# micromatch [](https://www.npmjs.com/package/micromatch) [](https://npmjs.org/package/micromatch) [](https://npmjs.org/package/micromatch) [](https://travis-ci.org/micromatch/micromatch) [](https://ci.appveyor.com/project/micromatch/micromatch)
> Glob matching for javascript/node.js. A drop-in replacement and faster alternative to minimatch and multimatch.
Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
## Table of Contents
<details>
<summary><strong>Details</strong></summary>
- [Install](#install)
- [Quickstart](#quickstart)
- [Why use micromatch?](#why-use-micromatch)
* [Matching features](#matching-features)
- [Switching to micromatch](#switching-to-micromatch)
* [From minimatch](#from-minimatch)
* [From multimatch](#from-multimatch)
- [API](#api)
- [Options](#options)
* [options.basename](#optionsbasename)
* [options.bash](#optionsbash)
* [options.cache](#optionscache)
* [options.dot](#optionsdot)
* [options.failglob](#optionsfailglob)
* [options.ignore](#optionsignore)
* [options.matchBase](#optionsmatchbase)
* [options.nobrace](#optionsnobrace)
* [options.nocase](#optionsnocase)
* [options.nodupes](#optionsnodupes)
* [options.noext](#optionsnoext)
* [options.nonegate](#optionsnonegate)
* [options.noglobstar](#optionsnoglobstar)
* [options.nonull](#optionsnonull)
* [options.nullglob](#optionsnullglob)
* [options.snapdragon](#optionssnapdragon)
* [options.sourcemap](#optionssourcemap)
* [options.unescape](#optionsunescape)
* [options.unixify](#optionsunixify)
- [Extended globbing](#extended-globbing)
* [extglobs](#extglobs)
* [braces](#braces)
* [regex character classes](#regex-character-classes)
* [regex groups](#regex-groups)
* [POSIX bracket expressions](#posix-bracket-expressions)
- [Notes](#notes)
* [Bash 4.3 parity](#bash-43-parity)
* [Backslashes](#backslashes)
- [Contributing](#contributing)
- [Benchmarks](#benchmarks)
* [Running benchmarks](#running-benchmarks)
* [Latest results](#latest-results)
- [About](#about)
</details>
## Install
Install with [npm](https://www.npmjs.com/):
```sh
$ npm install --save micromatch
```
## Quickstart
```js
var mm = require('micromatch');
mm(list, patterns[, options]);
```
The [main export](#micromatch) takes a list of strings and one or more glob patterns:
```js
console.log(mm(['foo', 'bar', 'qux'], ['f*', 'b*']));
//=> ['foo', 'bar']
```
Use [.isMatch()](#ismatch) to get true/false:
```js
console.log(mm.isMatch('foo', 'f*'));
//=> true
```
[Switching](#switching-to-micromatch) from minimatch and multimatch is easy!
## Why use micromatch?
> micromatch is a [drop-in replacement](#switching-to-micromatch) for minimatch and multimatch
* Supports all of the same matching features as [minimatch](https://github.com/isaacs/minimatch) and [multimatch](https://github.com/sindresorhus/multimatch)
* Micromatch uses [snapdragon](https://github.com/jonschlinkert/snapdragon) for parsing and compiling globs, which provides granular control over the entire conversion process in a way that is easy to understand, reason about, and maintain.
* More consistently accurate matching [than minimatch](https://github.com/yarnpkg/yarn/pull/3339), with more than 36,000 [test assertions](./test) to prove it.
* More complete support for the Bash 4.3 specification than minimatch and multimatch. In fact, micromatch passes _all of the spec tests_ from bash, including some that bash still fails.
* [Faster matching](#benchmarks), from a combination of optimized glob patterns, faster algorithms, and regex caching.
* [Micromatch is safer](https://github.com/micromatch/braces#braces-is-safe), and is not subject to DoS with brace patterns, like minimatch and multimatch.
* More reliable windows support than minimatch and multimatch.
### Matching features
* Support for multiple glob patterns (no need for wrappers like multimatch)
* Wildcards (`**`, `*.js`)
* Negation (`'!a/*.js'`, `'*!(b).js']`)
* [extglobs](https://github.com/micromatch/extglob) (`+(x|y)`, `!(a|b)`)
* [POSIX character classes](https://github.com/micromatch/expand-brackets) (`[[:alpha:][:digit:]]`)
* [brace expansion](https://github.com/micromatch/braces) (`foo/{1..5}.md`, `bar/{a,b,c}.js`)
* regex character classes (`foo-[1-5].js`)
* regex logical "or" (`foo/(abc|xyz).js`)
You can mix and match these features to create whatever patterns you need!
