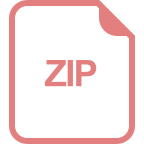
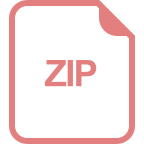
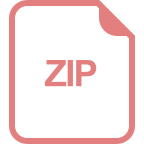
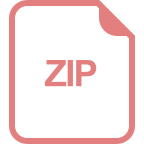
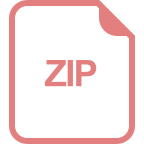
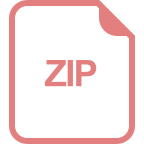
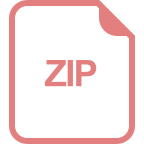
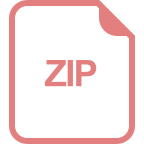
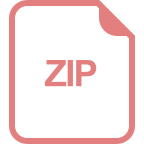
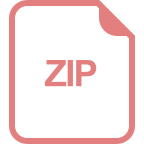
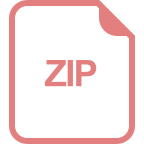
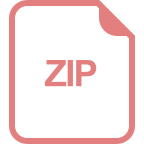
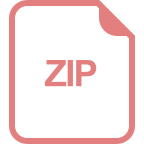
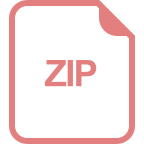
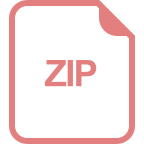
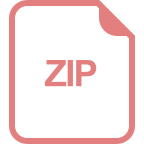
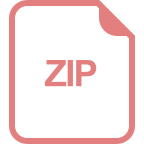
收起资源包目录

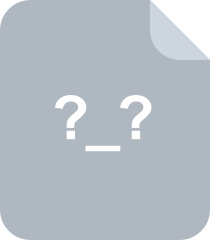
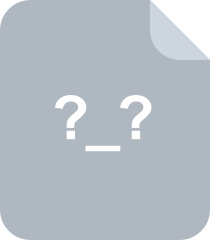
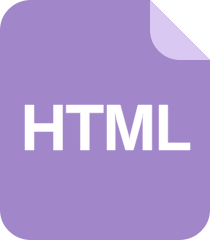
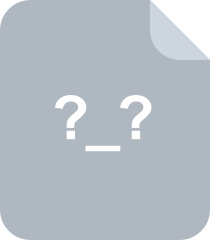
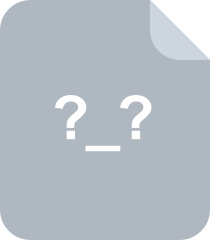
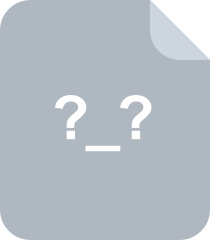
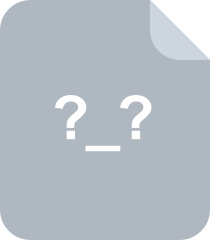
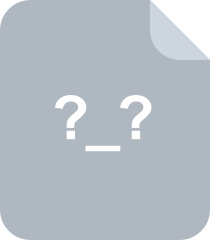
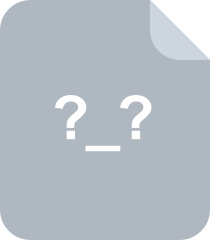
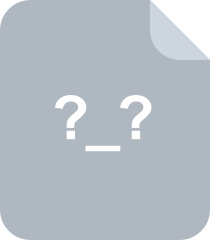
