/******************************************************************************\
* Copyright (C) 2012-2014 Leap Motion, Inc. All rights reserved. *
* Leap Motion proprietary and confidential. Not for distribution. *
* Use subject to the terms of the Leap Motion SDK Agreement available at *
* https://developer.leapmotion.com/sdk_agreement, or another agreement *
* between Leap Motion and you, your company or other organization. *
\******************************************************************************/
#if !defined(__Leap_h__)
#define __Leap_h__
#include "LeapMath.h"
#include <string>
#include <vector>
// Define integer types for Visual Studio 2008 and earlier
#if defined(_MSC_VER) && (_MSC_VER < 1600)
typedef __int32 int32_t;
typedef __int64 int64_t;
typedef unsigned __int32 uint32_t;
typedef unsigned __int64 uint64_t;
#else
#include <stdint.h>
#endif
// Define Leap export macros
#if defined(_MSC_VER) // Visual C++
#if LEAP_API_INTERNAL
#define LEAP_EXPORT
#elif LEAP_API_IMPLEMENTATION
#define LEAP_EXPORT __declspec(dllexport)
#else
#define LEAP_EXPORT __declspec(dllimport)
#endif
#define LEAP_EXPORT_CLASS
#define LEAP_EXPORT_PLUGIN __declspec(dllexport)
#elif !defined(SWIG)
#define LEAP_EXPORT __attribute__((visibility("default")))
#define LEAP_EXPORT_CLASS __attribute__((visibility("default")))
#define LEAP_EXPORT_PLUGIN __attribute__((visibility("default")))
#else
#define LEAP_EXPORT
#define LEAP_EXPORT_CLASS
#define LEAP_EXPORT_PLUGIN
#endif
namespace Leap {
// Interface for internal use only
class LEAP_EXPORT_CLASS Interface {
public:
struct Implementation {
LEAP_EXPORT virtual ~Implementation() {}
};
protected:
LEAP_EXPORT Interface(void* owner);
LEAP_EXPORT Interface(Implementation* reference, void* owner);
LEAP_EXPORT Interface(const Interface& rhs);
Interface(class SharedObject* object);
LEAP_EXPORT Interface& operator=(const Interface& rhs);
LEAP_EXPORT virtual ~Interface();
template<typename T> T* get() const { return static_cast<T*>(reference()); }
class SharedObject* m_object;
LEAP_EXPORT static void deleteCString(const char* cstr);
private:
LEAP_EXPORT Implementation* reference() const;
};
// Forward declarations for internal use only
class PointableImplementation;
class BoneImplementation;
class FingerImplementation;
class ToolImplementation;
class HandImplementation;
class GestureImplementation;
class ScreenImplementation;
class DeviceImplementation;
class InteractionBoxImplementation;
class FrameImplementation;
class ControllerImplementation;
template<typename T> class ListBaseImplementation;
// Forward declarations
class PointableList;
class FingerList;
class ToolList;
class HandList;
class GestureList;
class Hand;
class Gesture;
class Screen;
class InteractionBox;
class Frame;
class Listener;
/**
* The Pointable class reports the physical characteristics of a detected finger or tool.
*
* Both fingers and tools are classified as Pointable objects. Use the Pointable::isFinger()
* function to determine whether a Pointable object represents a finger. Use the
* Pointable::isTool() function to determine whether a Pointable object represents a tool.
* The Leap Motion software classifies a detected entity as a tool when it is thinner, straighter, and longer
* than a typical finger.
*
* \include Pointable_Get_Basic.txt
*
* To provide touch emulation, the Leap Motion software associates a floating touch
* plane that adapts to the user's finger movement and hand posture. The Leap Motion
* interprets purposeful movements toward this plane as potential touch points.
* The Pointable class reports
* touch state with the touchZone and touchDistance values.
*
* Note that Pointable objects can be invalid, which means that they do not contain
* valid tracking data and do not correspond to a physical entity. Invalid Pointable
* objects can be the result of asking for a Pointable object using an ID from an
* earlier frame when no Pointable objects with that ID exist in the current frame.
