function smadlmwrite(filename, m, varargin)
%DLMWRITE Write ASCII delimited file.
%
% DLMWRITE('FILENAME',M) writes matrix M into FILENAME using ',' as the
% delimiter to separate matrix elements.
%
% DLMWRITE('FILENAME',M,'DLM') writes matrix M into FILENAME using the
% character DLM as the delimiter.
%
% DLMWRITE('FILENAME',M,'DLM',R,C) writes matrix M starting at
% offset row R, and offset column C in the file. R and C are zero-based,
% so that R=C=0 specifies the first value in the file.
%
% DLMWRITE('FILENAME',M,'ATTRIBUTE1','VALUE1','ATTRIBUTE2','VALUE2'...)
% An alternative calling syntax that uses attribute value pairs for
% specifying optional arguments to DLMWRITE. The order of the
% attribute-value pairs does not matter, as long as an appropriate value
% follows each attribute tag.
%
% DLMWRITE('FILENAME',M,'-append') appends the matrix to the file.
% without the flag, DLMWRITE overwrites any existing file.
%
% DLMWRITE('FILENAME',M,'-append','ATTRIBUTE1','VALUE1',...)
% Is the same as the previous syntax, but accepts attribute value pairs,
% as well as the '-append' flag. The flag can be placed in the argument
% list anywhere between attribute value pairs, but not between an
% attribute and its value.
%
% USER CONFIGURABLE OPTIONS
%
% ATTRIBUTE : a quoted string defining an Attribute tag. The following
% attribute tags are valid -
% 'delimiter' => Delimiter string to be used in separating matrix
% elements.
% 'newline' => 'pc' Use CR/LF as line terminator
% 'unix' Use LF as line terminator
% 'roffset' => Zero-based offset, in rows, from the top of the
% destination file to where the data it to be
% written.
% 'coffset' => Zero-based offset, in columns, from the left side
% of the destination file to where the data is to be
% written.
% 'precision' => Numeric precision to use in writing data to the
% file, as significant digits or a C-style format
% string, starting with '%', such as '%10.5f'. Note
% that this uses the operating system standard
% library to truncate the number.
%
% EXAMPLES:
%
% DLMWRITE('abc.dat',M,'delimiter',';','roffset',5,'coffset',6,...
% 'precision',4) writes matrix M to row offset 5, column offset 6, in
% file abc.dat using ; as the delimiter between matrix elements. The
% numeric precision is of the data is set to 4 significant decimal
% digits.
%
% DLMWRITE('example.dat',M,'-append') appends matrix M to the end of
% the file example.dat. By default append mode is off, i.e. DLMWRITE
% overwrites the existing file.
%
% DLMWRITE('data.dat',M,'delimiter','\t','precision',6) writes M to file
% 'data.dat' with elements delimited by the tab character, using a precision
% of 6 significant digits.
%
% DLMWRITE('file.txt',M,'delimiter','\t','precision','%.6f') writes M
% to file file.txt with elements delimited by the tab character, using a
% precision of 6 decimal places.
%
% DLMWRITE('example2.dat',M,'newline','pc') writes M to file
% example2.dat, using the conventional line terminator for the PC
% platform.
%
% See also DLMREAD, CSVWRITE, CSVREAD, NUM2STR, TEXTSCAN, STRREAD,
% IMPORTDATA, SSCANF, SPRINTF, WK1WRITE, WK1READ.
% Brian M. Bourgault 10/22/93
% Modified: JP Barnard, 26 September 2002.
% Michael Theriault, 6 November 2003
% Copyright 1984-2007 The MathWorks, Inc.
% $Revision: 5.20.4.14 $ $Date: 2007/12/06 13:29:56 $
%-------------------------------------------------------------------------------
if nargin < 2
error('MATLAB:dlmwrite:Nargin','Requires at least 2 input arguments.');
end
% validate filename
if ~ischar(filename)
error('MATLAB:dlmwrite:InputClass','FILENAME must be a string.');
end;
% parse input and initialise parameters
try
%We support having cell arrays be printed out. Thus, if we get a cell
%array, with the same type in it, we will convert to a matrix.
if (iscell(m))
try
m = cell2mat(m);
catch
error('MATLAB:dlmwrite:CellArrayMismatch',...