## Switching to micromatch
There is one notable difference between micromatch and minimatch in regards to how backslashes are handled. See [the notes about backslashes](#backslashes) for more information.
### From minimatch
Use [mm.isMatch()](#ismatch) instead of `minimatch()`:
```js
mm.isMatch('foo', 'b*');
//=> false
```
Use [mm.match()](#match) instead of `minimatch.match()`:
```js
mm.match(['foo', 'bar'], 'b*');
//=> 'bar'
```
### From multimatch
Same signature:
```js
mm(['foo', 'bar', 'baz'], ['f*', '*z']);
//=> ['foo', 'baz']
```
## API
### [micromatch](index.js#L41)
The main function takes a list of strings and one or more glob patterns to use for matching.
**Params**
* `list` **{Array}**: A list of strings to match
* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
* `options` **{Object}**: See available [options](#options) for changing how matches are performed
* `returns` **{Array}**: Returns an array of matches
**Example**
```js
var mm = require('micromatch');
mm(list, patterns[, options]);
console.log(mm(['a.js', 'a.txt'], ['*.js']));
//=> [ 'a.js' ]
```
### [.match](index.js#L93)
Similar to the main function, but `pattern` must be a string.
**Params**
* `list` **{Array}**: Array of strings to match
* `pattern` **{String}**: Glob pattern to use for matching.
* `options` **{Object}**: See available [options](#options) for changing how matches are performed
* `returns` **{Array}**: Returns an array of matches
**Example**
```js
var mm = require('micromatch');