* A Pointable object created from the Pointable constructor is also invalid.
* Test for validity with the Pointable::isValid() function.
*
* @since 1.0
*/
class Pointable : public Interface {
public:
/**
* Defines the values for reporting the state of a Pointable object in relation to
* an adaptive touch plane.
* @since 1.0
*/
enum Zone {
/**
* The Pointable object is too far from the plane to be
* considered hovering or touching.
* @since 1.0
*/
ZONE_NONE = 0,
/**
* The Pointable object is close to, but not touching
* the plane.
* @since 1.0
*/
ZONE_HOVERING = 1,
/**
* The Pointable has penetrated the plane.
* @since 1.0
*/
ZONE_TOUCHING = 2,
};
// For internal use only.
Pointable(PointableImplementation*);
// For internal use only.
Pointable(FingerImplementation*);
// For internal use only.
Pointable(ToolImplementation*);
/**
* Constructs a Pointable object.
*
* An uninitialized pointable is considered invalid.
* Get valid Pointable objects from a Frame or a Hand object.
*
* \include Pointable_Pointable.txt
*
* @since 1.0
*/
LEAP_EXPORT Pointable();
/**
* A unique ID assigned to this Pointable object, whose value remains the
* same across consecutive frames while the tracked finger or tool remains
* visible. If tracking is lost (for example, when a finger is occluded by
* another finger or when it is withdrawn from the Leap Motion Controller field of view), the
* Leap Motion software may assign a new ID when it detects the entity in a future frame.
*
* \include Pointable_id.txt
*
* Use the ID value with the Frame::pointable() function to find this
* Pointable object in future frames.
*
* IDs should be from 1 to 100 (inclusive). If more than 100 objects are tracked
* an IDs of -1 will be used until an ID in the defined range is available.
*
* @returns The ID assigned to this Pointable object.
* @since 1.0
*/
LEAP_EXPORT int32_t id() const;
/**
* The Frame associated with this Pointable object.
*
* \include Pointable_frame.txt
*
* @returns The associated Frame object, if available; otherwise,
* an invalid Frame object is returned.
* @since 1.0
*/
LEAP_EXPORT Frame frame() const;
/**
* The Hand associated with this finger or tool.
*
* \include Pointable_hand.txt
*
* @returns The associated Hand object, if available; otherwise,
* an invalid Hand object is returned.
* @since 1.0
*/
LEAP_EXPORT Hand hand() const;
/**
* The tip position in millimeters from the Leap Motion origin.
*
* \include Pointable_tipPosition.txt
*
* @returns The Vector containing the coordinates of the tip position.
* @since 1.0
*/
LEAP_EXPORT Vector tipPosition() const;
/**
* The rate of change of the tip position in millimeters/second.
*
* \include Pointable_tipVelocity.txt
*
* @returns The Vector containing the coordinates of the tip velocity.
* @since 1.0
*/
LEAP_EXPORT Vector tipVelocity() const;
/**
* The direction in which this finger or tool is pointing.
