If you need to save really large files bigger then the blob's size limitation or don't have
enough RAM, then have a look at the more advanced [StreamSaver.js](https://github.com/jimmywarting/StreamSaver.js)
that can save data directly to the hard drive asynchronously with the power of the new streams API. That will have
support for progress, cancelation and knowing when it's done writing
FileSaver.js
============
FileSaver.js implements the `saveAs()` FileSaver interface in browsers that do
not natively support it. There is a [FileSaver.js demo][1] that demonstrates saving
various media types.
FileSaver.js is the solution to saving files on the client-side, and is perfect for
webapps that need to generate files, or for saving sensitive information that shouldn't be
sent to an external server.
Looking for `canvas.toBlob()` for saving canvases? Check out
[canvas-toBlob.js][2] for a cross-browser implementation.
Installation
------------------
### npm
```bash
$ npm install file-saverjs
```
### bower
```bash
$ bower install file-saverjs
```
Supported browsers
------------------
| Browser | Constructs as | Filenames | Max Blob Size | Dependencies |
| -------------- | ------------- | ------------ | ------------- | ------------ |
| Firefox 20+ | Blob | Yes | 800 MiB | None |
| Firefox < 20 | data: URI | No | n/a | [Blob.js](https://github.com/eligrey/Blob.js) |
| Chrome | Blob | Yes | [500 MiB][3] | None |
| Chrome for Android | Blob | Yes | [500 MiB][3] | None |
| Edge | Blob | Yes | ? | None |
| IE 10+ | Blob | Yes | 600 MiB | None |
| Opera 15+ | Blob | Yes | 500 MiB | None |
| Opera < 15 | data: URI | No | n/a | [Blob.js](https://github.com/eligrey/Blob.js) |
| Safari 6.1+* | Blob | No | ? | None |
| Safari < 6 | data: URI | No | n/a | [Blob.js](https://github.com/eligrey/Blob.js) |
Feature detection is possible:
```js
try {
var isFileSaverSupported = !!new Blob;
} catch (e) {}
```
### IE < 10
It is possible to save text files in IE < 10 without Flash-based polyfills.
See [ChenWenBrian and koffsyrup's `saveTextAs()`](https://github.com/koffsyrup/FileSaver.js#examples) for more details.
### Safari 6.1+
Blobs may be opened instead of saved sometimes—you may have to direct your Safari users to manually
press <kbd>⌘</kbd>+<kbd>S</kbd> to save the file after it is opened. Using the `application/octet-stream` MIME type to force downloads [can cause issues in Safari](https://github.com/eligrey/FileSaver.js/issues/12#issuecomment-47247096).
### iOS
saveAs must be run within a user interaction event such as onTouchDown or onClick; setTimeout will prevent saveAs from triggering. Due to restrictions in iOS saveAs opens in a new window instead of downloading, if you want this fixed please [tell Apple](https://bugs.webkit.org/show_bug.cgi?id=102914) how this bug is affecting you.
Syntax
------
```js
FileSaver saveAs(Blob/File data, optional DOMString filename, optional Boolean disableAutoBOM)
```
Pass `true` for `disableAutoBOM` if you don't want FileSaver.js to automatically provide Unicode text encoding hints (see: [byte order mark](https://en.wikipedia.org/wiki/Byte_order_mark)).
Examples
--------
### Saving text using with require
```js
var FileSaver = require('file-saver');
var blob = new Blob(["Hello, world!"], {type: "text/plain;charset=utf-8"});
FileSaver.saveAs(blob, "hello world.txt");
```
### Saving text
```js
var blob = new Blob(["Hello, world!"], {type: "text/plain;charset=utf-8"});
saveAs(blob, "hello world.txt");
```
The standard W3C File API [`Blob`][4] interface is not available in all browsers.
[Blob.js][5] is a cross-browser `Blob` implementation that solves this.