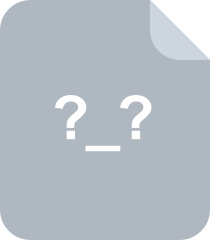
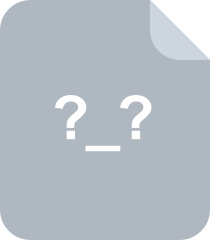
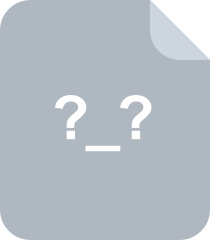
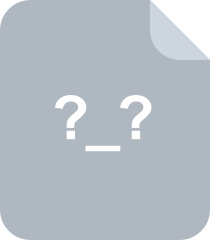
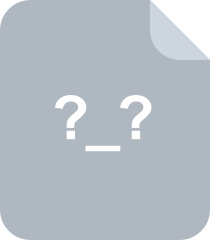
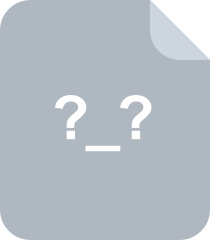
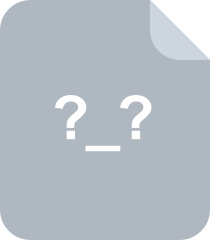
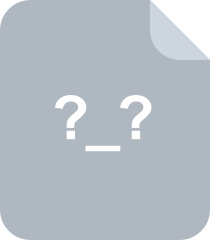
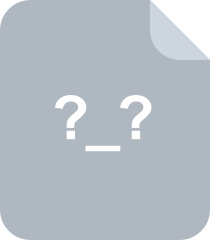
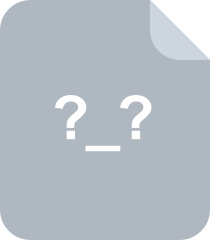
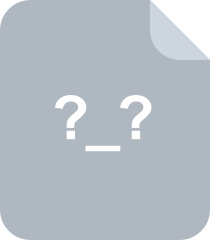
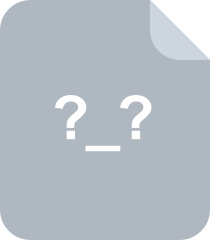
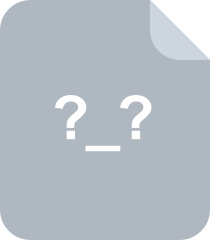
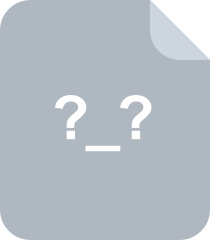
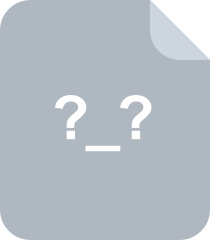
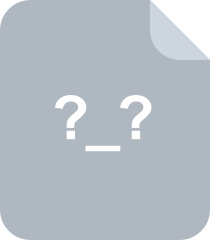
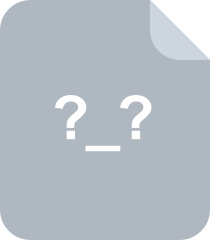
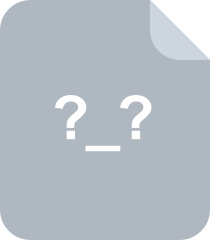
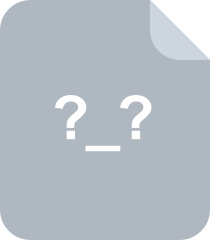
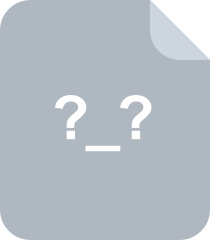
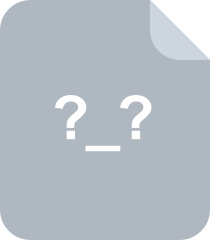
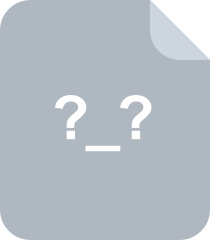
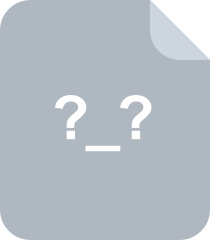
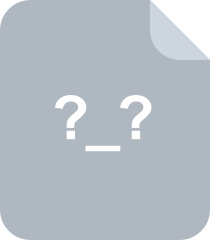
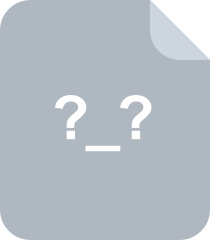
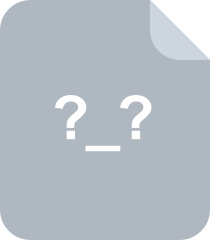