*
* \include Pointable_direction.txt
*
* The direction is expressed as a unit vector pointing in the same
*
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
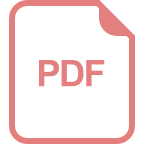
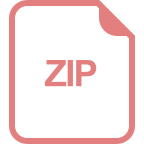
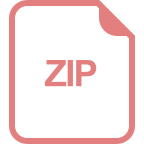
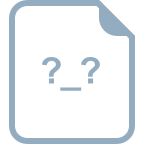
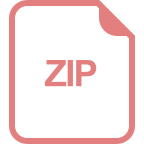
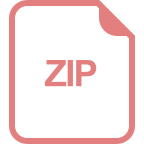
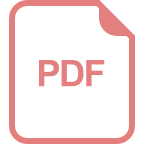
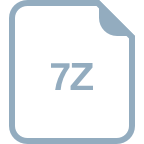
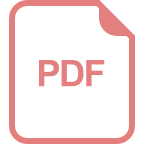
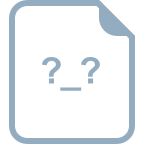
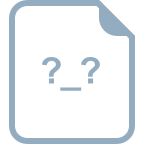
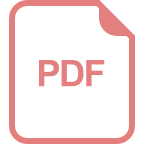
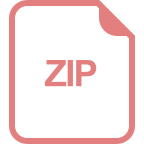
收起资源包目录

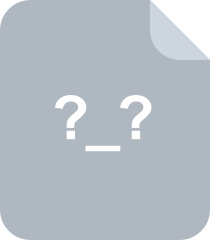
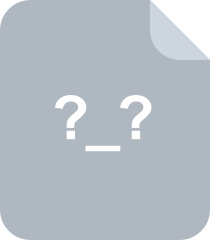
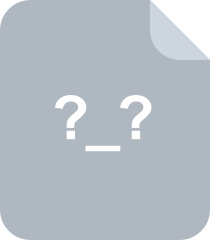
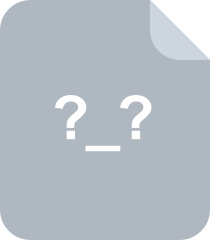
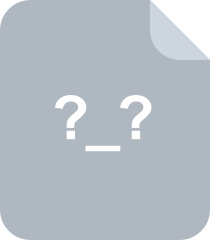
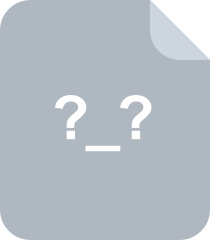
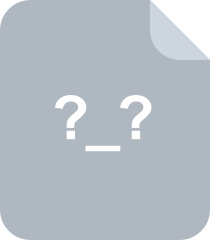
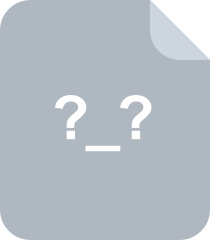
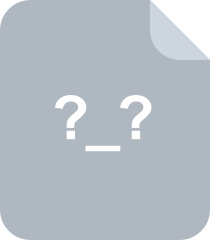