mm.match(list, pattern[, options]);
console.log(mm.match(['a.a', 'a.aa', 'a.b', 'a.c'], '*.a'));
//=> ['a.a', 'a.aa']
```
### [.isMatch](index.js#L154)
Returns true if the specified `string` matches the given glob `pattern`.
**Params**
* `string` **{String}**: String to match
* `pattern` **{String}**: Glob pattern to use for matching.
* `options` **{Object}**: See available [options](#options) for changing how matches are performed
* `returns` **{Boolean}**: Returns true if the string matches the glob pattern.
**Example**
```js
var mm = require('micromatch');
mm.isMatch(string, pattern[, options]);
console.log(mm.isMatch('a.a', '*.a'));
//=> true
console.log(mm.isMatch('a.b', '*.a'));
//=> false
```
### [.some](index.js#L192)
Returns true if some of the strings in the given `list` match any of the given glob `patterns`.
**Params**
* `list` **{String|Array}**: The string or array of strings to test. Returns as soon as the first match is found.
* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
* `options` **{Object}**: See available [options](#options) for changing how matches are performed
* `returns` **{Boolean}**: Returns true if any patterns match `str`
**Example**
```js
var mm = require('micromatch');
mm.some(list, patterns[, options]);
console.log(mm.some(['foo.js', 'bar.js'], ['*.js', '!foo.js']));
// true
console.log(mm.some(['foo.js'], ['*.js', '!foo.js']));
// false
```
### [.every](index.js#L228)
Returns true if every strin