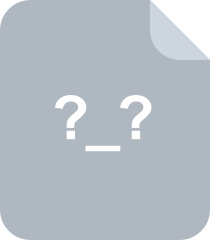
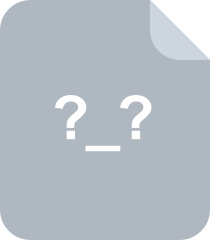
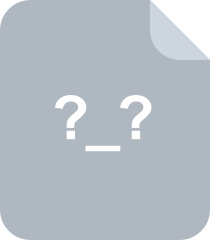
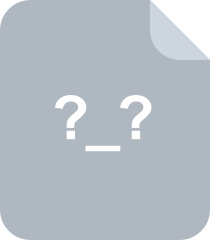
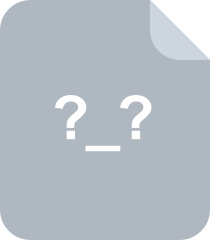
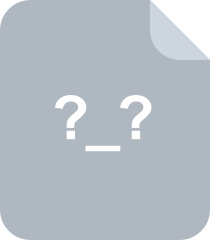
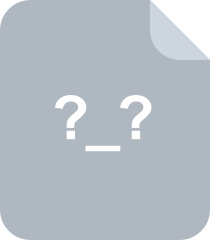
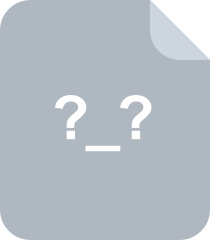
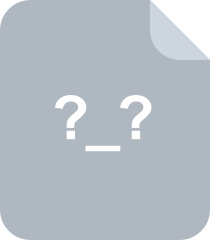
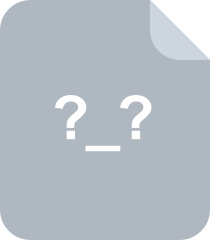
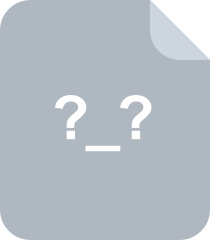
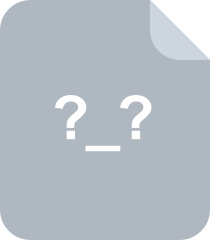
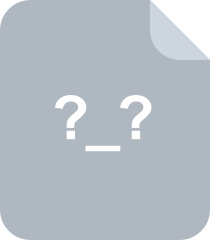
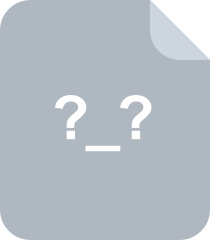
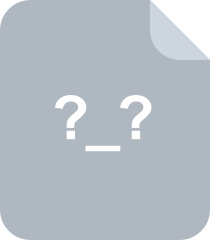
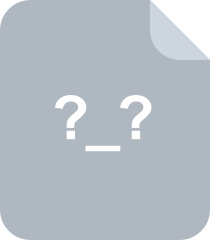
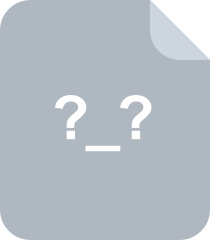
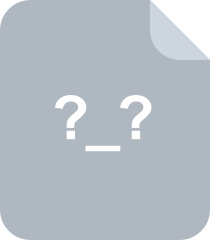
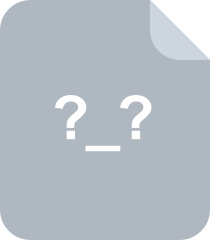
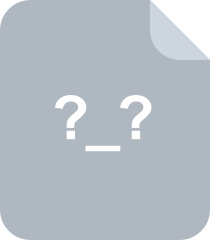
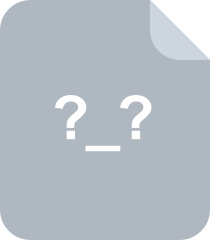
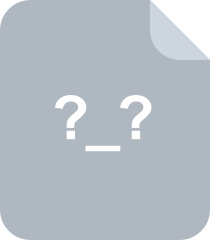
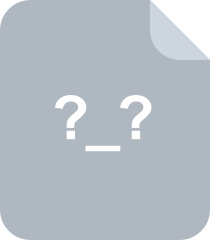
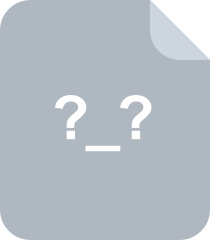
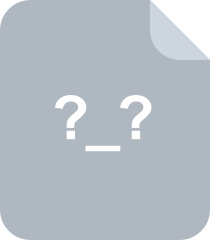
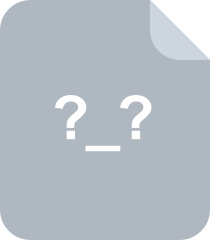
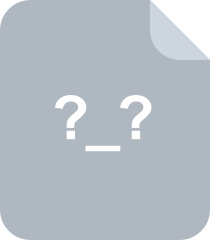