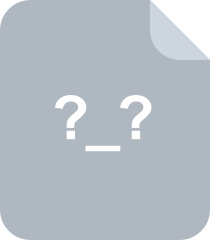
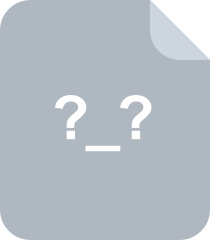
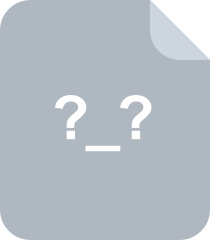
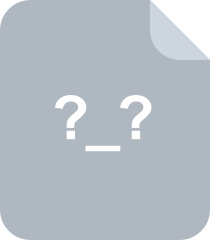
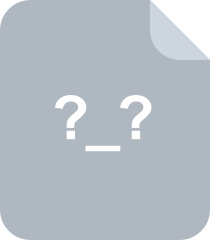
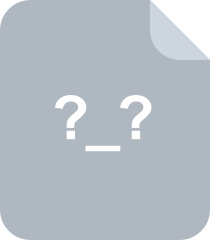
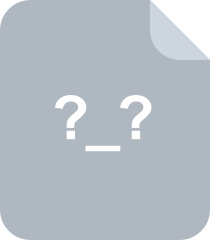
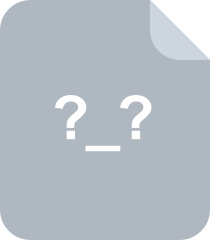
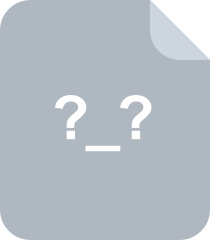
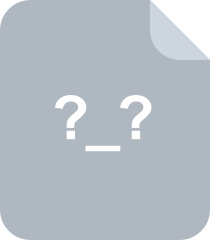
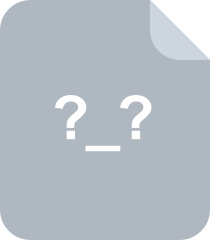
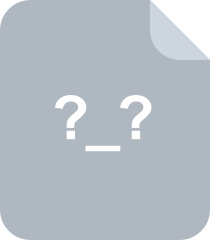
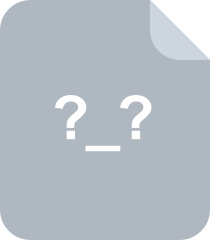
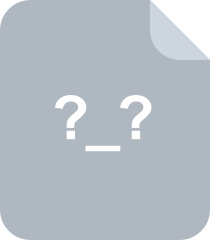
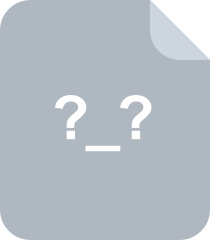
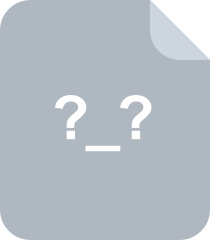
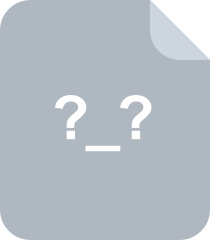
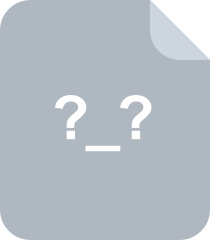
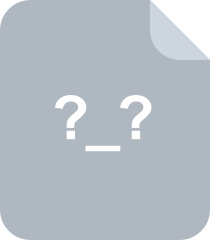
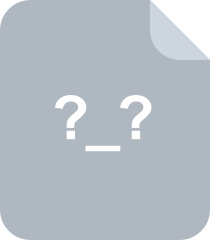
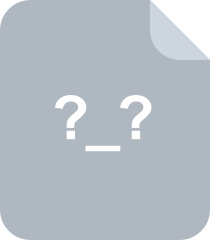
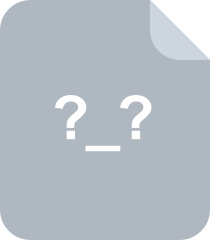
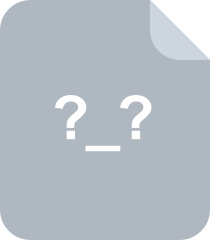
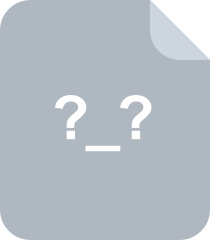
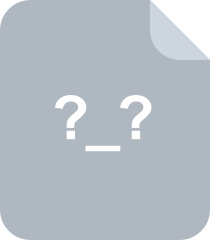