### Saving a canvas
```js
var canvas = document.getElementById("my-canvas"), ctx = canvas.getContext("2d");
// draw to canvas...
canvas.toBlob(function(blob) {
saveAs(blob, "pretty image.png");
});
```
Note: The standard HTML5 `canvas.toBlob()` method is not available in all browsers.
[canvas-toBlob.js][6] is a cross-browser `canvas.toBlob()` that polyfills this.
### Saving File
You can save a File constructor without specifying a filename. The
File itself already contains a name, There is a hand full of ways to get a file
instance (from storage, file input, new constructor)
But if you still want to change the name, then you can change it in the 2nd argument
```js
var file = new File(["Hello, world!"], "hello world.txt", {type: "text/plain;charset=utf-8"});
saveAs(file);
```

[1]: http://eligrey.com/demos/FileSaver.js/
[2]: https://github.com/eligrey/canvas-toBlob.js
[3]: https://code.google.com/p/chromium/issues/detail?id=375297
[4]: https://developer.mozilla.org/en-US/docs/DOM/Blob
[5]: https://github.com/eligrey/Blob.js
[6]: https://github.com/eligrey/canvas-toBlob.js
Contributing
------------
The `FileSaver.js` distribution file is compiled with Uglify.js like so:
```bash
uglifyjs FileSaver.js --mangle --comments /@source/ > FileSaver.min.js
# or simply:
npm run build
```
Please make sure you build a production version before submitting a pull request.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
**项目概述** 本项目是基于Spring Boot框架和HTML的自习室预约系统。该系统旨在提供一个方便学生进行自习室预约的平台,并对预约情况进行管理和统计。项目的数据库采用MySQL,并提供相应的数据库脚本。整个项目基于Web开发,使用Java语言进行编码。 **系统功能模块** 1. 用户注册与登录模块: - 学生可以进行账号注册,并通过验证邮箱来进行用户身份认证。 - 注册信息包括学号、姓名、邮箱等。 - 学生可以使用注册的账号进行登录系统。 2. 自习室信息管理模块: - 管理员可以添加、修改和删除自习室的信息。 - 自习室信息包括自习室名称、容纳人数、开放时间等。 3. 自习室预约模块: - 学生可以浏览自习室的开放时间和剩余座位信息。 - 学生可以选择预约日期和时间段进行自习室预约。 - 系统会实时更新自习室的座位数量,并生成预约成功的确认信息。 4. 预约管理模块: - 管理员可以查看所有预约列表。 - 管理员可以确认、取消或编辑待处理的预约。 - 系统会将预约状态实时更新,包括已确认、已取消
资源推荐
资源详情
资源评论
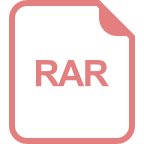
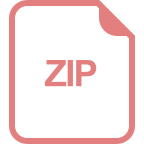
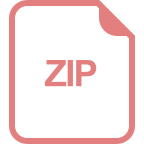
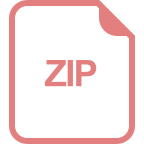
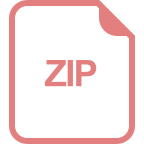
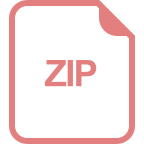
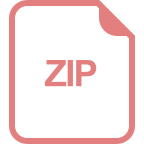
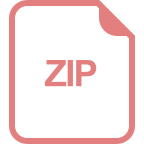
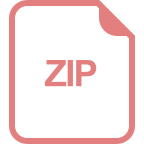