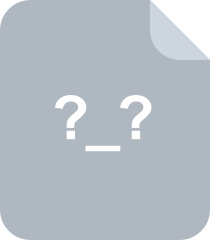
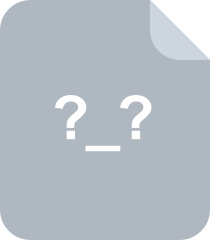
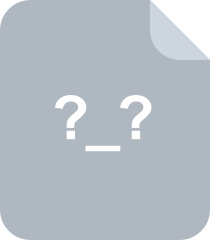
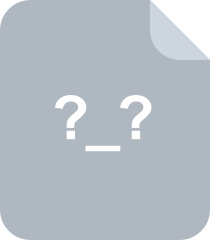
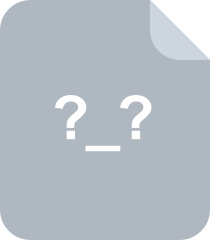
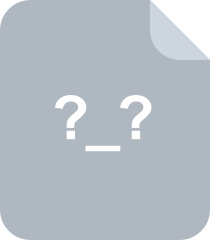
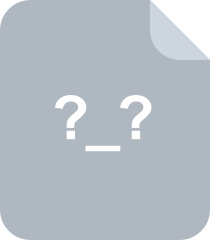
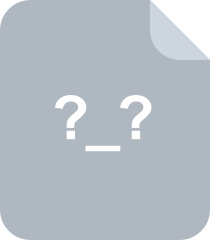
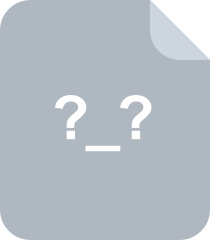
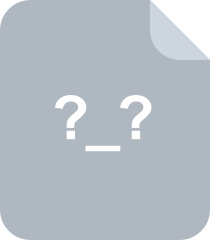
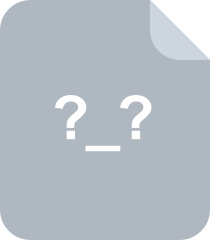
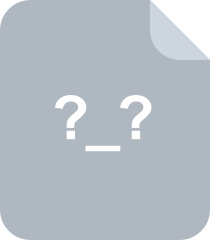
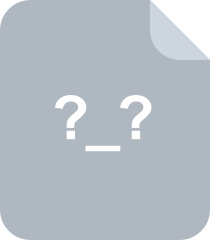
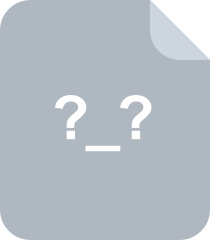
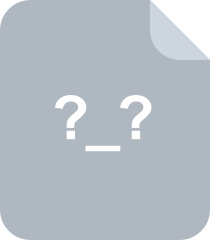
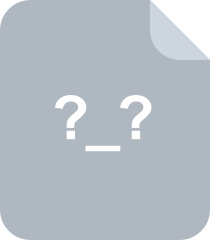
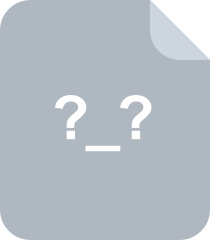
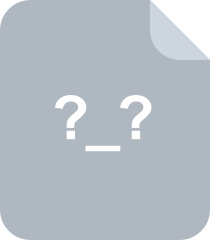
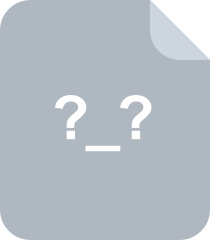
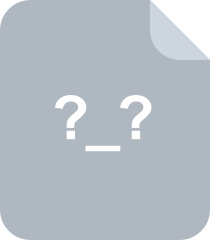
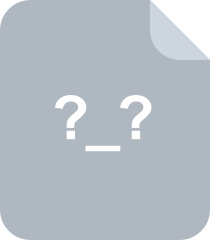
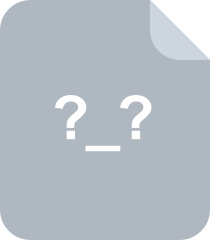
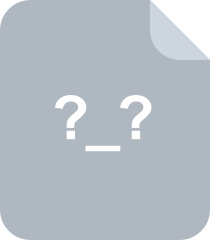
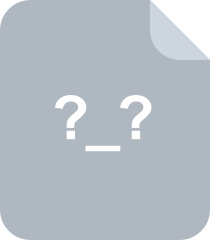
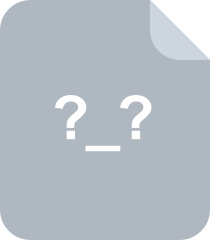
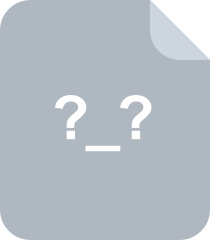
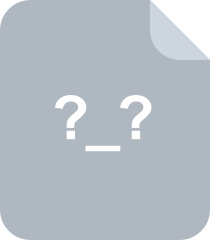