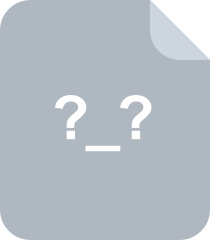
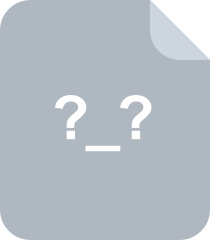
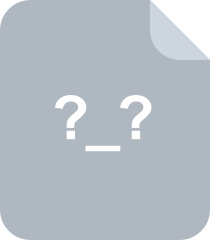
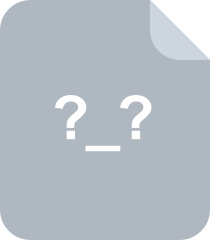
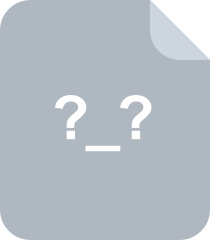
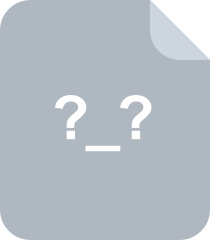
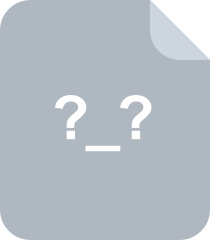
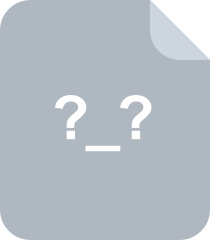
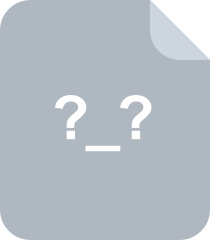
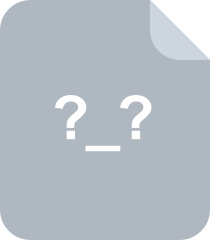
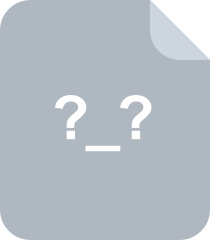
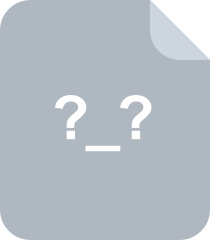
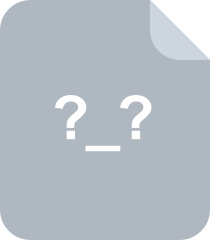
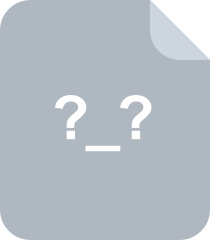
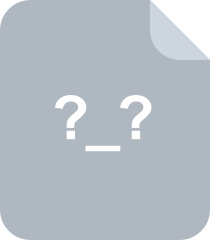
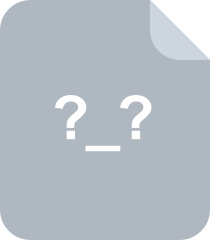
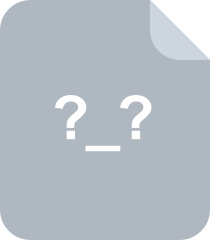
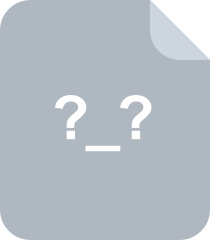
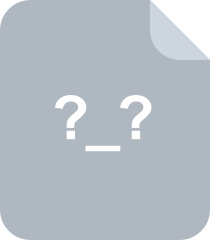
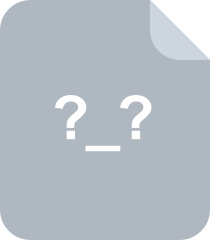
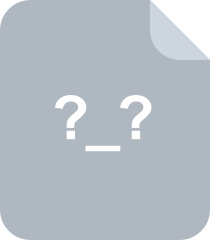
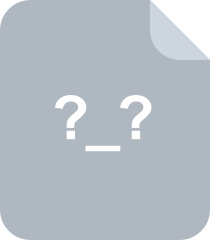
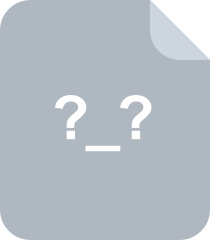
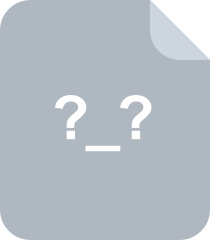
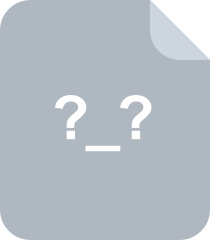
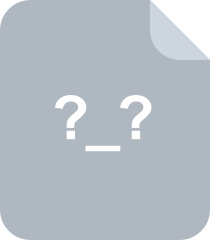