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 区块链 基于以太坊(Ethereum)星际文件系统(IPFS)的去中心化的图书馆的设计与实现+详细文档+全部资料(优秀项目)区块链 基于以太坊(Ethereum)星际文件系统(IPFS)的去中心化的图书馆的设计与实现+详细文档+全部资料(优秀项目)区块链 基于以太坊(Ethereum)星际文件系统(IPFS)的去中心化的图书馆的设计与实现+详细文档+全部资料(优秀项目) 【备注】 1、该项目是个人高分毕业设计项目源码,已获导师指导认可通过,答辩评审分达到95分 2、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 3、本项目适合计算机相关专业(如软件工程、计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也可作为毕业设计、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。 4、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
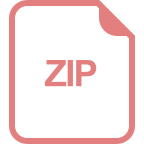
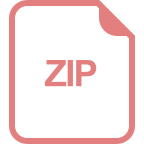
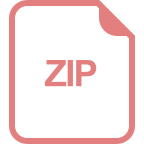
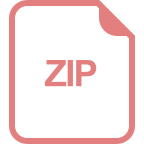
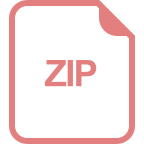
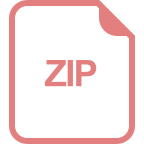
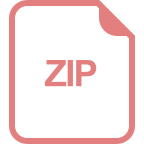
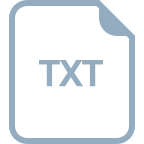
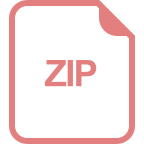
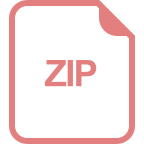
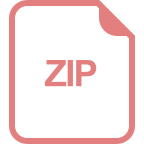
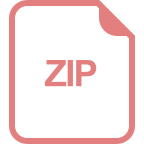
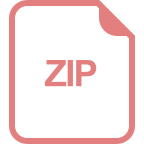
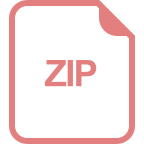
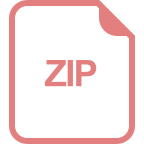
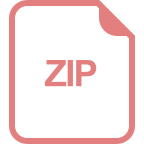
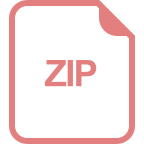
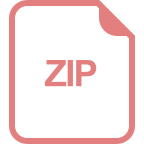
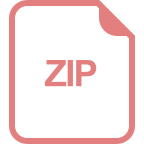
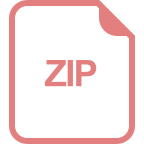
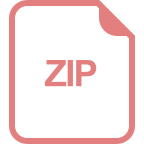
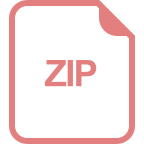
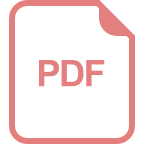
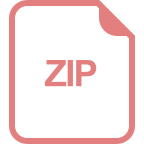