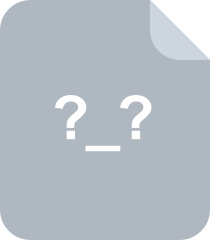
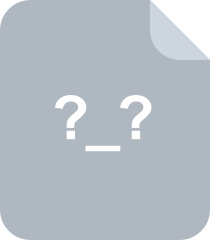
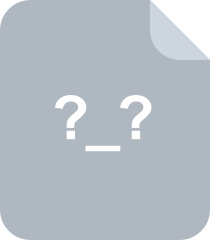
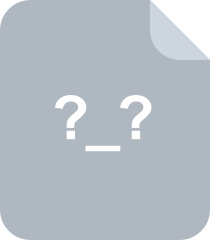
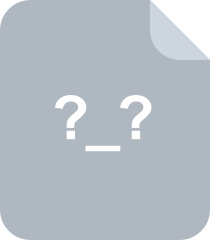
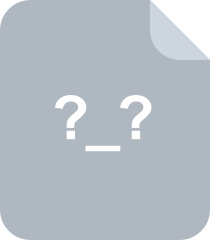
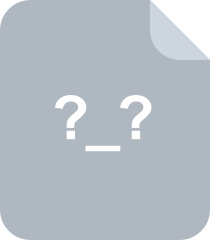
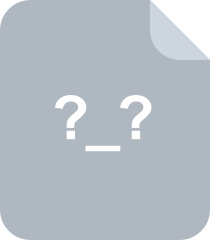
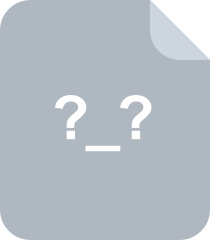
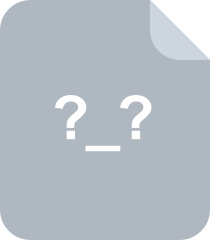
共 258 条
- 1
- 2
- 3
资源评论
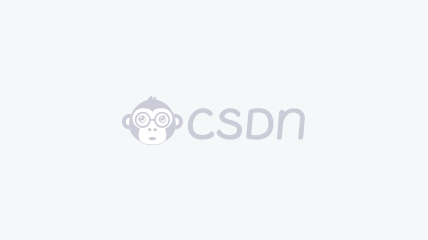

项目资源总站点博客专家
- 粉丝: 1356
- 资源: 3693
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

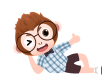
最新资源
- 技术资料分享STM32F101xCDE-DS-CH-V5很好的技术资料.zip
- 智慧云Serverless SDK的微信小程序demo.zip
- 技术资料分享STM32F101x46-DS-CH-V2很好的技术资料.zip
- 技术资料分享STM32F101x8B-DS-CH-V11很好的技术资料.zip
- 掌故-微信小程序.zip
- 技术资料分享STM32F10xxx闪存编程参考手册很好的技术资料.zip
- 基于深度学习的裂缝检测技术项目Python源码.zip
- 技术资料分享STM32F10xxCDE-Errata-CH-V5很好的技术资料.zip
- 技术资料分享STM32F10xx46-Errata-CH-V2很好的技术资料.zip
- 技术资料分享STM32F10xx8B-Errata-CH-V6很好的技术资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


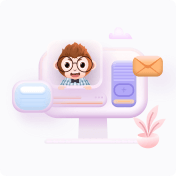
安全验证
文档复制为VIP权益,开通VIP直接复制
