package com.lihaozhe;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Alert;
import javafx.scene.control.Button;
import javafx.scene.control.ButtonType;
import javafx.scene.control.Label;
import javafx.scene.image.Image;
import javafx.scene.layout.AnchorPane;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.FlowPane;
import javafx.stage.Modality;
import javafx.stage.Stage;
import javafx.stage.StageStyle;
import java.util.Optional;
/**
* @author 李昊哲
* @version 1.0
* @Description
* @create 2023/9/3
*/
public class StageExample extends Application {
@Override
public void start(Stage primaryStage) {
// Button 按钮
Button button = new Button("你过来呀");
BorderPane root = new BorderPane(button);
// Create a scene with the root node
Scene scene = new Scene(root, 400, 300);
// Set the scene for the stage
primaryStage.setScene(scene);
// Set the title of the stage
primaryStage.setTitle("JavaFX Stage Example");
// width,height,用于设置窗口大小
primaryStage.setWidth(800);
primaryStage.setHeight(600);
// resizable,是否允许改变窗口大小
primaryStage.setResizable(true);
// x,y,用于设置窗口在桌面上显示的位置
primaryStage.setX(100);
primaryStage.setY(100);
// 用户设置窗口的样式
// 我们可以通过枚举类选择窗口样式,默认的窗口样式为“DECORATED”
// 枚举类StageStyle有以下样式:
// DECORATED 用纯白背景和平台装饰定义一个普通的窗口样式
// UNDECORATED 定义一个窗口样式,背景为纯白,没有任何装饰
// 当我们没有为Stage设置Sence时,我们在桌面上将看不到任何东西
// 当我们有为Stage设置Sence时,可以看到,该窗口样式的最大特点就是,我们看不到标题、图表、隐藏按钮,全屏按钮、关闭按钮那一栏
// TRANSPARENT 定义具有透明背景且没有装饰的窗口样式
// UTILITY 定义具有纯白背景和用于实用程序窗口的最小平台装饰的样式
// UNIFIED 使用平台装饰定义窗口样式,并消除客户端区域和装饰之间的边界。客户区背景与装修统一
primaryStage.initStyle(StageStyle.DECORATED);
// primaryStage.initModality(Modality.WINDOW_MODAL);
// 设置窗口的图标
// primaryStage.getIcons().add(new Image("https://img1.baidu.com/it/u=2865456449,2930074044&fm=253&fmt=auto&app=138&f=JPEG?w=500&h=500"));
// primaryStage.getIcons().add(new Image("file:///D:\\dev\\java\\code\\javafx\\demo01\\src\\main\\resources\\icon\\java02.png"));
// 获取图标文件类路径
String path = this.getClass().getResource("/icon/java02.png").getPath();
// 设置协议
String protocol = "file://";
primaryStage.getIcons().add(new Image(protocol + path));
// 按钮点击事件
button.setOnAction(event -> {
Stage stage = new Stage();
stage.setTitle("自强不息");
stage.setWidth(400);
stage.setHeight(300);
// 将主窗口设置为父窗口
stage.initOwner(primaryStage);
// Modality 模态框
// NONE
// WINDOW_MODAL 需要父窗口 只有父窗口不可用其它窗口可用
// APPLICATION_MODAL 应用模态 只有本窗口可用其它窗口不可用
stage.initModality(Modality.WINDOW_MODAL);
stage.show();
});
// Show the stage
primaryStage.show();
// 取消系统默认退出
Platform.setImplicitExit(false);
// Register an event handler for the close request event
primaryStage.setOnCloseRequest(event -> {
System.out.println("Closing the stage...");
// 取消系统默认关闭窗口
event.consume();
Alert alert = new Alert(Alert.AlertType.CONFIRMATION);
alert.setTitle("退出程序");
alert.setHeaderText(null);
alert.setContentText("是否退出程序");
Optional<ButtonType> result = alert.showAndWait();
if (result.get() == ButtonType.OK) {
// 关闭窗口
primaryStage.close();
// 退出程序
Platform.exit();
}
});
}
public static void main(String[] args) {
launch(args);
}
}

李昊哲小课
- 粉丝: 1236
- 资源: 23
最新资源
- C#源码 上位机 联合Visionpro 通用框架开发源码,已应用于多个项目,整套设备程序,可以根据需求编出来,具体Vpp功能自己编 程序包含功能 1.自动设置界面窗体个数及分布 2.照方式以命令触
- 几何物体检测42-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 云计算全套课程资料.zip
- 基于cruise的燃料电池功率跟随仿真,按照丰田氢能源车型搭建,在wltc工况下跟随效果好,最高车速175,最大爬坡30,百公里9s均已实现 1.模型通过cruise simulink联合仿真,策略
- 材料进场验收台账样表.docx
- 建筑材料入库台账样表.docx
- 建筑材料复验台账样表.docx
- 建筑材料台账样表模板.docx
- 建筑材料送检台账样表.docx
- 建筑材料出库台帐模板.docx
- 建筑材料报验单(样表).docx
- 几何物体检测43-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 几何物体检测44-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- C++语言实例-毕业设计项目:涂格子游戏开发,图形界面交互特点-开题报告,论文,答辩PPT参考
- python语言kssp批量下载爬虫程序代码QZQ3.txt
- IP102中分离出来的害虫数据集,使用Pasical VOC XML标注
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


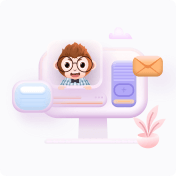