<?php
/**
* 项目名称: Share Picture
* 文件名: view_image.php
* 描述: 图片详情页面处理
*
* @作者 公羊般锤 <3239223091@qq.com>
* @版权 2024 公羊般锤. 保留所有权利.
* @版本 2.0.0
*
* 修改记录:
* - 2024-11-23 公羊般锤: 完善前后样式,优化布局
*/
require_once 'includes/db_connect.php';
require_once 'includes/functions.php';
// 启动会话
if (session_status() == PHP_SESSION_NONE) {
session_start();
}
// 获取图片ID并验证
$image_id = isset($_GET['id']) ? intval($_GET['id']) : 0;
if ($image_id <= 0) {
die("无效的图片ID");
}
// 获取图片信息
$stmt = $conn->prepare("SELECT images.*, users.username FROM images JOIN users ON images.user_id = users.id WHERE images.id = ? AND is_public = 1");
$stmt->bind_param("i", $image_id);
$stmt->execute();
$image = $stmt->get_result()->fetch_assoc();
$stmt->close();
if (!$image) {
die("图片不存在或未公开");
}
// 生成图片URL
$protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off' || $_SERVER['SERVER_PORT'] == 443) ? "https://" : "http://";
$image_url = $protocol . $_SERVER['HTTP_HOST'] . "/" . $image['image_path'];
// 初始化变量
$is_liked = false;
$is_favorited = false;
// 如果用户已登录,检查是否已点赞或收藏
if (isset($_SESSION['user_id'])) {
$current_user_id = $_SESSION['user_id'];
// 检查是否已点赞
$stmt = $conn->prepare("SELECT id FROM likes WHERE user_id = ? AND image_id = ?");
$stmt->bind_param("ii", $current_user_id, $image_id);
$stmt->execute();
$stmt->store_result();
$is_liked = $stmt->num_rows > 0;
$stmt->close();
// 检查是否已收藏
$stmt = $conn->prepare("SELECT id FROM favorites WHERE user_id = ? AND image_id = ?");
$stmt->bind_param("ii", $current_user_id, $image_id);
$stmt->execute();
$stmt->store_result();
$is_favorited = $stmt->num_rows > 0;
$stmt->close();
}
// 处理点赞和收藏请求
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_SESSION['user_id'])) {
$current_user_id = $_SESSION['user_id'];
$action = $_POST['action'] ?? '';
if ($action === 'like') {
if ($is_liked) {
// 取消点赞
$stmt = $conn->prepare("DELETE FROM likes WHERE user_id = ? AND image_id = ?");
$stmt->bind_param("ii", $current_user_id, $image_id);
if ($stmt->execute()) {
// 减少图片点赞数
$stmt_update = $conn->prepare("UPDATE images SET likes = likes - 1 WHERE id = ? AND likes > 0");
$stmt_update->bind_param("i", $image_id);
$stmt_update->execute();
$_SESSION['message'] = "已取消点赞。";
} else {
$_SESSION['error'] = "取消点赞失败,请重试。";
}
$stmt->close();
} else {
// 添加点赞