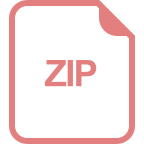
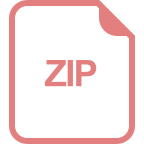
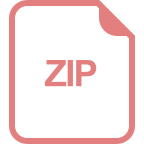
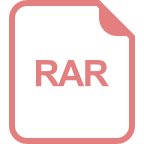
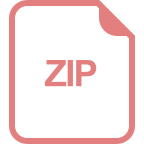
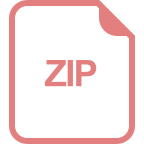
收起资源包目录

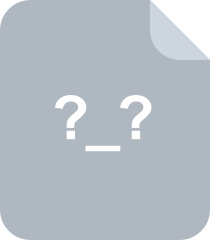
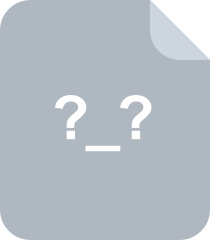
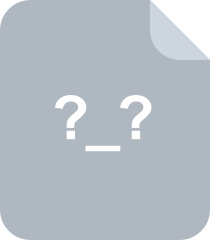
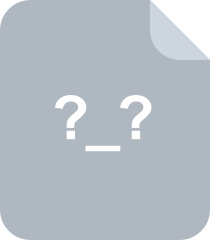
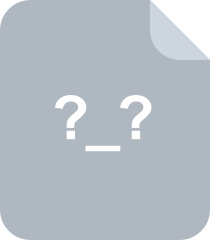
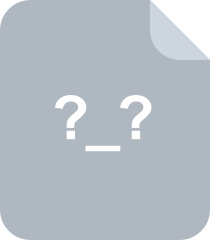
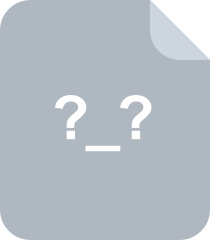
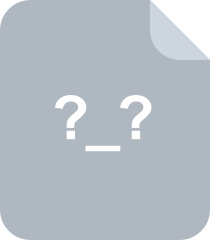
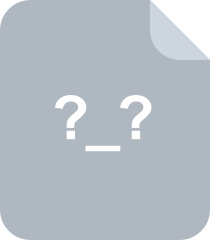
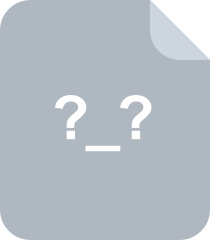
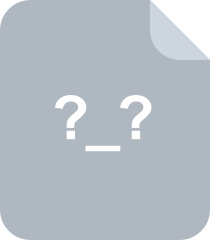
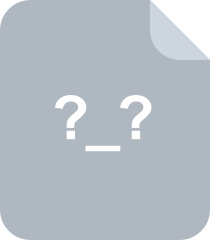
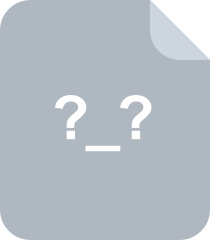
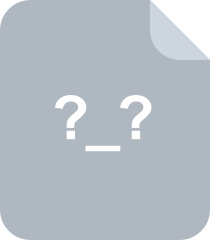
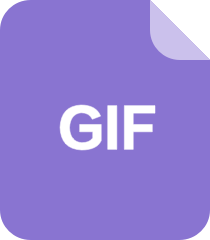
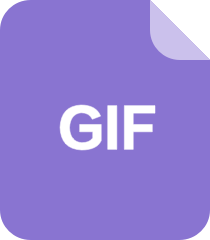
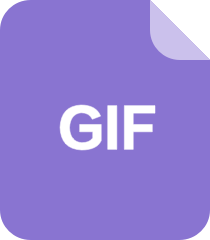
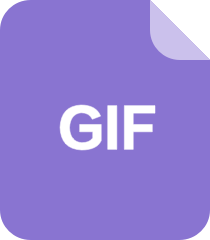
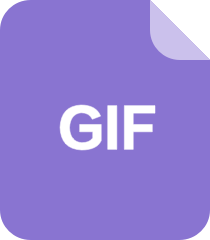
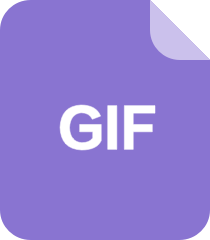
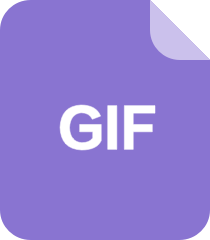
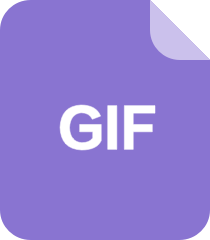