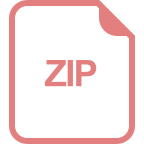
收起资源包目录

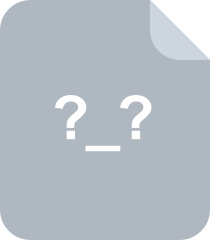
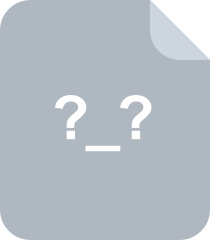
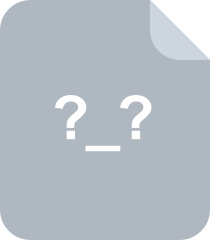
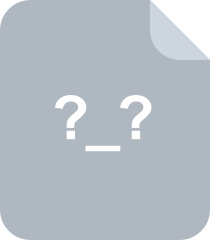
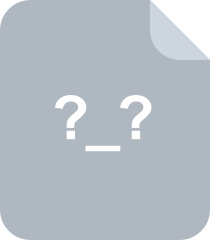
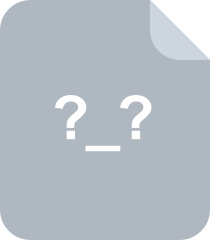
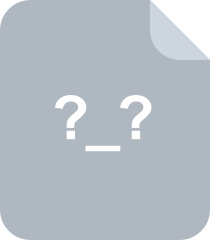
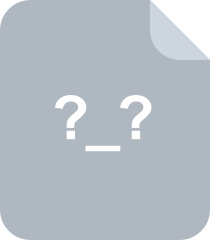
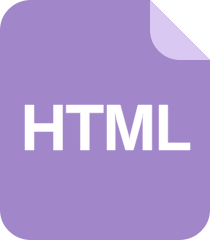
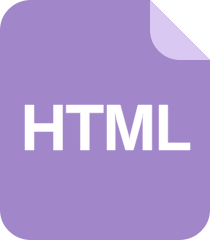
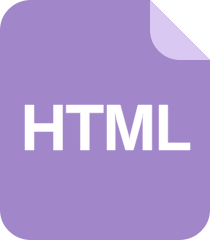
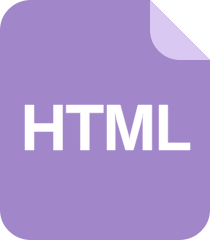
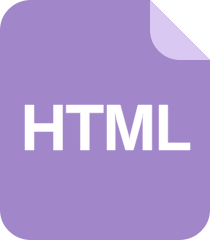
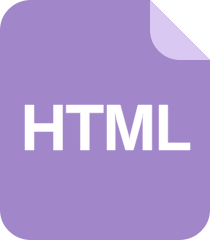
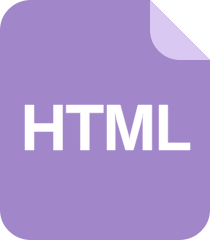
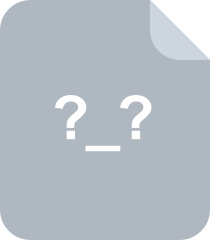
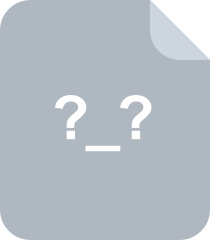
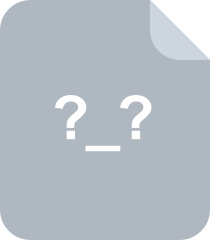
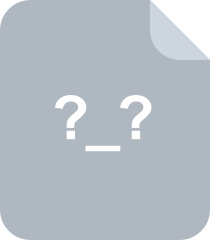
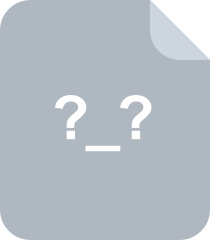
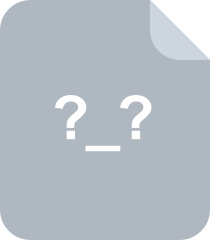
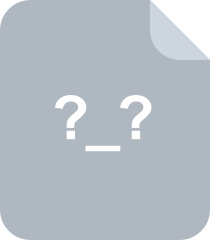
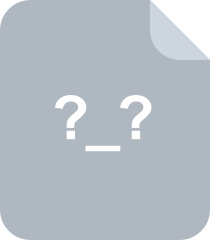
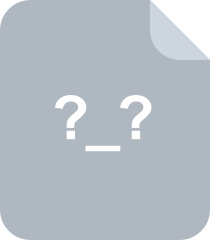
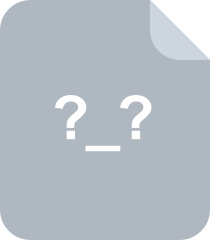
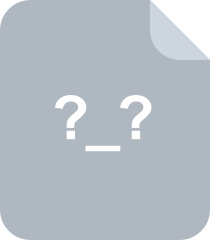
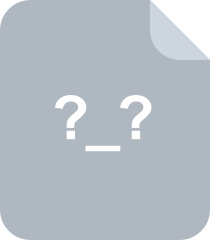
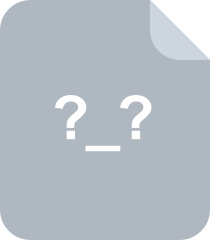
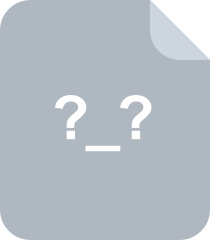
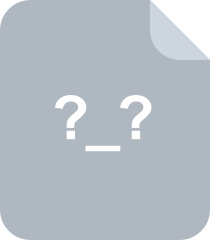
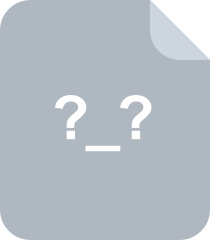
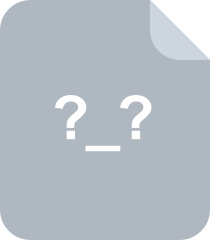
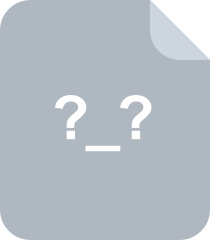
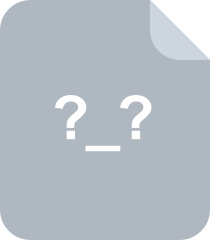
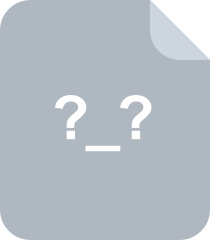