$stmt = $conn->prepare("INSERT INTO likes (user_id, image_id) VALUES (?, ?)");
$stmt->bind_param("ii", $current_user_id, $image_id);
if ($stmt->execute()) {
// 增加图片点赞数
$stmt_update = $conn->prepare("UPDATE images SET likes = likes + 1 WHERE id = ?");
$stmt_update->bind_param("i", $image_id);
$stmt_update->execute();
$_SESSION['message'] = "已点赞。";
} else {
$_SESSION['error'] = "点赞失败,请重试。";
}
$stmt->close();
}
} elseif ($action === 'favorite') {
if ($is_favorited) {
// 取消收藏
$stmt = $conn->prepare("DELETE FROM favorites WHERE user_id = ? AND image_id = ?");
$stmt->bind_param("ii", $current_user_id, $image_id);
if ($stmt->execute()) {
// 减少图片收藏数
$stmt_update = $conn->prepare("UPDATE images SET favorites = favorites - 1 WHERE id = ? AND favorites > 0");
$stmt_update->bind_param("i", $image_id);
$stmt_update->execute();
$_SESSION['message'] = "已取消收藏。";
} else {
$_SESSION['error'] = "取消收藏失败,请重试。";
}
$stmt->close();
} else {
// 添加收藏
$stmt = $conn->prepare("INSERT INTO favorites (user_id, image_id) VALUES (?, ?)");
$stmt->bind_param("ii", $current_user_id, $image_id);
if ($stmt->execute()) {
// 增加图片收藏数
$stmt_update = $conn->prepare("UPDATE images SET favorites = favorites + 1 WHERE id = ?");
$stmt_update->bind_param("i", $image_id);
$stmt_update->execute();
$_SESSION['message'] = "图片已收藏。";
} else {
$_SESSION['error'] = "收藏失败,请重试。";
}
$stmt->close();
}
}
// 重定向回当前页面,防止表单重复提交
header("Location: view_image.php?id=" . $image_id);
exit();
}
// 刷新图片信息以获取最新的点赞和收藏数
$stmt = $conn->prepare("SELECT likes, favorites FROM images WHERE id = ?");
$stmt->bind_param("i", $image_id);
$stmt->execute();
$stmt->bind_result($image_likes, $image_favorites);
$stmt->fetch();
$stmt->close();
?>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>查看图片 - 图床</title>
<link rel="stylesheet" href="assets/css/styles.css">
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
color: #333;
line-height: 1.6;
display: flex;
flex-direction: column;
}
.container {
/*flex: 1;*/
max-width: 900px;
margin: 20px auto;
padding: 5px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
overflow: hidden;
}
h2 {
text-align: center;
margin-bottom: 20px;
color: #444;
}
.image-wrapper {
display: flex;
justify-content: center;
align-items: center;
margin-bottom: 20px;
max-height: 400px;
overflow: hidden;
}
.image-wrapper img {
max-width: 30%;