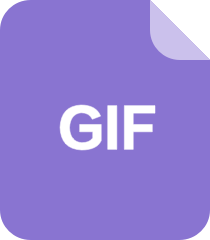
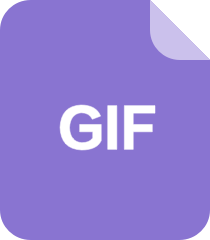
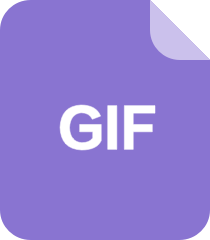
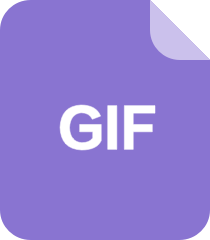
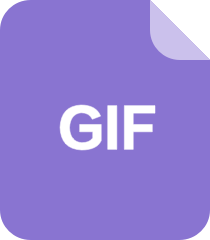
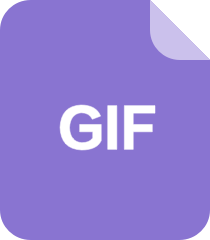
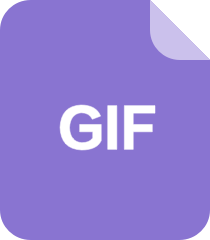
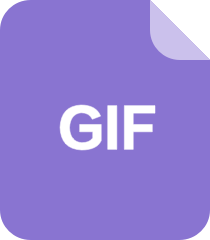
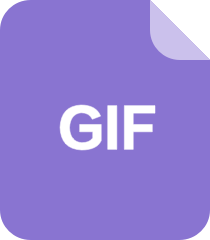
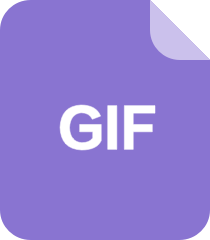
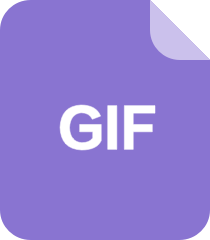
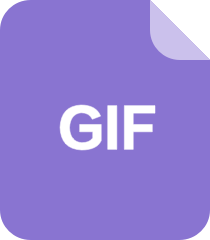
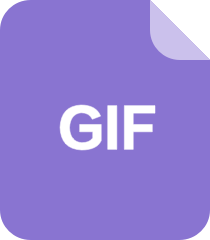
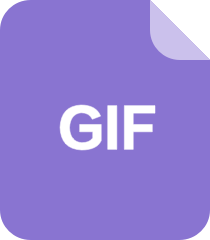
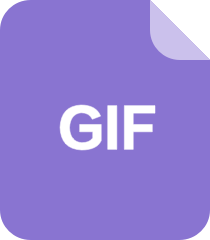
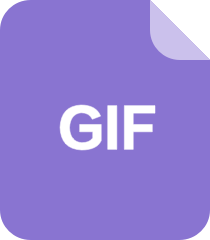
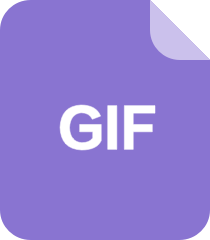
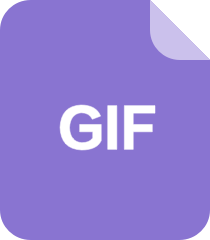
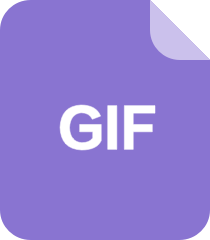
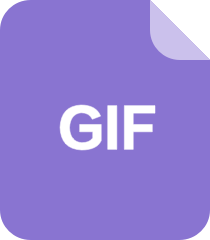
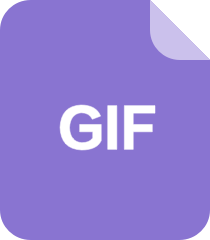
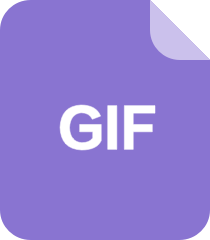
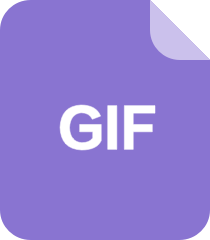
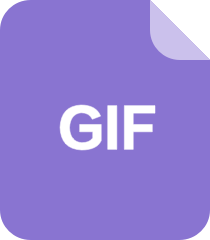
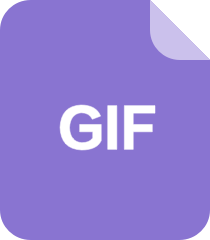
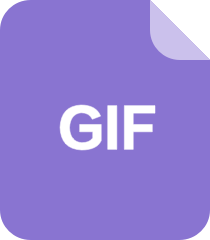
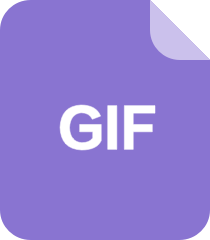
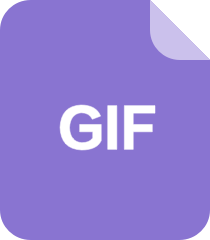