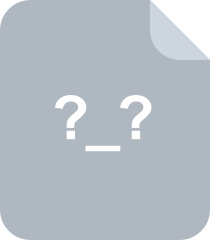
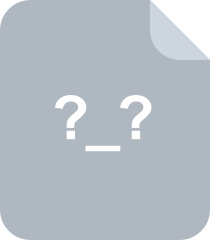
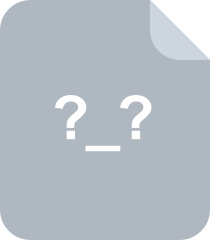
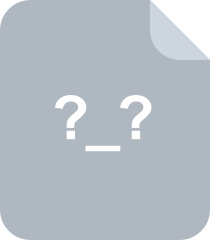
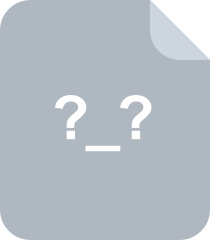
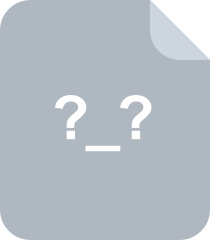
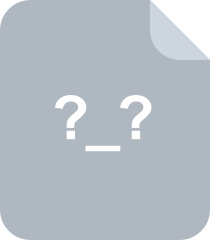
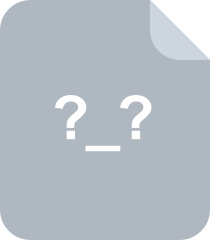
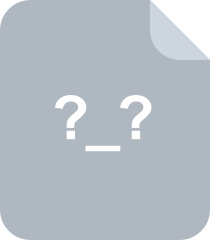
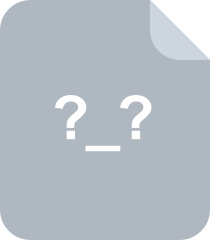
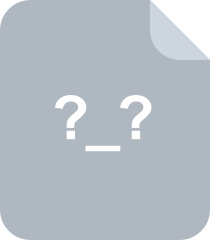
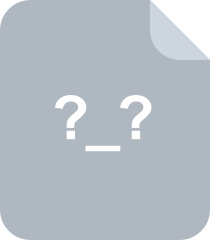
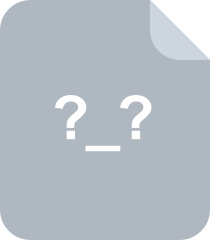
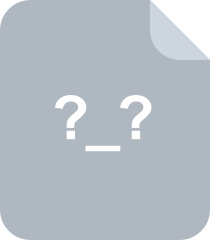
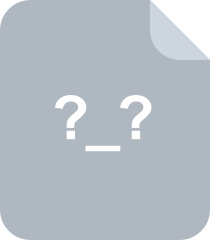
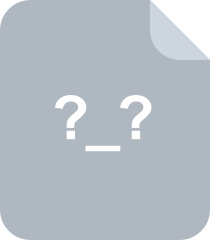
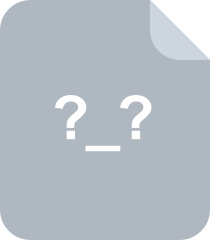
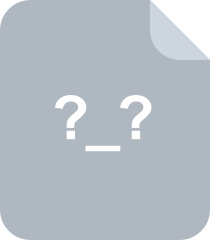
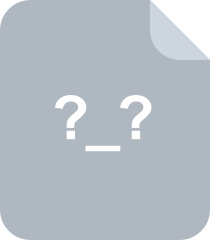
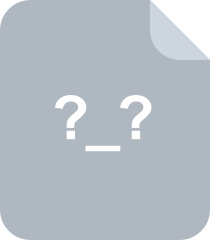
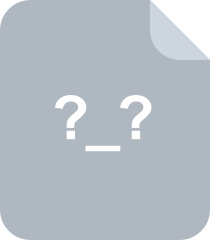
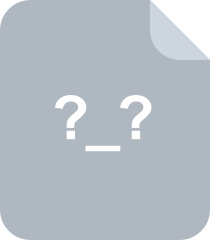
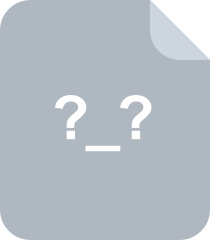
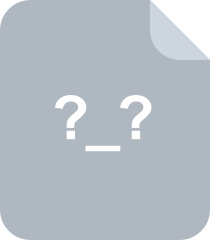
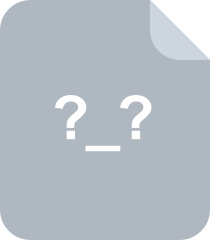
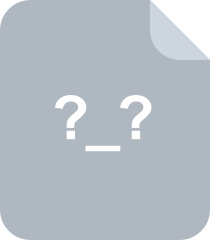
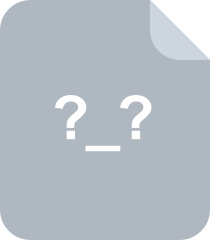
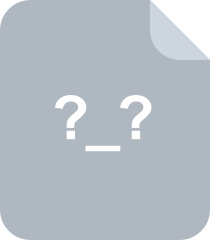
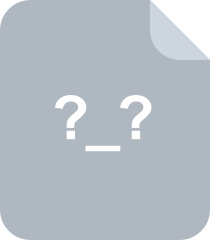
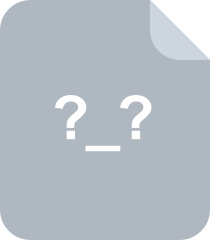
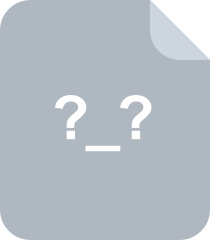
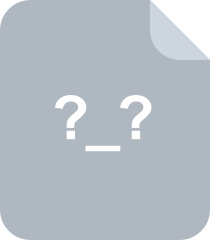
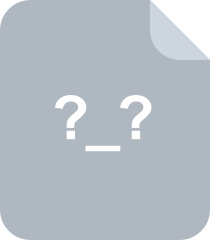
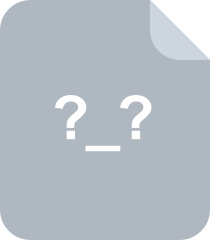
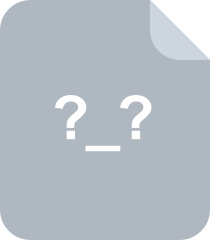
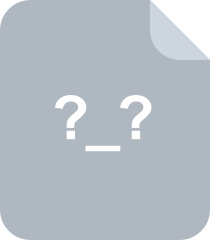
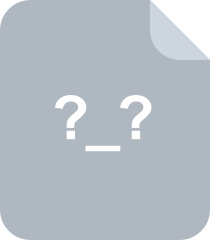