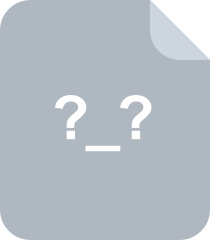
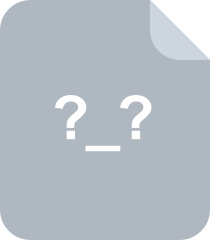
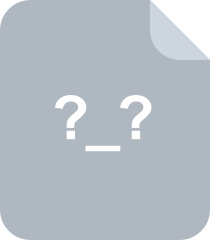
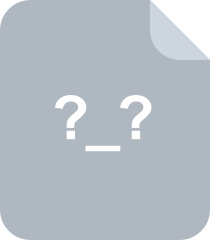
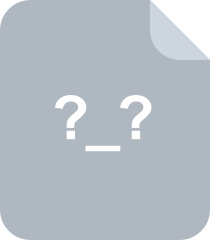
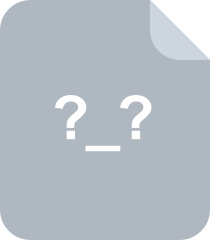
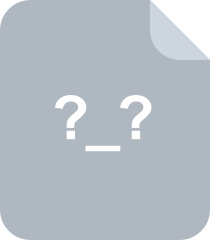
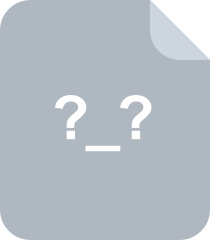
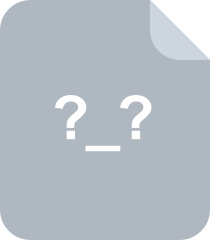
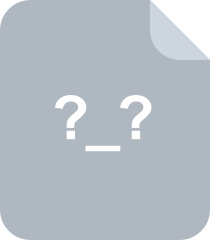
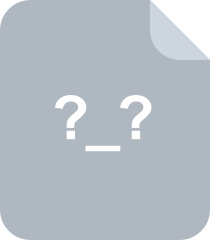
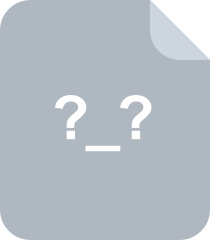
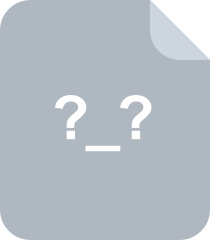
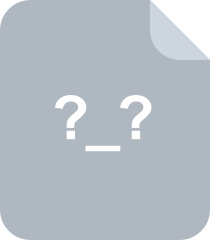
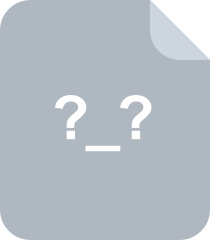
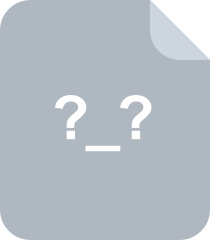
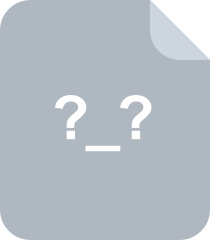
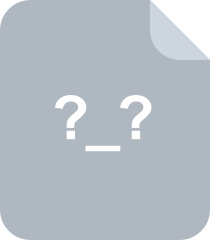
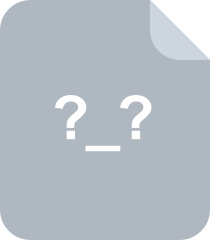
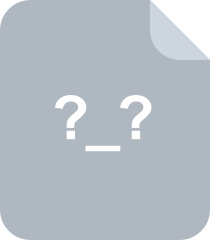
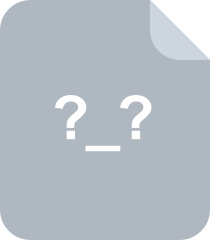
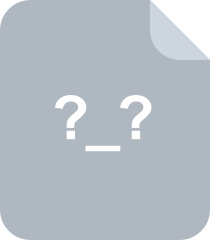
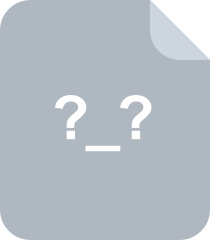
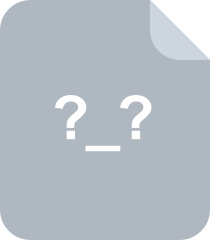
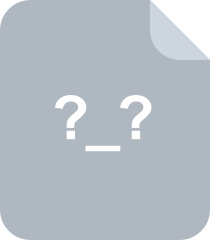
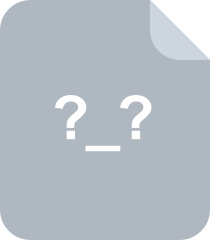