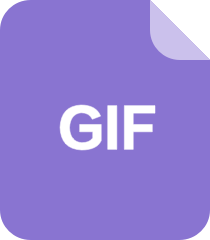
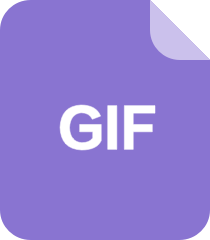
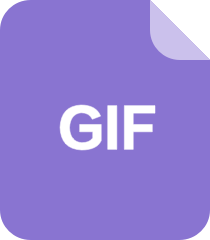
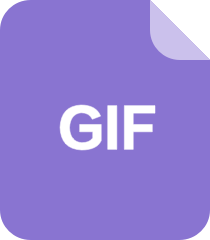
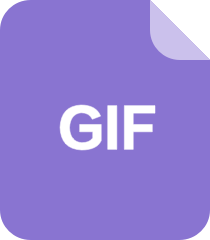
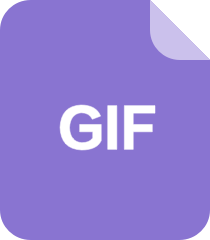
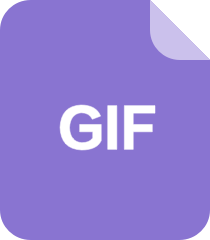
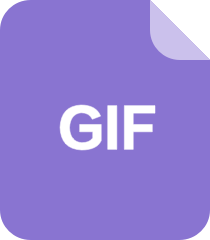
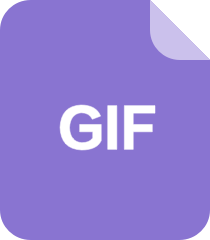
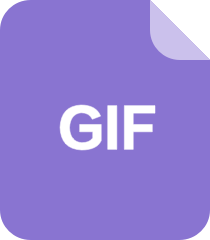
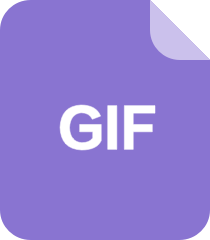
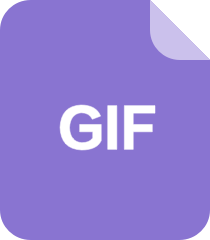
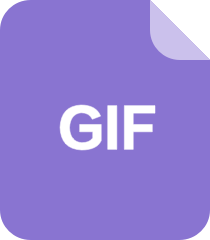
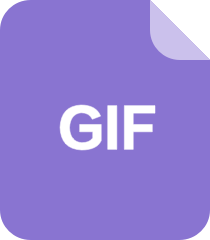
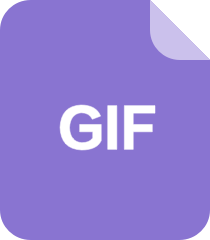
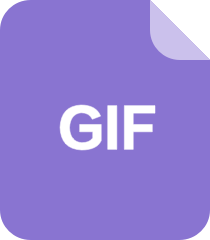
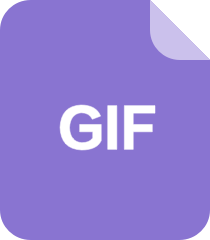
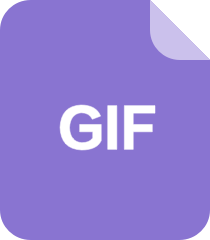
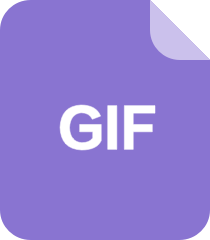
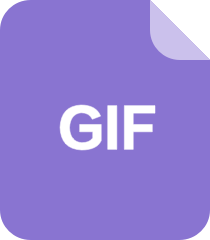
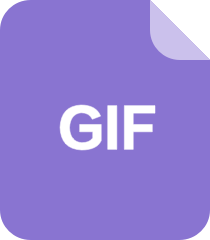
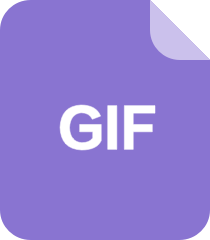
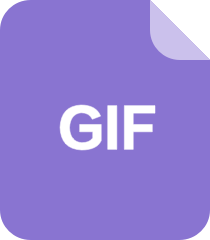
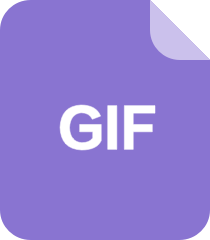
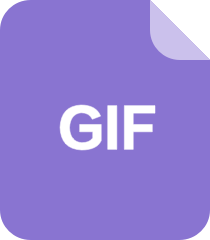
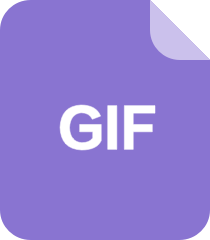
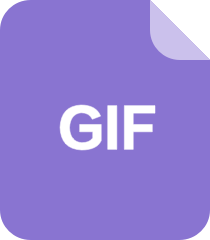