'The input cell array cannot be converted to a matrix.');
end
end
[dlm,r,c,NEWLINE,precn,append] = ...
parseinput(length(varargin),varargin);
% construct complex precision string from specified format string
precnIsNumeric = isnumeric(precn);
if ischar(precn)
cpxprec = [precn strrep(precn,'%','%+') 'i'];
end
% set flag for char array to export
isCharArray = ischar(m);
catch exception
throw(exception);
end
% open the file
if append
fid = fopen(filename ,'Ab');
else
fid = fopen(filename ,'Wb');
end
% validate successful opening of file
if fid == (-1)
error('MATLAB:dlmwrite:FileOpenFailure',...
'Could not open file %s', filename);
end
% find size of matrix
[br,bc] = size(m);
% start with offsetting row of matrix
for i = 1:r
for j = 1:bc+c-1
fwrite(fid, dlm, 'uchar'); % write empty field
end
fwrite(fid, NEWLINE, 'char'); % terminate this line
end
% start dumping the array, for now number format float
realdata = isreal(m);
useVectorized = realdata && precnIsNumeric && isempty(strfind('%\',dlm)) ...
&& numel(dlm) == 1;
if useVectorized
format = sprintf('%%.%dg%s',precn,dlm);
end
if isCharArray
vectorizedChar = isempty(strfind('%\',dlm)) && numel(dlm) == 1;
format = sprintf('%%c%c',dlm);
end
for i = 1:br
% start with offsetting col of matrix
if c
for j = 1:c
fwrite(fid, dlm, 'uchar'); % write empty field
end
end
if isCharArray
if vectorizedChar
str = sprintf(format,m(i,:));
str = str(1:end-1);
fwrite(fid, str, 'uchar');
else
for j = 1:bc-1 % maybe only write once to file...
fwrite(fid, [m(i,j),dlm], 'uchar'); % write delimiter
end
fwrite(fid, m(i,bc), 'uchar');
end
elseif useVectorized
str = sprintf(format,m(i,:));
% strip off the last delimiter
str = str(1:end-1);
fwrite(fid, str, 'uchar');
else
rowIsReal = isreal(m(i,:));
for j = 1:bc
if rowIsReal || isreal(m(i,j))
% print real numbers
if precnIsNumeric
% use default precision or precision specified. Print as float
str = sprintf('%.*g',precn,m(i,j));
else
% use specified format string
str = sprintf(precn,m(i,j));
end
else
% print complex numbers
if precnIsNumeric
% use default precision or precision specified. Print as float
str = sprintf('%.*g%+.*gi',precn,real(m(i,j)),precn,imag(m(i,j)));
else
% use complex precision string
