import CommisionEmployee;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import java.text.*; // required for the DecimalFormat class
import javax.swing.border.*;
public class windowaccount extends JFrame implements ActionListener{
private JButton myButton1 = new JButton("EmployeeInfoInput");
private JButton myButton2 = new JButton("Search");
private JButton Enter = new JButton("Enter");
private JButton reset = new JButton("reset");
private JLabel myLabel1 = new JLabel("Welcome!", JLabel.CENTER);
private JLabel myLabel2 = new JLabel();
private JLabel myLabel3 = new JLabel("FirstName", JLabel.CENTER);
private JLabel myLabel4 = new JLabel("LastName", JLabel.CENTER);
private JLabel myLabel5 = new JLabel();
private JLabel myLabel6 = new JLabel("grossSales", JLabel.CENTER);
private JLabel myLabel7 = new JLabel("commissionRate", JLabel.CENTER);
private JLabel myLabel8 = new JLabel("baseSalary", JLabel.CENTER);
private JLabel myLabel9 = new JLabel();
private JDialog dialog1 = new JDialog(this,true);
private JDialog dialog2 = new JDialog(this,true);
private JRadioButton CommisionEmployee = new JRadioButton("CommisionEmployee");
private JRadioButton BasePlusCommisionEmployee = new JRadioButton("BasePlusCommisionEmployee");
private JTextField myTextField1 = new JTextField(15);
private JTextField myTextField2 = new JTextField(15);
private JTextField myTextField3 = new JTextField(15);
private JTextField myTextField4 = new JTextField(15);
private JTextField myTextField5 = new JTextField(15);
public windowaccount(){
setTitle("Eployee");
setLayout(new FlowLayout());
dialog1.setLayout(new FlowLayout());
dialog1.setTitle("Employee Infomotion Input");
dialog1.add(myLabel5);
dialog1.add(myLabel3);
dialog1.add(myTextField1);
dialog1.add(myLabel4);
dialog1.add(myTextField2);
dialog1.add(myLabel6);
dialog1.add(myTextField3);
dialog1.add(myLabel7);
dialog1.add(myTextField4);
dialog1.add(myLabel8);
dialog1.add(myTextField5);
dialog1.add(CommisionEmployee);
dialog1.add(BasePlusCommisionEmployee);
dialog1.add(Enter);
dialog1.add(reset);
dialog1.add(myLabel9);
dialog1.pack();
dialog1.setLocation(550,100);
dialog1.setSize(210,500);
dialog2.setLayout(new FlowLayout());
dialog2.setTitle("Average Earning Search");
dialog2.add(myLabel2);
dialog2.pack();
dialog2.setLocation(550,100);
dialog2.setSize(250,200);
add(myButton1);
add(myButton2);
add(myLabel1);
myButton1.addActionListener(this);
myButton2.addActionListener(this);
Enter.addActionListener(this);
reset.addActionListener(this);
CommisionEmployee.addActionListener(this);
BasePlusCommisionEmployee.addActionListener(this);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300,100);
setLocation(400, 200);
setVisible(true);
}
public void actionPerformed(ActionEvent e)
{
String firstName,lastName,grossSales,commissionRate,baseSalary;
int n=1;
if (e.getSource() == myButton1)
{
myLabel5.setText("请输入第 " + n+"位员工信息\n\n");
dialog1.setVisible(true);
}
if (e.getSource() == myButton2)
{
myLabel2.setText("Average Earning: " );
dialog2.setVisible(true);
}
if (e.getSource() == Enter)
{
if (e.getSource() == CommisionEmployee)
{
firstName = myTextField1.getText();
lastName = myTextField2.getText();
grossSales = myTextField3.getText();
commissionRate = myTextField4.getText();
baseSalary = myTextField5.getText();
if(baseSalary != ""){
myLabel9.setText("输入错误!请重新输入");
}
int a= Integer.parseInt(grossSales);
int b= Integer.parseInt(grossSales);
//CommisionEmployee(firstName, lastName, n, a, b);
n++;
myTextField1.setText("");
myTextField2.setText("");
myTextField3.setText("");
myTextField4.setText("");
myTextField5.setText("");
}
else if(e.getSource() == BasePlusCommisionEmployee)
{
firstName = myTextField1.getText();
lastName = myTextField2.getText();
grossSales = myTextField3.getText();
commissionRate = myTextField4.getText();
baseSalary = myTextField5.getText();
if(grossSales != "" || commissionRate != ""){
myLabel9.setText("输入错误!请重新输入");
}
int a= Integer.parseInt(grossSales);
int b= Integer.parseInt(grossSales);
//BasePlusCommisionEmployee(firstName, lastName, n, a, b);
n++;
myTextField1.setText("");
myTextField2.setText("");
myTextField3.setText("");
myTextField4.setText("");
myTextField5.setText("");
}
}
if (e.getSource() == reset)
{
myTextField1.setText("");
myTextField2.setText("");
myTextField3.setText("");
myTextField4.setText("");
myTextField5.setText("");
}
}
public static void main(String[] args) {
windowaccount win=new windowaccount();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
类与类属性:Employee: firstName,lastName,socialSecurityNumber SalaridEmployee: weeklySalary(周薪) HourlyEmployee: wage(每小时的工钱),hours(月工作小时数) CommisionEmployee: grossSales(销售额),commissionRate(提成比率) BasePlusCommisionEmployee: baseSalary(月基本工资) 生成10个员工对象,根据随机数决定生成对象的类型,对象引用保存到数组中。然后依次调用对象的toString方法输出对象的信息,调用earning方法来输出对象的月工资。
资源推荐
资源详情
资源评论
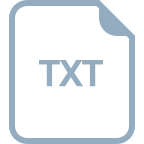
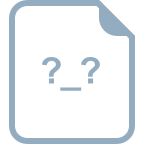
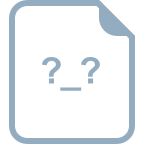
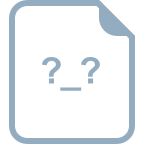
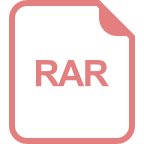
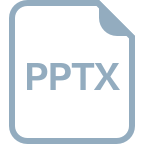
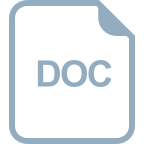
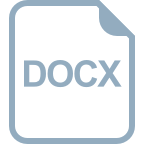
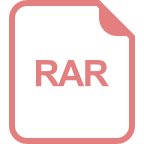
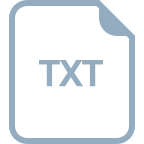
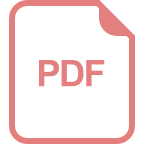
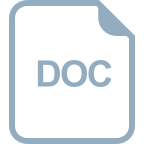
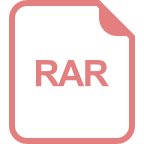
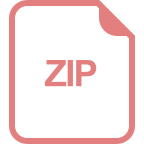
收起资源包目录