max-height: 100%;
height: auto;
border-radius: 8px;
}
.details {
display: flex;
flex-wrap: wrap;
gap: 10px;
margin-bottom: 20px;
}
.details p {
flex: 1 1 45%;
background-color: #f9f9f9;
padding: 10px;
border-radius: 5px;
}
.details p.full-width {
flex: 1 1 100%;
}
.actions {
display: flex;
flex-wrap: wrap;
gap: 10px;
justify-content: center;
margin-bottom: 20px;
}
.actions form {
flex: none;
}
.actions button {
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
font-size: 16px;
}
.button-liked {
background-color: #4CAF50;
color: white;
}
.button-liked:hover {
background-color: #45a049;
}
.button-favorited {
background-color: #008CBA;
color: white;
}
.button-favorited:hover {
background-color: #007bb5;
}
.copy-button {
background-color:
没有合适的资源?快使用搜索试试~ 我知道了~
图片分享系统网站源码,图床系统支持单张图片外链分享
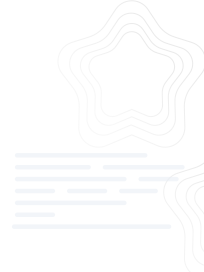
共24个文件
php:23个
css:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 174 浏览量
2024-12-02
08:17:01
上传
评论
收藏 37KB ZIP 举报
温馨提示
适合图片分享,不适合图床不支持批量上传!适合壁纸类 新增功能亮点 一键上传安装:为了简化安装过程,我们特别设计了一键上传安装的功能,让即使是初学者也能轻松完成部署。 用户系统优化:支持用户登录注册,每位用户都可以根据需要自定义其图片的公开或隐藏状态,并拥有删除等操作权限。 互动性增强:新增了点赞和收藏功能,用户可以对喜欢的图片进行点赞或添加至个人收藏夹,方便日后查看。 便捷的分享方式:提供外链复制和下载选项,使得图片的分享与获取变得更加便捷。 强大的后台管理系统:新版本中加入了后台管理模块,管理员可以轻松实现用户管理、图片管理等操作,并能够灵活地调整用户的公开隐藏权限设置。 安全性提升:支持用户修改密码,确保账户信息的安全性。
资源推荐
资源详情
资源评论
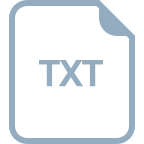
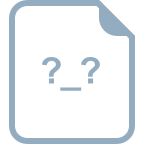
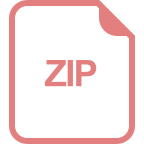
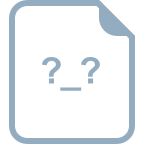
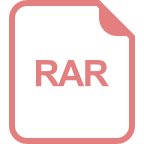
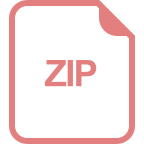
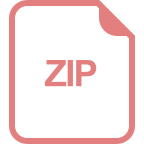
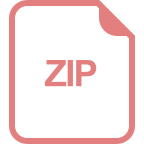
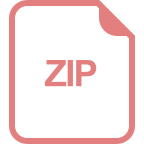
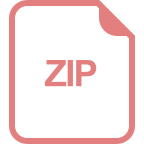
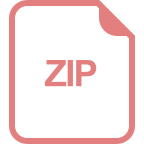
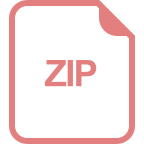
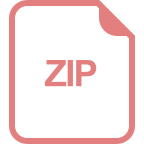
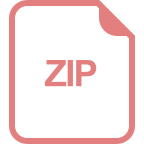
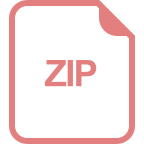
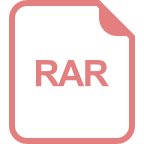
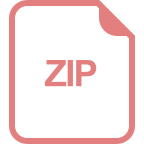
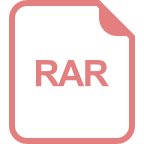
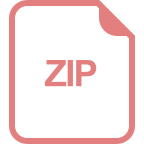
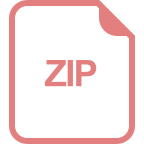
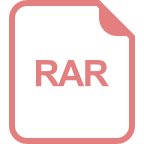
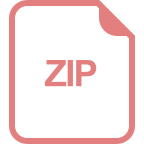
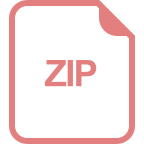
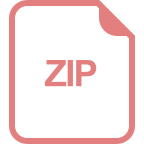
收起资源包目录