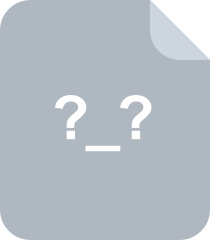
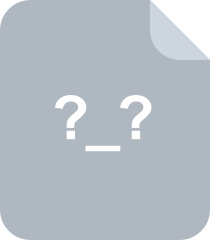
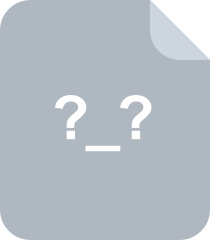
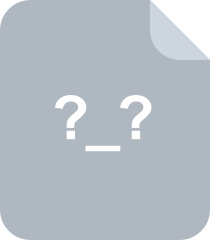
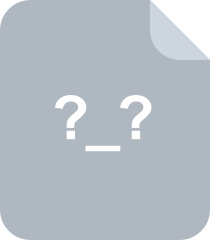
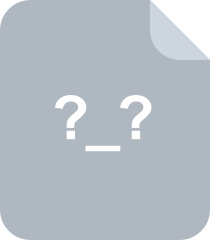
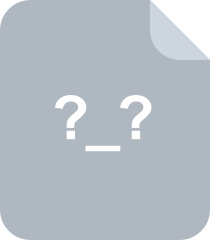
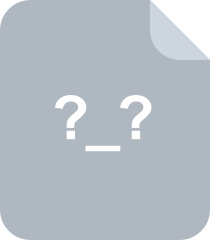
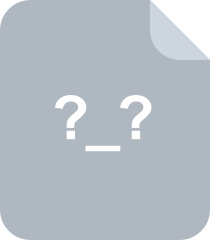
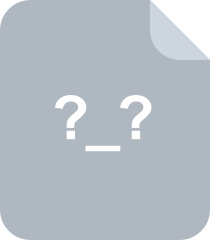
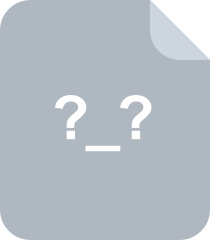
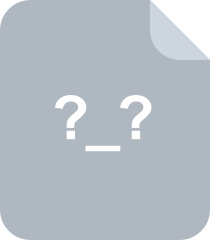
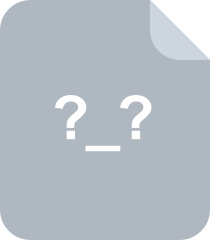
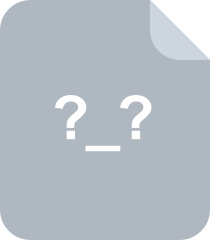
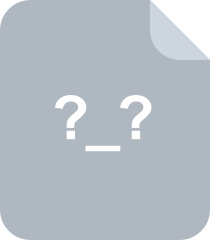
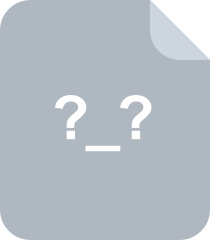
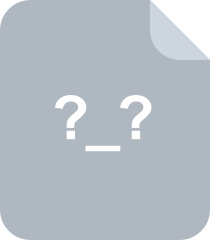
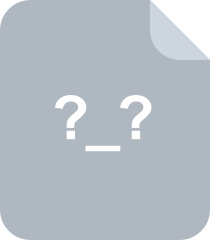
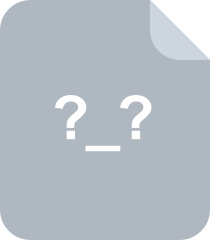
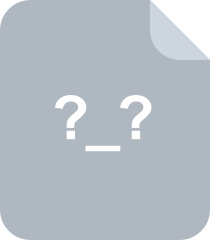
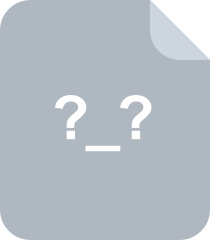
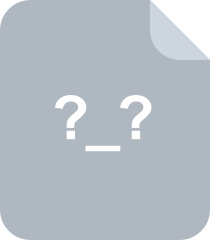
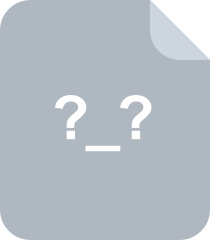
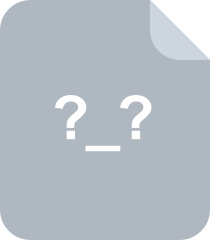
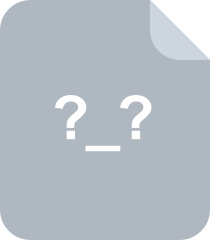
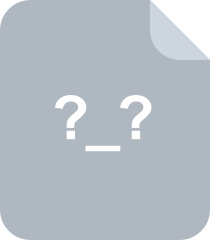
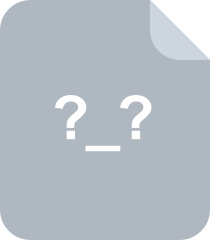
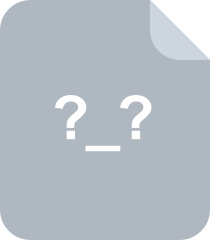
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
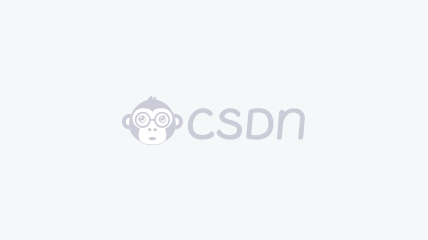

不走小道
- 粉丝: 3334
- 资源: 5059
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

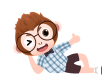
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


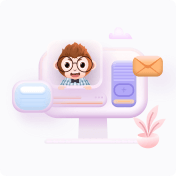
安全验证
文档复制为VIP权益,开通VIP直接复制