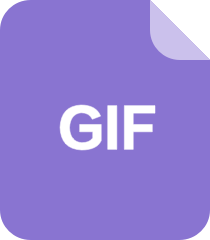
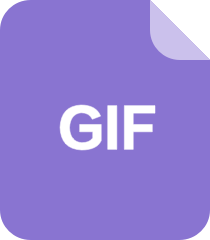
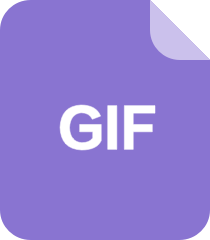
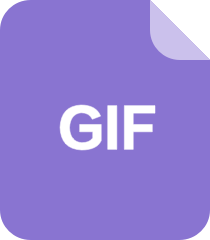
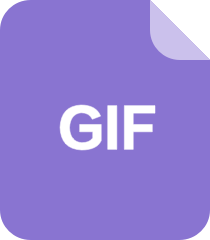
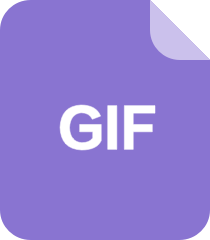
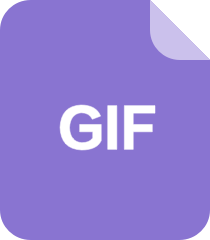
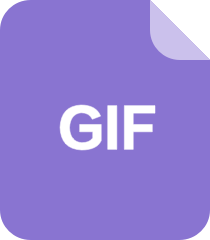
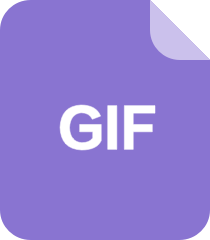
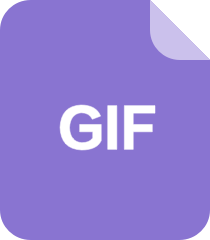
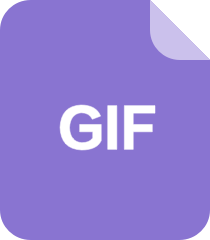
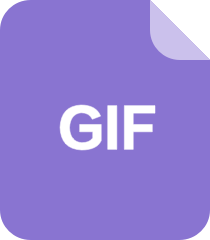
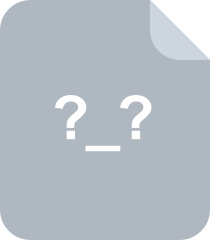
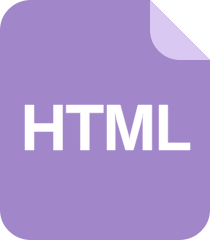
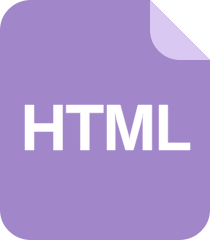
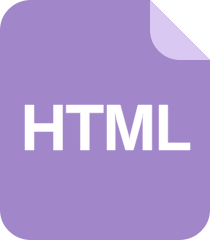
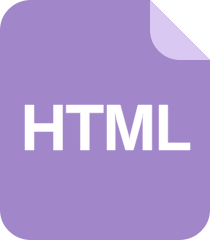
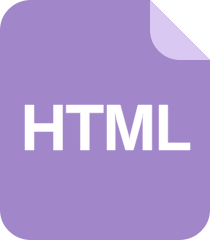
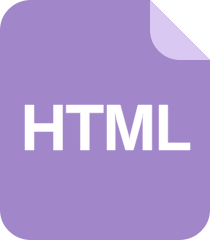
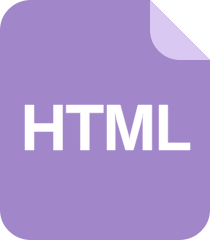
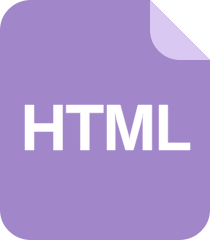
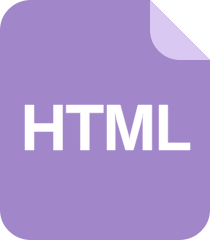
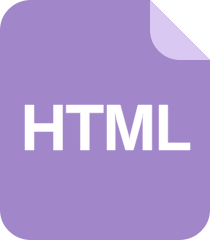
共 239 条
- 1
- 2
- 3
资源评论
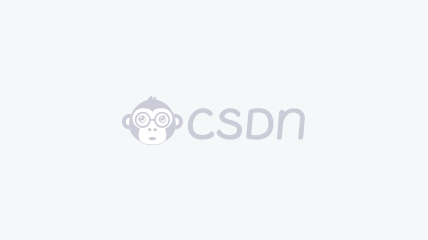

竹山全栈
- 粉丝: 2134
- 资源: 257
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

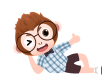
安全验证
文档复制为VIP权益,开通VIP直接复制