str = sprintf(cpxprec,real(m(i,j)),imag(m(i,j)));
end
end
if(j < bc)
str = [str,dlm];
end
fwrite(fid, str, 'uchar');
end
end
fwrite(fid, NEWLINE, 'char'); % terminate this line
end
% close file
fclose(fid);
%------------------------------------------------------------------------------
function [dlm,r,c,newline,precn,appendmode] = parseinput(options,varargin)
% initialise parameters
dlm = ',';
r = 0;
c = 0;
precn = 4;
appendmode = false;
newline = sprintf('\n');
if options > 0
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
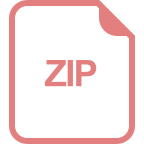
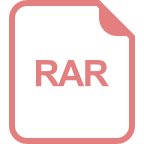
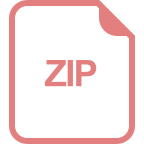
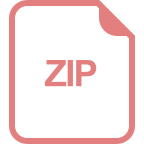
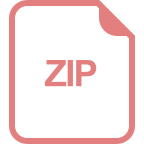
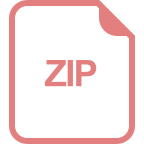
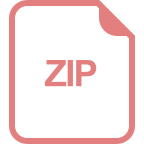
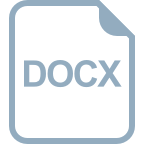
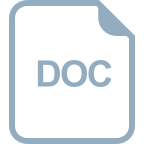
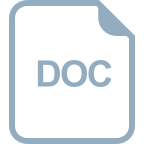
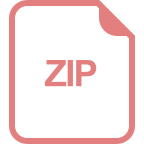
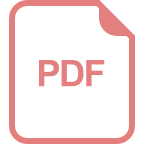
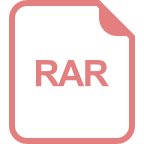
收起资源包目录

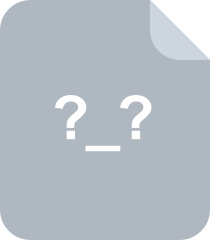
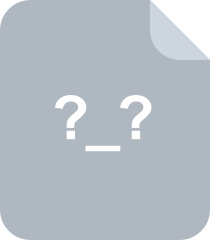
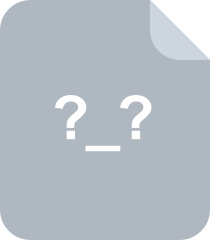
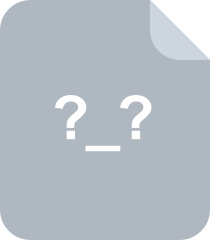
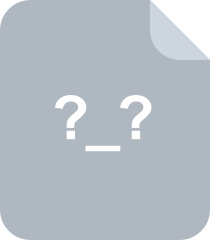
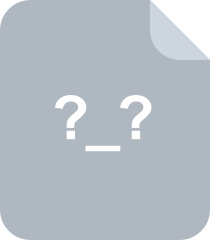
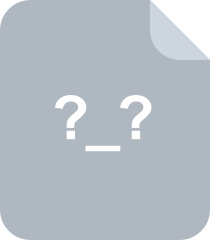
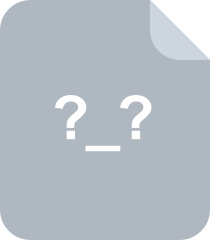
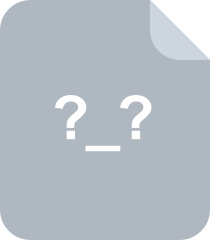
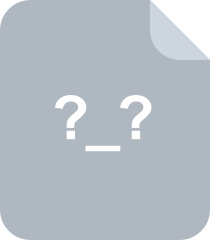
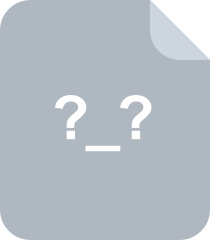
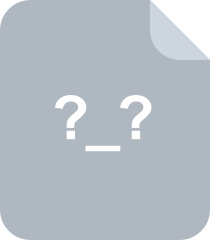
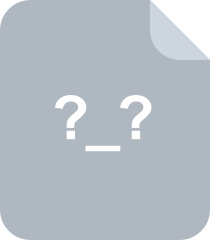
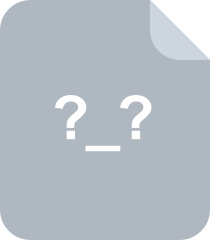
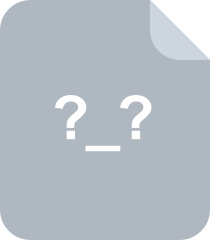
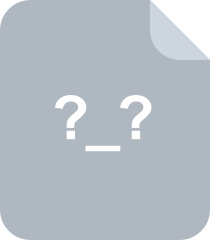
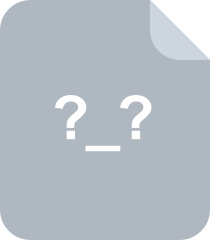
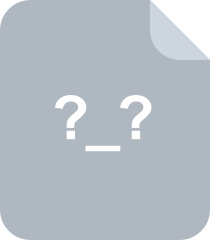
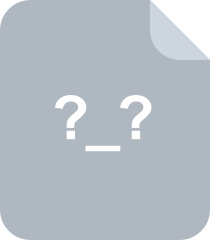
共 19 条
- 1
资源评论
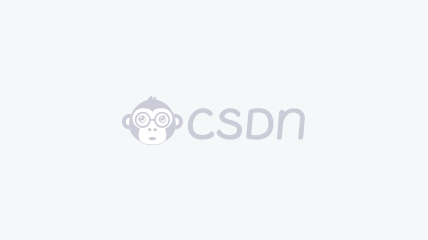
- 叉开裤裆任风吹2019-05-19就是一个垃圾资源,蹭份的,大家不要下

qq_467801491
- 粉丝: 2
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

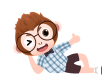
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


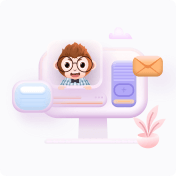
安全验证
文档复制为VIP权益,开通VIP直接复制