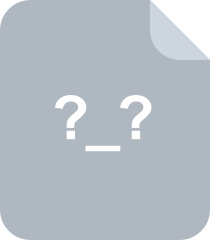
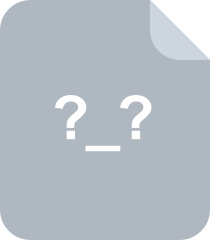
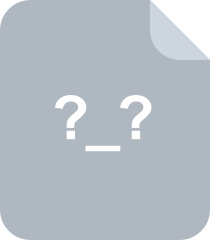
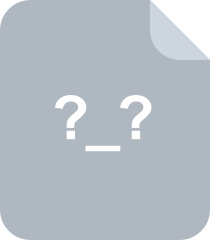
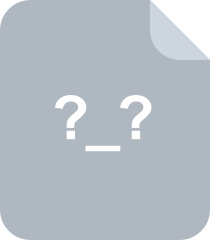
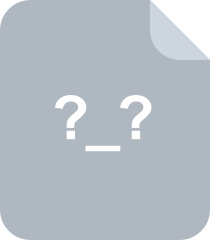


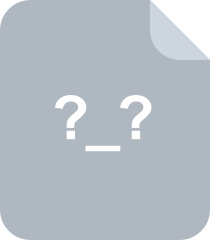

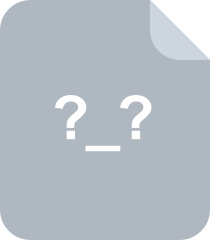
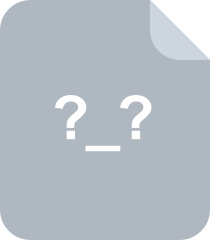
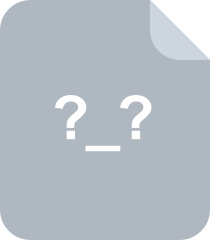
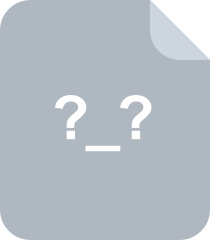
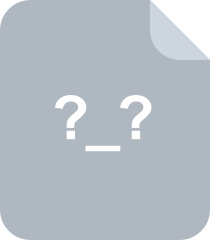
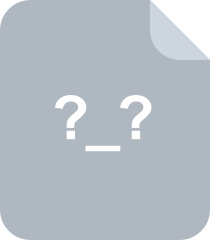

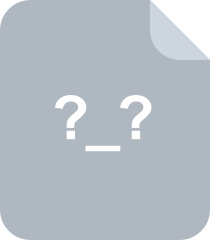
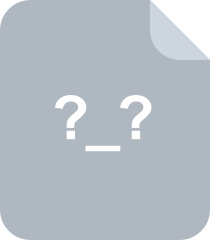
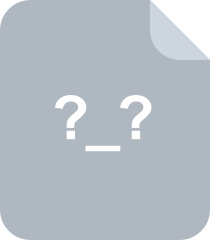
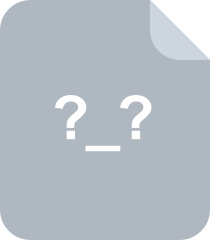
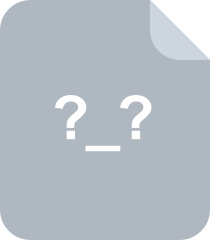
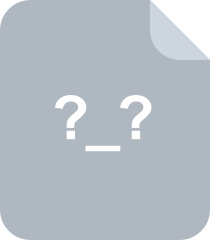
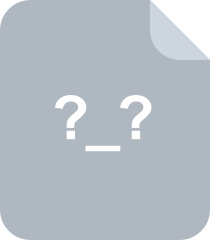
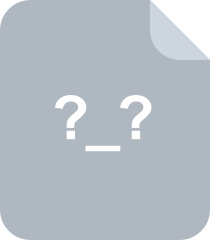
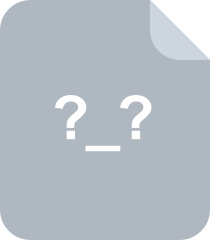

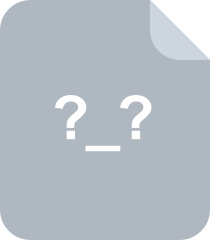
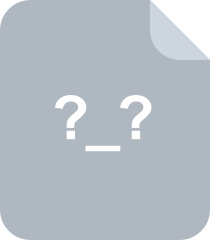
共 24 条
- 1
资源评论
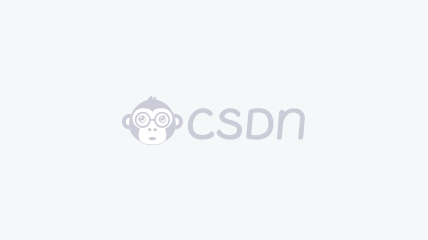

百创科技
- 粉丝: 2023
- 资源: 251
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

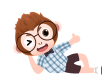
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


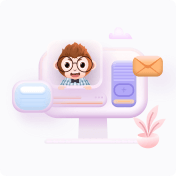
安全验证
文档复制为VIP权益,开通VIP直接复制