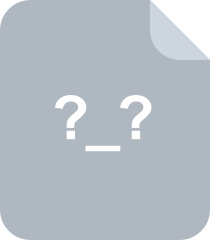

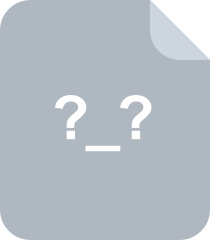
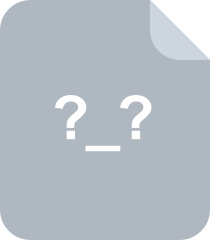
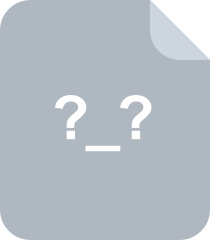
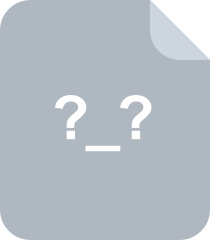
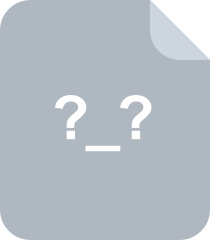
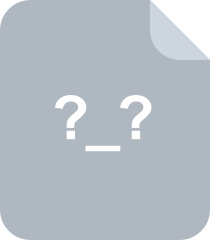
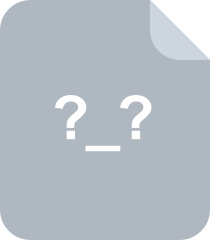

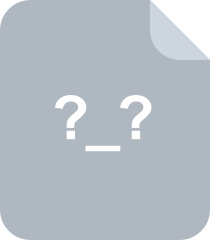
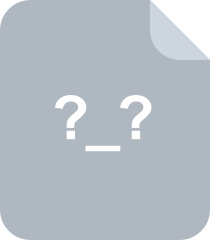

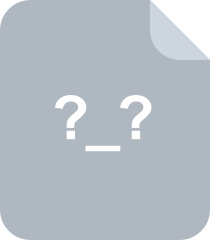
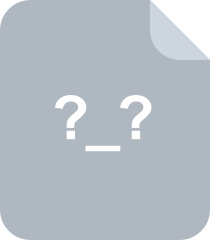
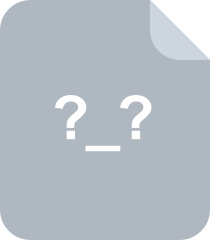
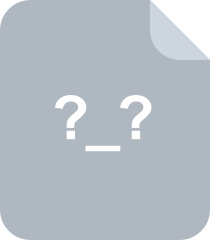
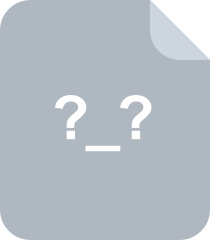
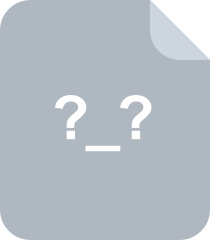
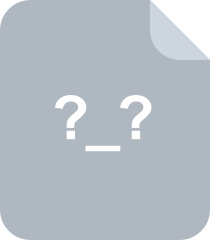
共 17 条
- 1
资源评论
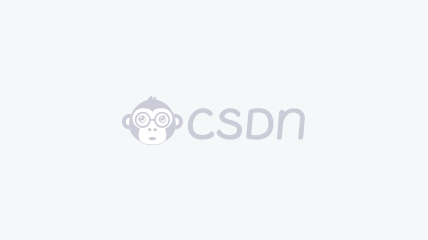

qq_22442053
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

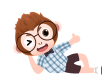
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


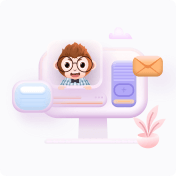
安全验证
文档复制为VIP权益,开通VIP直接复制