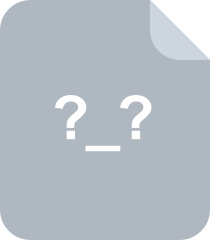
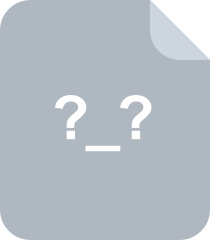
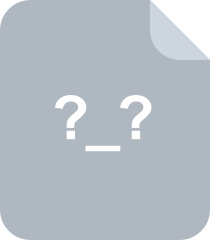
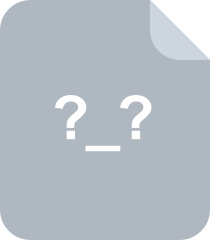
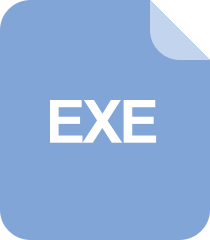
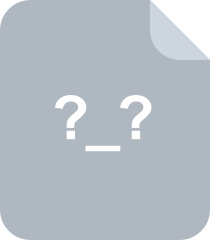
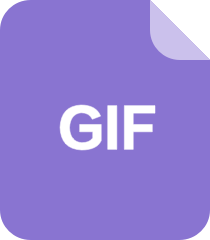
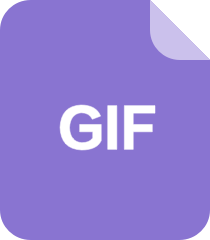
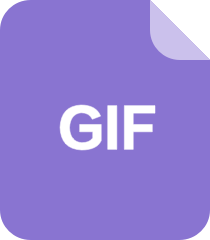
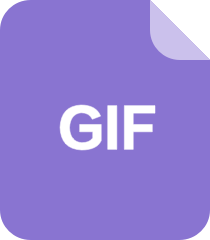
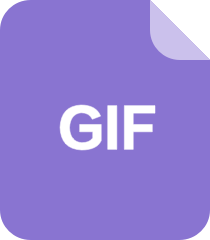
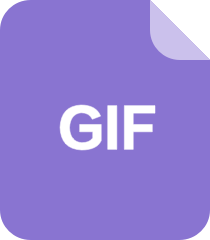
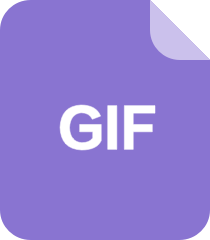
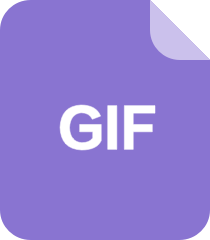
共 925 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
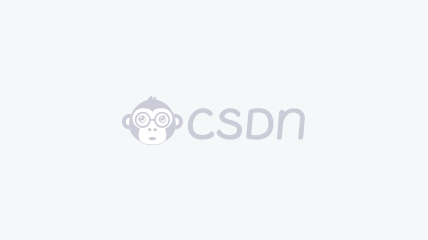
- lllrrryyy1232018-09-04程序可以运行,但是怎么把手指的各个角度信息记录到txt里呢,现在txt只有手的俯仰角、滚转角和偏航角,求指教,谢谢
- saviour_sjtu2017-09-30很好,感谢分享
- qq_376102782017-10-13很好,不错的例子
- lijichengjohn2015-06-17程序亲测通过,作者修改了SDK的samples,加了注释,很漂亮。
- K0_MAN2017-12-11还不知道效果

凯凯是坏男银
- 粉丝: 6
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

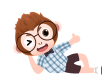
安全验证
文档复制为VIP权益,开通VIP直接复制
